Scroll element into view
You must have faced this problem of scrolling an element into user view. Just for the purpose of taking screenshot or enabling the element visibility, you end up needing to scroll the element.
Selenium usually does it before performing any action. However that in a non-deterministic way to scroll, in the sense that you have to perform an action to scroll the element to view. This way will suffice most of the time, still, we can understand how to make use of javascript to perform scroll actions more deterministically.
Let's start with a situation and we will see different ways to solve the situation
Situation
Let's say on your page you have an element that is located somewhere near the end of the web page. You want to get this element into view. You want to do it deterministically so that you are always in control of where the element is.
Approach 1
Use element.scrollIntoView() method of element object in JavaScript. The signature of the method is
element.scrollIntoView(alignWithTop)
alignWithTop is a Boolean, if you want to your element to come in view with top of the element touching the top of the viewport of browser put this as true else a false.
here is the code listing
public static void main(String[] args) throws Exception {
// TODO Auto-generated method stub
FirefoxDriver ff = new FirefoxDriver();
ff.get("https://toolsqa.com");
Thread.sleep(5000);
ff.executeScript("document.getElementById('text-8').scrollIntoView(true);");
}
Approach 2
We scroll the page by lines till our webelement is visible. To do this we will use the JavaScript function
window.scrollByLines(NumberofLines)
NumberofLines is an integer value specifying a number of lines to scroll. A positive value signifies downward scroll and a negative value specifies upward scroll.
here is the code listing of this
public static void main(String[] args) throws Exception {
// TODO Auto-generated method stub
FirefoxDriver ff = new FirefoxDriver();
ff.get("https://toolsqa.com");
Thread.sleep(5000);
//ff.executeScript("document.getElementById('text-8').scrollIntoView(false);");
WebElement targetElement = ff.findElementById("text-8");
int x = 0;
while((Double) ff.executeScript("return document.getElementById('text-8').getBoundingClientRect().top") > 0 )
{
x = x + 2;
ff.executeScript("window.scrollByLines(2)");
System.out.println("Client top is = " + (Double) ff.executeScript("return document.getElementById('text-8').getBoundingClientRect().top"));
}
System.out.println("Element is visible after " + x + " scrolls");
}
This is a vertical scroll approach. This should be enough at 100% zooms. However, if your page is not at 100% or is bigger then the viewport of the browser you can do a similar horizontal scroll. If you see that in the second approach we have assumed that element is somewhere down in the page view. It may not be the case always, please feel free to modify the method to suit the conditions where you have to scroll upwards too. Take it as a homework and post your code in comments.
I hope this article gives you a basic understanding if scroll options available to you via javaScript. Go through more detailed JavaScript functions and you may get something really worth using.
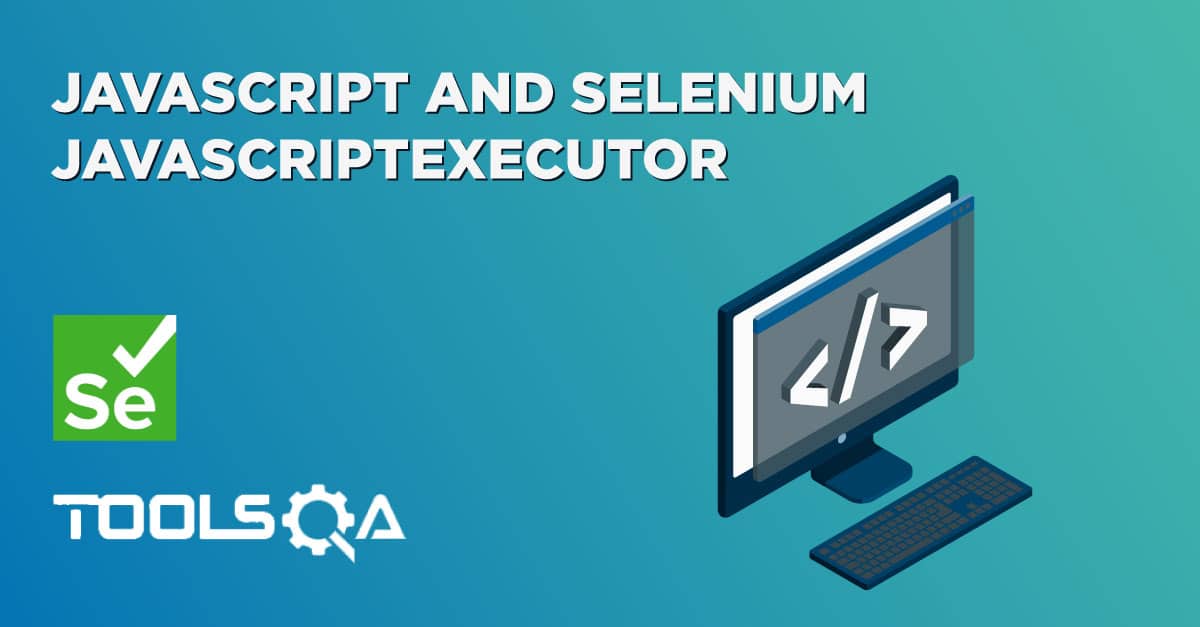
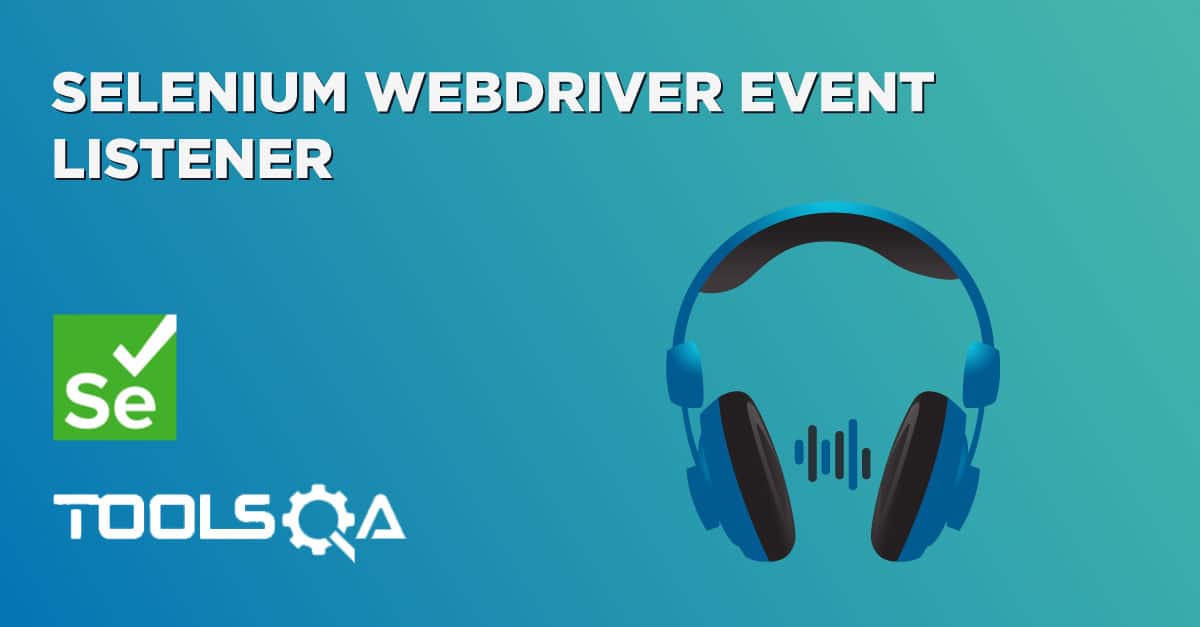