This tutorial is part of the series of Action Class in Selenium. This covers the concept of capturing ToolTip using WebDriver API in Selenium Automation. Let us start with understanding What is Tooltip, Why it is available on websites and the purpose to test it.
What is Tooltip?
In many web pages, it's very common that when hovered over some link, text, some text or sometimes image gets displayed. For example, in Gmail inbox, if hovered over right-hand side menu options, small "hover box" with text gets displayed. This is called a tooltip of web element.
This text usually is a brief description of the functionality of the object or in some cases, it displays a detailed description of the object. In some cases, it may display only the full name of the object. So basically, the purpose of a tooltip is to provide some hint to the user about the object. In many instances, it is required to verify if this text description being displayed as expected.
For the above reasons, it is required to retrieve the text inside the tooltip and verify the text. This can be done in different ways depending upon how tooltip is getting inserted in the HTML. Let's consider the following cases:
- Case One: When the tooltip is available in the 'title' attribute. Here, we can retrieve tooltip from By strategy
- Case Two: When the tooltip is available in 'div'. Here, we can retrieve tooltip using Actions class methods
We will be covering both cases. Let's consider, Case One: When the tooltip is available in the title attribute.
How to capture tooltip in Selenium using getAttribute?
Let’s consider an example from an already available Demo-Page on Toolsqa as http://demoqa.com/tool-tip. This is quite a simple way of tooltip display.
ToolTip gets displayed when hovered over an HTML object. This can also be seen from the Developer tool of the browser, tooltip text is seen as a value set in 'title' attribute.
In most cases, it is pretty straight forward to get the tooltip text. All you need to do it to locate the web-element for which tooltip needs to be retrieved.
WebElement webElement = driver.findElement(Any By strategy & locator);
Here, any By strategy to locate the WebElement like find element by its id, name attribute, etc can be used. To know more on all By strategies, please refer Find Elements tutorial.
Now, retrieve ‘title’ attribute value of the webElement.
String tooltipText = webElement .getAttribute("title");
With the above statement, text from the tooltip will be saved in the variable called tooltipText.
Practice Exercise to retrieve ToolTip in Selenium
Find below the steps of the scenario to be automated:
- Open URL http://demoqa.com/tooltip/
- Identify web element Age textbox
- Retrieve 'title' attribute value of the web element
- Verify the text of 'title' attribute value matches with expected text
- Close the page
Selenium Code Snippet:
package com.toolsqa.tutorials.actions;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.interactions.Actions;
public class TooltipDemo {
public static WebDriver driver;
public static void main(String[] args) {
//Set system properties for geckodriver This is required since Selenium 3.0
System.setProperty("webdriver.gecko.driver", "C:\\Selenium\\Toolsqa\\lib\\geckodriver.exe");
//Create a new instance of the Firefox driver driver = new FirefoxDriver();
//CASE 1: Using getAttribute
// Launch the URL driver.get("https://demoqa.com/tool-tips/");
System.out.println("Tooltip web Page Displayed");
//Maximise browser window
driver.manage().window().maximize();
// Get element for which we need to find tooltip
WebElement ageTextBox = driver.findElement(By.id("age"));
//Get title attribute value
String tooltipText = ageTextBox.getAttribute("title");
System.out.println("Retrieved tooltip text as :"+tooltipText);
//Verification if tooltip text is matching expected value
if(tooltipText.equalsIgnoreCase("We ask for your age only for statistical purposes.")){
System.out.println("Pass : Tooltip matching expected value");
}
else{
System.out.println("Fail : Tooltip NOT matching expected value");
}
// Close the main window
driver.close();
}
}
How to capture tooltip in Selenium using Actions Class?
There are different ways to display ToolTip in the HTML page. As mentioned above, it can be placed inside the title tag. The same way it can be extracted out in different fashions. Above we just located the title element and read its property by using the GetAttribute method of Selenium. The same way can also be displayed with the help of the Div element.
