What is JavaScript?
JavaScript is the preferred language inside the browser to interact with HTML dom. This means that a Browser has JavaScript implementation in it and understands the JavaScript commands. You can disable it using browser options in your browser.
JavaScript was also the language that was used by earlier Selenium versions, it is still used by Selenium web driver to perform some actions. For e.g. Selenium has Xpath implemented in JavaScript for IE, to overcome the lack of Xpath engine in IE. Understanding JavaScript is fun and it can enable you to do lot of cool things that otherwise you may find tricky. Let's understand how
WebDriver gives you a method called Driver.executeScript which executes the JavaScript in context of the loaded browser page.
What can JavaScript and Selenium JavaScriptExecutor do for us?
The first thing to know that the JavaScriptExecutor comes separately and also comes under the WebDriver but both do the same thing. Within the WebDriver, it is named as ExecuteScript. Below you will find the examples for both. You can use any of it.
Well, practically anything you want to do in browser. Let's see how, we try to parallel selenium commands with JavaScript.
Evaluating Xpaths
You can use javascripts to find an element by Xpath. This is a brilliant way to skip internal Xpath engines. Here is what you have to do
WebElement value = (WebElement) Driver.executeScript("return document.evaluate( '//body//div/iframe' ,document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null ).singleNodeValue;");
Notice the command document.evaluate(). This is the Xpath evaluator in javascript. Signature of the function is
document.evaluate( xpathExpression, contextNode, namespaceResolver, resultType, result );
- xpathExpression: A string containing the XPath expression to be evaluated.
- contextNode: A node in the document against which the xpathExpression should be evaluated, including any and all of its child nodes. The document node is the most commonly used.
- NamespaceResolver: A function that will be passed any namespace prefixes contained within xpathExpression which returns a string representing the namespace URI associated with that prefix.
- resultType: A constant that specifies the desired result type to be returned as a result of the evaluation. The most commonly passed constant is XPathResult.ANY_TYPE which will return the results of the XPath expression as the most natural type.
- result: If an existing XPathResult object is specified, it will be reused to return the results. Specifying null will create a new XPathResult object
Finding Element
Using selenium we can find any element on a page using something similar to
Driver.findElementById("Id of the element");
we can do the same thing using JavaScript like so
WebElement searchbox = null;
searchbox = (WebElement) ff.executeScript("return document.getElementById('gsc-i-id1');", searchbox);
you will get the element in the searchbox variable.
Finding elements Selenium command
List\<WebElement\> elements = List\<WebElement\> Driver.findElementByTagName("div");
using JavaScript
List elements = (List') ff.executeScript("return document.getElementsByTagName('div');", searchbox);
Changing style attribute of the elements
You can change the style property of elements to change the rendered view of the element. For e.g this is how you can create a border around the about me element on toolsQa home page. See the below images of same element before and after creating border
Here is the code.
Driver.executeScript("document.getElementById('text-4').style.borderColor = 'Red'");
Coloring elements can also help us take screenshots with visual markers to identify problematic elements.
Getting Element Attributes
You can get any value of valid attributes of the element. For eg
Driver.findElementById("gsc-i-id1").getAttribute("Class");
this code will get the value of class attribute of an element with id = gsc-i-id1.
In Javascript same thing can be executed using
String className = Driver.executeScript("return document.getElementById('gsc-i-id1').getAttribute('class');"));
Total Frames in Browser
Let's say you want to know total number of frames inside a webpage, including the Iframes. You cant do it using selenium directly, you may need to create your own logic to parse and find frames. However, in JavaScript is simple and is done like this.
ff.executeScript("document.frames.length;");
Beware of this one. It only works in IE, can't get it to work for Mozilla.
Adding an Element in DOM
Just for fun if you want to add an element in the DOM. Let's add a button HTML object
Driver.executeScript("var btn=document.createElement('BUTTON');"
+ "document.body.appendChild(btn);");
Size of Window
Finding the size of the inner browser window. It is the size of the window in which you see the web page.
Driver.executeScript("return window.innerHeight;")
Driver.executeScript("return window.innerWidth;")
Navigating to different Page
ff.executeScript("window.location = 'https://yahoo.com'");
What is JavaScriptExecutor?
JavaScriptExecutor is an interface that provides a mechanism to execute Javascript through selenium driver. It provides “executescript” & "executeAsyncScript" methods, to run JavaScript in the context of the currently selected frame or window.
Generate Alert Pop Window
JavascriptExecutor js = (JavascriptExecutor)driver;
Js.executeScript("alert('hello world');");
Click Action
JavascriptExecutor js = (JavascriptExecutor)driver;
js.executeScript("arguments[0].click();", element);
Refresh Browser
JavascriptExecutor js = (JavascriptExecutor)driver;
driver.executeScript("history.go(0)");
Get InnerText of a Webpage
JavascriptExecutor js = (JavascriptExecutor)driver;
string sText = js.executeScript("return document.documentElement.innerText;").toString();
Get Title of a WebPage
JavascriptExecutor js = (JavascriptExecutor)driver;
string sText = js.executeScript("return document.title;").toString();
Scroll Page
JavascriptExecutor js = (JavascriptExecutor)driver;
//Vertical scroll - down by 150 pixels
js.executeScript("window.scrollBy(0,150)");
Similarly, you can execute practically any JavaScript command using Selenium. I hope this blog gives you an idea of what can be done and you can take it forward by playing around with different JavaScripts.
