When you try to execute Selenium Script first time on IE Browser, there are good number of chances that you get some IE errors. As such there are five common issues that most people new to using Selenium IE face when getting started.
Selenium Internet Explorer (IE) Errors
In this chapter, we will cover the Challenges to run Selenium Scripts with IE Browser. These challenges are well documented on the Selenium Official Website. But still I often see these questions on various forums, so I want to capture this for the people who all are following my step by steps Selenium Tutorials.
- The path to the driver executable must be set by the webdriver.ie.driver
- Protected Mode settings are not the same for all zones.
- Unexpected error Browser zoom level
- SendKeys types character very slow when running script in IE browser
- Untrusted SSL certificate error in IE browser
Let's start discussing one by one.
Path to IE Driver
The very first issue anybody would face with IE Browser is that people expect IE Browser to work exactly like the Firefox Browser in Selenium. See what happens when you use the below Selenium Script to Open an application on IE Browser.
// Change the package name accordingly, as per your
project
package selenium;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class IEExplorerTest {
public static void main(String[] args) {
InternetExplorerDriver driver = new InternetExplorerDriver();
driver.get("https://demoqa.com");
}
}
Once the above code is executed, Selenium will throw an exception:
Exception in thread "main" java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.ie.driver system property; for more information, see http://code.google.com/p/selenium/wiki/InternetExplorerDriver. The latest version can be downloaded from http://selenium-release.storage.googleapis.com/index.html
The exception clearly says that the path to the driver must be set by the webdriver.ie.driver system property. This error typically is caused by either not having the required IEDriverServer.exe on your local machine or not having it set up in your PATH environment variable.
How to Set the System Property for IE Browser in Selenium Script
I hope you have gone through the chapter of Running Test in IE Explorer and you know how to download & from where to download IEDriverServer exe. I expect you have downloaded the IEDriverServer and saved it on to your local system at some xyz location.
Selenium Script would look like this:
// Change the package name accordingly, as per your project
package selenium;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class IEExplorerTest {
public static void main(String[] args) {
//Path to the folder where you have extracted the IEDriverServer executable
String service = "D:\\ToolsQA\\trunk\\Library\\drivers\\IEDriverServer.exe";
System.setProperty("webdriver.ie.driver", service);
InternetExplorerDriver driver = new InternetExplorerDriver();
driver.get("https://demoqa.com");
}
}
How to Set the Path Environment Variable for IE Browser
- Open the Control Panel -> System or Security –> System; the same thing can be done by right-clicking on 'MyComputer' and choosing Properties.
- Choose 'Advanced system settings'.
- Under the Advanced tab Choose the 'Environment Variable...' option.
4) Now we need to specify the location in the PATH variable. For PATH, most probably it already exists in your machine. So just select it and choose the Edit option.
- In the editor add the value 'Your Location of IE Browser' and click OK.
Note: The new values are separated by a semicolon from the existing ones and be careful and do not make any changes in the existing string, as it is very sensitive information.
Now try opening an IE Browser without specifying System Property in the Selenium Script.
// Change the package name accordingly, as per your project
package selenium;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class IEExplorerTest {
public static void main(String[] args) {
InternetExplorerDriver driver = new InternetExplorerDriver();
driver.get("https://demoqa.com");
}
}
Note: In case it does not work, please reboot the machine.
Protected Mode Settings
First, let's just see the code to Open an Internet Explorer driver using Selenium WebDriver Script.
package selenium;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class IEExplorerTest {
public static void main(String[] args) {
//Path to the folder where you have extracted the IEDriverServer executable
String service = "D:\\ToolsQA\\trunk\\Library\\drivers\\IEDriverServer.exe";
System.setProperty("webdriver.ie.driver", service);
InternetExplorerDriver driver = new InternetExplorerDriver();
driver.get("https://demoqa.com");
}
}
Once the above code is executed, Selenium will throw an exception:
Exception in thread "main" org.openqa.selenium.remote.SessionNotFoundException: Unexpected error launching Internet Explorer. Protected Mode settings are not the same for all zones. Enable Protected Mode must be set to the same value (enabled or disabled) for all zones. (WARNING: The server did not provide any stacktrace information)
Go through the above error, it clearly says that there is some issue with the Protected Mode Settings and the Protected Mode Settings are not the same for all the zones. To avoid this error we need to set the Protected Mode Settings the same for all the Zones.
