Welcome to this tutorial on Log4j Appenders. This is in continuation to the tutorials on Log4j, I hope you have read through our previous tutorials on Introduction, LogManager and Loggers.
Appenders
Appenders are the Log4j objects which deliver logs to the required destinations. For example, a ConsoleAppender will deliver the logs to the console and a FileAppender to the log file. There are many types of Appenders that we have in Log4j, the ones that we will cover today are
- FileAppender
- ConsoleAppender
- JDBCAppender
File Appenders
Almost all the time we want to log our data in a file instead of printing it on the console. This is for obvious reasons, we want a copy of the logs so that we can keep it for reference and browse through it to find problems. Whenever you need to log in a file you will use a FileAppender. This code sample explains you how to create a FileAppender object and then set it to the required logger.
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.FileAppender;
import org.apache.log4j.Layout;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import org.apache.log4j.SimpleLayout;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
Logger OurLogger = LogManager.getLogger("OurLogger");
//create a FileAppender object and
//associate the file name to which you want the logs
//to be directed to.
//You will also have to set a layout also, here
//We have chosen a SimpleLayout
FileAppender fileAppender = new FileAppender();
fileAppender.setFile("logfile.txt");
fileAppender.setLayout(new SimpleLayout());
//Add the appender to our Logger Object.
//from now onwards all the logs will be directed
//to file mentioned by FileAppender
OurLogger.addAppender(fileAppender);
fileAppender.activateOptions();
//lets print some log 10 times
int x = 0;
while(x < 11){
OurLogger.debug("This is just a log that I want to print " + x);
x++;
}
}
}
While creating an appender you have to add the LayOut that you would like to choose. In our case, we have chosen SimpleLayout(). Also, whenever you make a change in Appender object, for example, adding a file path or adding the Layout you have to call .activateOptions(), activateOptions() will activate the options set previously. This is important because your chances to Appender object won't take place until .activateOptions(). You will find the log file in the project folder in your eclipse workspace. Also, this is how the logs look in the logfile:
DEBUG - This is just a log that I want to print 0
DEBUG - This is just a log that I want to print 1
DEBUG - This is just a log that I want to print 2
DEBUG - This is just a log that I want to print 3
DEBUG - This is just a log that I want to print 4
DEBUG - This is just a log that I want to print 5
DEBUG - This is just a log that I want to print 6
DEBUG - This is just a log that I want to print 7
DEBUG - This is just a log that I want to print 8
DEBUG - This is just a log that I want to print 9
DEBUG - This is just a log that I want to print 10
Console Appenders
For testing purposes, you may want to redirect your output logs to the console. Actually ConsoleAppender directs the logs to System.err and System.out streams. These streams are also read by Console and hence the output is displayed at the console as well. Let's see with a code sample on how to use ConsoleAppender Object
