JSON is one of the most used Data-Exchange format. It is a light weight format represented as pure text. Due to the ability of almost all the languages to parse text, Json becomes independent of the programming language.
What is JSON?
JSON stands for JavaScript Object Notation. JSON is a human and machine-readable format to represent data as Structured Data. JSON is used primarily to transfer data from one computer to another or even between different programs on the same computer.
To visualize JSON, let us say that we want to represent a Person with following details in JSON
- First Name = Virender
- Last Name = Singh
- Age = 34
- Profession = Engineer
In JSON , this will turn out to be
{
"FirstName" : "Virender",
"LastName" : "Singh",
"Age" : 34,
"Profession": "Engineer"
}
At this point, we do not know the details of the JSON structure but we can still figure out what is being presented in JSON . Let us now understand different elements used in JSON .
Note: It is always beneficial to represent Keys without spaces in the name.
Key-Value Pairs
Key-Value pairs in JSON are used to represent a property of the Object. In the above example, we tried to represent a Person. This person has some properties like
- First Name
- Last Name
- Age
- Profession
Each of these properties have a value associated with it. For e.g First Name has a value of Virender. Similarly, Age has a value of 34. To write a Key-Value in JSON we have to follow these rules
- Key-value pairs are separated by a : (colon)
- Key is always present in Double Quotes " "
- Value could be anything depending on the Data Type
From the above example, a Key-Value pair is FirstName. Try to find other Key-Value pairs yourself
"FirstName" : "Virender"
Values can be of following data types
- Boolean: True or False
- Number: Numerical values
- Object: An associative array of Key-Value pairs
- Array: Associative array of just values
Object in JSON
In JSON an object is represented by a collection of Key-Value pairs. This collection of Key-Value pairs are grouped using { } (opening and closing curly braces}. Rules to writing an Object are
