Welcome to this new tutorial in the continuing series on JUnit. Thus far we have discussed following topics on JUnits:
Muliple Test Method & Test Classes
Now let's talk about how we can write our Selenium tests in Junit? or before answering this question, should we even write Selenium tests as part of Junit?
Junit is a framework for unit-level testing and Selenium is functional level tests. I would suggest that don't mix them, because Unit and Function level tests are completely different. Having said that, it doesn't mean that we cannot use Junit for writing our Selenium tests. Or I rather suggest you to use TestNG Framework, as it is much powerful than JUnit and it gives the beautiful HTML reports, which we actually need in Selenium.
For the sake of doing it lets write two Selenium tests in Junit and let's explore a few more annotations.
JUnit Selenium Test case
package unitTests;
import java.util.concurrent.TimeUnit;
import org.junit.AfterClass;
import org.junit.Assert;
import org.junit.BeforeClass;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
public class SeleniumTest {
private static FirefoxDriver driver;
WebElement element;
@BeforeClass
public static void openBrowser(){
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
}
@Test
public void valid_UserCredential(){
System.out.println("Starting test " + new Object(){}.getClass().getEnclosingMethod().getName());
driver.get("https://www.store.demoqa.com");
driver.findElement(By.xpath(".//*[@id='account']/a")).click();
driver.findElement(By.id("log")).sendKeys("testuser_3");
driver.findElement(By.id("pwd")).sendKeys("Test@123");
driver.findElement(By.id("login")).click();
try{
element = driver.findElement (By.xpath(".//*[@id='account_logout']/a"));
}catch (Exception e){
}
Assert.assertNotNull(element);
System.out.println("Ending test " + new Object(){}.getClass().getEnclosingMethod().getName());
}
@Test
public void inValid_UserCredential()
{
System.out.println("Starting test " + new Object(){}.getClass().getEnclosingMethod().getName());
driver.get("https://www.store.demoqa.com");
driver.findElement(By.xpath(".//*[@id='account']/a")).click();
driver.findElement(By.id("log")).sendKeys("testuser");
driver.findElement(By.id("pwd")).sendKeys("Test@123");
driver.findElement(By.id("login")).click();
try{
element = driver.findElement (By.xpath(".//*[@id='account_logout']/a"));
}catch (Exception e){
}
Assert.assertNotNull(element);
System.out.println("Ending test " + new Object(){}.getClass().getEnclosingMethod().getName());
}
@AfterClass
public static void closeBrowser(){
driver.quit();
}
}
What Just Happened...
Let just understand it block by block
- Before Class Annotation
@BeforeClass
public static void openBrowser()
{
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
}
Annotation before class will tell Junit to run this piece of code before starting any test. As you can see we did not start any browser in the test method 'valid_UserCredential()' & 'Invalid_UserCredential()'. So our test needs a browser to execute steps. With the help of the BeforeClass we would be able to open the browser before running the test.
- Test Method
@Test
public void valid_UserCredential(){
System.out.println("Starting test " + new Object(){}.getClass().getEnclosingMethod().getName());
driver.get("https://www.store.demoqa.com");
driver.findElement(By.xpath(".//*[@id='account']/a")).click();
driver.findElement(By.id("log")).sendKeys("testuser_3");
driver.findElement(By.id("pwd")).sendKeys("Test@123");
driver.findElement(By.id("login")).click();
try{
element = driver.findElement (By.xpath(".//*[@id='account_logout']/a"));
}catch (Exception e){
}
Assert.assertNotNull(element);
System.out.println("Ending test " + new Object(){}.getClass().getEnclosingMethod().getName());
}
This is a simple test of Log In functionality of our Demo application. But what you can notice in it is try/catch block & assert statement. This try block is to test the presence of the element, if it does not find it the element will remain as null. With that assert statement will verify that the element is not null. The basic rule of assert statement is that they act only on failure. We would be soon writing a complete chapter on Junit Asserts.
- After Class Method
@AfterClass
public static void closeBrowser(){
driver.quit();
}
This is to tell Junit engine that execute this piece of code once all the test has been executed. This will finally close the browser down, as after this no test would be needed any browser to execute any steps.
There are other annotations as well like @BeforeTest & @AfterTest. This is your exercise to use this annotation in your selenium examples.
