Javascript, these days, is one of the most used scripting languages which is used mostly on the client-side scripting like having validations in Html pages. But nowadays Javascript is becoming more popular when NodeJS has been released and developers started to write more powerful frameworks like angular, react which are written in Javascript and run on NodeJS server.
Now the most important question is why QA needs to learn Javascript. After Nodejs is introduced new tools for automation are also introduced which makes QA automation scripts easy to write and maintain. Some of the automation tools are the most commonly used selenium, webdriverIO, Protractor, Puppeteer, etc.
We have broken down the Javascript tutorials into 3 parts as below:
Javascript Tutorial - Basics:
In basics, we mostly concentrate on very basic details for the Novice who does not know anything about Javascript.
What is JavaScript: JavaScript is a lightweight and robust frontend scripting language that is used for web, gaming, and mobile application development the most. Historically, it’s creation took place to make dynamic web pages. The programs written in javascript were scripts. Scripts were a part of HTML and ran automatically when the web page loaded in the browser.
Run JavaScript Programs: JavaScript is an interpreted language where code is explained and executed at the runtime. Additionally, we know that web browsers understand HTML and CSS and converting those languages into a visual display on the screen.
Variables: Each programming language has a fundamental need to store data in memory for doing some computations on that data. Now to store and access this data, the memory location needs a name, and this name is called “Variable”. In addition to this, each memory location can store only a specific type of data. And the “DataType” of the variable helps in identifying this.
Functions: Unlike other programming languages, JavaScript functions are objects. In other words, it is an instance of the Function type. Consequently, it has properties and methods like other objects. Also, the name of a function is merely a pointer that points to the function object. Let’s discuss in the below sections, how to declare and invoke functions in JavaScript.
Operators: Operators are the symbols or keywords which signifies the operation that needs to carry out on the operands. In other words, an operator is capable of either manipulating a particular value or producing a result by comparing or operating on the given values. Like other programming languages, JavaScript also supports multiple types of operators.
Conditional Statements: Javascript has specific statements enabled in it that allow us to check a condition, like all other programming languages. And it then executes certain parts of code depending on whether the condition is true or false.
Loops: Loops are one of the most fundamental concepts available in all programming languages. The loop will execute the set of code repeatedly until the given condition is satisfied. Loop will ask a question; if the given answer is satisfied, then it will perform some actions, again it will ask a question, and this repeats until no further action required. Each time the question is asked, it is called an iteration.
Comments: Comments are one of the basic functionality provided by all the programming languages. Good comments convey essential information to the human reader of the code that the code cannot convey by itself. They make the source code more natural for humans to understand, and compilers and interpreters generally ignore them.
Equality/Inequality Operators:Comparing any two values for equality/inequality is one of the basic functionalities provided by any programming language. Each programming language provides some operators to compare all kinds of operands. JavaScript also inherits and extends the same concept and provides two variants of operators for comparing whether two operands are equal or not.
Arrays: The array is one of the most used data structure. Additionally, it stores a collection of similar kinds of objects. Moreover, it helps to organize the data so that values can be easily maintained, searched, and sorted.
Javascript Tutorial - Intermediate
In these tutorials, we are concentrating on the intermediate level, more on Arrays inbuilt functions, object, Different inbuilt objects like String, Math.
Simplify Arrays using inbuilt functions: Arrays in JavaScript provide multiple inbuilt functions for the manipulation of array elements. We already covered a few of them related to the addition and deletion of individual elements to the arrays in the previous article.
Let vs Var vs Const: Let, Var, and Const are the various ways that JavaScript provides for declaration of variables. Var is an old way of declaring variables. Whereas, Let & Const came into the picture from the ES6 version. Before starting the discussion about JavaScript let Vs var Vs const, let's understand what ES is? ES stands for Ecma Script, which is a scripting language specification specified by ECMA international. It standardized various implementations of JavaScript.
