In this tutorial, the concepts of how to perform mouse events like Right Click and Double Click in Selenium are covered in details. Right click and Double click are two important user actions for interacting with a website. There comes a need to perform these actions in Selenium Test Automation.
How to Right Click in Selenium using Action Class?
Before jumping to Right click action in Selenium Automation, let's first understand What is Right Click in general.
What is Right click?
As the name suggests, when a user tries to click the Right mouse button on a website or a web element to look at its context menu.
E.g. This is a very common action used almost daily especially while working with Folders in Windows Explorer. Similarly in Gmail, performing right-click on any mail in the inbox opens a menu displaying the options like Reply, Delete, Reply All, etc. Out of which any option can be selected to perform the desired action.
In many cases, automation test scripts need to perform similar action i.e. right click on some element on the web page and then selecting an option from the displayed context menu. To perform the right-click action through a Selenium script, WebDriver API does not have the capability for right-click command like other Action commands: click, sendKeys.
That is where Action class comes into play by providing various important methods to simulate user actions. And one of the most commonly used methods of the class is contextClick(WebElement), which is used to perform the Right-Click action.
Note: Action class is present in package: org.openqa.selenium.interactions package
Right Click in Selenium?
Let's see how to use Action class methods to right click:
First, instantiate an Actions class:
Actions actions = new Actions(driver);
Now as seen above, the contextClick() method has argument WebElement to be passed. So, need to pass a WebElement object to the method. This WebElement should be the button or any web element on which we want to perform Right click. To find the element use the below command:
WebElement webElement = driver.findElement(Any By strategy & locator);
Here, you can use any By strategy to locate the WebElement like find element by its id, name attribute, etc. To know more on all By strategies, please refer Find Elements tutorial.
Now, when we have got the action class object and the element as well, just invoke perform() method for the right-click:
actions.contextClick(webElement).perform();
Let's see what happens internally when invoke the perform() method above:
- Move to Element: contextClick() method first performs mouseMove to the middle of the element location. This function performs the right click at the middle of the web element.
- Build: build() method is used to generate a composite action containing all actions. But if you observe, we have not invoked it in our above command. The build is executed in the perform method internally.
- Perform: perform() method performs the actions we have specified. But before that, it internally invokes build() method first. After the build, the action is performed.
Practice Exercise to Perform Right Click using Action Class in Selenium
Let’s consider an example from an already available demo page on Toolsqa as "http://demoqa.com/buttons".
In the above image, performing the right click on the button displays the context menu. Now let's write a selenium script to right-click the button using contextClick() method.
Find below the steps of the scenario to be automated:
- Launch the web browser and launch our practice page https://demoqa.com/tooltip-and-double-click/
- Find the required element i.e. button in our sample and do right click on the element
- Go to the options ‘copy’ and click on it
- Accept the alert message
- Close the browser to end the program
Find below the code for performing right click on the web element:
package com.toolsqa.tutorials.actions;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.interactions.Actions;
public class RightClickDemo {
public static void main(String[] args) {
//Note: Following statement is required since Selenium 3.0,
//optional till Selenium 2.0
//Set system properties for geckodriver
System.setProperty("webdriver.gecko.driver", "C:\\Selenium\\Toolsqa\\lib\\geckodriver.exe");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
// Launch the URL
driver.get("https://demoqa.com/buttons");
System.out.println("demoqa webpage displayed");
//Maximise browser window
driver.manage().window().maximize();
//Instantiate Action Class
Actions actions = new Actions(driver);
//Retrieve WebElement to perform right click
WebElement btnElement = driver.findElement(By.id("rightClickBtn"));
//Right Click the button to display Context Menu
actions.contextClick(btnElement).perform();
System.out.println("Right click Context Menu displayed");
//Following code is to select item from context menu which gets open up on right click, this differs
//depending upon your application specific test case:
//Select and click 'Copy me' i.e. 2nd option in Context menu
WebElement elementOpen = driver.findElement(By.xpath(".//div[@id='rightclickItem']/div[1]"));
elementOpen.click();
// Accept the Alert
driver.switchTo().alert().accept();
System.out.println("Right click Alert Accepted");
// Close the main window
driver.close();
}
}
Note: If confused to see Alert window action, take a look at the tutorial of How to handle Alerts in Selenium?
