During the running of test case user wants some information to be logged in the console. Information could be any detail depends upon the purpose. Keeping this in mind that we are using Selenium for testing, we need the information which helps the User to understand the test steps or any failure during the test case execution. With the help of Log4j it is possible to enable loggings during the Selenium test case execution for e.g. let’s say you have encountered a failure in automation test script and it has to be reported in the system. The set of information that you have required to report a bug is :
- A complete test steps to replicate the scenario
- Issue, Description of the failure or reason for the failed test case
- Time stamp for the developers to investigate the issue in detail
Log4j helps us to acheive the above objectives in Selenium Webdriver. When logging is wisely used, it can prove to be an essential tool.
Logging inside the Methods
Logging inside the testcase is very tedious task and sooner or later you will find it boring and annoying. Plus everybody has their own way of writing log messages and messages can be less informative and confusing. So why not make it universal. Writing logs message inside the methods is much helpful way, with that one can avoid lots of confusions, save lot of time and maintain consistency.
Step 1: Set up Log4j
1) Download JAR files of Log4j and Add Jars to your project library. You can download it from here.
- Create a new XML file – 'log4j.xml' and place it under the Project root folder.
3) Paste the following code in the log4j.xml file.
<!DOCTYPE log4j:configuration SYSTEM "log4j.dtd">
<log4j:configuration xmlns:log4j="https://jakarta.apache.org/log4j/" debug="false">
<appender name="fileAppender" class="org.apache.log4j.FileAppender">
<param name="Threshold" value="INFO" />
<param name="File" value="logfile.log"/>
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern" value="%d %-5p [%c{1}] %m %n" />
</layout>
</appender>
<root>
<level value="INFO"/>
<appender-ref ref="fileAppender"/>
</root>
</log4j:configuration>
Note: After pasting the code make sure that the code is exactly same, as copying from HTML may change some symbols(“) to (?).
Step 2: Set up Static Log class
- Create a ‘New Class’ file and name it as 'Log', by right click on the 'utility' package and select New > Class.
- All the methods of this log class will be a static, so that it's methods can accessed in any of the class.
Log4j log Class:
package utility;
import org.apache.log4j.Logger;
public class Log {
//Initialize Log4j logs
private static Logger Log = Logger.getLogger(Log.class.getName());//
// This is to print log for the beginning of the test case, as we usually run so many test cases as a test suite
public static void startTestCase(String sTestCaseName){
Log.info("****************************************************************************************");
Log.info("****************************************************************************************");
Log.info("$$$$$$$$$$$$$$$$$$$$$ "+sTestCaseName+ " $$$$$$$$$$$$$$$$$$$$$$$$$");
Log.info("****************************************************************************************");
Log.info("****************************************************************************************");
}
//This is to print log for the ending of the test case
public static void endTestCase(String sTestCaseName){
Log.info("XXXXXXXXXXXXXXXXXXXXXXX "+"-E---N---D-"+" XXXXXXXXXXXXXXXXXXXXXX");
Log.info("X");
Log.info("X");
Log.info("X");
Log.info("X");
}
// Need to create these methods, so that they can be called
public static void info(String message) {
Log.info(message);
}
public static void warn(String message) {
Log.warn(message);
}
public static void error(String message) {
Log.error(message);
}
public static void fatal(String message) {
Log.fatal(message);
}
public static void debug(String message) {
Log.debug(message);
}
}
Step 3: Enter logs in Action Keyword Class
Action Keyword Class:
package config;
import java.util.concurrent.TimeUnit;
import static executionEngine.DriverScript.OR;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import utility.Log;
public class ActionKeywords {
public static WebDriver driver;
public static void openBrowser(String object){
Log.info("Opening Browser");
driver=new FirefoxDriver();
}
public static void navigate(String object){
Log.info("Navigating to URL");
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get(Constants.URL);
}
public static void click(String object){
Log.info("Clicking on Webelement "+ object);
driver.findElement(By.xpath(OR.getProperty(object))).click();
}
public static void input_UserName(String object){
Log.info("Entering the text in UserName");
driver.findElement(By.xpath(OR.getProperty(object))).sendKeys(Constants.UserName);
}
public static void input_Password(String object){
Log.info("Entering the text in Password");
driver.findElement(By.xpath(OR.getProperty(object))).sendKeys(Constants.Password);
}
public static void waitFor(String object) throws Exception{
Log.info("Wait for 5 seconds");
Thread.sleep(5000);
}
public static void closeBrowser(String object){
Log.info("Closing the browser");
driver.quit();
}
}
Step 4: Initialize Logs in Driver Script
- Once done with logging inside the methods of all the class, just initialize the logs from the main driver script.