- Key-Value pairs should be separated by a , (Comma)
- Each Object should Start with an Opening { (Opening Curly Brace)
- Each Object should End with a Closing } (Closing Curly Brace)
An example here is the Person Object, discussed above. The Person object follows the rules mentioned for representing an Object
{
"FirstName" : "Virender",
"LastName" : "Singh",
"Age" : 34,
"Profession": "Engineer"
}
Array in JSON
Arrays are similar to Arrays that you know from any other programming language. In JSON an Array is collection of Values separated by Comma. Here are the rules to write an Array
- An Array starts with an opening [ (Bracket)
- An Array ends with a closing ] (Bracket)
- Values in the Array are separated by , (Comma)
To understand an Array let us add one more property to the Person Object. Let us add hobby also, a Person can have multiple hobbies. This makes it suitable to represent hobbies as an Array. As shown in the JSON below
{
"FirstName" : "Virender",
"LastName" : "Singh",
"Age" : 34,
"Profession": "Engineer",
"Hobbies" : ["Videos games", "Computers", "Music"]
}
See how multiple hobbies are represented using an Array. Array starts and ends with [ ] and contains values separated by ,
These are the different components of a JSON , using these components we can create complex JSON . As a small exercise for you, try to decode the JSON below and identify different structures involved in it.
{
"Description": "Map containing Country, Capital, Currency, and some States of that Country",
"Region": "Asia",
"Countries": [
{
"Country": "India",
"Data": {
"Capital": "New Delhi",
"minimum temp (Degree Celsius)": 6,
"maximum temp (Degree Celsius)": 45,
"Currency": "Rupee"
}
},
{
"Country": "Nepal",
"Data": {
"Capital": "Katmandu",
"minimum temp (Degree Celsius)": 9,
"maximum temp (Degree Celsius)": 23,
"Currency": "Nepalese rupee"
}
}
]
}
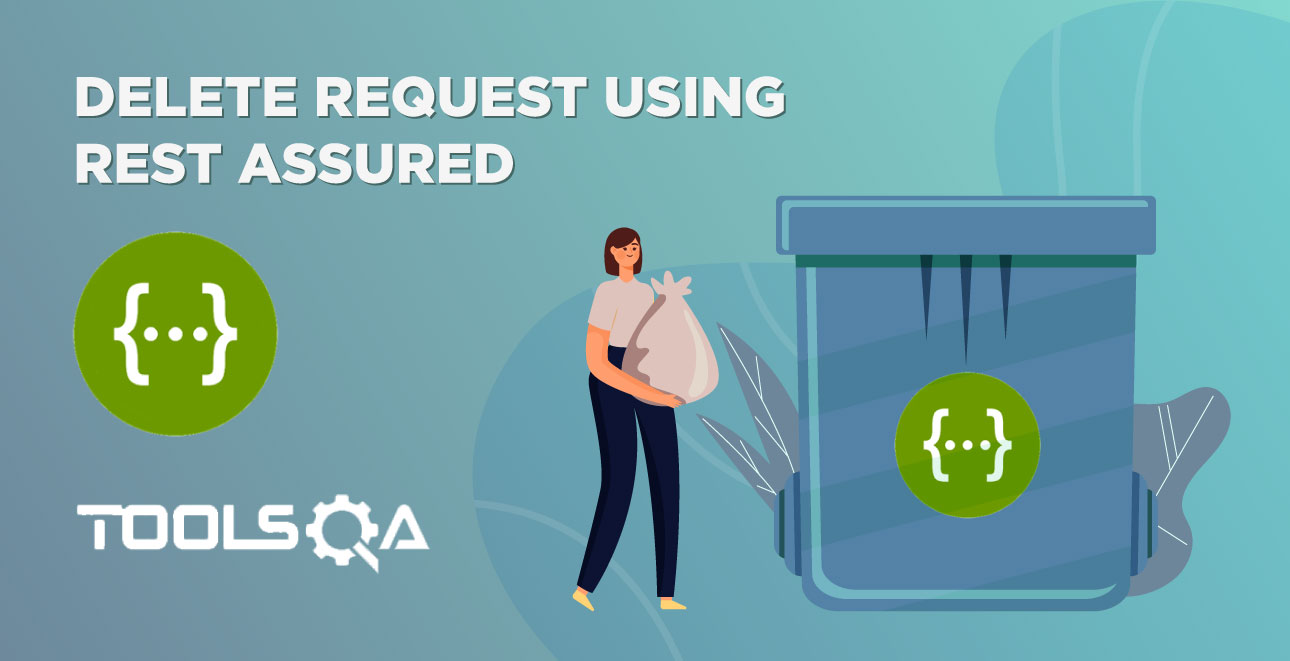
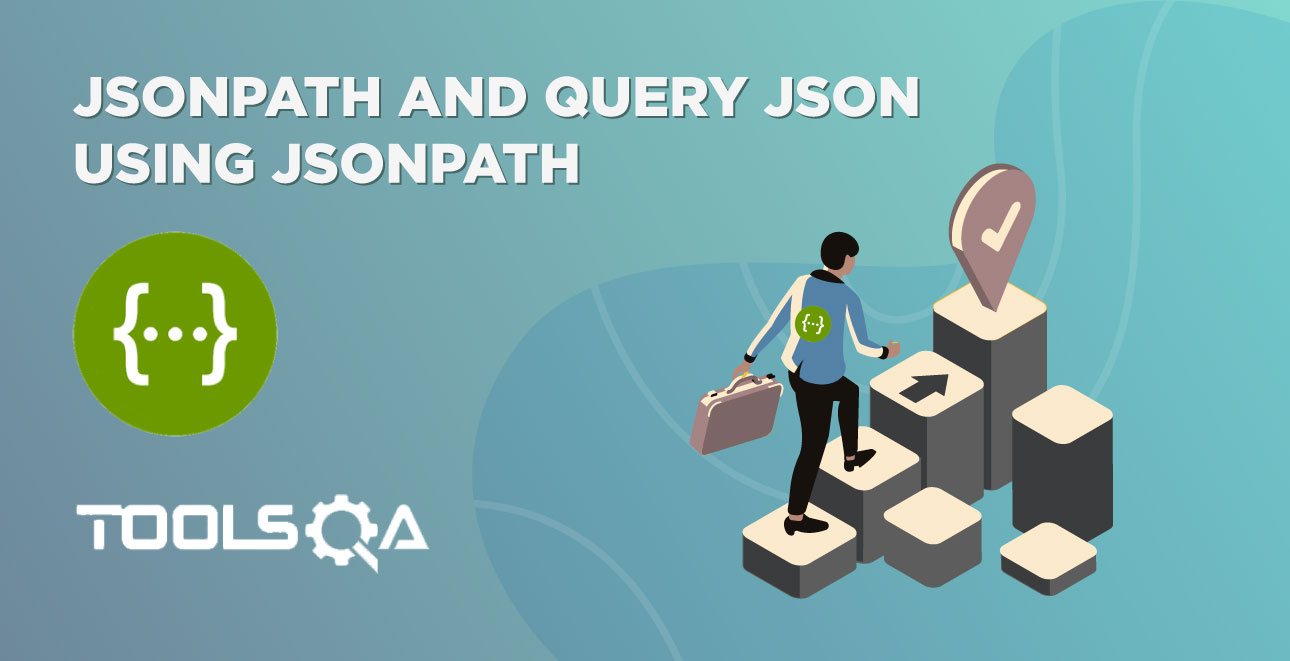