Selenium undoubtedly eases repetitive testing tasks by mocking up human interactions on the web browser, but there are specific differences while executing tests through automation. You might have come across a case when manually opening a website works fine, but the same action through Selenium WebDriver throws an error that says- "This Connection is Untrusted". Have you ever wondered why this happens? The answer is simple, on manually opening a URL, the browser automatically imports the required certificates, and no error occurs. Simultaneously, in Selenium WebDriver, each run occurs on a new profile that doesn't have the SSL Certificates, hence the error. This article will understand everything about the SSL Certificates and look at how we can handle the SSL certificate in Selenium and take appropriate actions on SSL certificate errors across various browsers. We will be covering the below details:
- What is an SSL certificate?
- What is an untrusted SSL certificate?
- How does the SSL certificate work?
- What are the different types of SSL certificates?
- What are the different types of SSL certificate errors?
- How to handle SSL certificate error using Selenium WebDriver?
- How does Selenium Webdriver Handle SSL certificate in Chrome?
- How does Selenium Webdriver Handle SSL certificate in Firefox?
- How does Selenium Webdriver handle the SSL certificate in Edge?
- How does Selenium Webdriver handle the SSL certificate in Safari?
What is an SSL certificate?
SSL(Secure Sockets Layer) is a standard security protocol that establishes a secure connection between the server and the client(browser). The information sent using an SSL certificate is encrypted and ensures that it delivers to the right server. It is a validator to the website's identity and helps to keep hackers at bay.
What is an untrusted SSL certificate?
Whenever you try to access a website, the SSL certificate helps determine if the website is what it claims to be. If there is any issue with the certificate, you will see an error on your browser window saying: "This connection is untrusted". or "Your connection is not private", as shown below:
As you can see, a sample error that might come up in case of an untrusted SSL. In the following sections, we will see what different types of SSL certificate errors are there and how we can handle them in Selenium automation. Before jumping onto that, let us now see how does an SSL certificate work?
How does the SSL certificate work?
As already said, the SSL certificate helps create a secure connection. The image below shows the working on SSL handshake between a client and a server:
We can sum up the internal working in the steps below:
- The browser sends the HTTPS request to the server.
- To prove that the server is trusted, the server sends the SSL certificate to the browser.
- Now each browser has its own Trusted Certificate Authority(CA) list. The browser verifies the certificate against the trusted CAs to check if the certificate is present and is unexpired, unrevoked, and its common name is valid for the website requested.
- If all the checks in the step above are correct, the browser trusts the certificate. Additionally, it also leads to creating an encrypted session that happens between the server and the browser.
- The server and the browser can now send messages to each other in an encrypted format.
What are the different types of SSL certificates?
If you have ever seen the zip file received from Certificate Authority for your website SSL certificate, you would have seen that the zip file contains not one but different SSL files. The Certificate Signing Authority provides three types of SSL certificates:
- Root Certificate
- Intermediate Certificate
- Server Certificate
The Root Certificate is a digital certificate belonging to the Certificate Authority. It is pre-downloaded in most of the browsers, and the CAs closely guard it.
The Intermediate Certificate, on the other hand, is like a link between the Root and the Server certificate. It links the client to the CA and needs installation on your server.
The Server Certificate is the primary certificate that you get for installation on your server.
Since our agenda is handling of untrusted SSL certificates using Selenium, we will quickly jump on to our next topic, and you can read more about SSL Certificateshttps://en.wikipedia.org/wiki/Transport_Layer_Security.
What are the different types of SSL certificate errors?
There might be chances when you perform an HTTPS request and get a message like "This site is not secure" or "Your connection is not private". This SSL certificate error might show different error messages in other browsers, as seen below:
- Chrome - Your connection is not private.
2. Firefox - Warning: Potential Security Risk Ahead.
3. IE - This site is not secure.
4. Safari - Safari can't verify the identity of the website.
Although the error messages that appear on different browsers may vary, there is one thing common in all these errors, i.e., the reason for the error. Every untrusted SSL has a reason for it. A few of the standard error reasons are listed below:
- Expired Certificate Error - Occurs when the website certificate has expired and shows an error code like ERR_CERT_DATE_INVALID.
- Revoked Certificate Error - Occurs when a website's certificate is revoked and shows an error code like - ERR_CERT_REVOKED
- Self-Signed Certificate Error - Occurs when either the certificate is self-signed or is signed by an untrusted source and shows an error code ERR_CERT_AUTHORITY_INVALID.
The errors mentioned above are common, but you might face some other SSL errors for the website you access. Let's now understand how we can handle the errors of untrusted errors in various widely used browsers?