Driver Script:
package executionEngine;
import java.io.FileInputStream;
import java.lang.reflect.Method;
import java.util.Properties;
import org.apache.log4j.xml.DOMConfigurator;
import config.ActionKeywords;
import config.Constants;
import utility.ExcelUtils;
import utility.Log;
public class DriverScript {
public static Properties OR;
public static ActionKeywords actionKeywords;
public static String sActionKeyword;
public static String sPageObject;
public static Method method[];
public static int iTestStep;
public static int iTestLastStep;
public static String sTestCaseID;
public static String sRunMode;
public DriverScript() throws NoSuchMethodException, SecurityException{
actionKeywords = new ActionKeywords();
method = actionKeywords.getClass().getMethods();
}
public static void main(String[] args) throws Exception {
ExcelUtils.setExcelFile(Constants.Path_TestData);
//This is to start the Log4j logging in the test case
DOMConfigurator.configure("log4j.xml");
String Path_OR = Constants.Path_OR;
FileInputStream fs = new FileInputStream(Path_OR);
OR= new Properties(System.getProperties());
OR.load(fs);
DriverScript startEngine = new DriverScript();
startEngine.execute_TestCase();
}
private void execute_TestCase() throws Exception {
int iTotalTestCases = ExcelUtils.getRowCount(Constants.Sheet_TestCases);
for(int iTestcase=1;iTestcase<=iTotalTestCases;iTestcase++){
sTestCaseID = ExcelUtils.getCellData(iTestcase, Constants.Col_TestCaseID, Constants.Sheet_TestCases);
sRunMode = ExcelUtils.getCellData(iTestcase, Constants.Col_RunMode,Constants.Sheet_TestCases);
if (sRunMode.equals("Yes")){
iTestStep = ExcelUtils.getRowContains(sTestCaseID, Constants.Col_TestCaseID, Constants.Sheet_TestSteps);
iTestLastStep = ExcelUtils.getTestStepsCount(Constants.Sheet_TestSteps, sTestCaseID, iTestStep);
Log.startTestCase(sTestCaseID);
for (;iTestStep<=iTestLastStep;iTestStep++){
sActionKeyword = ExcelUtils.getCellData(iTestStep, Constants.Col_ActionKeyword,Constants.Sheet_TestSteps);
sPageObject = ExcelUtils.getCellData(iTestStep, Constants.Col_PageObject, Constants.Sheet_TestSteps);
execute_Actions();
}
Log.endTestCase(sTestCaseID);
}
}
}
private static void execute_Actions() throws Exception {
for(int i=0;i<method.length;i++){
if(method[i].getName().equals(sActionKeyword)){
method[i].invoke(actionKeywords,sPageObject);
break;
}
}
}
}
Note: For any explanation on above non commented code, please refer previous chapters.