Callback Function: A callback, as the name suggests, is a function that is to execute after another function has finished executing. As we know, in JavaScript, functions are objects. Because of this, functions can take functions as arguments, and other functions can also return it. Functions that take the additional function as a parameter are called higher-order functions, and the function which passes as an argument is called a callback function.
Strings: Similar to other programming languages, the String is one of the data types in Javascript, which represent a sequence of characters. Still, in JavaScript, String can be both a primitive data type and a composite data type.
Math Class: JavaScript’s Math object performs mathematical operations on numbers. Unlike other global objects, Math is not a constructor. In other words, all the properties and methods of Math are static, and one can call them by using Math as an object without creating it. Additionally, the Math object provides a set of properties and methods for mathematical constants and functions.
Objects: An object is a non-primitive data type that represents a collection of properties and the methods which manipulate and expose those properties. In other words, we can think of an object as a list that contains items, and a name-value pair store each item in the list. Objects in JavaScript, similar to other programming languages, can be compared to real-life objects.
Javascript Advanced
In these tutorials, we are concentrating on more advanced topics like scheduling, event handlers, error handling, Promises, etc.
Scheduling Tasks in JavaScript: There can be multiple scenarios where a programmer decides to execute a function at a later time, instead of running it instantaneously. This kind of behavior is "scheduling as call" or "scheduling a timeout".
Promises in JavaScript: A promise in real life is just an assurance about ‘something’. So what happens when somebody makes a promise to you? They give you an assurance based on which you can plan something. Now, the promise can either be kept or broken. So, when a promise is kept you expect something out of that promise. You can make use of the output of a promise for your further actions or plans. But, when a promise is broken, you would like to know why the person who made the promise was not able to keep up his side of the bargain and will take the next action accordingly.
DOM in JavaScript: DOM is a data representation of the objects in the HTML and XML pages. The document loaded in your browser is represented by a document object model. Moreover, it is a "tree structure" representation created by the browser that enables the HTML structure to be easily accessed by programming languages.
Closures in JavaScript: Closures are one of the fundamental concepts of JavaScript that every JavaScript developer should know and understand. Yet, it's a concept that confuses many new JavaScript developers. Having an accurate understanding of closures will help you to write better, more efficient, and clean code. A closure is a function that has access to its outer function scope even after the return of the outer function. It means a closure can access variables and arguments of its outer function even after the function has finished.
Async/Await in JavaScript: We all know that JavaScript is the synchronous and single-threaded programming language. i.e., only one task can be performed at any point in time. But on the contrary, JavaScript also provides the ability to make it behave like an asynchronous language with the help of promises mechanisms. Async and Await concept, which was introduced in ES 2017, is an advanced way of handling "Promises in JavaScript".
Arrow operators in JavaScript: An Arrow Function in JavaScript is a syntactically compact option/ alternative to a regular function expression. These are anonymous functions with their unique syntax that accept a fixed number of arguments and operate in the context of their enclosing scope - i.e., the function or other code where they are defined.
This Keyword in JavaScript: All the object-oriented programming languages such as C++, Java, etc provides the "this" keyword to refer to an instance of an object from within its own class definition. But the "this" keyword in JavaScript has varying usage and creates a lot of confusion to someone who is new to JavaScript.
Prototype: Dynamically altering an object in JavaScript: A prototype is existing inbuilt functionality in JavaScript. Whenever a function is created, JavaScript adds a prototype property to that function. A prototype is an object, where it can be used to add new variables and methods to the existing object. i.e., Prototype is a base class for all the objects and it helps us to achieve the inheritance.
Destructuring in JavaScript: Consider a scenario, when you need to assign the array values or properties of an object to individual variables. Wasn't that a tedious task to access and assign each individual value of the array or object and then assign to the individual variable. Destructuring has made it very easy in JavaScript.
Rest Parameters and Spread operator: All the programming languages provide different ways to pass an indefinite arbitrary number of parameters to a function. Javascript has also provided concepts that make the "passing of arbitrary parameters" to a function very easy.