But this time we try to mimic the same behavior as any other user does on the website. Hover over the object and try to read the description of the tooltip. And this can only be done with the help of Actions Class in Selenium. Let's see how to use Actions class methods to get it:
First, instantiate an Actions class to make use of its object.
Actions actions = new Actions(driver);
Locate the tooltip web-element.
WebElement element = driver.findElement(Any By strategy & locator);
Now, invoke moveToElement(), this method of Actions class moves the mouse to the middle of the element.
actions.moveToElement(element).perform();
As explained in the tutorial of Actions Class, perform() method actually performs the action and internally invokes build() method. So, here when this line of code will execute, you can actually see the tooltip of the element on the browser.
Now get that tooltip element and invoke getText() to get it's description.
WebElement toolTip = driver.findElement(Any By strategy & locator);
String toolTipText = toolTip.getText();
Practice Exercise to retrieve ToolTip in Selenium with Actions Class.
Find below the steps of the scenario to be automated:
- Open URL http://demoqa.com/tool-tips
- Identify 'div' web element
- Generate action moveToElement and perform the action
- Identify webelement displaying tooltip
- Retrieve text attribute value
- Verify tooltip text value matches with expected text
- Close the page
Selenium Code Snippet:
package com.toolsqa.tutorials.actions;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.interactions.Actions;
public class TooltipDemo {
public static WebDriver driver;
public static void main(String[] args) {
//Set system properties for geckodriver This is required since Selenium 3.0
System.setProperty("webdriver.gecko.driver", "C:\\Selenium\\Toolsqa\\lib\\geckodriver.exe");
// Create a new instance of the Firefox driver
driver = new FirefoxDriver();
//CASE 2 : Using Actions class method
driver.get("https://demoqa.com/tool-tips");
System.out.println("demoqa webpage Displayed");
//Maximise browser window
driver.manage().window().maximize();
//Instantiate Action Class
Actions actions = new Actions(driver);
//Retrieve WebElement
WebElement element = driver.findElement(By.id("tooltipDemo"));
// Use action class to mouse hover
actions.moveToElement(element).perform();
WebElement toolTip = driver.findElement(By.cssSelector(".tooltiptext"));
// To get the tool tip text and assert
String toolTipText = toolTip.getText();
System.out.println("toolTipText-->"+toolTipText);
//Verification if tooltip text is matching expected value
if(toolTipText.equalsIgnoreCase("We ask for your age only for statistical purposes.")){
System.out.println("Pass* : Tooltip matching expected value");
}else{
System.out.println("Fail : Tooltip NOT matching expected value");
}
// Close the main window
driver.close();
}
}
In the next tutorial, we will take a look at the scenario of How to perform Drag and Drop in Selenium.
