What is Table in HTML?
Table is a kind of HTML data which is displayed with the help of <table>
tag in conjunction with the <tr>
and <td>
tags. Although there are other tags for creating tables, these are the basics for creating a table in HTML. Here tag <tr>
defines the row and tag <td>
defines the column of the table.
Excel sheet is a simple example of table structures. Whenever we put some data into excel we give them some heading as well. In HTML we use <th>
tag for headings which defines heading of the table.
Each cell in the Excel sheet can be represented as <td>
in the HTML table. The <td>
elements are the data containers and these can contain all sorts of HTML elements like text, images, lists, other tables, etc.
Look at the below simple example of HTML table where the first row is defined as header and later two rows are containing data.
<table>
<tbody>
<tr>
<th>Automation Tool</th>
<th>Licensing</th>
<th>Market response</th>
</tr>
<tr>
<td>Selenium</td>
<td>Free</td>
<td>In</td>
</tr>
<tr>
<td>QTP</td>
<td>Paid</td>
<td>Out</td>
</tr>
</tbody>
</table>
Automation Tool | Licensing | Market response |
---|---|---|
Selenium | Free | In |
QTP | Paid | Out |
Handle Dynamic WebTables in Selenium Webdriver
There is no rocket science in the handling of tables. All you need to is to inspect the table cell and get the HTML location of it. In most cases, tables contain text data and you might simply like to extract the data given in each row or column of the table. But sometimes tables have links or images as well, and you can easily able to perform any action on those elements if you can find the HTML location of the containing cell.
Example 1:
Let's take an example of the above table and choose Column 1 of Row 2 which is 'Selenium' in the above case:
/html/body/div[1]/div[2]/div/div[2]/article/div/table/tbody/tr[2]/td[1]
If we divide this xpath into three different parts it will be like this
- Part 1 - Location of the table in the webpage </html/body/div[1]/div[2]/div/div[2]/article/div/>
- Part 2 - Table body (data) starts from here <table/tbody/>
- Part 3 - It says table row 2 and table column 1 <tr[2]/td[1]>
If you use this xpath you would be able to get the Selenium cell of the table. Now what? How to get the text 'Selenium' from the table cell?
You need to use the 'getText()' method of the WebDriver element.
driver.findElement(By.xpath("/html/body/div[1]/div[2]/div/div[2]/article/div/table/tbody/tr[2]/td[1]")).getText();
Example 2:
Table operations are not always so simple like above because tables can contain a large amount of data and may be you need to pass rows and columns dynamically in your test case.
In that case, you need to build your xpath with using variables and you will pass rows and columns to your xpath in the form of variables.
String sRow = "2";
String sCol = "1";
driver.findElement(By.xpath("/html/body/div[1]/div[2]/div/div[2]/article/div/table/tbody/tr["+sRow+"]/td["+sCol+"]")).getText();
Example 3:
The above example is still easy as at-least you know the row number and the column number to be fetched from the table and you are able to provide it in the xpath from external excel sheet or any source of data sheet. But what would you do when the row and columns are itself dynamic and all you know is the Text value of any cell only and you like to take out the corresponding values of that particular cell.
