I welcome you to the series on Log4j. This is a very crucial off the shelf API that can be used to create logging infra structure in your tests. Let's start with Log4j Introduction.
Why logging is important in any application?
Logging is very important to any application. It helps us collect information about how the application is running and also helps us debug if any failure occurs.
What is Log4j?
Log4j is a brilliant logging API available both on Java and .net framework. Advantages are:
- Log4j allows you to have a very good logging infrastructure without putting in any effort.
- Log4j gives you the ability to categorize logs at different levels (Trace, Debug, Info, Warn, Error and Fatal).
- Log4j gives you the ability to direct logs to different outputs. For e.g. to a file, Console or a Database.
- Log4j gives you the ability to define the format of output logs.
- Log4j gives you the ability to write Asynchronous logs which helps to increase the performance of the application.
- Loggers in Log4j follow a class hierarchy that may come handy to your applications.
If you are not able to understand any of these points then don't worry. Things will get clearer as we approach to the end of Log4j Tutorial series.
Log4j
Log4j consists of four main components
- LogManager
- Loggers
- Appenders
- Layouts
With these comes some additional components which will be covered in the individual headings in the following tutorials.
LogManager
This is the static class that helps us get loggers with different names and hierarchy. You can consider LogManager as a factory producing logger objects. A sample code will be:
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
public class SampleEntry {
//mainLogger is a logger object that we got from LogManager. All loggers are
//using this method only. We can consider LogManager as a factory to create
//Logger objects
static Logger mainLogger = LogManager.getLogger(SampleEntry.class.getName());
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
mainLogger.info("This is just a logger");
}
}
Logger
This is a class that helps you log information at different logging levels. In the above sample code, you can see that we have created a logger named mainLogger using the LogManager static class. Now we can use it to write logs. As you can see we have mainLogger.info("Comments that you want to log") statement which logs the string.
Appenders
Appenders are objects which help Logger objects write logs to different outputs. Appenders can specify a file, console or a database as the output location.
In this code sample, you will see that we have used a console appender to print logs like we would do using System.out or System.err.
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.ConsoleAppender;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
public class SampleEntry {
//All the loggers that can be used
static Logger mainLogger = LogManager.getLogger(SampleEntry.class.getName());
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
//Create a console appender and attach it to our mainLogger
ConsoleAppender appender = new ConsoleAppender();
mainLogger.addAppender(appender);
mainLogger.info("This is just a logger");
}
}
Layouts
Layout class helps us define how the log information should appear in the outputs. Here is a sample code which uses PatternLayout Class to change the formatting of logs:
package Log4jSample;
import java.util.Enumeration;
import org.apache.log4j.Appender;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.ConsoleAppender;
import org.apache.log4j.Layout;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import org.apache.log4j.PatternLayout;
public class SampleEntry {
//All the loggers that can be used
static Logger mainLogger = LogManager.getLogger(SampleEntry.class.getName());
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
ConsoleAppender appender = new ConsoleAppender();
appender.activateOptions();
PatternLayout layoutHelper = new PatternLayout();
layoutHelper.setConversionPattern("%-5p [%t]: %m%n");
layoutHelper.activateOptions();
//mainLogger.getAppender("ConsoleAppender").setLayout(layoutHelper);
appender.setLayout(layoutHelper);
mainLogger.addAppender(appender);
//Create a console appender and attach it to our mainLogger
mainLogger.info("Pattern 1 is displayed like this");
layoutHelper.setConversionPattern("%C %m%n");
mainLogger.info("Pattern 2 is displayed like this");
}
}
Expected output on the console will be
INFO [main]: Pattern 1 is displayed like this Log4jSample.SampleEntry
Pattern 2 is displayed like this
This is a brief explanation of the different components inside Log4j APIs. Let's understand each component individually.