Classes in JavaScript: During programming, we all should have faced the scenarios, when multiple instances of one type need to be created. To support such functionality, the object-oriented programming came into existence, which used the concept of classes to represent living types and allowed to create multiple objects of the same type. Similar to other OOPS based programming languages, JavaScript also supports the concept of classes. Classes were introduced in JavaScript from ES6 onwards.
Error Handling in JavaScript: Error handling, as the name states, is a strategy used to handle the errors or exceptions which occur at runtime. As Javascript is a loosely coupled language, so it doesn't raise many errors at compile/interpretation level and you will see many errors at runtime only
Event Handling in JavaScript: When an event occurs like click or mouse-over action then programming to javascript code to perform some actions against it. The function that executes in response to an event is called an event handler. The event handlers are properties on some of the DOM elements to manage how that element should react to an event.
Regular Expressions in JavaScript: A JavaScript Regular Expression is an object, which specifies a pattern of characters. Moreover, a typical example of a regular expression implementation is the find and replace functionality provided by all the text editors.
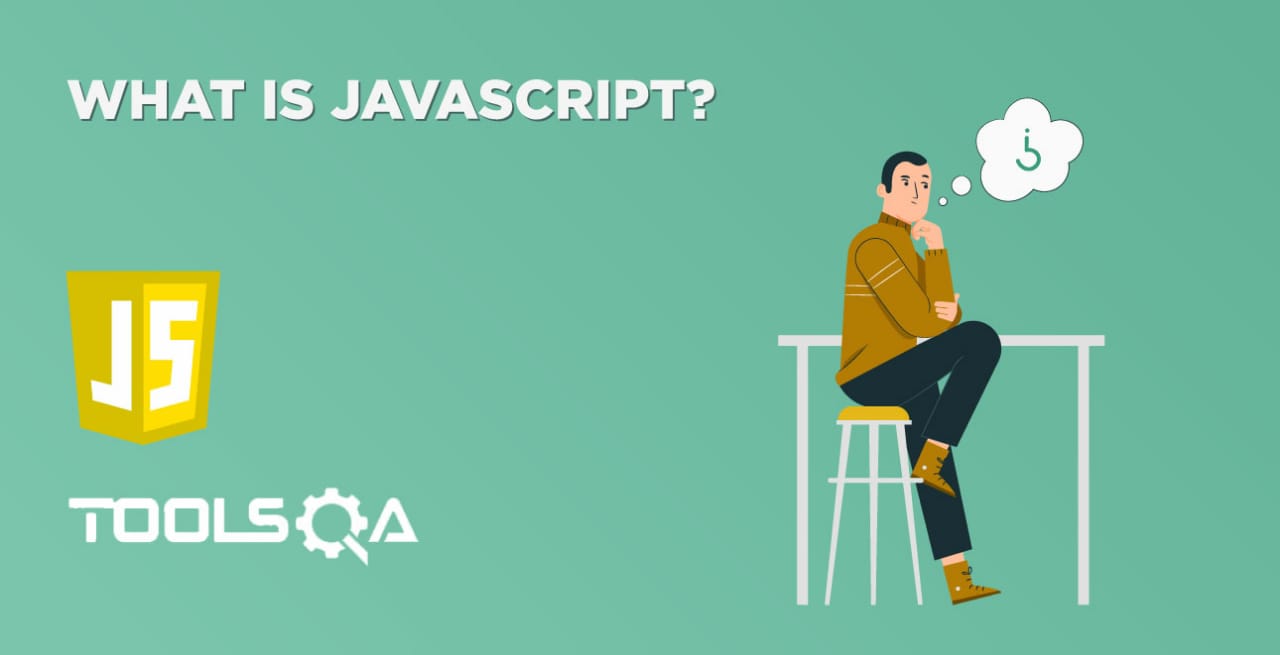