In this chapter, we will implement the Deserialization of JSON Responses we receive. It helps us to read the body of the response. Subsequently, in this process of deserialization, we will Convert JSON Response Body to Java Objects. It is also known as Object Representation of JSON responses.
Additionally, to learn more about the deserialization of JSON response, we will recommend you to go through the Deserialize JSON Response.
We will follow the below steps to achieve the deserialization of our responses in the framework. We followed them in the previous chapter of Convert JSON Request Body to Java Object as well.
- Create POJO classes for each of our Response Objects:
- Book
- Books
- Token
- User Account
- Replace the Response bodies in Step files with POJO class objects
- Run the tests
Convert JSON Response Body to Java Object
Firstly, we need to convert the JSON Response into a POJO class for our response object. So, let us learn to create a POJO class out of a JSON Response. Under deserialization, we will study our JSON body parameters and create a POJO class of it. Let's begin with a straightforward request example for Get A Book request:
Create POJO class Book
As a part of this request, we are sending the ISBN parameter to get details of a specific book.
Consequently, the response body from the Swagger Bookstore API, as highlighted in the above image is:
{
"books": [
{
"isbn": "9781449325862",
"title": "Git Pocket Guide",
"subTitle": "A Working Introduction",
"author": "Richard E. Silverman",
"published": "2013-08-02T00:00:00",
"publisher": "O'Reilly Media",
"pages": 234,
"description": "This pocket guide is the perfect on-the-job companion to Git, the distributed version control system. It provides a compact, readable introduction to Git for new users, as well as a reference to common commands and procedures for those of you with Git experience.",
"website": "https://chimera.labs.oreilly.com/books/1230000000561/index.html"
}
}
As a part of the next step, we will create a POJO class for our response object. Additionally, you may use the same online utility, as we discussed in the previous chapter, to convert the response to a POJO class.
To create a POJO class of it, follow the below steps:
1. Firstly, Right-click on the model Package under the apiEngine package. After that, select New>>Class. Name it as Book. Moreover, we will capture the book response under this class.
Book.class
package apiEngine.model;
public class Book {
public String isbn;
public String title;
public String subTitle;
public String author;
public String published;
public String publisher;
public int pages;
public String description;
public String website;
}
Code Explanation
This Book class will contain all the fields we got in the response, such as title, isbn, author, no.of pages, etc.
Create a POJO class for Books Response Object:
The response body from the Bookstore API, as highlighted in the below image is:
{
"userID": "9b5f49ab-eea9-45f4-9d66-bcf56a531b85",
"userName": "TOOLSQA-Test",
"books": [
{
"isbn": "9781449325862",
"title": "Git Pocket Guide",
"subTitle": "A Working Introduction",
"author": "Richard E. Silverman",
"published": "2013-08-02T00:00:00",
"publisher": "O'Reilly Media",
"pages": 234,
"description": "This pocket guide is the perfect on-the-job companion to Git, the distributed version control system. It provides a compact, readable introduction to Git for new users, as well as a reference to common commands and procedures for those of you with Git experience.",
"website": "https://chimera.labs.oreilly.com/books/1230000000561/index.html"
}
]
}
Note: As a part of the response body, we got the book details of the book we added for the user as well as other user details such as userName and userID.
To create a POJO class of it, follow the below steps:
-
Firstly, in this model Package, Right-click on the model and select New >> Package. Name it as responses. Additionally, we will capture all the response classes under this package.
-
Secondly, Right-click on the above-created responses Package and select New >> Class. Name it as Books.
Books.class
package apiEngine.model.responses;
import java.util.List;
import apiEngine.model.Book;
public class Books {
public List<Book> books;
}
Code Explanation We created the class Books to hold a list of the type Book which we receive in the JSON response in GET Books Request.