Thanks Virender
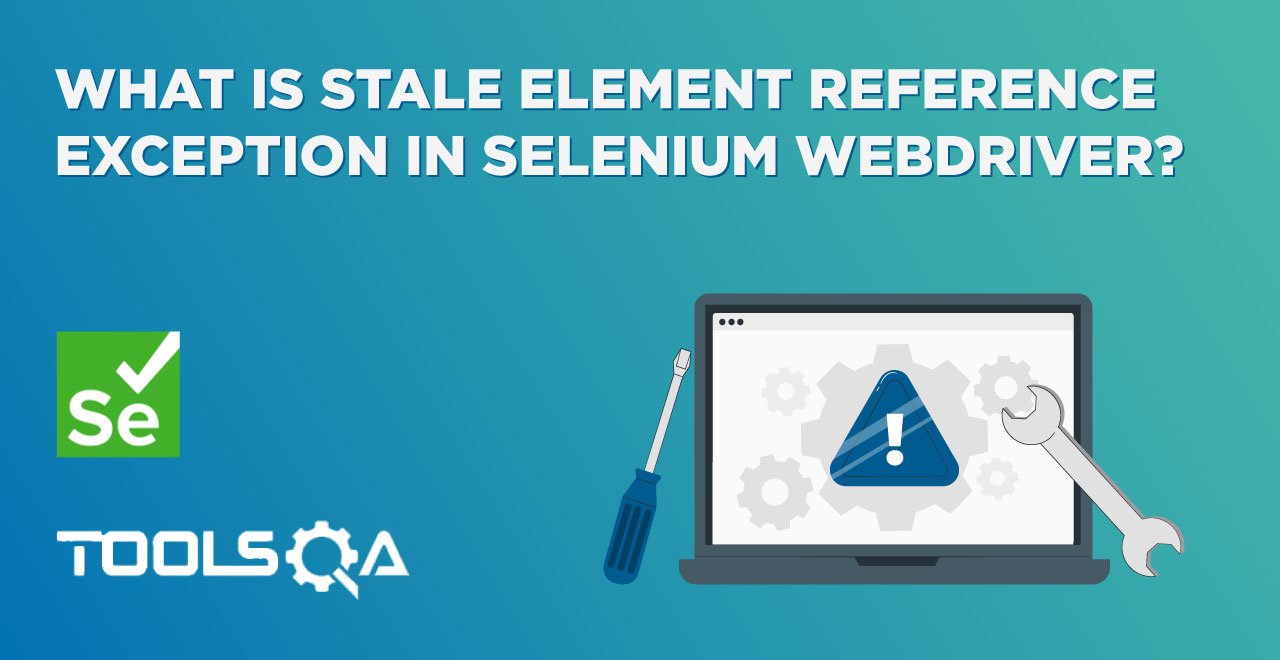
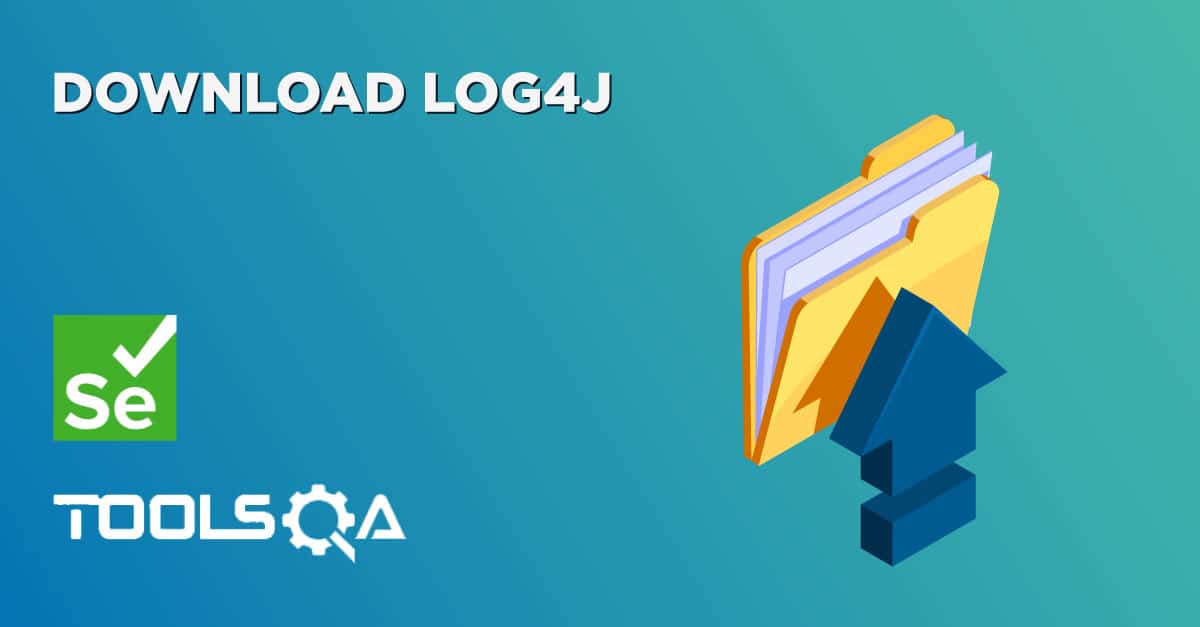