Table of Contents
In the last chapter of Exception handling we learned how to deal with exceptions in the framework. In all the methods of Action Keyword class and Excel Utils class, try catch block is set up. So now the framework is forced to eat all the exceptions and forced to change the value of 'bresult' boolean variable to 'false'. In this chapter we will learn to handle the situation when the 'bResult' variable is 'false'.
The whole point of setting the bResult variable to false is to stop the execution of the test case and mark the test case as failed.
How to do it...
Step 1: Create an extra column in the Test Case sheet and name it as 'Results'
Step 2: Create another extra column in Test Steps sheet and name it as 'Results'
Step 3: Create two new Constants variables for the results column of Test Case sheet and Test Step sheet
public static final int Col_Result =3 ;
public static final int Col_TestStepResult =5 ;
Step 4: Create two new Constants variables for the Pass results & Fail result
public static final String KEYWORD_FAIL = "FAIL";
public static final String KEYWORD_PASS = "PASS";
Step 5: Create a new method in the Excel Util class which will set the desired value to the Data Engine Excel sheet.
@SuppressWarnings("static-access")
//This method is use to write value in the excel sheet
//This method accepts four arguments (Result, Row Number, Column Number & Sheet Name)
public static void setCellData(String Result, int RowNum, int ColNum, String SheetName) throws Exception {
try{
ExcelWSheet = ExcelWBook.getSheet(SheetName);
Row = ExcelWSheet.getRow(RowNum);
Cell = Row.getCell(ColNum, Row.RETURN_BLANK_AS_NULL);
if (Cell == null) {
Cell = Row.createCell(ColNum);
Cell.setCellValue(Result);
} else {
Cell.setCellValue(Result);
}
// Constant variables Test Data path and Test Data file name
FileOutputStream fileOut = new FileOutputStream(Constants.Path_TestData);
ExcelWBook.write(fileOut);
//fileOut.flush();
fileOut.close();
ExcelWBook = new XSSFWorkbook(new FileInputStream(Constants.Path_TestData));
}catch(Exception e){
DriverScript.bResult = false;
}
}
Step 6: Modify the main Driver Script:
package executionEngine;
import java.io.FileInputStream;
import java.lang.reflect.Method;
import java.util.Properties;
import org.apache.log4j.xml.DOMConfigurator;
import config.ActionKeywords;
import config.Constants;
import utility.ExcelUtils;
import utility.Log;
public class DriverScript {
public static Properties OR;
public static ActionKeywords actionKeywords;
public static String sActionKeyword;
public static String sPageObject;
public static Method method[];
public static int iTestStep;
public static int iTestLastStep;
public static String sTestCaseID;
public static String sRunMode;
public static boolean bResult;
public DriverScript() throws NoSuchMethodException, SecurityException{
actionKeywords = new ActionKeywords();
method = actionKeywords.getClass().getMethods();
}
//The main script is divided in to three parts now
//First is main[] method, execution starts from here
public static void main(String[] args) throws Exception {
ExcelUtils.setExcelFile(Constants.Path_TestData);
DOMConfigurator.configure("log4j.xml");
String Path_OR = Constants.Path_OR;
FileInputStream fs = new FileInputStream(Path_OR);