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.ConsoleAppender;
import org.apache.log4j.FileAppender;
import org.apache.log4j.Layout;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import org.apache.log4j.SimpleLayout;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
Logger OurLogger = LogManager.getLogger("OurLogger");
//create a ConsoleAppender object
//You will also have to set a layout also, here
//We have chosen a SimpleLayout
ConsoleAppender ConsoleAppender = new ConsoleAppender();
ConsoleAppender.setLayout(new SimpleLayout());
//Add the appender to our Logger Object.
//from now onwards all the logs will be directed
//to file mentioned by FileAppender
OurLogger.addAppender(ConsoleAppender);
ConsoleAppender.activateOptions();
//lets print some log 10 times
int x = 0;
while(x < 11){
OurLogger.debug("This is just a log that I want to print " + x);
x++;
}
}
}
This way you will redirect all the logs to the console and can see the output in the console.
JDBC Appender
JDBCAppenders are used to write logs to a Database. These appenders accept database connection credentials to connect to DB. Let's see a code sample to understand how JDBCAppenders work
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.ConsoleAppender;
import org.apache.log4j.FileAppender;
import org.apache.log4j.Layout;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import org.apache.log4j.SimpleLayout;
import org.apache.log4j.jdbc.JDBCAppender;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
Logger OurLogger = LogManager.getLogger("OurLogger");
//create a JDBCAppender class instance
JDBCAppender dataBaseAppender = new JDBCAppender();
//Provide connection details to the
//Database appender
dataBaseAppender.setURL("jdbc:mysql://localhost/test"); //Connection url
dataBaseAppender.setUser("User1"); //Username for the DB connection
dataBaseAppender.setPassword("ThisPassword"); //Password for the DB connection
dataBaseAppender.setDriver("com.mysql.jdbc.Driver"); // Drivers to use to connect to DB
//set the sql insert statement that you want to use
dataBaseAppender.setSql("INSERT INTO LOGS VALUES ('%x', now() ,'%C','%p','%m'");
//activate the new options
dataBaseAppender.activateOptions();
//Attach the appender to the Logger
OurLogger.addAppender(dataBaseAppender);
int x = 0;
while(x < 11){
OurLogger.debug("This is just a log that I want to print " + x);
x++;
}
}
}
This code explains how to set up a JDBCAppender object and use it for logging. After seeing the code you will notice that we have to give the insert statement to the JDBCAppender. This statement is used to insert logs in the desired database table. We have used the statement INSERT INTO LOGS VALUES ('%x', now() ,'%C','%p','%m') It says that logs are inserted in the table named LOGS. Don't worry about %x %C and other similar terms in the Insert Statement. We will cover them in our next topic called Layouts Appenders is a very big topic, we have just covered 3 most commonly used Appender types. We also have lots of other appenders that can be used. I encourage you to read more about appenders from the official Log4j documentation. I hope this tutorial was helpful for you. If you have any comments do drop me an email. Stay tuned for our next tutorial called Layouts. Thanks
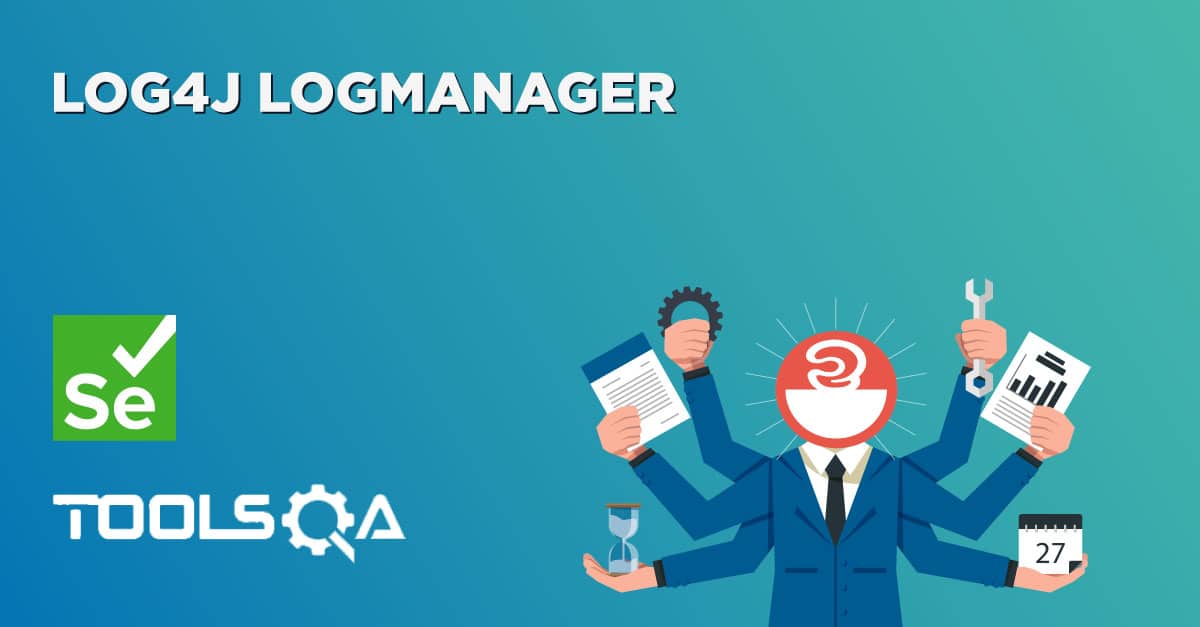
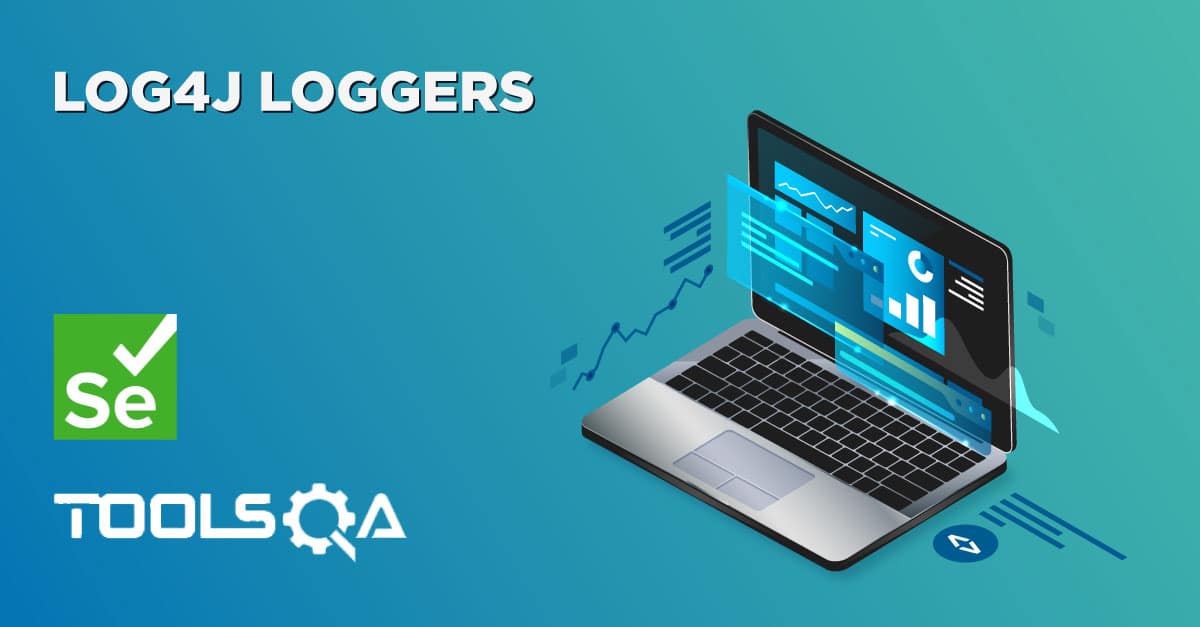