Create a POJO class for Token Object:
We have understood the process of creating a POJO class by now, as shown in the above examples. So, in the next APIs, I will directly add the POJO of respective API's. Consequently, the response body for Generate Token API from our Bookstore API is:
{
"token": "string",
"expires": "2020-03-14T13:42:15.716Z",
"status": "string",
"result": "string"
}
To create a POJO class of it, Right-click on the above-created responses Package and select New >> Class. After that, name it as Token.
package apiEngine.model.responses;
public class Token {
public String token;
public String expires;
public String status;
public String result;
}
Code Explanation
We created the class Token that will contain all the fields we got in the response field, namely, token, expiry, status, and a result which we receive in the JSON response of GenerateToken.
Create a POJO class for User Account Object:
Similarly, for User Account API, the response body is:
{
"userID": "9b5f49ab-eea9-45f4-9d66-bcf56a531b85",
"userName": "TOOLSQA-Test",
"books": []
}
To create a POJO class of the response body, Right-click on the above-created responses Package. After that, select New >> Class. Name it as UserAccount.
package apiEngine.model.responses;
import java.util.List;
import apiEngine.model.Book;
public class UserAccount {
public String userID;
public String userName;
public List<Book> books;
}
Code Explanation
We created the class UserAccount to hold the information associated with the user account, such as books associated with the user, the userID, and userName.
As our next step of project implementation, we shall modify our Steps class.
Replace the Response bodies in Step files with POJO class objects
Now it is the time to make use of the POJO classes created above in the test code. Let's have a look at the one of the old cucumber step below in the Step Definition:
@Given("^I am an authorized user$")
public void iAmAnAuthorizedUser() {
AuthorizationRequest credentials = new AuthorizationRequest("TOOLSQA-Test","Test@@123");
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
request.header("Content-Type", "application/json");
response = request.body(credentials).post("/Account/v1/GenerateToken");
String jsonString = response.asString();
//We will deserialize the Response body into Token Response
token = JsonPath.from(jsonString).get("token");
}
we update it likewise:
@Given("^I am an authorized user$")
public void iAmAnAuthorizedUser() {