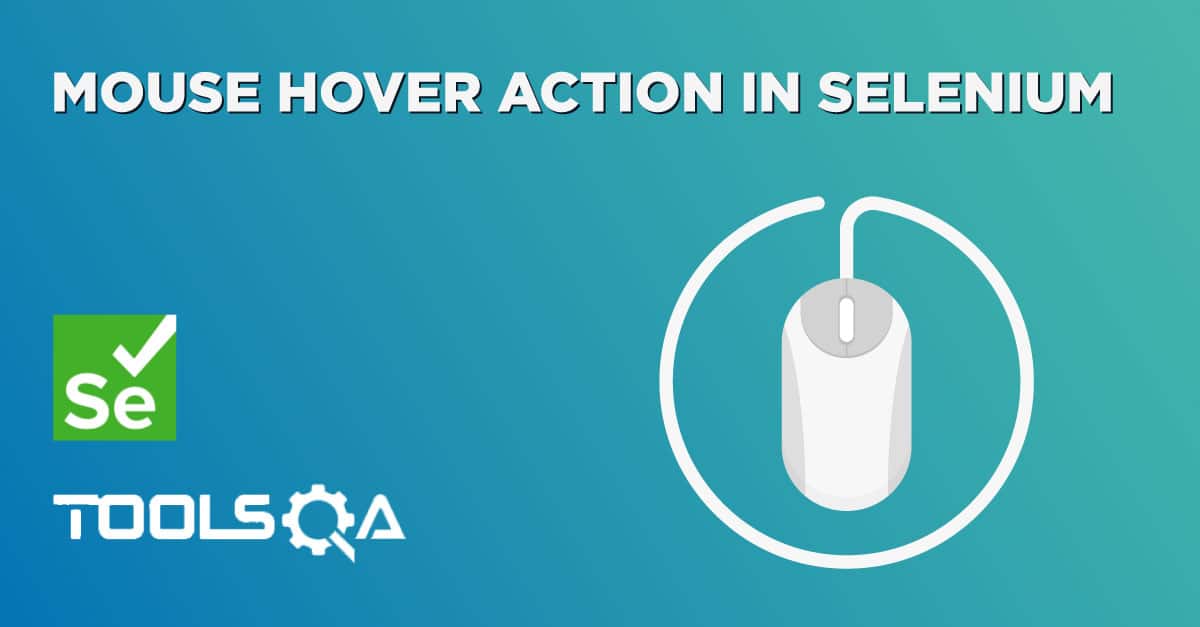
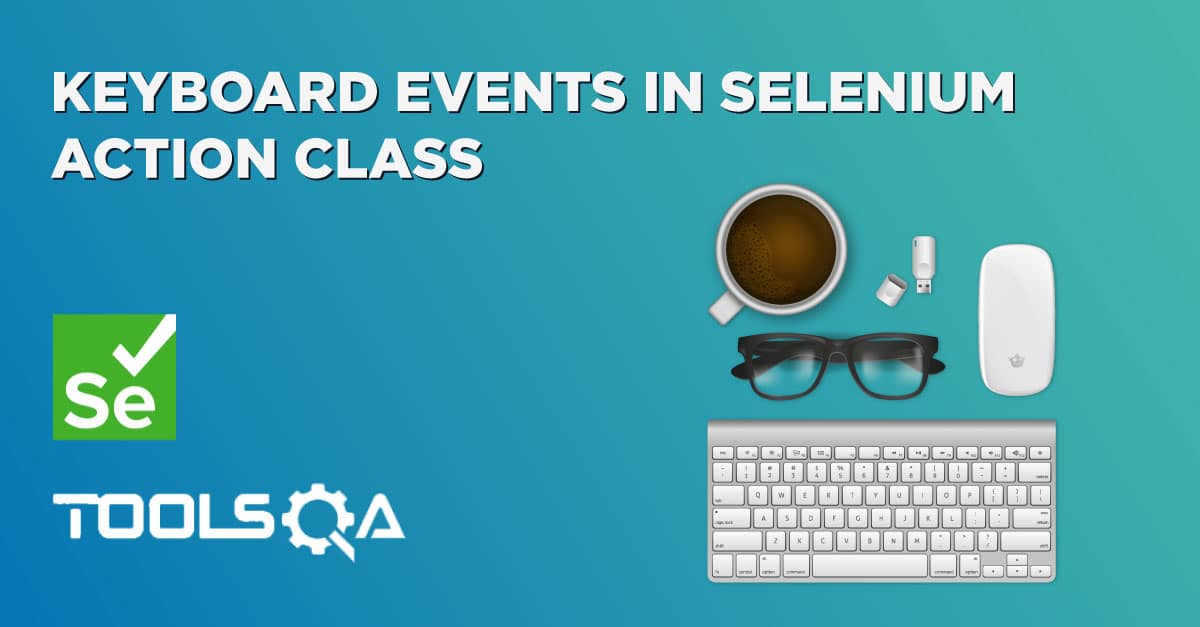