What is Data Driven Testing?
Data-driven testing means using a single test to verify many different test cases by driving the test with input and expected values from an external data source instead of using the same hard-coded values each time the test runs.
If you look into our framework, you will find that till now we have been passing values in the code in the hard coded form. For e.g. UserName & Password. So far only one user name and password are being used and that is being declared in the constants class but just think of a scenario when there will be N number of combinations to test for a log-in functionality. And this is just not limited to username & password only, even in the open browser method we have been using just one browser. In the real world, there will be many more browsers to test and to pass the browser to test case is necessary and that can only be done with the use of Data-driven technique.
One more flaw is there in our framework, as there are two different input methods for username and password which are 'input_UserName' & 'input_Password'. Which is not at all right, as we move along with writing more test of the application, we will find N number of input fields and we will end up with hundreds of method of input text field.
This situation can be overcome with the help of data-driven technique. Once we start with passing test data from the external source, we would not need different input text fields method. Only one method of input would be good enough for us, which will accept the test data.
As we have been driving everything from the Data Engine excel sheet, it is better to drive the set of test data from it as well.
Step 1: Set up Data Engine excel sheet
- Create an extra column in the test step sheet and name it as 'Data Set'. Insert this Data set column before the Results column.
- Enter the browser name, username, and password in the Data set column for the relevant rows.
- Replace the keyword 'input_Username' with just 'input' and do the same for 'input_Password' as well.
Test Steps sheet:
Step 2: Modify Constants class
- Create a new constant variable for column Data Set.
- As we have shifted the result columns, do not forget to increment the number of columns for the Result constant variable.
public static final int Col_DataSet =5 ;
public static final int Col_TestStepResult =6 ;
Step 3: Modify Action Keyword Class
- Change the method of Action Keyword class to accept data as an argument.
- As we have followed the concept of reflection class of java, we had to change each and every method of Action Keyword class to accept data as an argument, even if there is no data to be supplied for any action such as click() method. Click method just needs an object to click on, it does not need and test data but still, we need to change it to follow the rule of reflection, otherwise reflection won't work at all.
- Change the working code of the method input, replace the constants variable of username to data.
- Change the working code of the method open browser.
package config;
import java.util.concurrent.TimeUnit;
import static executionEngine.DriverScript.OR;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
import executionEngine.DriverScript;
import utility.Log;
public class ActionKeywords {
public static WebDriver driver;
//This block of code will decide which browser type to start
public static void openBrowser(String object,String data){
Log.info("Opening Browser");
try{
//If value of the parameter is Mozilla, this will execute
if(data.equals("Mozilla")){
driver=new FirefoxDriver();
Log.info("Mozilla browser started");
}
else if(data.equals("IE")){
//You may need to change the code here to start IE Driver
driver=new InternetExplorerDriver();
Log.info("IE browser started");
}
else if(data.equals("Chrome")){
driver=new ChromeDriver();
Log.info("Chrome browser started");
}
int implicitWaitTime=(10);
driver.manage().timeouts().implicitlyWait(implicitWaitTime, TimeUnit.SECONDS);
}catch (Exception e){
Log.info("Not able to open the Browser --- " + e.getMessage());
DriverScript.bResult = false;
}
}
public static void navigate(String object, String data){
try{
Log.info("Navigating to URL");
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
//Constant Variable is used in place of URL
driver.get(Constants.URL);
}catch(Exception e){
Log.info("Not able to navigate --- " + e.getMessage());
DriverScript.bResult = false;
}
}
public static void click(String object, String data){
try{
Log.info("Clicking on Webelement "+ object);
driver.findElement(By.xpath(OR.getProperty(object))).click();
}catch(Exception e){
Log.error("Not able to click --- " + e.getMessage());
DriverScript.bResult = false;
}
}
//Now this method accepts two value (Object name & Data)
public static void input(String object, String data){
try{
Log.info("Entering the text in " + object);
driver.findElement(By.xpath(OR.getProperty(object))).sendKeys(data);
}catch(Exception e){
Log.error("Not able to Enter UserName --- " + e.getMessage());
DriverScript.bResult = false;
}
}
public static void waitFor(String object, String data) throws Exception{
try{
Log.info("Wait for 5 seconds");
Thread.sleep(5000);
}catch(Exception e){
Log.error("Not able to Wait --- " + e.getMessage());
DriverScript.bResult = false;
}
}
public static void closeBrowser(String object, String data){
try{
Log.info("Closing the browser");
driver.quit();
}catch(Exception e){
Log.error("Not able to Close the Browser --- " + e.getMessage());
DriverScript.bResult = false;
}
}
}
Step 4: Modify Driver Engine script
- Create new variable for Test Data 'public static String sData;'
- Add a statement to assign value to data variable from the Data Set column of sheet Test Step
- In 'execute_Action()' block, pass 'sData' variable to invoke method.
