In the last two tutorials we have learnt about Response Status Code, Status line and Headers. We will continue with the same example in those tutorials and verify the body if the Response. If you have not gone through the first two tutorials then I would suggest you go through these links
- First Test with Rest-Assured
- Validate Response Status code and Status Line using Rest-Assured
- Validate Response Header using Rest-Assured
In this tutorial, we will learn about How to Read JSON Response Body using Rest Assured? and How to Validate Content of a Response Body?
Read JSON Response Body using Rest Assured
Let us continue with the example of Weather web service that we used in the previous tutorials. When we request for the Weather details of a particular city, Server responds by sending the Weather details of the city as the Response Body. Response interface contains two methods to get the Response Body
- Response.body() : returns ResponseBody
- Response.getBody() : returns ResponseBody
Using these methods we can get an Object of type io.restassured.response.ResponseBody. This class represents the Body of a received Response. Using this class you can get and validate complete or parts of the Response Body. In the below code we will simply read the complete Response Body by using Response.getBody() and will print it out on the console window.
@Test
public void WeatherMessageBody()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Retrieve the body of the Response
ResponseBody body = response.getBody();
// By using the ResponseBody.asString() method, we can convert the body
// into the string representation.
System.out.println("Response Body is: " + body.asString());
}
ResponseBody interface also has a method called .asString(), as used in the above code, which converts a ResponseBody into its String representation. If you run this test the output will look something like this:
Note: Response.body() method does exactly the same thing. So you can even use .body() method in the above code.
How to Validate Response Body contains some String?
ResponseBody can return the response body in a String format. We can use simple String methods to verify certain basic level of values in the Response. For e.g. we can use the String.contains() method to see if the Response contains a "Hyderabad" in it. The below code shows how to check for sub string presence.
@Test
public void WeatherMessageBody()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Retrieve the body of the Response
ResponseBody body = response.getBody();
// To check for sub string presence get the Response body as a String.
// Do a String.contains
String bodyAsString = body.asString();
Assert.assertEquals(bodyAsString.contains("Hyderabad") /*Expected value*/, true /*Actual Value*/, "Response body contains Hyderabad");
}
Check String presence by ignoring alphabet casing
We can also ignore the casing using the String internal methods. To do this we will convert the Response in lower case and then compare it with our lower case string value. Below code demonstrates that.
@Test
public void WeatherMessageBody()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Retrieve the body of the Response
ResponseBody body = response.getBody();
// To check for sub string presence get the Response body as a String.
// Do a String.contains
String bodyAsString = body.asString();
// convert the body into lower case and then do a comparison to ignore casing.
Assert.assertEquals(bodyAsString.toLowerCase().contains("hyderabad") /*Expected value*/, true /*Actual Value*/, "Response body contains Hyderabad");
}
The above two approaches suffer from a classical problem, what if the string "Hyderabad" is present in a wrong node or may be multiple instances of the same string are present. This is not a fool proof way of testing a particular node in the Response. There are better ways, Response interface gives you a mechanism to extract nodes based on a given JsonPath. There is a method called Response.JsonPath(), which returns a io.restassured.path.json.JsonPath Object. This object can be used to further query specific parts of the Response Json.
If you are not aware of JsonPath, please go through these tutorials
How to Extract a Node text from Response using JsonPath?
Let us continue with the above example and retrieve the City from the Response. To do so, we will simply get the JsonPath object from the Response interface and then query for the particular node. Just to be very clear, let us look at the Weather API response again.
{
"City": "Hyderabad",
"Temperature": "25.51 Degree celsius",
"Humidity": "94 Percent",
"Weather Description": "mist",
"Wind Speed": "1 Km per hour",
"Wind Direction degree": " Degree"
}
In this response, if we want to go to the City node, all we have to do is have the following JsonPath: $.City. Try it out on the JsonPath Evaluator to verify the output.
Now let us look at the code, pay specific attention to the comments in the code.
Note: In Java JsonPath you do not need to have $ as the root node. You can completely skip that.
@Test
public void VerifyCityInJsonResponse()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// First get the JsonPath object instance from the Response interface
JsonPath jsonPathEvaluator = response.jsonPath();
// Then simply query the JsonPath object to get a String value of the node
// specified by JsonPath: City (Note: You should not put $. in the Java code)
String city = jsonPathEvaluator.get("City");
// Let us print the city variable to see what we got
System.out.println("City received from Response " + city);
// Validate the response
Assert.assertEquals(city, "Hyderabad", "Correct city name received in the Response");
}
The output of the code passes the assertion and it also prints the City name retrieved from the Response. As shown in the image below
On the similar lines, you can extract any part of the Json response using the JsonPath implementation of Rest-Assured. This is very convenient, compact and easy way to write tests.
Sample Code to read all the nodes from Weather API Response
Now that we know how to read a node using JsonPath, here is a small piece of code that reads all the nodes and prints them to the Console.
@Test
public void DisplayAllNodesInWeatherAPI()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// First get the JsonPath object instance from the Response interface
JsonPath jsonPathEvaluator = response.jsonPath();
// Let us print the city variable to see what we got
System.out.println("City received from Response " + jsonPathEvaluator.get("City"));
// Print the temperature node
System.out.println("Temperature received from Response " + jsonPathEvaluator.get("Temperature"));
// Print the humidity node
System.out.println("Humidity received from Response " + jsonPathEvaluator.get("Humidity"));
// Print weather description
System.out.println("Weather description received from Response " + jsonPathEvaluator.get("Weather"));
// Print Wind Speed
System.out.println("City received from Response " + jsonPathEvaluator.get("WindSpeed"));
// Print Wind Direction Degree
System.out.println("City received from Response " + jsonPathEvaluator.get("WindDirectionDegree"));
}
In the next chapter, we will study about XmlPath usage on XML Responses.