For example, all you know is the Text 'Licensing' in the above example and you like to record the value for that particular column only such as Free & Paid.
String sColValue = "Licensing";
//First loop will find the 'ClOCK TWER HOTEL' in the first column
for (int i=1;i<=3;i++){
String sValue = null;
sValue = driver.findElement(By.xpath(".//*[@id='post-2924']/div/table/tbody/tr[1]/th["+i+"]")).getText();
if(sValue.equalsIgnoreCase(sColValue)){
// If the sValue match with the description, it will initiate one more inner loop for all the columns of 'i' row
for (int j=1;j<=2;j++){
String sRowValue= driver.findElement(By.xpath(".//*[@id='post-2924']/div/table/tbody/tr["+j+"]/td["+i+"]")).getText();
System.out.println(sRowValue);
}
break;
}
}
Practice Exercise 1
- Launch new Browser
- Open URL “http://toolsqa.com/automation-practice-table/”
- Get the value from cell 'Dubai' and print it on the console
- Click on the link 'Detail' of the first row and last column
package automationFramework;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class PracticeTables {
public static void main(String[] args) {
WebDriver driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://toolsqa.com/automation-practice-table");
//Here we are storing the value from the cell in to the string variable
String sCellValue = driver.findElement(By.xpath(".//*[@id='content']/table/tbody/tr[1]/td[2]")).getText();
System.out.println(sCellValue);
// Here we are clicking on the link of first row and the last column
driver.findElement(By.xpath(".//*[@id='content']/table/tbody/tr[1]/td[6]/a")).click();
System.out.println("Link has been clicked otherwise an exception would have thrown");
driver.close();
}
}
Practice Exercise 2
- Launch new Browser
- Open URL “http://toolsqa.com/automation-practice-table/”
- Get the value from cell 'Dubai' with using dynamic xpath
- Print all the column values of row 'Clock Tower Hotel'
package automationFramework;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class PracticeTable_2 {
public static void main(String[] args) {
WebDriver driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://toolsqa.com/automation-practice-table");
String sRow = "1";
String sCol = "2";
//Here we are locating the xpath by passing variables in the xpath
String sCellValue = driver.findElement(By.xpath(".//*[@id='content']/table/tbody/tr[" + sRow + "]/td[" + sCol + "]")).getText();
System.out.println(sCellValue);
String sRowValue = "Clock Tower Hotel";
//First loop will find the 'ClOCK TWER HOTEL' in the first column
for (int i=1;i<=5;i++){
String sValue = null;
sValue = driver.findElement(By.xpath(".//*[@id='content']/table/tbody/tr[" + i + "]/th")).getText();
if(sValue.equalsIgnoreCase(sRowValue)){
// If the sValue match with the description, it will initiate one more inner loop for all the columns of 'i' row
for (int j=1;j<=5;j++){
String sColumnValue= driver.findElement(By.xpath(".//*[@id='content']/table/tbody/tr[" + i + "]/td["+ j +"]")).getText();
System.out.println(sColumnValue);
}
break;
}
}
driver.close();
}
}
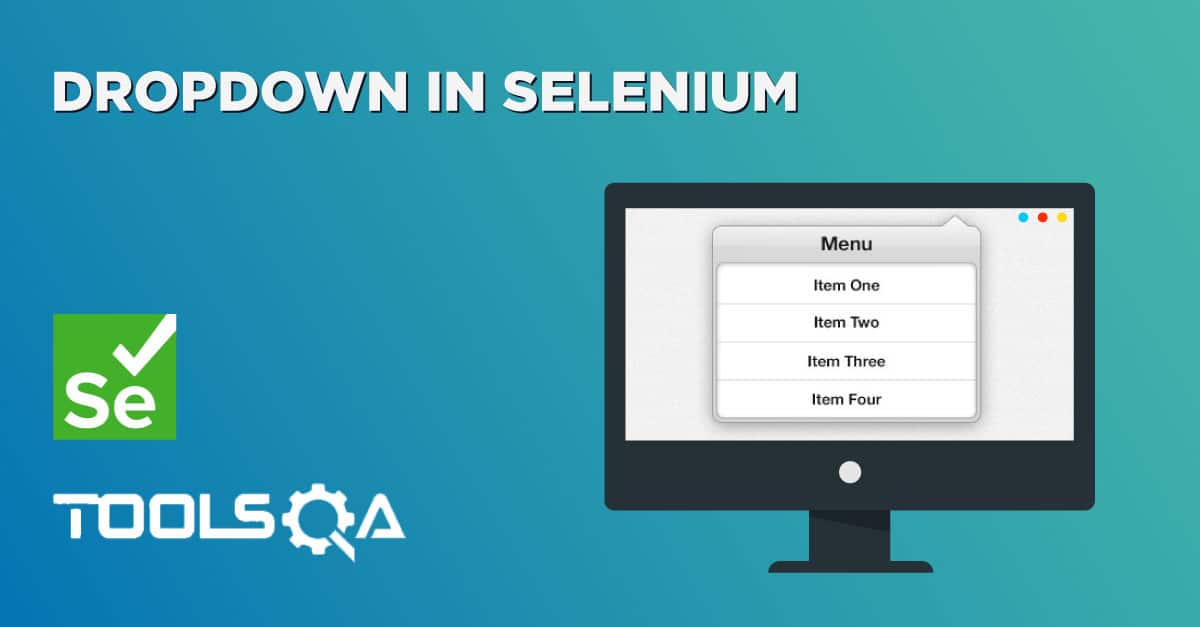
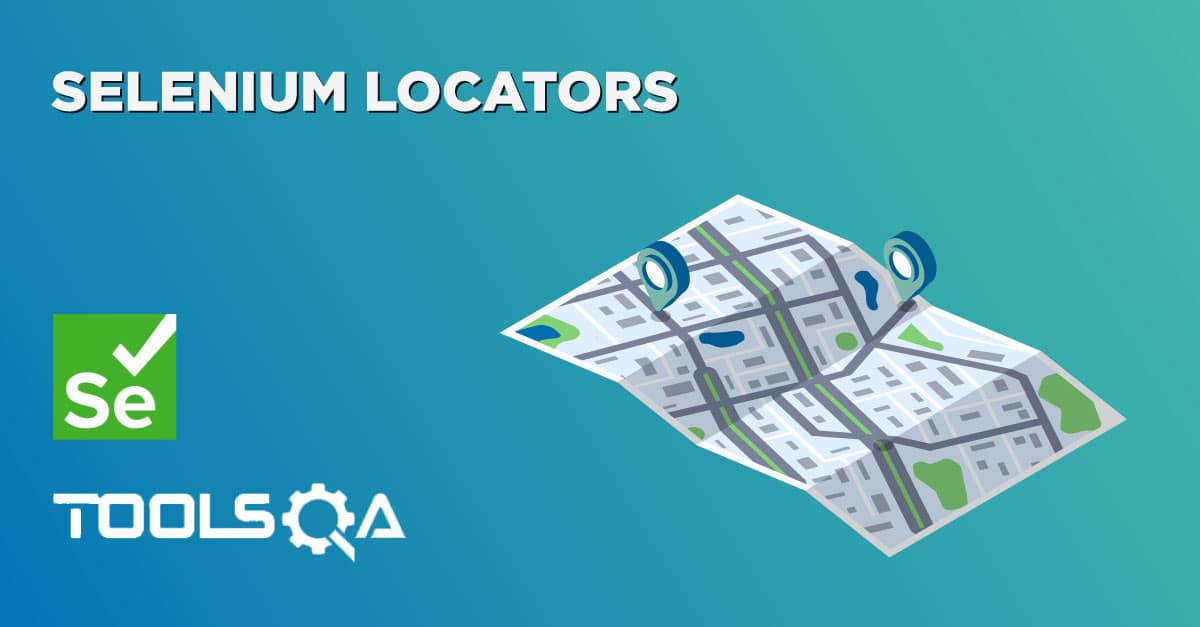