Selenium has been one of the most reliable tools for web app testing and gathers the most market share among its counterparts. Its developers have always been keen on the tester's feedback (thanks to being open source) and implementing new features with each update. One such feature comes out as Selenium Waits or also known as Selenium Wait commands. As its name already provides a subtle imprint, Selenium Wait commands let the automation execution wait for some time before continuing the normal execution. In my opinion, it does make look our execution a little "human" or similar to what end-users would face during their internet browsing.
The chapter on Selenium Wait details the different commands that implement different types of wait i.e. how can we achieve halting the process till our specified time. For example, what if I want to wait every time on every element? Or what if I just want to wait when the title contains a specific word in it? All the answers emerge slowly as we gradually move ahead into the post. Hence, to give you a brief idea of the content, the post will move in the following order:
- What is Wait in Selenium?
- Types of Selenium Waits
- Implicit Wait in Selenium
- implicitlyWait()
- pageLoadTimeout()
- setScriptTimeout()
- Explicit Wait in Selenium
- WebDriverWait
- FluentWait
- Implicit Wait in Selenium
What is Wait in Selenium?
In simple words, Selenium Wait is just a set of commands that wait for a specified period of time before executing test scripts on the elements. When to wait and how long to wait depends on the written script and type of wait used. You may be waiting for an element to load or become visible or you may want to wait till the complete page loads. Selenium Wait is therefore a preferred method when such scenarios are encountered or are expected on a web page (which is quite often due to the use of asynchronous property). It helps prevent NoSuchElementFound exception.
Technically speaking, Selenium Wait commands prevent race conditions among the elements of a web page. The core problem that gives birth to Selenium Wait is that Selenium just waits for document.readyState of the page to become "complete". In this process, due to heavy JS usage, a lot of elements add up even after the status change to "complete". This can execute the script on elements that are not yet available on the web page.
Types of Selenium Waits
Selenium wait commands has two sections:
- Implicit wait
- Explicit wait
Both of these sections have their own relevance and use cases under which they are preferred by a tester in automation testing. In the subsequent sections, we shall be discussing these two sections with various commands that they hold under their authority.
Implicit wait in Selenium
An implicit wait is a condition-less wait command in Selenium. Since it is condition-less, it is applied to all the web elements on the web page.
This means that we can tell Selenium that we would like it to wait for a certain amount of time before throwing an exception that it cannot find the element on the page or the page is not loaded or the javascript execution is not finished. Also, important to note that once set, Implicit Wait stays in place for the entire duration for which the browser is open.
We can apply implicit wait through three functions:
- implicitlyWait()
- pageLoadTimeout()
- setScriptTimeout()
Even though they are three separate callable functions, all of them are part of timeout in Selenium.
implicitlyWait command in Selenium timeout
During implicitlyWait, the WebDriver will poll the DOM for certain specified time units while trying to find any element. If the element is found earlier, the test executes at that point otherwise the WebDriver waits for the specified duration.
The polling time (or polling interval) is the time interval in which Selenium starts searching again after the last failed try. It depends on the type of browser driver you are working on. Some may have 500 milliseconds while some may have 1 second as polling time. The polling time is inbuild in implicitlyWait and there is no way to modify the time interval. But in Fluent wait, you can specify and overwrite the polling interval.
To add implicit waits in test scripts, import the following package.
import java.util.concurrent.TimeUnit;
Syntax
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
The implicitlyWait command waits for an element to load for a specified duration.
WebDriver driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
driver.get("https://somedomain/url_that_delays_loading");
WebElement myDynamicElement = driver.findElement(By.id("myDynamicElement"))
Here, the tester has specified to wait for 10 seconds (through Duration.ofSeconds(10)) before moving ahead with the interaction with the element. However, the tester can also apply other units of time such as shown by this autofill option in IntelliJ:
The default value of implicit wait is 0.
Please follow the rest of the code with our Selenium beginner series that explains the functions in detail.
pageLoadTimeout command in Selenium timeout
As the name suggests, pageLoadTimeout command waits for the page to load completely for a specified number of seconds. The default value is 0 and a negative value means infinite wait.