How to set the Protected Mode settings in IE Browser
-
Go to Tools > Internet Options and Under Internet Options click on the Security tab.
-
Click on the Internet zone to Select a zone and to view its Protected Mode property.
-
Now check the Enable Protected Mode check-box. Whichever one you choose, needs to be set for all the other zones. This means either it can be OFF for all the zones or ON for all the zones.
- Repeat this task for all the zones
- Internet
- Local Intranet
- Trusted Sites
- Restricted Sites
- Click OK and run the Selenium script again. It will work this time.
Browser Zoom Level
If your application is not starting or you are just getting one or multiple InternetExplorerDriver Listening on port window messages or if the below exception is appeared in the Eclipse Console, then your IE browser’s zoom level might be set to something other than 100%.
Exception in thread "main" org.openqa.selenium.remote.SessionNotFoundException: Unexpected error launching Internet Explorer. Browser zoom level was set to 125%. It should be set to 100% (WARNING: The server did not provide any stacktrace information)
To fix make sure that the Internet Explorer’s Zoom property is set to 100%
SendKeys Slowness on IE Browser
This is again a very common issue with IE Browser that the Send key works very slow with Selenium script. But again not rocket science to resolve this issue. Simply replace the current IEDriverServer.exe (if your machine is 64-bit machine) with IEDriverServer.exe of 32 bit
- Download IEDriverServer.exe of the 32-bit system even if the system is a 64-bit machine. Download Link
- Extract the Zip file and place the IEDriverServer in the same folder where the earlier version of IEDriverServer is available. It will replace the old file with new.
- Run the script again and this time the sendsKey() would work and the way it works in Chrome and Firefox.
Untrusted SSL Certificate
Internet Explorer is the product of Microsoft and IE is much worried about security and IE is known as the most secured browser. At times using IE Browser with Selenium gives SLL Certificate pop up.
There are two ways to resolve the SLL Certificate issue.
Solution One
Add the below script just under the code to open the application:
driver.navigate().to(“javascript:document.getElementById(‘overridelink’).click()”);
Complete code will look like this:
// Change the package name accordingly, as per your project
package selenium;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class IEExplorerTest {
public static void main(String[] args) {
//Path to the folder where you have extracted the IEDriverServer executable
String service = "D:\\ToolsQA\\trunk\\Library\\drivers\\IEDriverServer.exe";
System.setProperty("webdriver.ie.driver", service);
InternetExplorerDriver driver = new InternetExplorerDriver();
driver.get("URL for which certificate error is coming");
driver.navigate().to("javascript:document.getElementById('overridelink').click()");
}
}
Solution Two
Another way of avoiding this error is to use DesiredCapability settings of the browser.
// Change the package name accordingly, as per your project
package selenium;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class IEExplorerTest {
public static void main(String[] args) {
// Path to the folder where you have extracted the IEDriverServer executable
String service = "D:\\ToolsQA\\trunk\\Library\\drivers\\IEDriverServer.exe";
System.setProperty("webdriver.ie.driver", service);
// Create the DesiredCapability object of InternetExplorer
DesiredCapabilities capabilities = DesiredCapabilities.internetExplorer();
// Settings to Accept the SSL Certificate in the Capability object
capabilities.setCapability(CapabilityType.ACCEPT_SSL_CERTS, true);
InternetExplorerDriver driver = new InternetExplorerDriver(capabilities);
driver.get("URL for which certificate error is coming");
}
}
After putting above code, run your script, this time no SSL Certificate Error would appear on screen and script would run fine.