How to handle SSL certificate error using Selenium WebDriver?
Now that we understand why we get the SSL error, we will now see how we can handle this error in Selenium Automation. Since we can get this error in any of the browsers, we will see how we can handle this in Firefox, Chrome, IE, and Safari browsers. But before jumping onto the handing part, let us first see what happens if we access a website that has SSL certificate issues.
We will use a Demo website(https://badssl.com/) with different types of certificate errors, which you can refer to practicing as well. Below is a simple code when we navigate to the URL in the Chrome browser, it raises the Certificate error:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SSLHandling {
public static void main(String[] args) {
//Creating instance of Chrome driver (Assuming Chromedriver is installed at system level)
WebDriver driver = new ChromeDriver();
//Launching the URL
driver.get("https://expired.badssl.com/");
System.out.println("The page title is : " +driver.getTitle());
driver.quit();
}
}
On executing the above code, you will see that the web page launches. Still, the screen displays Connection Error, one of the SSL certificate errors for an expired/invalid certificate.
The execution logs are capturing the page title as shown in the image below-
We will now see how we can handle such error(s) in different web browsers and see the actual web page after handling the SSL certificate in Selenium code.
How does Selenium Webdriver handle SSL certificate in Chrome?
For the Chrome browser, one can handle the SSL certificates using ChromeOptions class provided by Selenium WebDriver. It is a class that we can use to set properties for the Chrome browser. Let us now see what additions we will make to the existing code, and then we will understand the same.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
public class SSLHandling {
public static void main(String[] args) {
//Create instance of ChromeOptions Class
ChromeOptions handlingSSL = new ChromeOptions();
//Using the accept insecure cert method with true as parameter to accept the untrusted certificate
handlingSSL.setAcceptInsecureCerts(true);
//Creating instance of Chrome driver by passing reference of ChromeOptions object
WebDriver driver = new ChromeDriver(handlingSSL);
//Launching the URL
driver.get("https://expired.badssl.com/");
System.out.println("The page title is : " +driver.getTitle());
driver.quit();
}
}
We can summarize the above code as below-
- ChromeOptions handlingSSL = new ChromeOptions(); -- We create an object of ChromeOptions class.
- handlingSSL.setAcceptInsecureCerts(true); -- Now we will use a capability setAcceptInsecureCerts. We pass the parameter as true, which means the invalid certificate will be trusted implicitly by the browser.
- WebDriver driver = new ChromeDriver(handlingSSL); -- Next, we create an instance of chrome driver and pass the ChromeOptions object as an argument so that our browser session will inherit the properties that we have just set.
You will see that the web page doesn't show any error we saw earlier on running the code. Also, note that the page title is not "Privacy error" in the console logs-
Note: For your practice, try by keeping the argument as "False" and seeing the same code results.
How does Selenium Webdriver handle SSL certificate in Firefox?
Before Selenium 4, We used to handle SSL certificate errors in Firefox using FirefoxOptions or FirefoxProfile or DesiredCapabilities. But with the introduction of the latest and updated version on Selenium WebDriver, the SSL certificate is auto handled for Firefox. Unlike the error we face in Chrome and other browsers, we need not write the additional code lines to accept the untrusted SSL certificates in Firefox. Let us see the execution without writing any code to handle SSL certificates in Selenium for Firefox browser:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class HandleSsl {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "D:\\Selenium\\geckodriver.exe");
WebDriver driver = new FirefoxDriver();
driver.get("https://self-signed.badssl.com/");
System.out.println("The page title is : " +driver.getTitle());
driver.quit();
}
}
As you can see from the code above that, we did not use any additional class. On executing/ running the above code, you will see results like below-
Note: Since our article series is on Selenium 4, we assume that you must be using the latest selenium versions.
As you can see that you need not write any special code to handle untrusted certificates in Firefox; let's try out what if we explicitly use the FirefoxOptions with setAcceptInsecureCerts method having as an argument "False" for Firefox execution.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxOptions;
public class SSLHandling {
public static void main(String[] args) {
//Creating an object of the FirefoxOptions Class
FirefoxOptions firefoxOptions = new FirefoxOptions();
//Using the setAcceptInsecureCerts() method to pass parameter as False
firefoxOptions.setAcceptInsecureCerts(false);
WebDriver driver = new FirefoxDriver(firefoxOptions);
driver.get("https://self-signed.badssl.com/");
System.out.println("The page title is : " +driver.getTitle());
driver.quit();
}
}
- FirefoxOptions opts = new FirefoxOptions(); -- An object of FirefoxOptions is first created.
