Till now we have been using so much of hard coded values in the classes, functions & scripts. It is easy to hard code the test data into the test scripts. But sometimes the fixed test data is also used in so many scripts and if it gets changed then it is a huge task to update the entire test scripts for example the URL of your test application. It remains the same but once you shifted to other environment, you need to change it in all of your test scripts. We can easily place the URL in Text file or Excel file outside our test scripts but Java gives us a special feature of Constants Variables which works exactly the same as Environment and Global variable in QTP.
Set Up Java Constant Variables
- Create a ‘New Class‘ file, by right click on the 'config' package and select New > Class and name it as 'Constants'.
- Assign logical variable names to the fixed data for e.g. Url, Username and Password.
Constants Class:
package config;
public class Constants {
//This is the list of System Variables
//Declared as 'public', so that it can be used in other classes of this project
//Declared as 'static', so that we do not need to instantiate a class object
//Declared as 'final', so that the value of this variable can be changed
// 'String' & 'int' are the data type for storing a type of value
public static final String URL = "https://www.store.demoqa.com";
public static final String Path_TestData = "D://Tools QA Projects//trunk//Hybrid KeyWord Driven//src//dataEngine//DataEngine.xlsx";
public static final String File_TestData = "DataEngine.xlsx";
//List of Data Sheet Column Numbers
public static final int Col_TestCaseID = 0;
public static final int Col_TestScenarioID =1 ;
public static final int Col_ActionKeyword =3 ;
//List of Data Engine Excel sheets
public static final String Sheet_TestSteps = "Test Steps";
// List of Test Data
public static final String UserName = "testuser_3";
public static final String Password = "Test@123";
}
Constants Variables are declared as 'public static', so that they can be called outside its class and in any other methods without Instantiating the class.
Constant Variables are declared as 'final', so that they cannot be changed during the execution.
3. Changes are required in the Action Keyword class.
Action Keyword Class:
package config;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class ActionKeywords {
public static WebDriver driver;
public void openBrowser(){
driver=new FirefoxDriver();
}
public static void navigate(){
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
//Constant Variable is used in place of URL
//As it was declared as 'static', it can be used by referring the class name
//Type the class name 'Constants' and press '.' dot, it will display all the memebers of the class Constants
driver.get(Constants.URL);
}
public static void click_MyAccount(){
driver.findElement(By.xpath(".//*[@id='account']/a")).click();
}
public static void input_Username(){
//Constant Variable is used in place of UserName
driver.findElement(By.id("log")).sendKeys(Constants.UserName);
}
public static void input_Password(){
//Constant Variable is used in place of Password
driver.findElement(By.id("pwd")).sendKeys(Constants.Password);
}
public static void click_Login(){
driver.findElement(By.id("login")).click();
}
public static void waitFor() throws Exception{
Thread.sleep(5000);
}
public static void click_Logout(){
driver.findElement (By.xpath(".//*[@id='account_logout']/a")).click();
}
public static void closeBrowser(){
driver.quit();
}
}
- Changes are required in the main Driver Script as well, need to replace all the hard coded values with the Constants Variables.
Driver Script:
package executionEngine;
import java.lang.reflect.Method;
import config.ActionKeywords;
import config.Constants;
import utility.ExcelUtils;
public class DriverScript {
public static ActionKeywords actionKeywords;
public static String sActionKeyword;
public static Method method[];
public DriverScript() throws NoSuchMethodException, SecurityException{
actionKeywords = new ActionKeywords();
method = actionKeywords.getClass().getMethods();
}
public static void main(String[] args) throws Exception {
//Instead of hard coded Excel path, a Constant Variable is used
String sPath = Constants.Path_TestData;
//Here we are passing the Excel path and SheetName to connect with Excel file
//Again a Constant Variable is used in place of Excel Sheet Name
ExcelUtils.setExcelFile(sPath, Constants.Sheet_TestSteps);
//Hard coded values are used for Excel row & columns for now
//In later chapters we will use these hard coded value much efficiently
//This is the loop for reading the values of the column 3 (Action Keyword) row by row
for (int iRow=1;iRow<=9;iRow++){
//Constant Variable is used in place of Column number
sActionKeyword = ExcelUtils.getCellData(iRow, Constants.Col_ActionKeyword);
execute_Actions();
}
}
private static void execute_Actions() throws Exception {
for(int i=0;i<method.length;i++){
if(method[i].getName().equals(sActionKeyword)){
method[i].invoke(actionKeywords);
break;
}
}
}
}
Note: For any explanation on above uncommented code, please refer previous chapters.
