It is always a good practice to pass parameters when calling the method, rather than providing parameters inside the method. We can pass parameters through methods, just as in normal programming code. The code below will show us how we can login with parameterized username and password.
How to do it...
- First, let's have a look over our previous example of SignIn_Action class.
package framework.appModule;
import org.openqa.selenium.WebDriver;
import framework.pageObject.Home_Page;
import framework.pageObject.LogIn_Page;
public class SignIn_Action{
public static void Execute(WebDriver driver){
Home_Page.lnk_SignIn(driver).click();
LogIn_Page.txtbx_UserName(driver).sendKeys("testuser_1");
LogIn_Page.txtbx_Password(driver).sendKeys("Test@123");
LogIn_Page.btn_LogIn(driver).click();
}
}
Note: Please visit First Test Case, Page Object Model & Modularity first, in case you are finding it hard to understand the above used SignIn_Action class.
- Modify the above Execute method of class SignIn_Action to accept string Arguments (Username & Password).
package appModules;
import org.openqa.selenium.WebDriver;
import pageObjects.Home_Page;
import pageObjects.LogIn_Page;
public class SignIn_Action {
// Pass Arguments (Username and Password) as string
public static void Execute(WebDriver driver,String sUsername, String sPassword){
Home_Page.lnk_MyAccount(driver).click();
// Enter sUsername variable in place of hardcoded value
LogIn_Page.txtbx_UserName(driver).sendKeys(sUsername);
// Enter sPassword variable in place of hardcoded value
LogIn_Page.txtbx_Password(driver).sendKeys(sPassword);
LogIn_Page.btn_LogIn(driver).click();
}
}
3) Create a New Class and name it as Param_TC by right click on the ‘automationFramework‘ Package and select New > Class. We will be creating all our test cases under this package.
Now convert your old Module_TCin to the new passing parameters based test case.
package automationFramework;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import appModules.SignIn_Action;
import pageObjects.Home_Page;
public class Param_TC {
private static WebDriver driver = null;
public static void main(String[] args) {
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.store.demoqa.com");
// Pass the Arguments (Username and Password) to this method
SignIn_Action.Execute(driver, "testuser_1","Test@123");
System.out.println("Login Successfully, now it is the time to Log Off buddy.");
Home_Page.lnk_LogOut(driver).click();
driver.quit();
}
}
You see how easy it is to pass arguments to your methods. It increases your code readability.
Now your Project explorer window will look like this:
Note: But it is still not the best idea to hardcode your input anywhere in your test script, as it will still impact the bulk of your test scripts if there is any change in user data. I used this example just to give you an idea of how we use arguments in method.
In the next chapter of Constant Variable we will learn how to avoid hardcode values in our code.
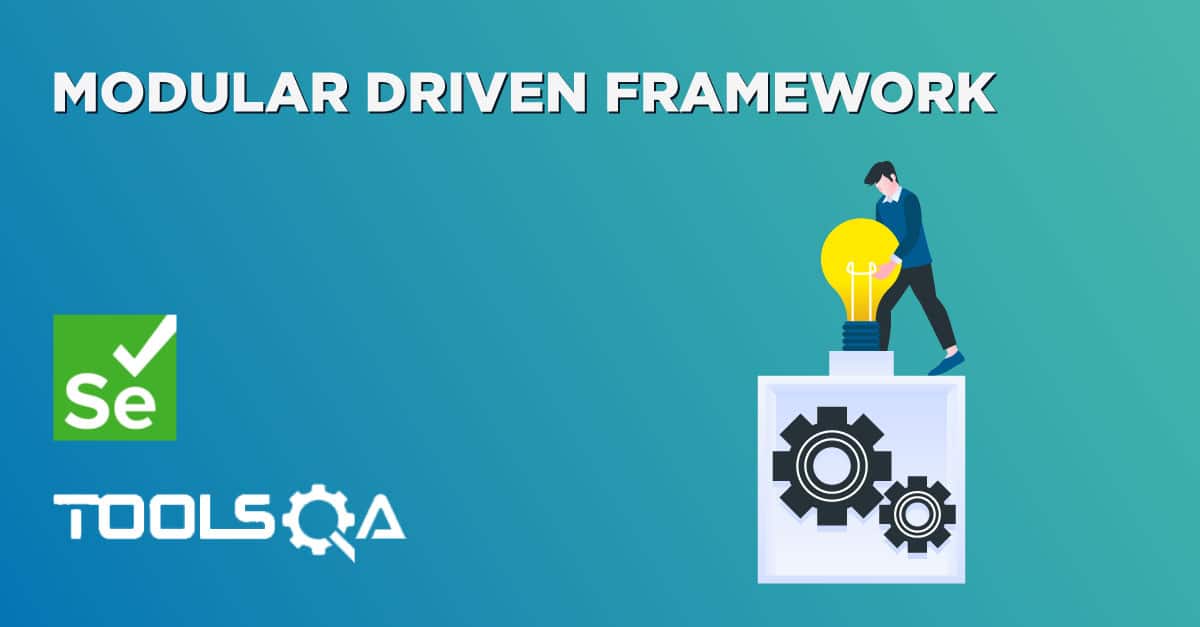
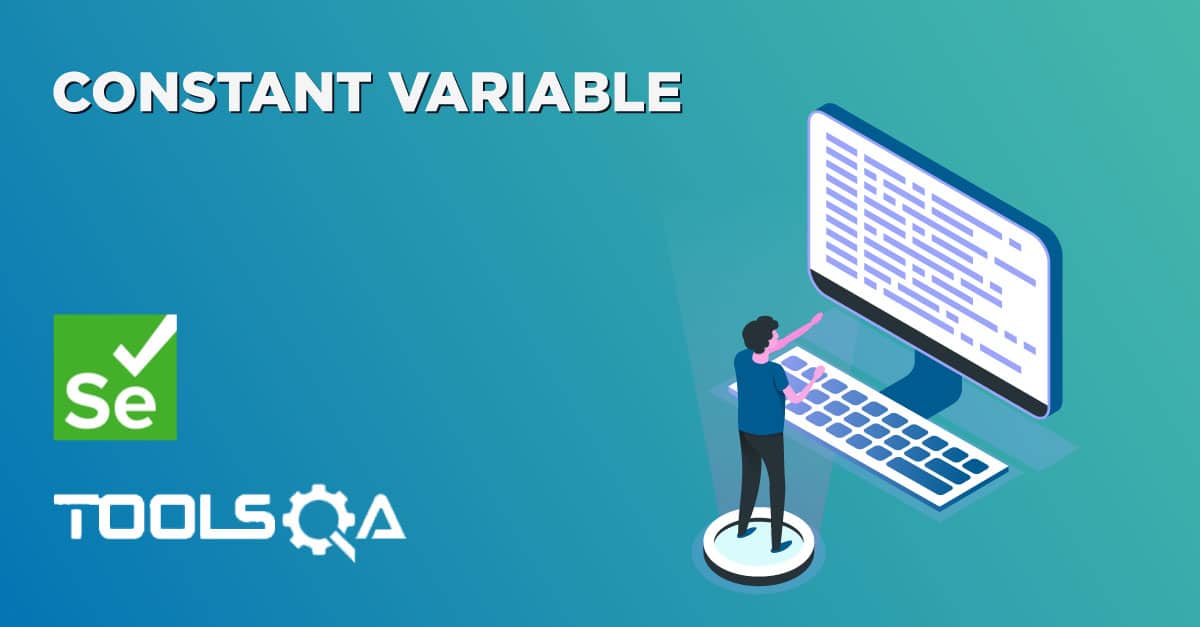