Logs would produce like this:
Now project would look like this:
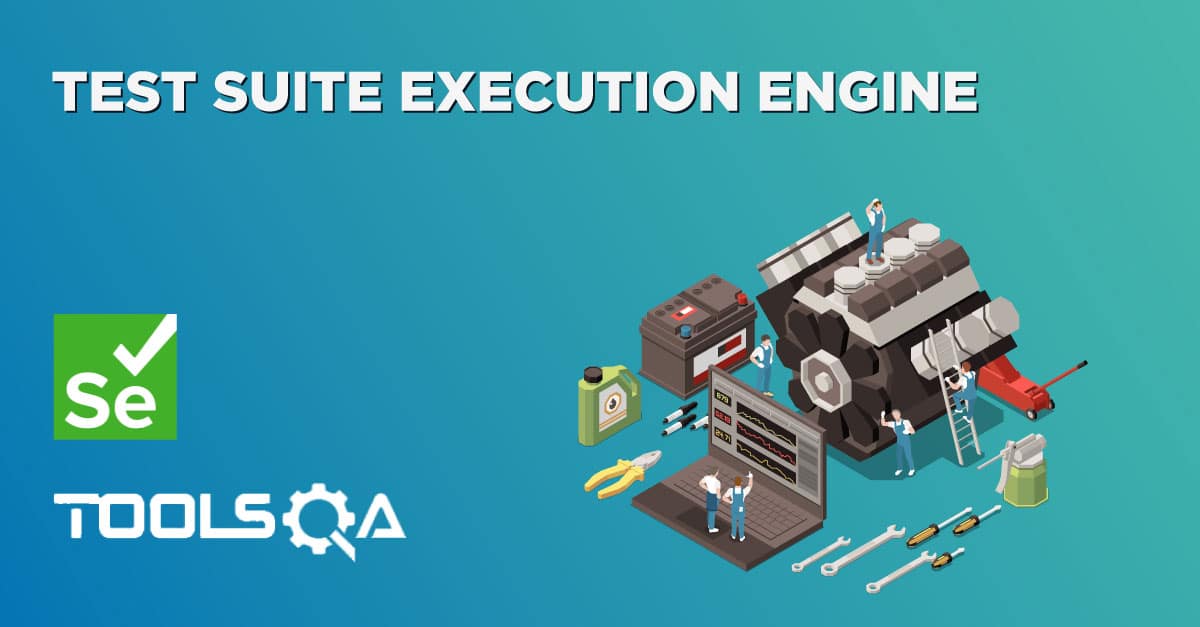
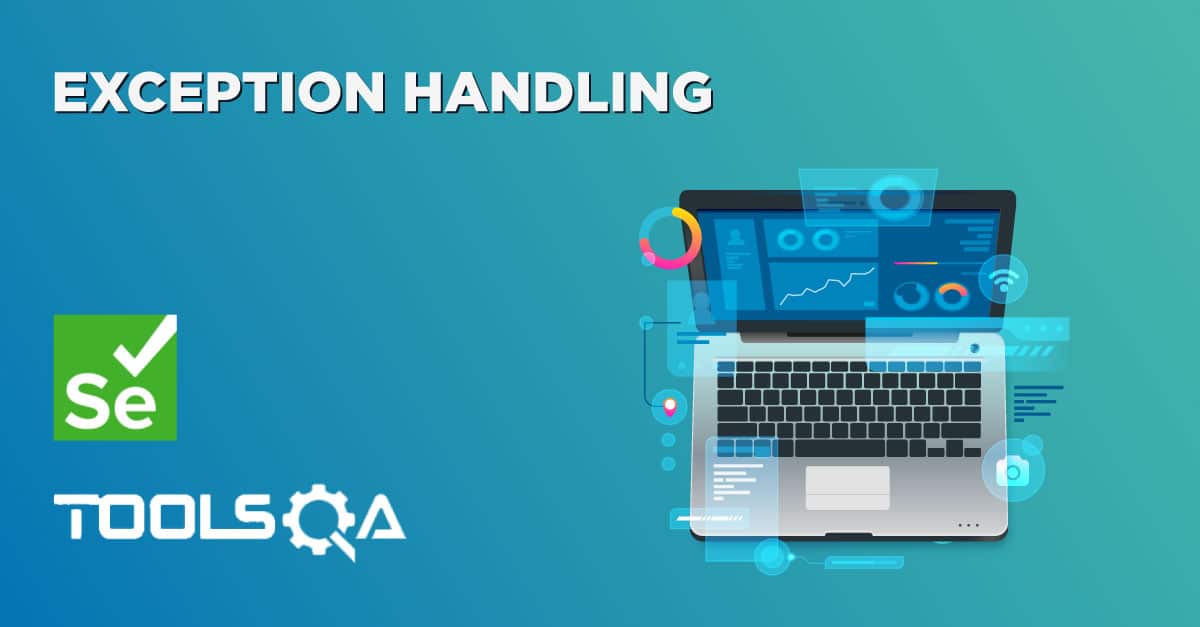