Project folder in Eclipse will look like this now:
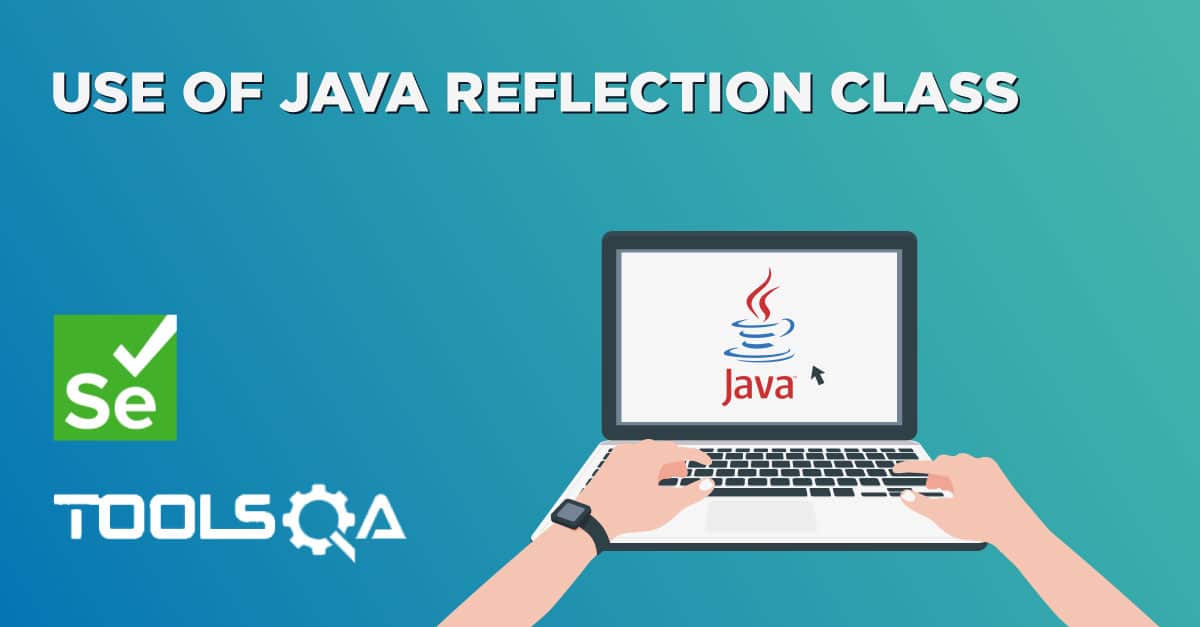
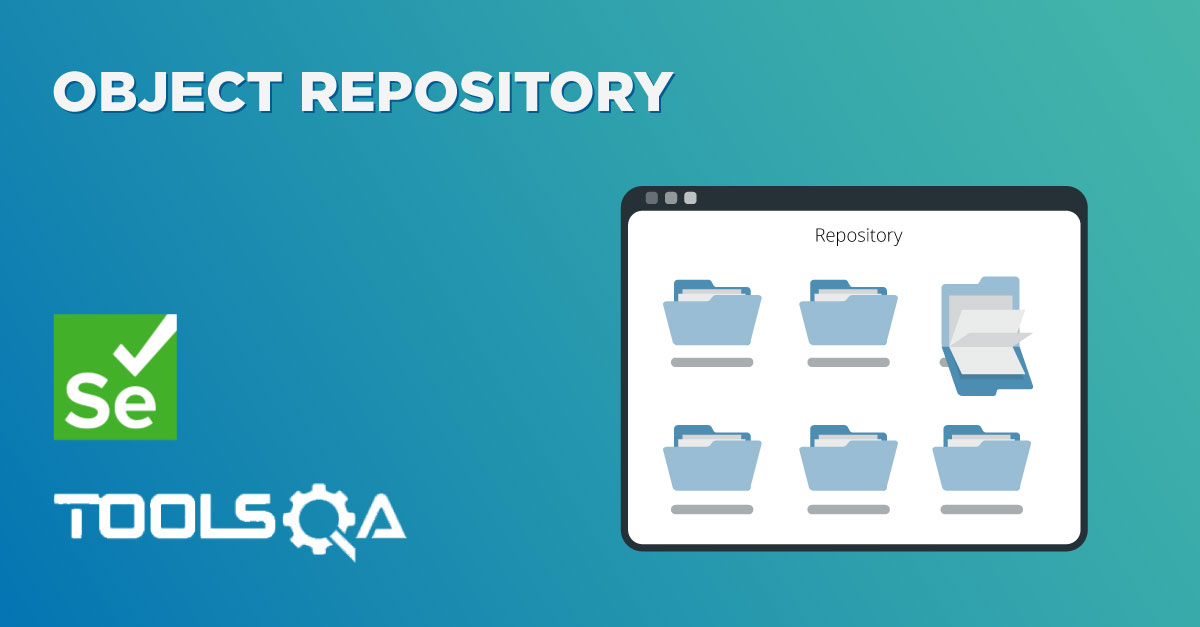