- opts.setAcceptInsecureCerts(false); -- We decline the acceptance of untrusted certificate by passing Boolean false as an argument to the Accept Insecure certificates method.
- WebDriver driver = new FirefoxDriver(opts); -- We are now passing the Firefox options to the WebDriver instance so that the browser opens with the preloaded setting.
Note: For your understanding and learning, execute the above code, and see the results.
How does Selenium Webdriver handle the SSL certificate in Edge?
As you must be aware, the Edge browser is slowly taking over the Internet Explorer, with the later to be deprecated soon. We will now see the handling of SSL in Selenium for the Edge browser by using EdgeOptions class provided by Selenium WebDriver. It is similar to using ChromeOptions or FirefoxOptions.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.edge.EdgeOptions;
public class SSLHandling {
public static void main(String[] args) {
//Creating an object of EdgeOptions class
EdgeOptions edgeOptions = new EdgeOptions();
//Accepting the Insecure certificates through boolean parameter
edgeOptions.setAcceptInsecureCerts(true);
//Creating instance of Edge driver by passing reference of EdgeOptions object
// Assuming EdgeDriver path has been set in system properties
WebDriver driver = new EdgeDriver(edgeOptions);
driver.get("https://self-signed.badssl.com/");
System.out.println("The page title is : " +driver.getTitle());
driver.quit();
}
}
As already said, the EdgeOptions work similar to the Firefox and Chrome options. Consequently, our code would be similar to that of the other two browsers but vary in using the class name.
- EdgeOptions ssl = new EdgeOptions(); -- Object of EdgeOptions is created.
- ssl.setAcceptInsecureCerts(true); -- Insecure certificates are accepted by using Boolean true as an argument.
- WebDriver driver = new EdgeDriver(ssl); -- The options now pass to the WebDriver instance to start with the desired settings.
The above code's execution will fetch us the web page after accepting the untrusted/insecure certificate and subsequently print the page's title.
How does Selenium Webdriver handle the SSL certificate in Safari?
For Safari, the untrusted certificates handling is a bit different. We need to execute a JavaScript piece that will allow the browser to pass through the certificate and navigate to the intended page. Let's see, how the error will look like in the Safari Browser when we try to navigate to a webpage having an untrusted SSL certificate:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.safari.SafariDriver;
public class SSLHandling {
public static void main(String[] args) {
WebDriver driver = new SafariDriver();
driver.get("https://revoked.badssl.com");
System.out.println("The page title is : " + driver.getTitle());
driver.quit();
}
}
When we execute the above test script, it will be stuck in the browser at the SSL certificate error screen, as shown below:
Also, it will show the output in the console, as shown below:
Now, to handle this scenario in the Safari browser, we can update the above-written Selenium script by adding the following piece of JavaScript:
CertificateWarningController.visitInsecureWebsiteWithTemporaryBypass()
It will allow bypassing the insecure SSL certificate temporarily. The final code snippet after inclusion of this JavaScript code will look as follows:
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.safari.SafariDriver;
public class SSLHandling {
public static void main(String[] args) {
WebDriver driver = new SafariDriver();
driver.get("https://revoked.badssl.com");
JavascriptExecutor js = (JavascriptExecutor)driver;
js.executeScript("CertificateWarningController.visitInsecureWebsiteWithTemporaryBypass()");
System.out.println("The page title is : " + driver.getTitle());
driver.quit();
}
}
When we execute the above code snippet, it will be able to skip the untrusted SSL certificate and will show the output as shown below:
As we can see, now the correct title of the page is printing.
Note: We can handle the SSL certificates in Selenium for the Internet Explorer browser, also using the same code of JavaScript.
Key Takeaways:
- SSL helps to establish a secure connection between the client and the server.
- Moreover, the SSL certificate works in incremental steps to establish a secure connection.
- In addition to that, Root, Intermediate, and Server certificates are the different types of certificates that the Certificate Signing Authority provides.
- Various types of SSL certificate errors are like Revoked, Self-Signed & Expired.
- Additionally, untrusted SSL certificates can be handled using ChromeOptions(), FirefoxOptions() & EdgeOptions() in Chrome, Firefox & Edge browsers respectively.
- Lastly, handling untrusted SSL in Safari can happen through JavaScriptExecutor.