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
request.header("Content-Type", "application/json");
AuthorizationRequest authRequest = new AuthorizationRequest("TOOLSQA-Test", "Test@@123");
response = request.body(authRequest).post("/Account/v1/GenerateToken");
// Deserializing the Response body into tokenResponse
tokenResponse = response.getBody().as(Token.class);
}
Note The import statement: import io.restassured.path.json.JsonPath; is no longer needed as we have deserialized our response to Token class.
Code Explanation: We deserialized the response body into the Token class in this step above. This response body gets saved in the "tokenResponse" variable. The saved variable will be used further in a request along with the token.
We put together these code snippets for our Steps file:
package stepDefinitions;
import java.util.List;
import java.util.Map;
import org.junit.Assert;
import apiEngine.model.Book;
import apiEngine.model.requests.AddBooksRequest;
import apiEngine.model.requests.AuthorizationRequest;
import apiEngine.model.requests.ISBN;
import apiEngine.model.requests.RemoveBookRequest;
import apiEngine.model.response.Books;
import apiEngine.model.response.Token;
import apiEngine.model.response.UserAccount;
import io.cucumber.java.en.Given;
import io.cucumber.java.en.Then;
import io.cucumber.java.en.When;
import io.restassured.RestAssured;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
public class Steps {
private static final String USER_ID = "9b5f49ab-eea9-45f4-9d66-bcf56a531b85";
private static final String BASE_URL = "https://bookstore.toolsqa.com";
private static Response response;
private static Token tokenResponse;
private static Book book;
@Given("I am an authorized user")
public void iAmAnAuthorizedUser() {
AuthorizationRequest credentials = new AuthorizationRequest("TOOLSQA-Test","Test@@123");
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
request.header("Content-Type", "application/json");
response = request.body(credentials).post("/Account/v1/GenerateToken");
// Deserializing the Response body into tokenResponse
tokenResponse = response.getBody().as(Token.class);
}
@Given("A list of books are available")
public void listOfBooksAreAvailable() {
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
response = request.get("/BookStore/v1/Books");
// Deserializing the Response body into Books class
Books books = response.getBody().as(Books.class);
book = books.books.get(0);
}
@When("I add a book to my reading list")
public void addBookInList() {