Now, let's move on to another important action i.e. Double Click.
How to Double Click in Selenium using Action Class?
What is Double click?
Double-click is a frequently used user action. The most common use of double click happens in File Explorer E.g. In File Explorer, any Folder or File in a folder can be opened by double-clicking on it.
Similarly, on any webpage, there might be some elements that require a double click to invoke an action on them. Similar to Right Click, in Selenium there is no WebDriver API command which has the capability to double click the webelement.
Hence, the Action class method doubleClick(WebElement) is required to be used to perform this user action.
Double click in Selenium
Let's see how to use Actions class methods to double click:
First, let's instantiate an Actions class
Actions actions = new Actions(driver);
Now as seen above, doubleClick() method has argument WebElement to be passed. So pass WebElement object to the method on which need to perform Double click.
WebElement webElement = driver.findElement(Any By strategy);
Again just like Right-Click, use any By strategy to locate the WebElement like find element by its id, name attribute, etc.
Now, just invoke build and perform for our double click
actions.doubleClick(webElement).perform();
doubleClick() method also follows the same process of Move to Element >> Build >> Perform which is same as for right-click.
Practice Exercise to Perform Double Click using Action Class in Selenium
Let’s consider the following example:
In the above sample, performing a double click on the button displays the alert message on the screen.
Find below the steps of the scenario to be automated:
- Launch the web browser and launch our practice page http://demoqa.com/buttons
- Find the required element i.e. button in our sample and do double click on the element
- Accept the alert message
- Close the browser to end the program
Find below the code for performing a double click on the web element:
package com.toolsqa.tutorials.actions;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.interactions.Actions;
public class DoubleClickDemo {
public static void main(String[] args) {
//Note: Following statement is required since Selenium 3.0,
//optional till Selenium 2.0
//Set system properties for geckodriver
System.setProperty("webdriver.gecko.driver", "C:\\Selenium\\Toolsqa\\lib\\geckodriver.exe");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
// Launch the URL
driver.get("https://demoqa.com/buttons");
System.out.println("Demoqa Web Page Displayed");
//Maximise browser window
driver.manage().window().maximize();
//Instantiate Action Class
Actions actions = new Actions(driver);
//Retrieve WebElement to perform double click WebElement
btnElement = driver.findElement(By.id("doubleClickBtn"));
//Double Click the button
actions.doubleClick(btnElement).perform();
System.out.println("Button is double clicked");
//Following code just click on OK button on alert , this differs
//depending upon application(under test) specific test case
// Accept the Alert
driver.switchTo().alert().accept();
System.out.println("Double click Alert Accepted");
//Close the main window
driver.close();
}
}
Summary
In this article, we have seen how we can perform Right Click and Double Click on web elements using Action Class methods. Actions class offers other various methods also to perform various keyboard events. We will see those in another article in the Selenium series.