Thanks for reading this. Any suggestion to improve my tutorials is welcomed at [email protected]
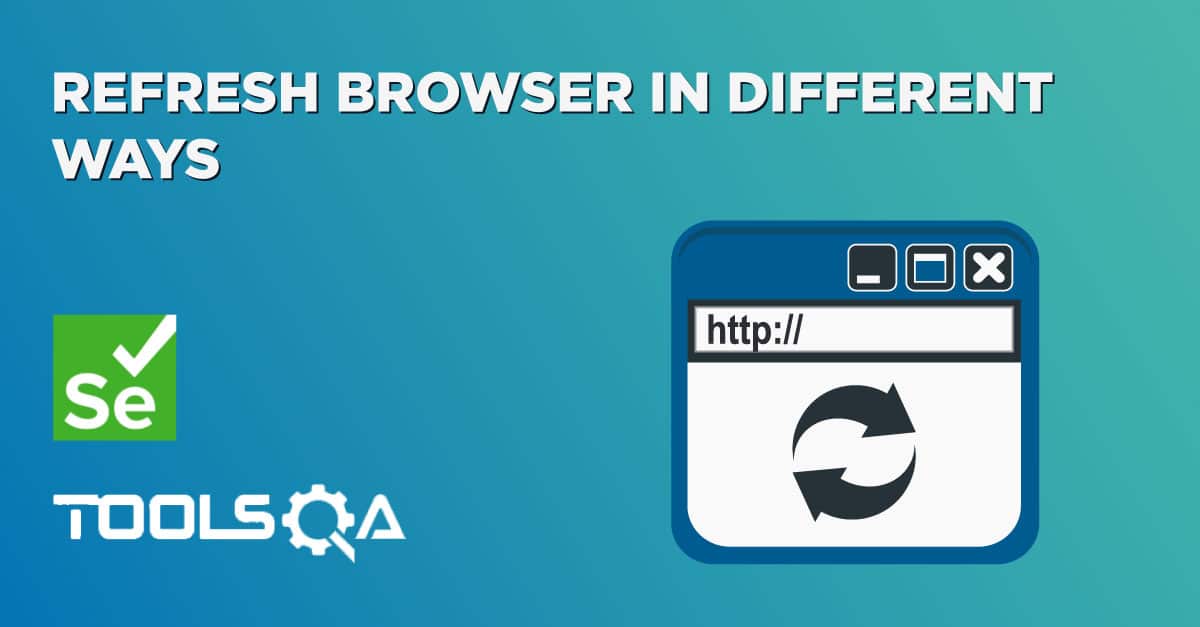
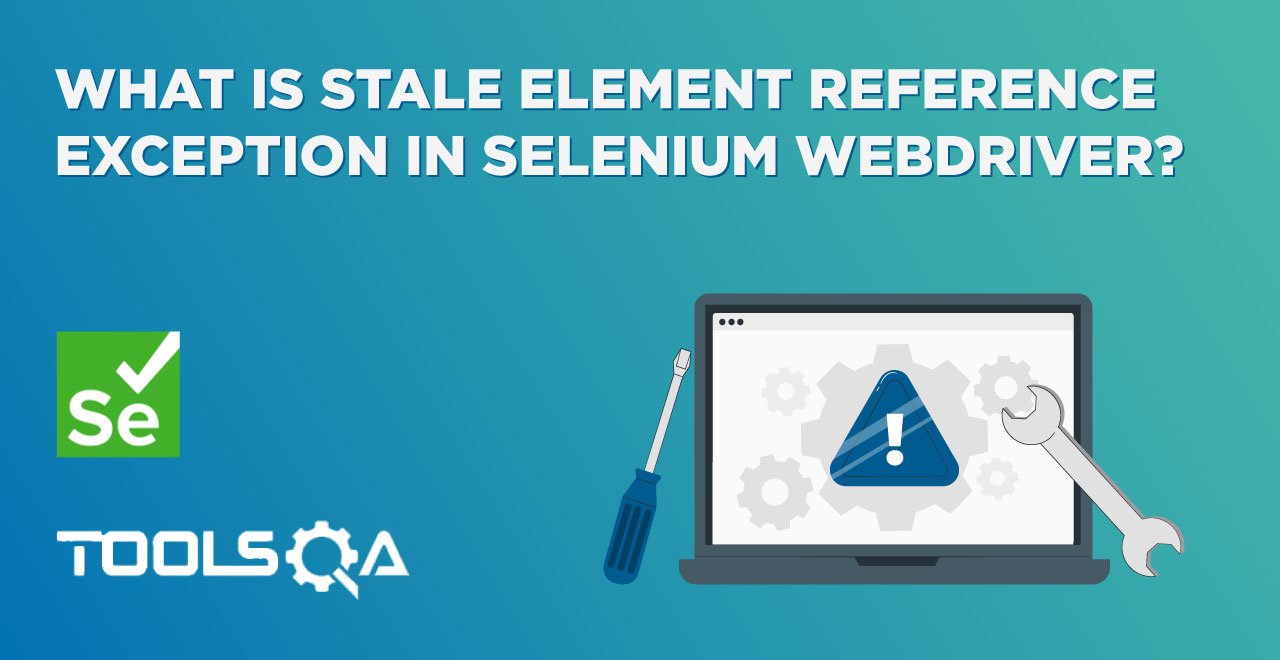