WebDriver driver = new FirefoxDriver();
driver.manage().timeouts().pageLoadTimeout(30, TimeUnit.SECONDS);
driver.get("https://www.google.com/");
Here, the tester has specified to wait for 30 seconds before moving ahead to interact with the element. Therefore, WebDriver will wait for a maximum of 30 seconds. The pageLoadTimeout is a good option when you are looking to test the page load performance to be within limits. For example, we can use network throttling and slow down the bandwidth to check page load time here. Using above code in the same scenario, we can know if our page can load on a 3G network or 2G network etc.
setScriptTimeout command in Selenium timeout
The setScriptTimeout command waits for the asynchronous parts of the web page to finish loading for a specified number of seconds.
driver.manage().timeouts().setScriptTimeout(15, TimeUnit.SECONDS);
((JavascriptExecutor) driver).executeScript("alert('hello world');");
((JavascriptExecutor) driver).executeAsyncScript("window.setTimeout(arguments[arguments.length - 1], 500);");
Here, the tester has specified to wait for 15 seconds before moving ahead with the interaction with the element.
The type of implicit wait command to use depends on the tester and the decision is influenced by the test script and the target element's characteristic. With this introduction to implicit wait in Selenium, it is important to note that it does come up with a couple of disadvantages and an important warning.
The implicit wait is not advised to be mixed up with explicit wait in the test scripts. A test script with both the waits can produce unpredictable behavior due to erroneous timeout durations.
In addition to the above warning, it is recommended to use implicit wait only when you are in complete control of the script and it is extremely necessary to use this type of wait. Since implicit wait is applied for the lifetime of the Webdriver, it can extend the test execution times to a large value depending on the number of elements on which it is being called. With that being said, if the tester knows their script, the implicit wait can be applied without worrying.
Explicit wait in Selenium
An explicit wait is a conditional wait strategy in Selenium in other words you wait until the condition you specified becomes true or the time duration has elapsed. Since explicit wait works with a condition, they help in synchronizing the browser, document object model, and the test execution script. Hence, the overall execution results are satisfactory and time-bound. Explicit wait provides the following conditions for usage:
- alertIsPresent()
- elementSelectionStateToBe()
- elementToBeClickable()
- elementToBeSelected()
- frameToBeAvaliableAndSwitchToIt()
- invisibilityOfTheElementLocated()
- invisibilityOfElementWithText()
- presenceOfAllElementsLocatedBy()
- presenceOfElementLocated()
- textToBePresentInElement()
- textToBePresentInElementLocated()
- textToBePresentInElementValue()
- titleIs()
- titleContains()
- visibilityOf()
- visibilityOfAllElements()
- visibilityOfAllElementsLocatedBy()
- visibilityOfElementLocated()
The above conditions come under two types of explicit wait commands:
- WebDriverWait
- FluentWait
Let's see what relevance each of them has in test execution.
WebDriverWait command in Selenium
WebDriverWait specifies the condition and time for which the WebDriver needs to wait. Practically, WebDriverWait and explicit wait go synonymously as their definitions and usage match perfectly. So if someone asks you to write some explicit wait scripts, it is safe to assume that the required scripts demand WebDriverWait.
WebElement firstResult = new WebDriverWait(driver, Duration.ofSeconds(10))
.until(ExpectedConditions.elementToBeClickable(By.xpath("//a/h3")));
Here, the wait time is specified as 10 seconds by the tester by Duration.ofSeconds(10), and the condition used is elementToBeClickable. Hence, the above code summarises as - "The WebDriver will wait till a maximum of 10 seconds for the element defined by the XPath to become clickable. All these commands will serve as a core theme when we talk about the explicit wait in the next chapter.
Fluent wait in Selenium
The fluent wait is similar to explicit wait in Selenium with one additional argument of frequency (also known as polling time). The frequency number tells the WebDriver to keep checking for the element at regular intervals and wait till the maximum of "Duration.ofSeconds". This saves execution time. If the element becomes available earlier, we can proceed with our execution and finish quickly.