ISBN isbn = new ISBN(book.isbn);
AddBooksRequest addBooksRequest = new AddBooksRequest(USER_ID, isbn);
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
request.header("Authorization", "Bearer " + tokenResponse.token)
.header("Content-Type", "application/json");
response = request.body(addBooksRequest).post("/BookStore/v1/Books");
}
@Then("The book is added")
public void bookIsAdded() {
Assert.assertEquals(201, response.getStatusCode());
UserAccount userAccount = response.getBody().as(UserAccount.class);
Assert.assertEquals(USER_ID, userAccount.userID);
Assert.assertEquals(book.isbn, userAccount.books.get(0).isbn);
}
@When("I remove a book from my reading list")
public void removeBookFromList() {
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
RemoveBookRequest removeBookRequest = new RemoveBookRequest(USER_ID, book.isbn);
request.header("Authorization", "Bearer " + tokenResponse.token)
.header("Content-Type", "application/json");
response = request.body(removeBookRequest).delete("/BookStore/v1/Book");
}
@Then("The book is removed")
public void bookIsRemoved() {
Assert.assertEquals(204, response.getStatusCode());
RestAssured.baseURI = BASE_URL;
RequestSpecification request = RestAssured.given();
request.header("Authorization", "Bearer " + tokenResponse.token)
.header("Content-Type", "application/json");
response = request.get("/Account/v1/User/" + USER_ID);
Assert.assertEquals(200, response.getStatusCode());
UserAccount userAccount = response.getBody().as(UserAccount.class);
Assert.assertEquals(0, userAccount.books.size());
}
}
Run the Cucumber Test
Run the Tests as JUnit
We are all set now to run the updated Cucumber test. First, Right -Click on TestRunner class and Click Run As >> JUnit Test. Cucumber runs the script in the same way as Selenium WebDriver. Consequently, the result will display in the JUnit tab of the console.
Run the Tests from Cucumber Feature
To run the tests as a Cucumber Feature, right-click on the End2End_Test.feature file. After that, select the Run As>>Cucumber Feature.
Our tests passed with the changes we made for the conversion of the JSON Response Body to Java Object. Please try to implement it in your framework, as explained above, and share your valuable feedback.
After creating the above POJO classes, the project folder structure will look likewise:
Subsequently, we will attempt the separation of Test Layer with API Services in our next chapter.
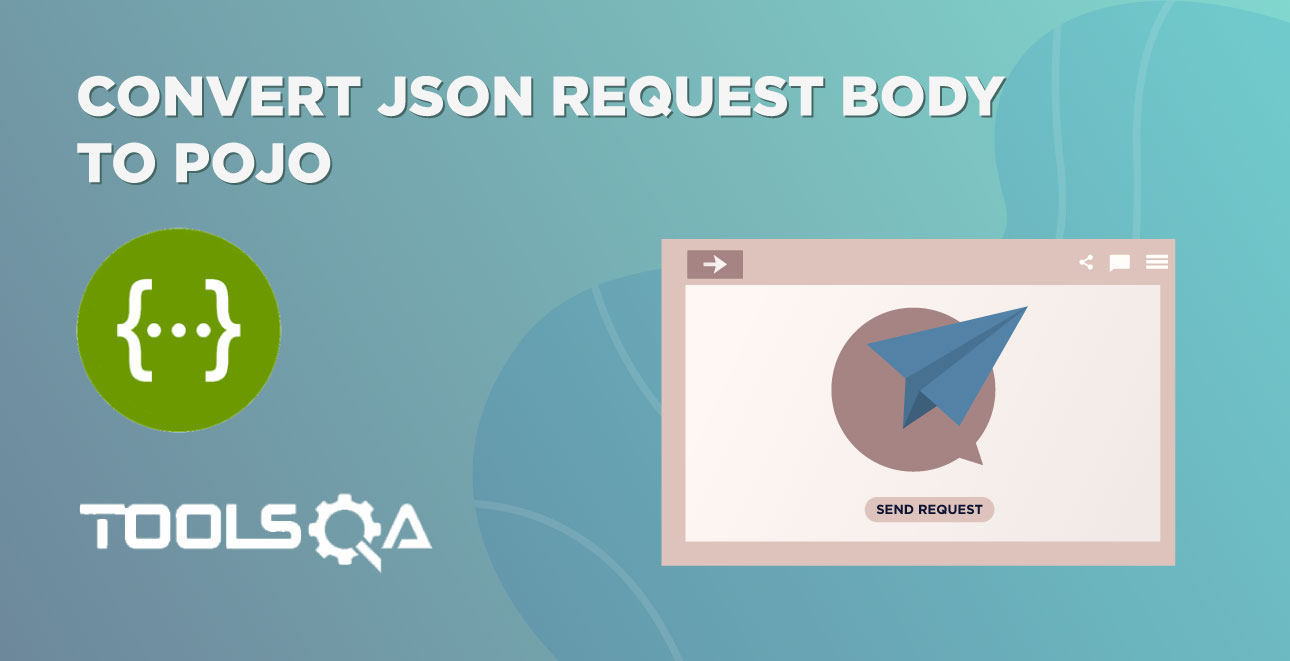
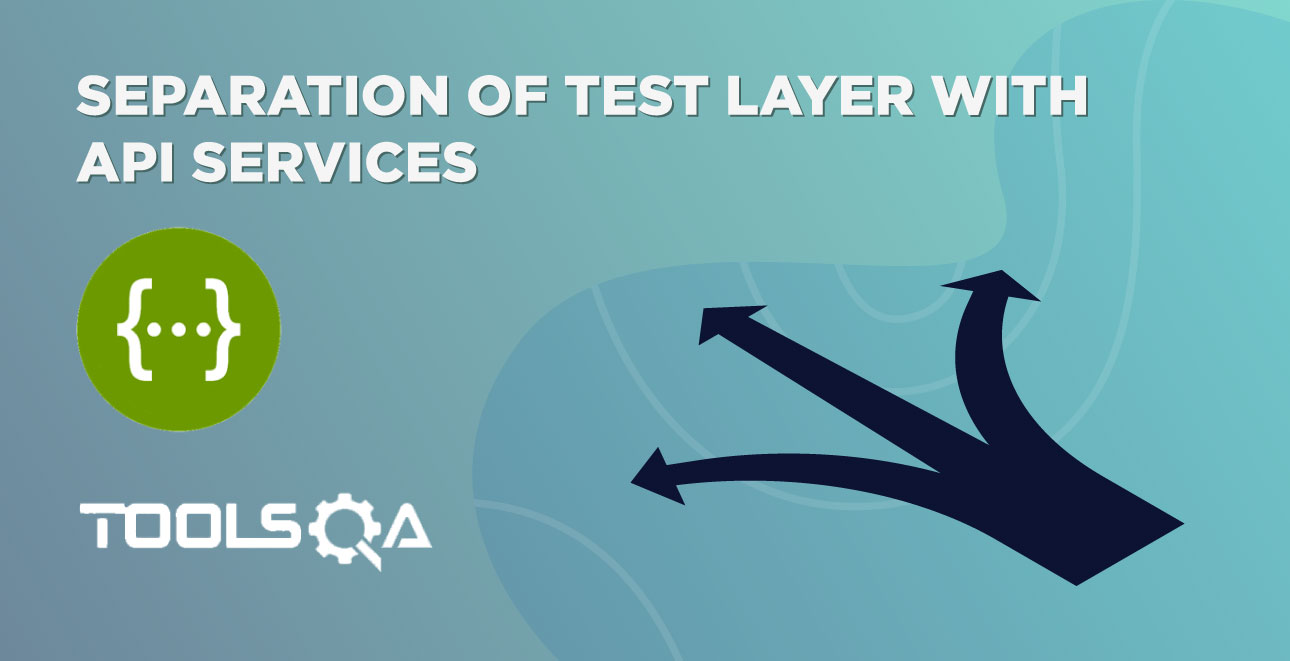