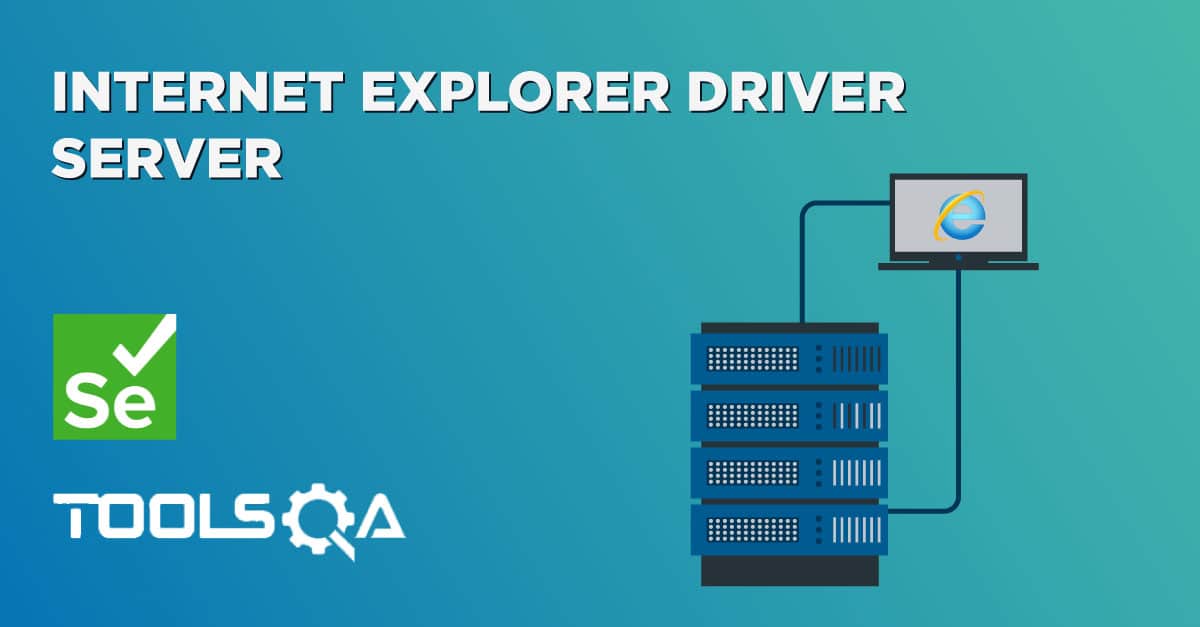
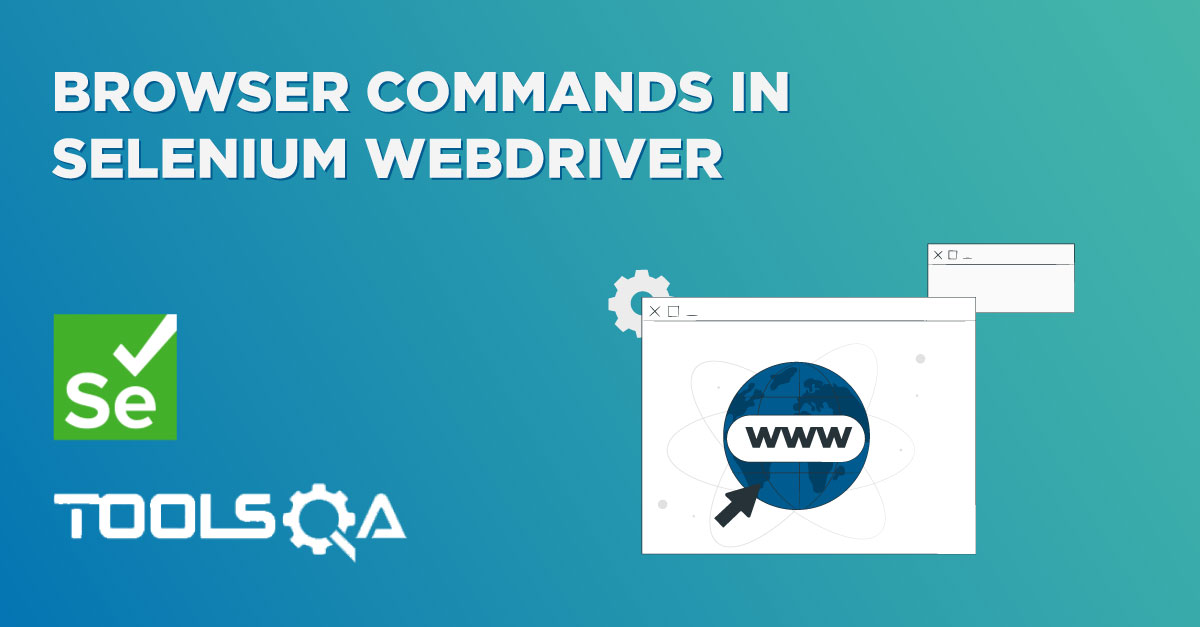