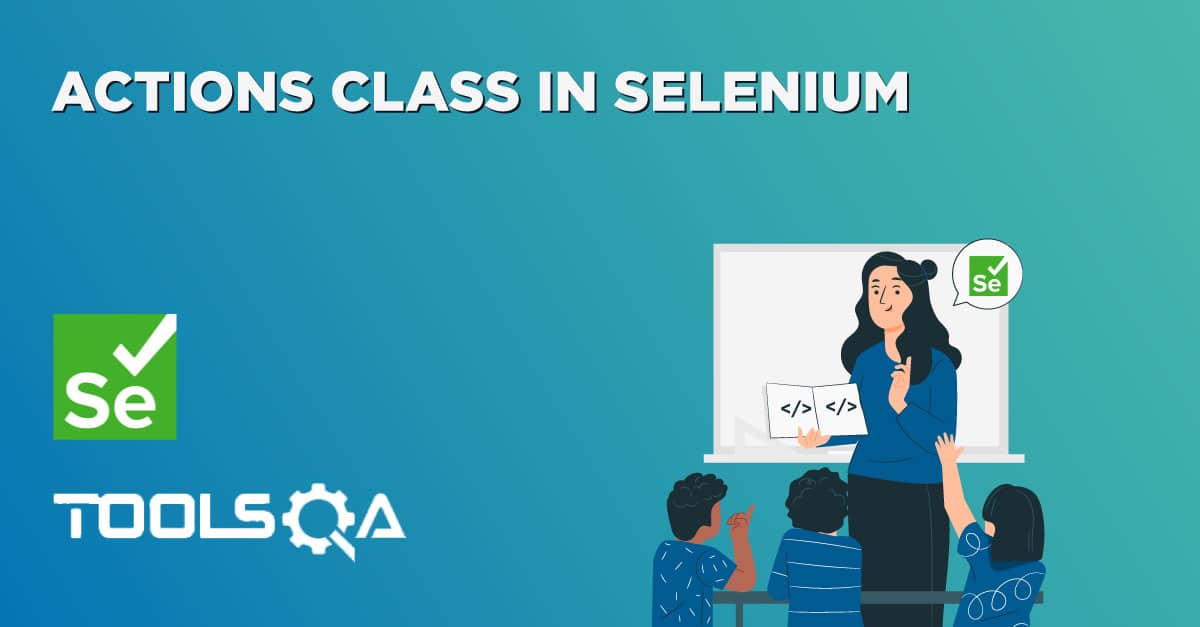
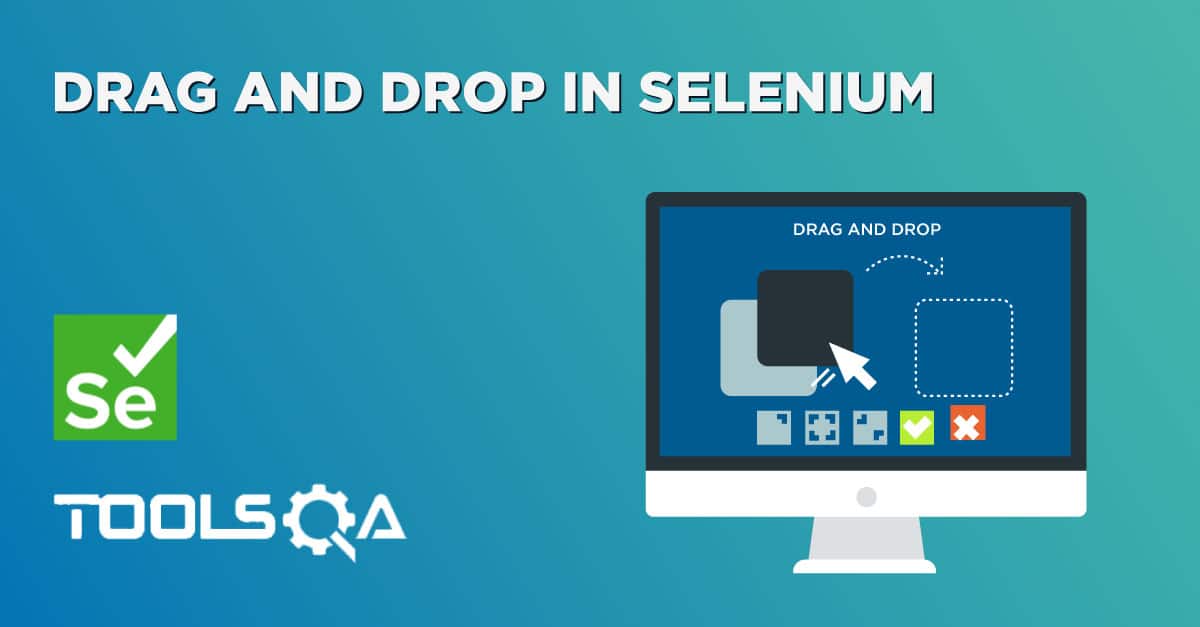