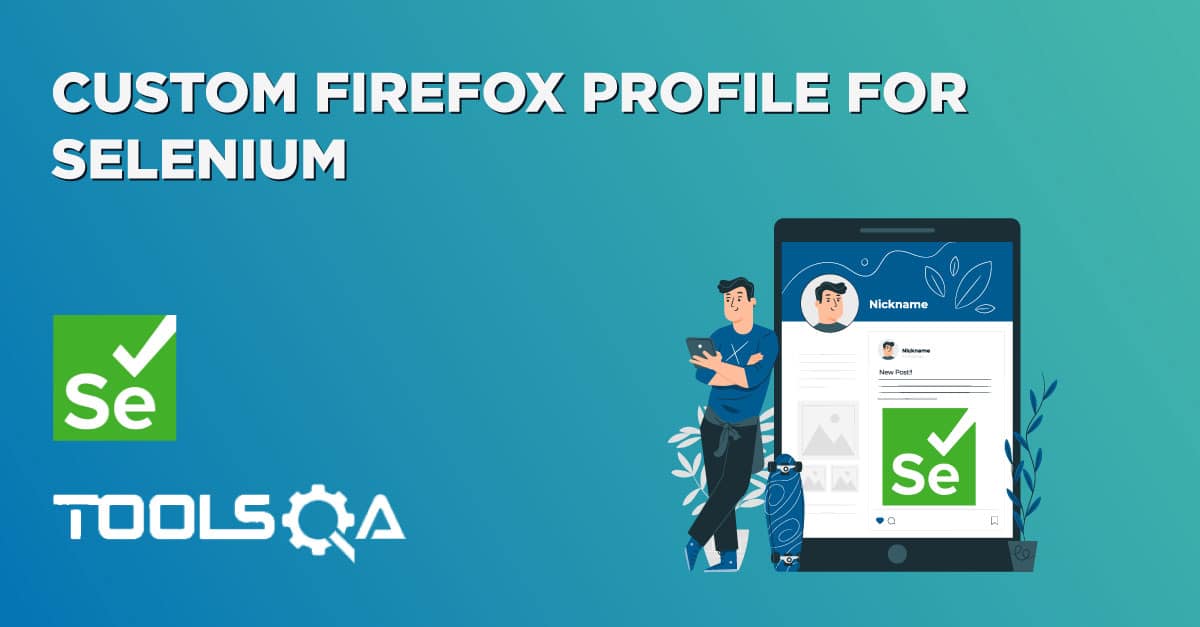
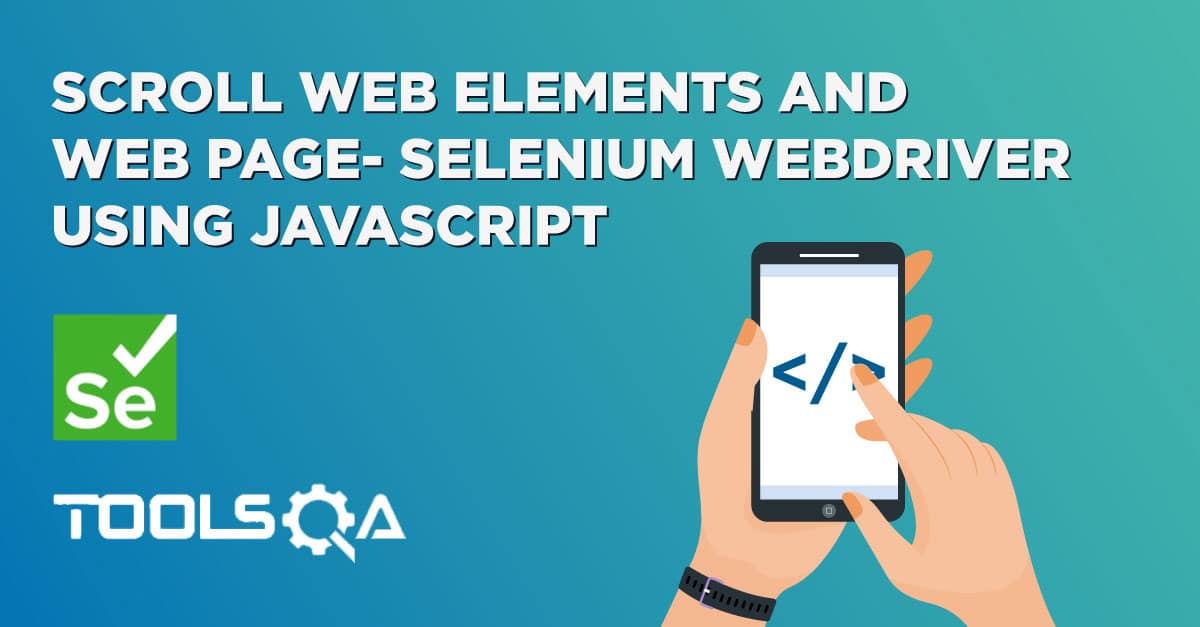