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
In the above code, the maximum allowed time for waiting is specified as 30 seconds (Duration.ofSeconds(30)) and the polling time is 5 seconds (pollingEvery(Duration.ofSeconds(5))). With this arrangement, the WebDriver will keep checking for the element every 5 seconds to a maximum elapse of 30 seconds.
In the last line of the code, notice another function "ignoring". This is a custom configuration written by the tester and is not required each time. The significance of "ignoring" is to ignore certain exceptions that can hinder the test execution process. In this code, NoSuchElementException is the ignored exception.
FluentWait in Selenium is an important class when we are dealing with AJAX elements. When a user is exploring the website on a slower network, these elements may take more time to load than what they would have taken in lab testing. To play safe, FluentWait helps us mimic such scenarios and produce the best quality web application.
Key Takeaways
Following are the key takeaways from our post on Selenium Wait:
- Selenium wait is a concept that tells Selenium to wait for some specified time or until the element is visible/has loaded/enabled.
- Selenium wait disects into implicit and explicit waiting.
- Implicit wait specifies a time to wait for the lifetime of WebDriver and is applicable for each element i.e. done once. Explicit waits, however, depend on the conditions.
- The explicit wait depends on the specified condition or the maximum duration of allowed time for waiting.
FAQs
Is explicit wait better than implicit wait?
The core nature of explicit wait saves time and is not applicable to all the elements. This gives an impression that explicit waits are better and preferred for test creation. However, there are no such proofs and each type of wait has its own relevance.
When to use implicit wait and when to use explicit wait?
The implicit wait is applied once for all the elements and the next wait cycle starts once the previous one completes. If such a situation matches your requirement, implicit wait commands are best in such cases. For example, if you want to perform a task only after the complete page loads, you can go ahead with pageLoadTimeout. But this wait should be applied anyways.
The explicit wait is conditional in nature. If you want to wait for only a few select elements based on conditions, the explicit wait is the way to go. However, implicit waits used without a solid understanding of scripts can lead to an unnecessarily long time for the completion of scripts.
What is polling in implicit wait?
The implicit wait is done until the element is found or the maximum time has elapsed. But for fallbacks, implicit wait in Selenium comes with a feature called polling. With polling, implicit wait keeps searching for the element at regular intervals till the time it finds the element or the maximum time has elapsed.
Which is the best type of Selenium wait?
There is no "best" type of Selenium wait when it comes to testing a web application. Different use cases demand different types of wait conditions and therefore ask for appropriate commands.
Exercises
You can proceed with the following exercises on Selenium Wait to make your basics stronger.
- Launch a new browser (such as ChromeDriver).
- Open URL "https://demoqa.com/dynamic-properties".
- Maximize the window.
- Find an element with id "visibleAfter". This element takes 5 secs to load which means this element will appear after 5 secs.
- Use implicitlyWait to wait for that element.
package org.example;
import java.time.Duration;
import java.util.concurrent.TimeUnit;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ImplicitWait {
protected WebDriver driver;
@Test
public void implicitWaitExcercise()
{
driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
// launch Chrome and redirect it to the URL
driver.get("https://demoqa.com/dynamic-properties");
//This element will appear after 5 secs
driver.findElement(By.id("visibleAfter")).click();
//close browser
driver.close();
}
}
You would notice that even though the element takes 5 secs to appear, still the selenium script would pass. Because of implicitly wait, as it automatically waits for the element to appear.