OR= new Properties(System.getProperties());
OR.load(fs);
DriverScript startEngine = new DriverScript();
startEngine.execute_TestCase();
}
//Second method, this is to figure out the test cases execution one by one
//And to figure out test step execution one by one
private void execute_TestCase() throws Exception {
int iTotalTestCases = ExcelUtils.getRowCount(Constants.Sheet_TestCases);
for(int iTestcase=1;iTestcase<iTotalTestCases;iTestcase++){
//Setting the value of bResult variable to 'true' before starting every test case
bResult = true;
sTestCaseID = ExcelUtils.getCellData(iTestcase, Constants.Col_TestCaseID, Constants.Sheet_TestCases);
sRunMode = ExcelUtils.getCellData(iTestcase, Constants.Col_RunMode,Constants.Sheet_TestCases);
if (sRunMode.equals("Yes")){
iTestStep = ExcelUtils.getRowContains(sTestCaseID, Constants.Col_TestCaseID, Constants.Sheet_TestSteps);
iTestLastStep = ExcelUtils.getTestStepsCount(Constants.Sheet_TestSteps, sTestCaseID, iTestStep);
Log.startTestCase(sTestCaseID);
//Setting the value of bResult variable to 'true' before starting every test step
bResult=true;
for (;iTestStep<iTestLastStep;iTestStep++){
sActionKeyword = ExcelUtils.getCellData(iTestStep, Constants.Col_ActionKeyword,Constants.Sheet_TestSteps);
sPageObject = ExcelUtils.getCellData(iTestStep, Constants.Col_PageObject, Constants.Sheet_TestSteps);
execute_Actions();
//This is the result code, this code will execute after each test step
//The execution flow will go in to this only if the value of bResult is 'false'
if(bResult==false){
//If 'false' then store the test case result as Fail
ExcelUtils.setCellData(Constants.KEYWORD_FAIL,iTestcase,Constants.Col_Result,Constants.Sheet_TestCases);
//End the test case in the logs
Log.endTestCase(sTestCaseID);
//By this break statement, execution flow will not execute any more test step of the failed test case
break;
}
}
//This will only execute after the last step of the test case, if value is not 'false' at any step
if(bResult==true){
//Storing the result as Pass in the excel sheet
ExcelUtils.setCellData(Constants.KEYWORD_PASS,iTestcase,Constants.Col_Result,Constants.Sheet_TestCases);
Log.endTestCase(sTestCaseID);
}
}
}
}
private static void execute_Actions() throws Exception {
for(int i=0;i<method.length;i++){
if(method[i].getName().equals(sActionKeyword)){
method[i].invoke(actionKeywords,sPageObject);
//This code block will execute after every test step
if(bResult==true){
//If the executed test step value is true, Pass the test step in Excel sheet
ExcelUtils.setCellData(Constants.KEYWORD_PASS, iTestStep, Constants.Col_TestStepResult, Constants.Sheet_TestSteps);
break;
}else{
//If the executed test step value is false, Fail the test step in Excel sheet
ExcelUtils.setCellData(Constants.KEYWORD_FAIL, iTestStep, Constants.Col_TestStepResult, Constants.Sheet_TestSteps);
//In case of false, the test execution will not reach to last step of closing browser
//So it make sense to close the browser before moving on to next test case
ActionKeywords.closeBrowser("");
break;
}
}
}
}
}
Try giving it a run and see if it works fine on your system. Once you done that try changing the xpath of any object and see the framework handles the failed test case well or not.