package executionEngine;
import java.io.FileInputStream;
import java.lang.reflect.Method;
import java.util.Properties;
import org.apache.log4j.xml.DOMConfigurator;
import config.ActionKeywords;
import config.Constants;
import utility.ExcelUtils;
import utility.Log;
public class DriverScript {
public static Properties OR;
public static ActionKeywords actionKeywords;
public static String sActionKeyword;
public static String sPageObject;
public static Method method[];
public static int iTestStep;
public static int iTestLastStep;
public static String sTestCaseID;
public static String sRunMode;
public static String sData;
public static boolean bResult;
public DriverScript() throws NoSuchMethodException, SecurityException{
actionKeywords = new ActionKeywords();
method = actionKeywords.getClass().getMethods();
}
public static void main(String[] args) throws Exception {
ExcelUtils.setExcelFile(Constants.Path_TestData);
DOMConfigurator.configure("log4j.xml");
String Path_OR = Constants.Path_OR;
FileInputStream fs = new FileInputStream(Path_OR);
OR= new Properties(System.getProperties());
OR.load(fs);
DriverScript startEngine = new DriverScript();
startEngine.execute_TestCase();
}
private void execute_TestCase() throws Exception {
int iTotalTestCases = ExcelUtils.getRowCount(Constants.Sheet_TestCases);
for(int iTestcase=1;iTestcase<iTotalTestCases;iTestcase++){
bResult = true
sTestCaseID = ExcelUtils.getCellData(iTestcase, Constants.Col_TestCaseID, Constants.Sheet_TestCases);
sRunMode = ExcelUtils.getCellData(iTestcase, Constants.Col_RunMode,Constants.Sheet_TestCases);
if (sRunMode.equals("Yes")){
iTestStep = ExcelUtils.getRowContains(sTestCaseID, Constants.Col_TestCaseID, Constants.Sheet_TestSteps);
iTestLastStep = ExcelUtils.getTestStepsCount(Constants.Sheet_TestSteps, sTestCaseID, iTestStep);
Log.startTestCase(sTestCaseID);
bResult=true;
for (;iTestStep<iTestLastStep;iTestStep++){
sActionKeyword = ExcelUtils.getCellData(iTestStep, Constants.Col_ActionKeyword,Constants.Sheet_TestSteps);
sPageObject = ExcelUtils.getCellData(iTestStep, Constants.Col_PageObject, Constants.Sheet_TestSteps);
//Now we will use the data value and pass it to the methods
sData = ExcelUtils.getCellData(iTestStep, Constants.Col_DataSet, Constants.Sheet_TestSteps);
execute_Actions();
if(bResult==false){
ExcelUtils.setCellData(Constants.KEYWORD_FAIL,iTestcase,Constants.Col_Result,Constants.Sheet_TestCases);
Log.endTestCase(sTestCaseID);
break;
}
}
if(bResult==true){
ExcelUtils.setCellData(Constants.KEYWORD_PASS,iTestcase,Constants.Col_Result,Constants.Sheet_TestCases);
Log.endTestCase(sTestCaseID);
}
}
}
}
private static void execute_Actions() throws Exception {
for(int i=0;i<method.length;i++){
if(method[i].getName().equals(sActionKeyword)){
//This code will pass three parameters to every invoke method
method[i].invoke(actionKeywords,sPageObject, sData);
if(bResult==true){
ExcelUtils.setCellData(Constants.KEYWORD_PASS, iTestStep, Constants.Col_TestStepResult, Constants.Sheet_TestSteps);
break;
}else{
ExcelUtils.setCellData(Constants.KEYWORD_FAIL, iTestStep, Constants.Col_TestStepResult, Constants.Sheet_TestSteps);
ActionKeywords.closeBrowser("","");
break;
}
}
}
}
}
Note: For any explanation on un-commented code, please refer previous chapters.
You can see now the whole Keyword Driven Framework is set for use. Now it is up to you to implement other enhancements in this framework like you can implement HTML reporting in it or you can even implement some sort of automatic email reply on any test fail or an automatic email sent to the project stakeholders with test execution results or any thing.
But for me the next chapter is dam so important, so do not wait just jump on it.