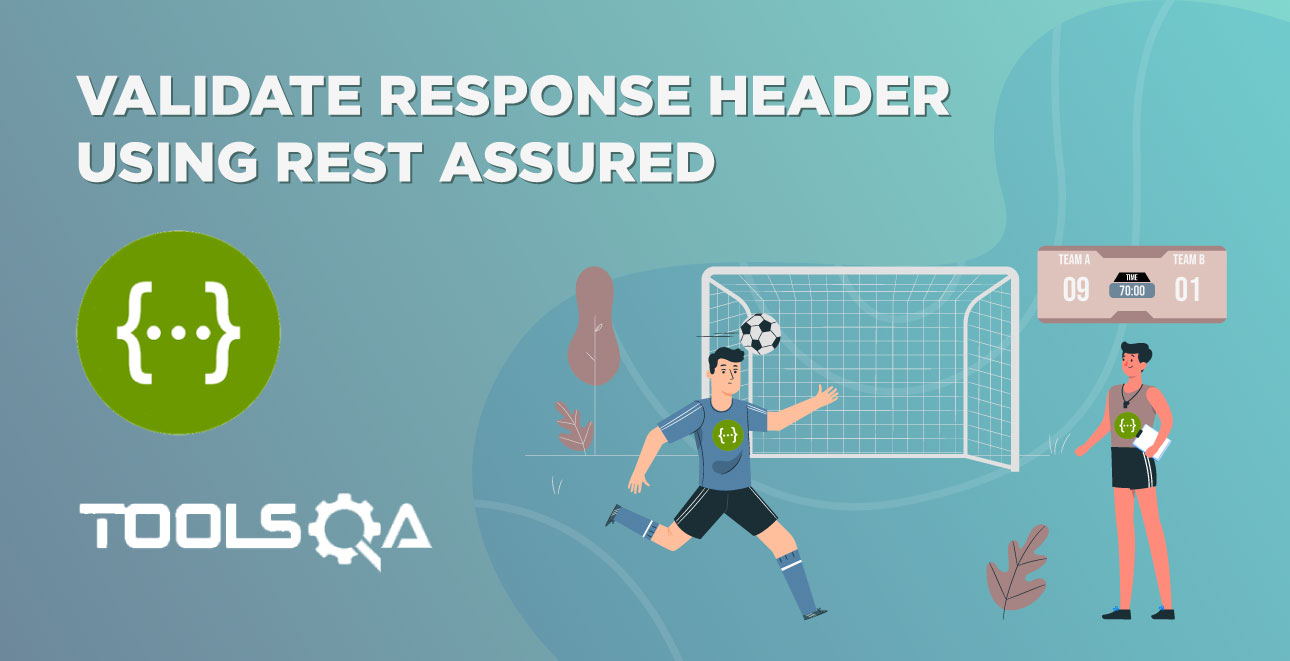
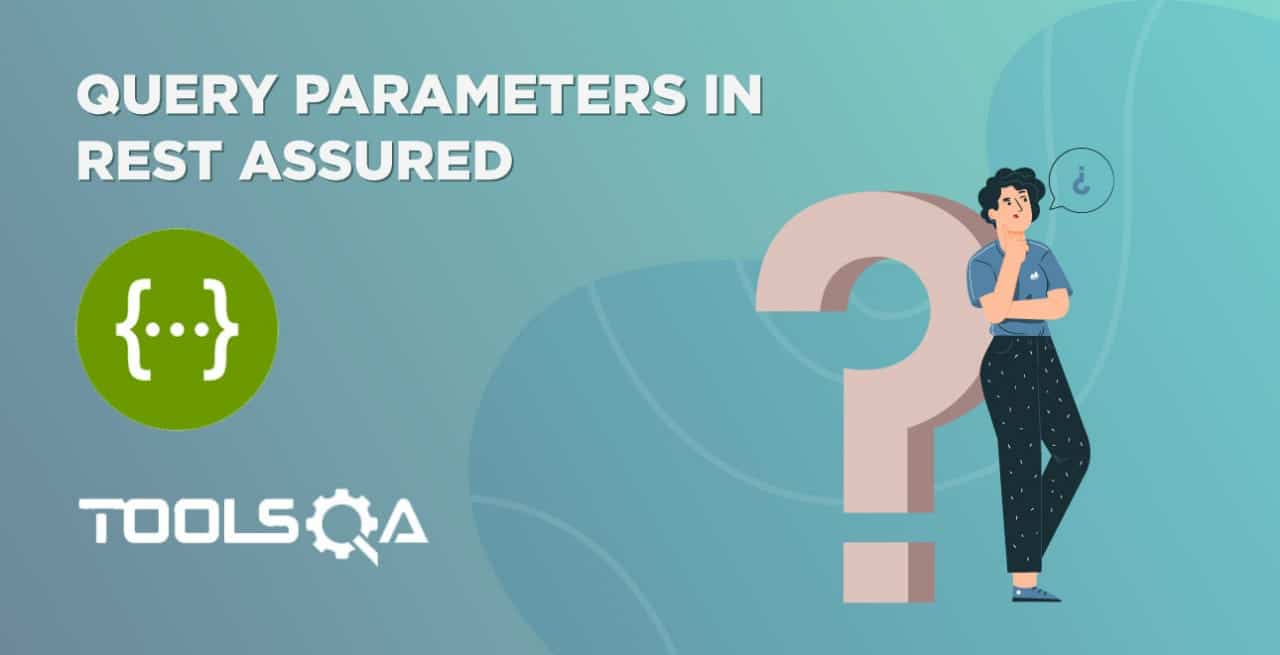