Welcome to the next chapter in the series of tutorials on Log4j Loggers. I hope you have read at least Log4j Introduction and LogManager,
Lets get started. The next object in the Log4j component list is the Logger class. This is the most important class that you will need. This is the object which lets you log information to the required Log location, be it console or a file or even a database.
Logger objects follow hierarchy similar to class hierarchy in any OOP language. The naming convention of Logger hierarchy is in the name. Each object name decide which hierarchy it follows. For example, we have a logger named "Main.Utility". So Utility is the child of Main and Main is the father of Utility. Also, all Loggers are derived from root Logger. The actual hierarchy will be root.Main.Utility with root being an ancestor of Utility and Father of Main. This can be shown in a diagram as
These relationships are managed by the LogManager class. Let's illustrate it using an example
package Log4jSample;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
Logger chance = LogManager.getLogger(SampleEntry.class.getName());
Logger logger1 = LogManager.getLogger("Child1");
Logger logger1Child = logger1.getLogger("Child1.ChildOfLogger1");
Logger loggerGrandChild = LogManager.getLogger("Child1.ChildOfLogger1.GrandChild");
System.out.println("logger1's full name is " + logger1.getParent().getName());
System.out.println("logger1Child's full name is " + logger1Child.getParent().getName());
System.out.println("loggerGrandChild's full name is " + loggerGrandChild.getParent().getName());
}
}
Output will be
As you can see that logger1 is the parent of logger1Child and grandparent of loggerGrandChild's. This is how we can create hierarchy of logger objects based on the application need.
Logging levels
Logger class have following print methods that help you log information.
- Trace
- Debug
- Info
- Warn
- Error
- Fatal
So let's say you want to print a Debug log you would just do it by saying, Logger.Debug("This is a debug log"). You may choose to use any other overloaded Logger.Debug() method. All these print statements are called Levels.
Question comes, why do we need log levels?
Each log level expects a certain type of information for e.g Debug level expects logging of that information which may help a programmer debug the application in case of failures. Similarly Error Level expects all the Errors to be logged using this level.
You can set log level of a logger using the Logger.setLevel method. Once you set the Log level of your logger only loggers with that and higher level will be logged. Log levels have following order TRACE < DEBUG < INFO < WARN < ERROR < FATAL.
Let's understand this with an example, in the code below we have set the level to DEBUG first and than WARN. You will see that only the logs which are at that or higher level will be logged. Here is the code sample.
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
Logger Mylogger = LogManager.getLogger("DebugLogger");
//Setting up the log level of both loggers
Mylogger.setLevel(Level.DEBUG);
Mylogger.trace("This is the trace log - DEBUG");
Mylogger.debug("This is debug log - DEBUG");
Mylogger.info("This is info log - DEBUG");
Mylogger.warn("This is Warn log - DEBUG");
Mylogger.error("This is error log - DEBUG");
Mylogger.fatal("This is Fatal log - DEBUG");
Mylogger.setLevel(Level.WARN);
Mylogger.trace("This is the trace log - WARN");
Mylogger.debug("This is debug log - WARN");
Mylogger.info("This is info log - WARN");
Mylogger.warn("This is Warn log - WARN");
Mylogger.error("This is error log - WARN");
Mylogger.fatal("This is Fatal log - WARN");
}
}
Output looks like this
You can see that when Log level was DEBUG all the logs DEBUG to FATAL are displayed. Once the log level is set to WARN all the logs from WARNS to FATAL are displayed.
Log level Inheritance
As discussed in the previous chapters we know that Loggers follow hierarchy. Similarly, Log levels also follow a hierarchy, what I mean is that if a Level Hierarchy of a logger is not defined then it is picked from the Level of parent. Let's say we have two loggers
LoggerParent and LoggerParent.Child and let's say Logger LoggerParent has log level set to LoggerParent.setLevel(Level.WARN) to warn. Now if we don't set the Level of Logger child than the default logging Level of Child will be set to Level.WARN which is the Level of its parent.
Let's see this with a code sample
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
Logger LoggerParent = LogManager.getLogger("LoggerParent");
Logger LoggerChild = LogManager.getLogger("LoggerParent.Child");
//Setting up the log level of both loggers
LoggerParent.setLevel(Level.WARN);
LoggerParent.trace("This is the trace log - PARENT");
LoggerParent.debug("This is debug log - PARENT");
LoggerParent.info("This is info log - PARENT");
LoggerParent.warn("This is Warn log - PARENT");
LoggerParent.error("This is error log - PARENT");
LoggerParent.fatal("This is Fatal log - PARENT");
LoggerChild.trace("This is the trace log - CHILD");
LoggerChild.debug("This is debug log - CHILD");
LoggerChild.info("This is info log - CHILD");
LoggerChild.warn("This is Warn log - CHILD");
LoggerChild.error("This is error log - CHILD");
LoggerChild.fatal("This is Fatal log - CHILD");
}
}
Output of this sample is
Logging run time Exceptions
This is a very important feature of a Logger class, it enables you to pass on the exception to the output. This comes handy specifically in the cases where we have intentionally caught the exception but we also want to log the information about the exception. Every print method (TRACE, DEBUG.... FATAL) has an overload which is Logger.Debug(Object message, Throwable t), off course we have just taken the example of .Debug only, this allows us to pass the exception. Let's see how it's beneficial using a code sample
package Log4jSample;
import org.apache.log4j.BasicConfigurator;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
public class SampleEntry {
public static void main(String[] args) {
// TODO Auto-generated method stub
BasicConfigurator.configure();
Logger LoggerParent = LogManager.getLogger("LoggerParent");
try
{
// We will get a divide by zero exception her
int x = 200 / 0;
}
catch(Exception exp)
{
LoggerParent.warn("Following exception was raised", exp);
}
}
}
As you can see that in the output you will see exception details being logged. This information is very handy while debugging. Important point to observe is that Exception message and the Line number of exception is printed automatically. Output of above code is
I hope this tutorial gives you an idea about Logger class. If you have any comment do drop me an email. Hope to see you in the next tutorial.
