In most of the web application, we have few set of actions which are always executed in the series of actions. Rather than writing those actions again and again in our test, we can club those actions into a method and then calling that method in our test script. Modularity avoids duplicacy of code. In the future, if there is any change in the series of action, all you have to do is to make changes in your main modular method script. No test case will be impacted by the change.
How to do it...
Look for repeated functionality in your application, for example, the ‘login’ functionality. We can simply wrap this functionality in a method and we can give it a sensible name.
-
Create a 'New Package' file and name it as 'appModule', by right click on the Project and select New > Package. We will be creating different packages for Page Objects, Utilities, Test Data, Test Cases and Modular actions. It is always recommended to use this structure, as it is easy to understand, easy to use and easy to maintain.
-
Create 'New Class' and name it as SignIn_Action by right click on package 'appModule' and select New > Class. It will add new class 'SignIn_Action' under package 'appModule'.
3) Now create a Public Static Void Method and name it as Execute and club the following steps into it:
- Click on the My Account link.
- Enter Username
- Enter Password
- Click on the Submit button
This method will not have any Argument (driver) and Return value as it is a void method.
package framework.appModule;
import org.openqa.selenium.WebDriver;
import framework.pageObject.Home_Page;
import framework.pageObject.LogIn_Page;
public class SignIn_Action{
public static void Execute(WebDriver driver){
Home_Page.lnk_SignIn(driver).click();
LogIn_Page.txtbx_UserName(driver).sendKeys("testuser_1");
LogIn_Page.txtbx_Password(driver).sendKeys("Test@123");
LogIn_Page.btn_LogIn(driver).click();
}
}
Note: Please visit First Test Case & Page Object Model first, in case you are finding it hard to understand the above used SignIn_Action class.
- Create a New Class and name it as Module_TC by right click on the ‘automationFramework‘ Package and select New > Class. We will be creating all our test cases under this package.
Now convert your old POM_TC into the new Module based test case.
package automationFramework;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
// Import package appModule.*
import appModules.SignIn_Action;
import pageObjects.Home_Page;
public class Module_TC {
private static WebDriver driver = null;
public static void main(String[] args) {
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.store.demoqa.com");
// Use your Module SignIn now
SignIn_Action.Execute(driver);
System.out.println("Login Successfully, now it is the time to Log Off buddy.");
Home_Page.lnk_LogOut(driver).click();
driver.quit();
}
}
You will notice that your call to SignIn_Action will automatically execute all the steps mentioned under it.
Your Project explorer window will look like this now.
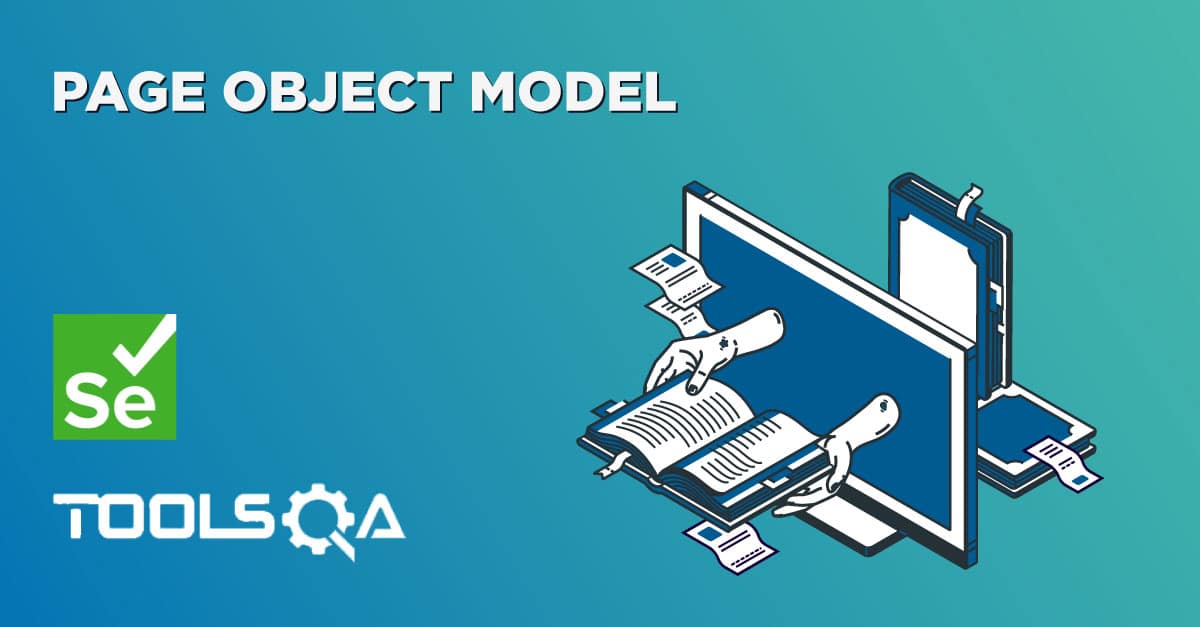
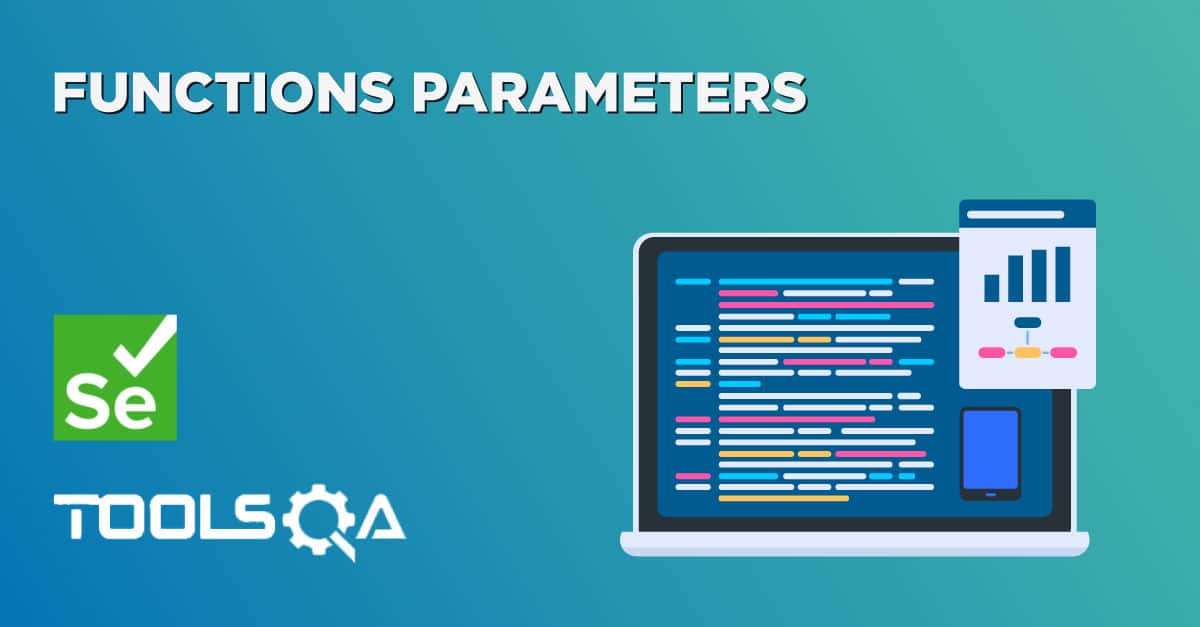