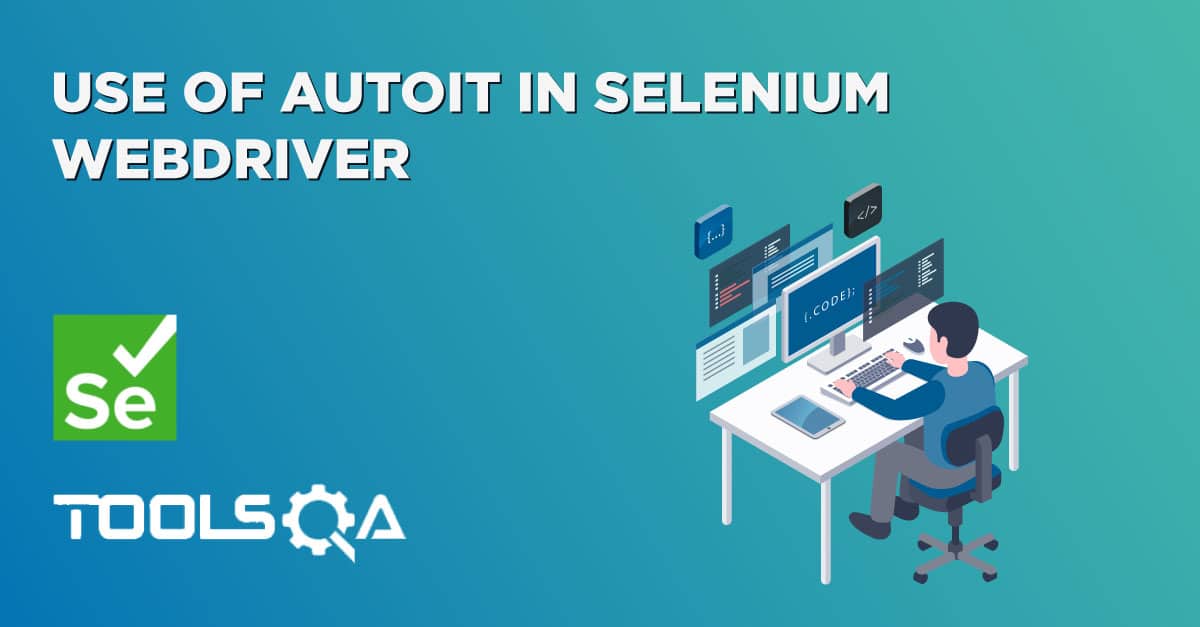
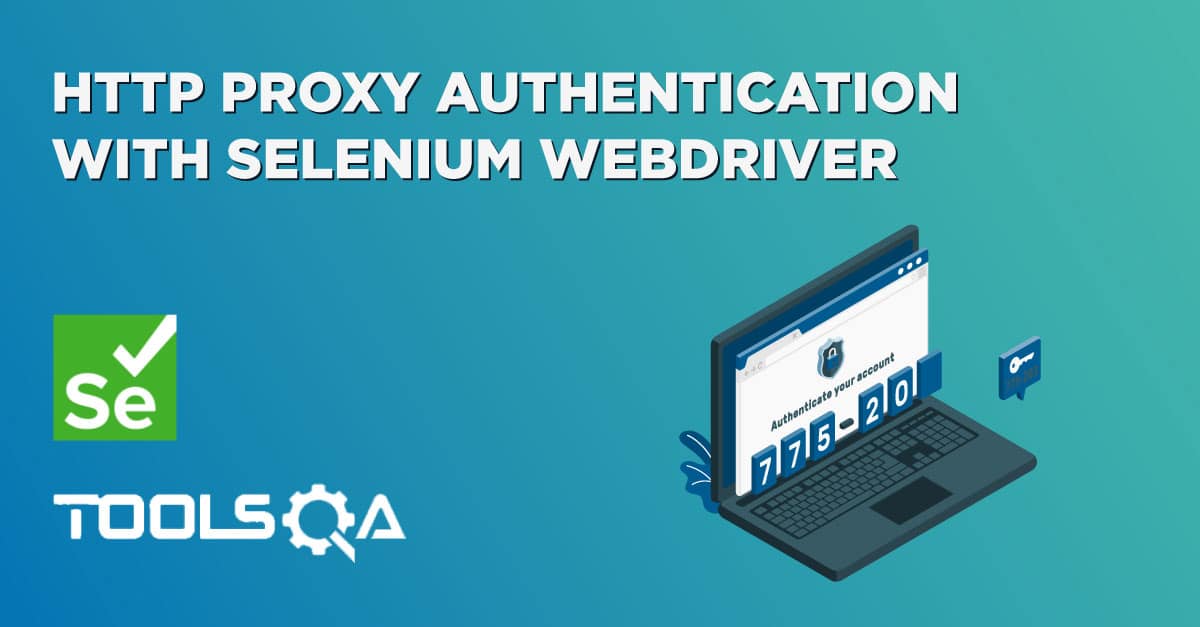