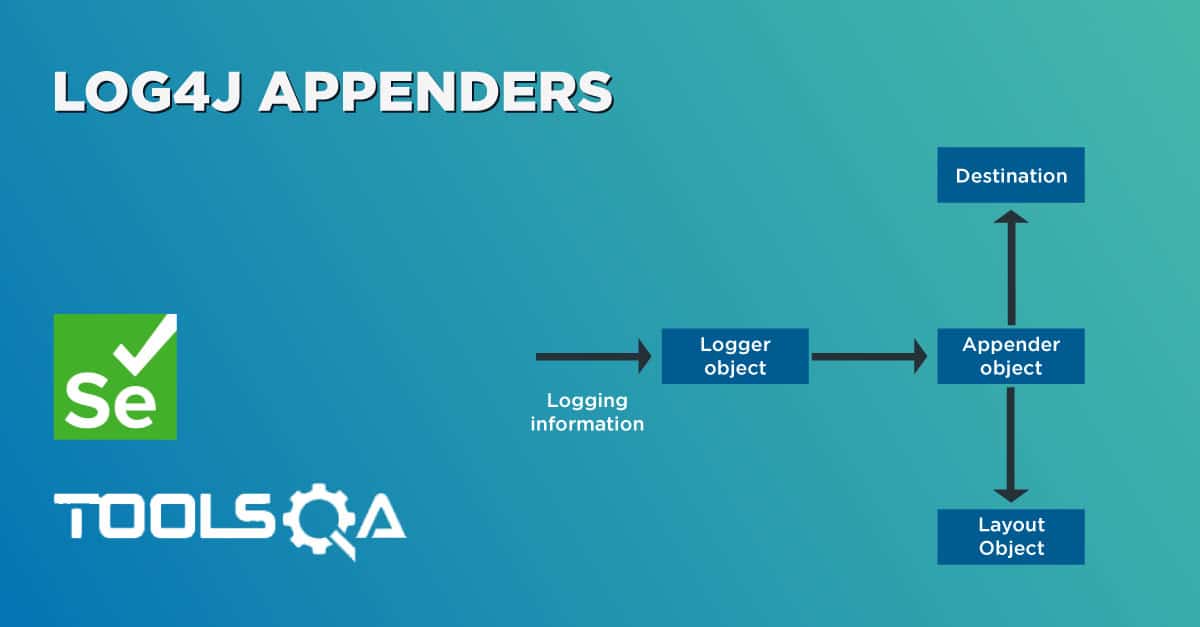
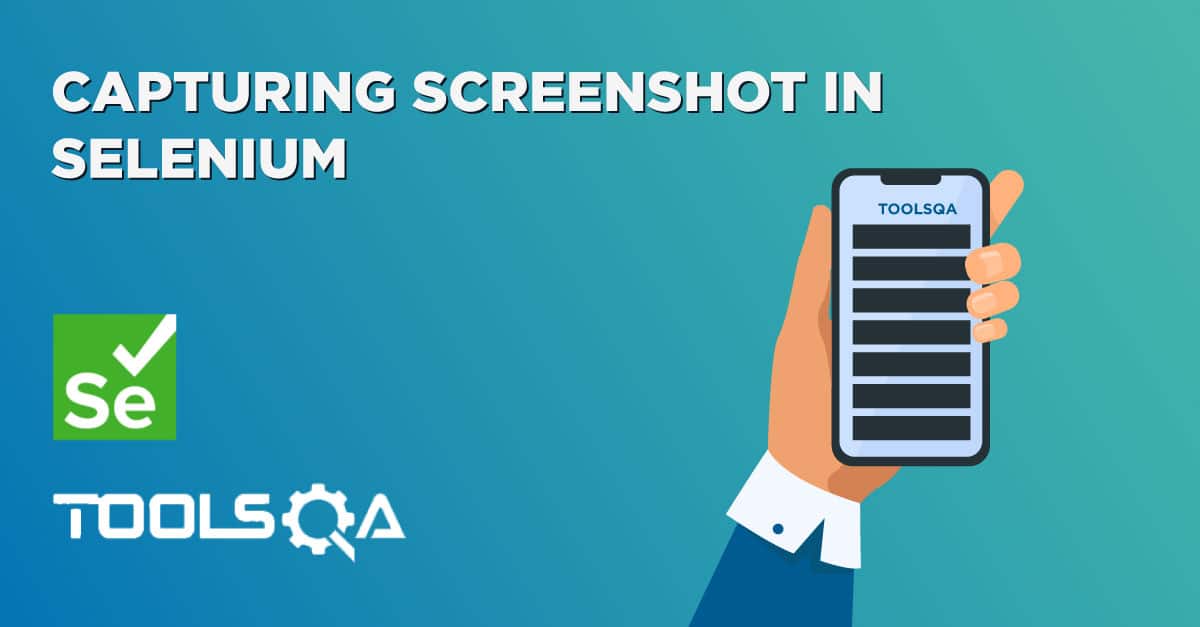