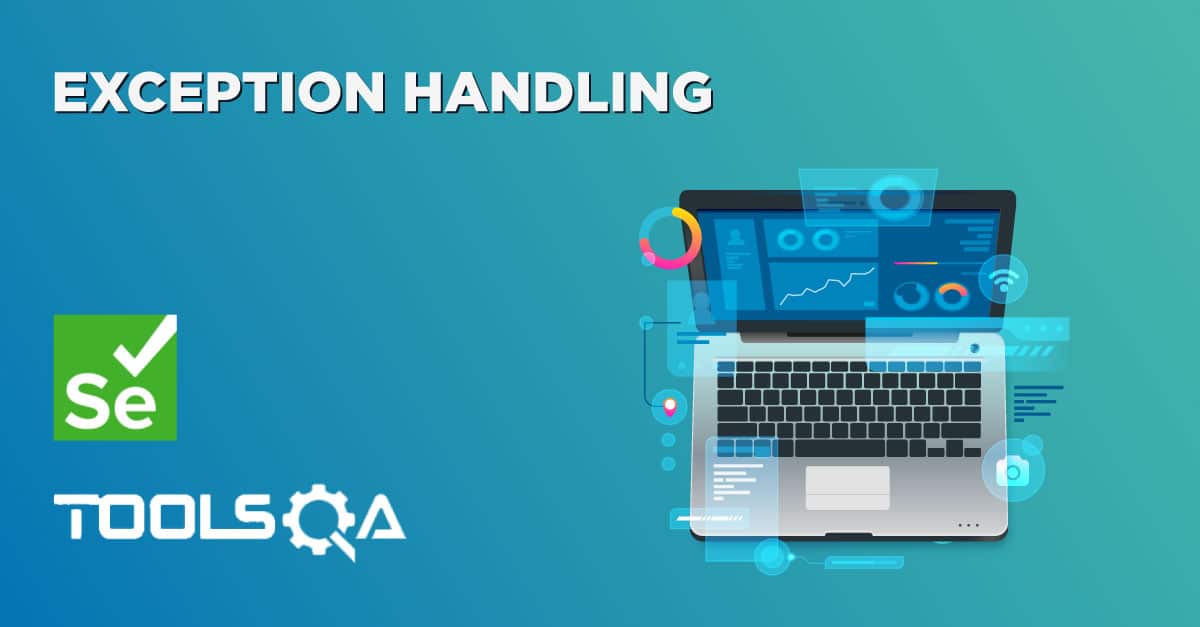
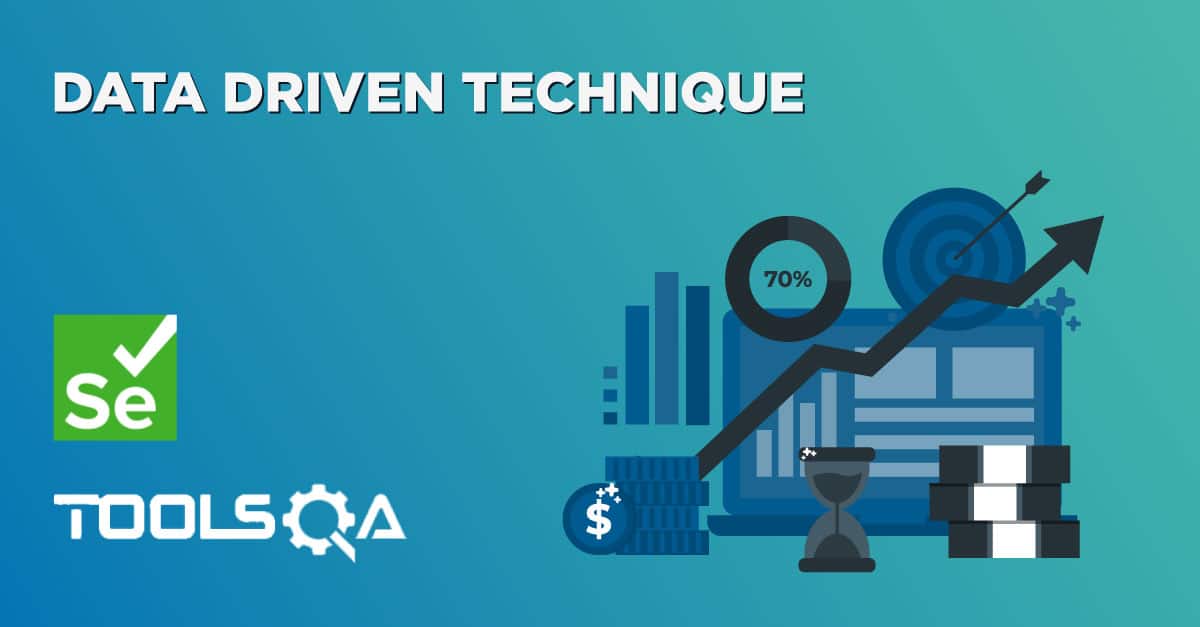