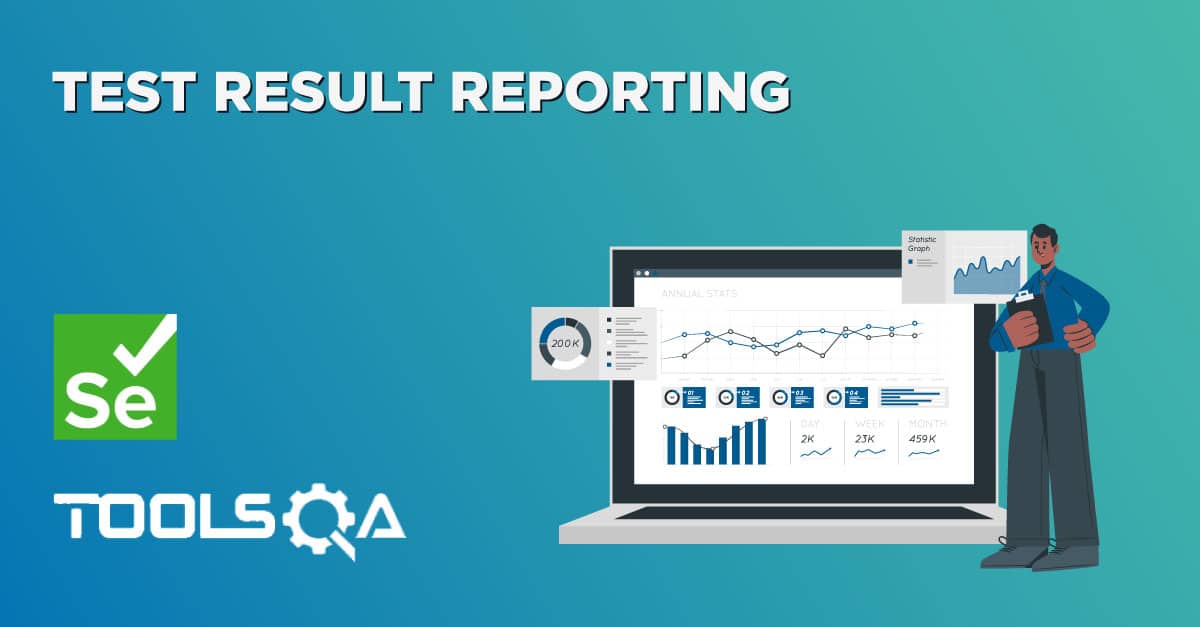
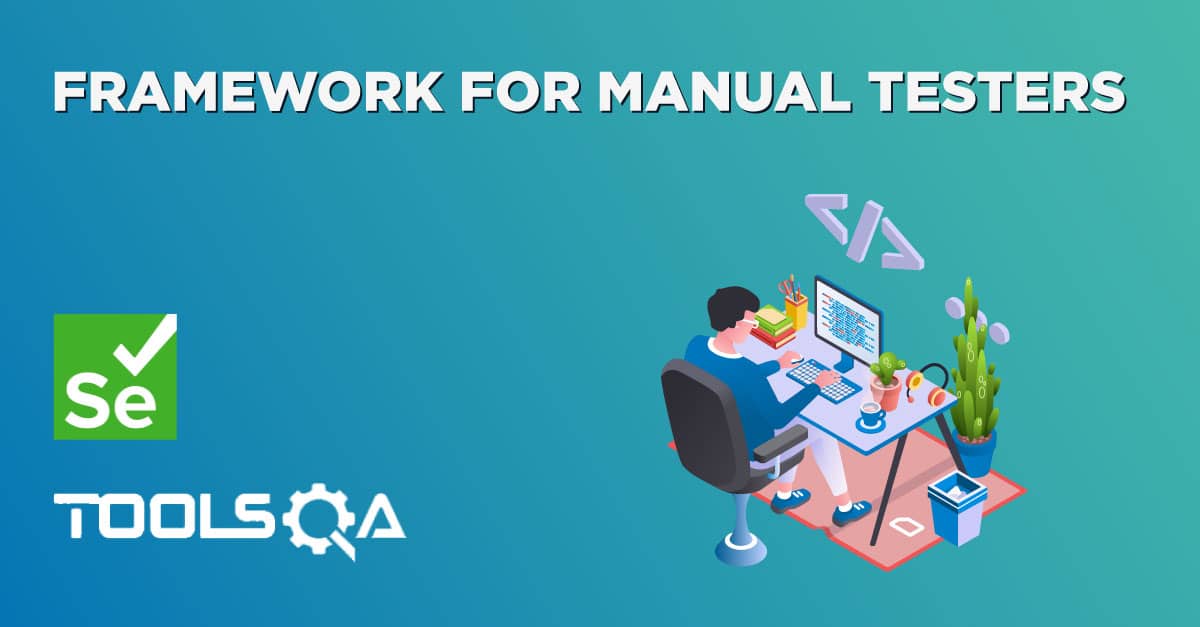