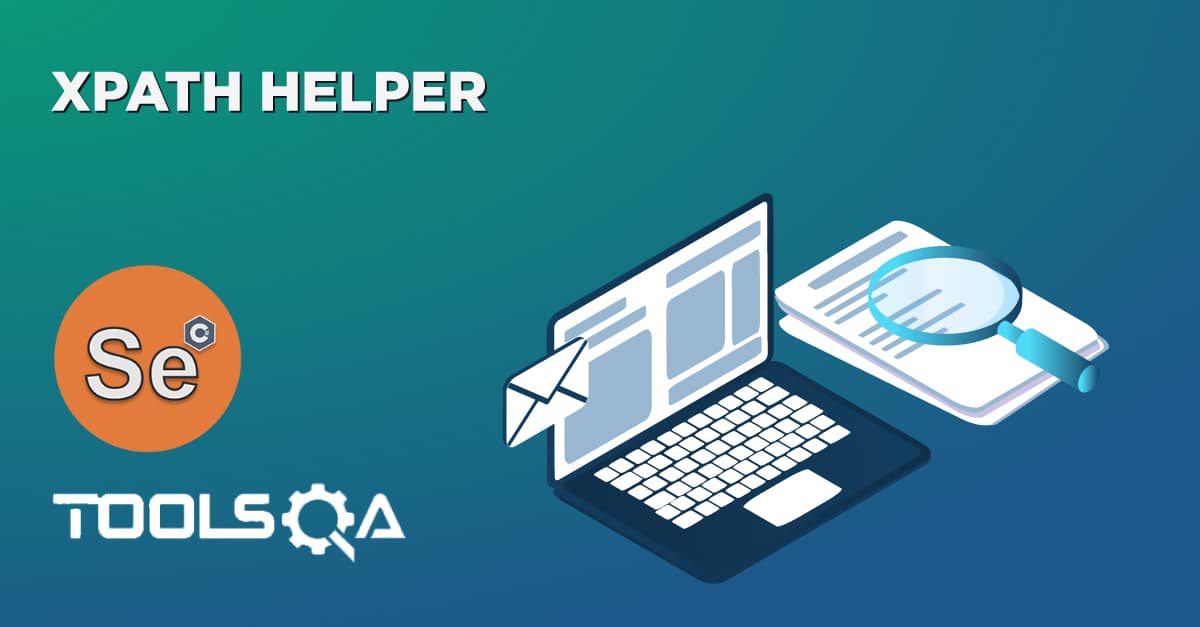
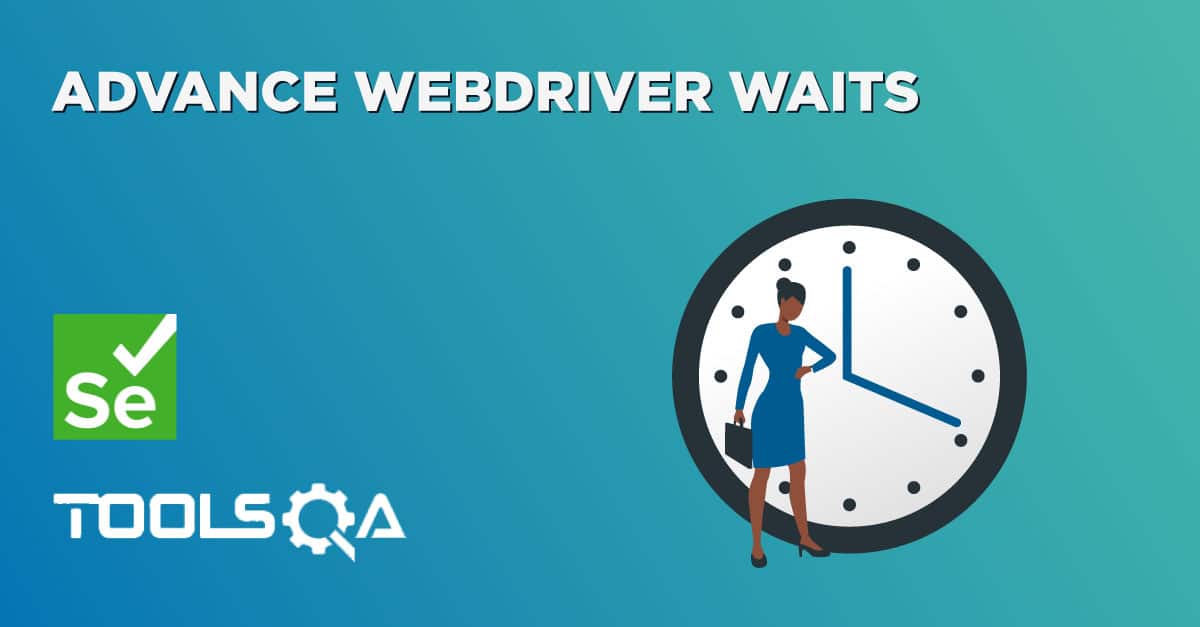