Let us learn How to Deserialize JSON Array to an Array? But before we start, it is very important that you go through the previous set of Tutorials. You can take a look at the tutorials here:
Deserialize JSON Array to an Array
This tutorial further builds upon our understanding of Deserialization of a JSON Response into an object of a given Type. Please go through the basics of Serialization and Deserialization in the below tutorial
We will take an example of demo REST API endpoint: https://bookstore.demoqa.com/swagger/#/BookStore/BookStoreV1BooksGet If you hit this Endpoint you will get a Collection of books in the Json response. Below is the response:
{
"books": [
{
"isbn": "9781593275846",
"title": "Eloquent JavaScript, Second Edition",
"subtitle": "A Modern Introduction to Programming",
"author": "Marijn Haverbeke",
"published": "2014-12-14T00:00:00.000Z",
"publisher": "No Starch Press",
"pages": 472,
"description": "JavaScript lies at the heart of almost every modern web application, from social apps to the newest browser-based games. Though simple for beginners to pick up and play with, JavaScript is a flexible, complex language that you can use to build full-scale applications.",
"website": "http:\/\/eloquentjavascript.net\/"
},
{
"isbn": "9781449331818",
"title": "Learning JavaScript Design Patterns",
"subtitle": "A JavaScript and jQuery Developer's Guide",
"author": "Addy Osmani",
"published": "2012-07-01T00:00:00.000Z",
"publisher": "O'Reilly Media",
"pages": 254,
"description": "With Learning JavaScript Design Patterns, you'll learn how to write beautiful, structured, and maintainable JavaScript by applying classical and modern design patterns to the language. If you want to keep your code efficient, more manageable, and up-to-date with the latest best practices, this book is for you.",
"website": "http:\/\/www.addyosmani.com\/resources\/essentialjsdesignpatterns\/book\/"
},
{
"isbn": "9781449365035",
"title": "Speaking JavaScript",
"subtitle": "An In-Depth Guide for Programmers",
"author": "Axel Rauschmayer",
"published": "2014-02-01T00:00:00.000Z",
"publisher": "O'Reilly Media",
"pages": 460,
"description": "Like it or not, JavaScript is everywhere these days-from browser to server to mobile-and now you, too, need to learn the language or dive deeper than you have. This concise book guides you into and through JavaScript, written by a veteran programmer who once found himself in the same position.",
"website": "http:\/\/speakingjs.com\/"
},
{
"isbn": "9781491950296",
"title": "Programming JavaScript Applications",
"subtitle": "Robust Web Architecture with Node, HTML5, and Modern JS Libraries",
"author": "Eric Elliott",
"published": "2014-07-01T00:00:00.000Z",
"publisher": "O'Reilly Media",
"pages": 254,
"description": "Take advantage of JavaScript's power to build robust web-scale or enterprise applications that are easy to extend and maintain. By applying the design patterns outlined in this practical book, experienced JavaScript developers will learn how to write flexible and resilient code that's easier-yes, easier-to work with as your code base grows.",
"website": "http:\/\/chimera.labs.oreilly.com\/books\/1234000000262\/index.html"
},
{
"isbn": "9781593277574",
"title": "Understanding ECMAScript 6",
"subtitle": "The Definitive Guide for JavaScript Developers",
"author": "Nicholas C. Zakas",
"published": "2016-09-03T00:00:00.000Z",
"publisher": "No Starch Press",
"pages": 352,
"description": "ECMAScript 6 represents the biggest update to the core of JavaScript in the history of the language. In Understanding ECMAScript 6, expert developer Nicholas C. Zakas provides a complete guide to the object types, syntax, and other exciting changes that ECMAScript 6 brings to JavaScript.",
"website": "https:\/\/leanpub.com\/understandinges6\/read"
},
{
"isbn": "9781491904244",
"title": "You Don't Know JS",
"subtitle": "ES6 & Beyond",
"author": "Kyle Simpson",
"published": "2015-12-27T00:00:00.000Z",
"publisher": "O'Reilly Media",
"pages": 278,
"description": "No matter how much experience you have with JavaScript, odds are you don\u2019t fully understand the language. As part of the 'You Don\u2019t Know JS' series, this compact guide focuses on new features available in ECMAScript 6 (ES6), the latest version of the standard upon which JavaScript is built.",
"website": "https:\/\/github.com\/getify\/You-Dont-Know-JS\/tree\/master\/es6%20&%20beyond"
},
{
"isbn": "9781449325862",
"title": "Git Pocket Guide",
"subtitle": "A Working Introduction",
"author": "Richard E. Silverman",
"published": "2013-08-02T00:00:00.000Z",
"publisher": "O'Reilly Media",
"pages": 234,
"description": "This pocket guide is the perfect on-the-job companion to Git, the distributed version control system. It provides a compact, readable introduction to Git for new users, as well as a reference to common commands and procedures for those of you with Git experience.",
"website": "http:\/\/chimera.labs.oreilly.com\/books\/1230000000561\/index.html"
},
{
"isbn": "9781449337711",
"title": "Designing Evolvable Web APIs with ASP.NET",
"subtitle": "Harnessing the Power of the Web",
"author": "Glenn Block, et al.",
"published": "2014-04-07T00:00:00.000Z",
"publisher": "O'Reilly Media",
"pages": 538,
"description": "Design and build Web APIs for a broad range of clients\u2014including browsers and mobile devices\u2014that can adapt to change over time. This practical, hands-on guide takes you through the theory and tools you need to build evolvable HTTP services with Microsoft\u2019s ASP.NET Web API framework. In the process, you\u2019ll learn how design and implement a real-world Web API.",
"website": "http:\/\/chimera.labs.oreilly.com\/books\/1234000001708\/index.html"
}
]
}
This JSON Response contains an Array of Books. Each item in the JSON Array represents a book and has properties of a book like "isbn", "title", "author" etc. In the earlier tutorial, we learnt How to Deserialize JSON Resposne of Single Node to an Instance of Class. However, in Deserializing a Collection of Nodes into Array becomes little tricky. Let us see how Rest Assured helps us achieve this quickly and without writing any boilerplate code.
Deserialize JSON Array to an Array using JSONPath of Rest Assured?
Similarly, we can convert a Json Array into a Java Array. JsonPath class has method called getObject. This method can be used to convert the response directly into a Java Array of Book. The only thing the we need to do is pass Book[].class as the second argument to the method to signify that we want the Json to be deserialized into an Array of Book. Here is the code that will do this
@Test
public void JsonArrayToArray()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/books/getallbooks";
RequestSpecification request = RestAssured.given();
Response response = request.get();
System.out.println("Response Body -> " + response.body().asString());
// We can convert the Json Response directly into a Java Array by using
// JsonPath.getObject method. Here we have to specify that we want to
// deserialize the Json into an Array of Book. This can be done by specifying
// Book[].class as the second argument to the getObject method.
Book[] books = response.jsonPath().getObject("books",Book[].class );
for(Book book : books)
{
System.out.println("Book title " + book.title);
}
}
The above two techniques are important to write Concise test code. Apart from writing concise tests by using the above techniques we fully utilize the features given to us by Rest-Assured. This helps us have lesser dependencies outside Rest Assured and also enables us to write reliable tests.
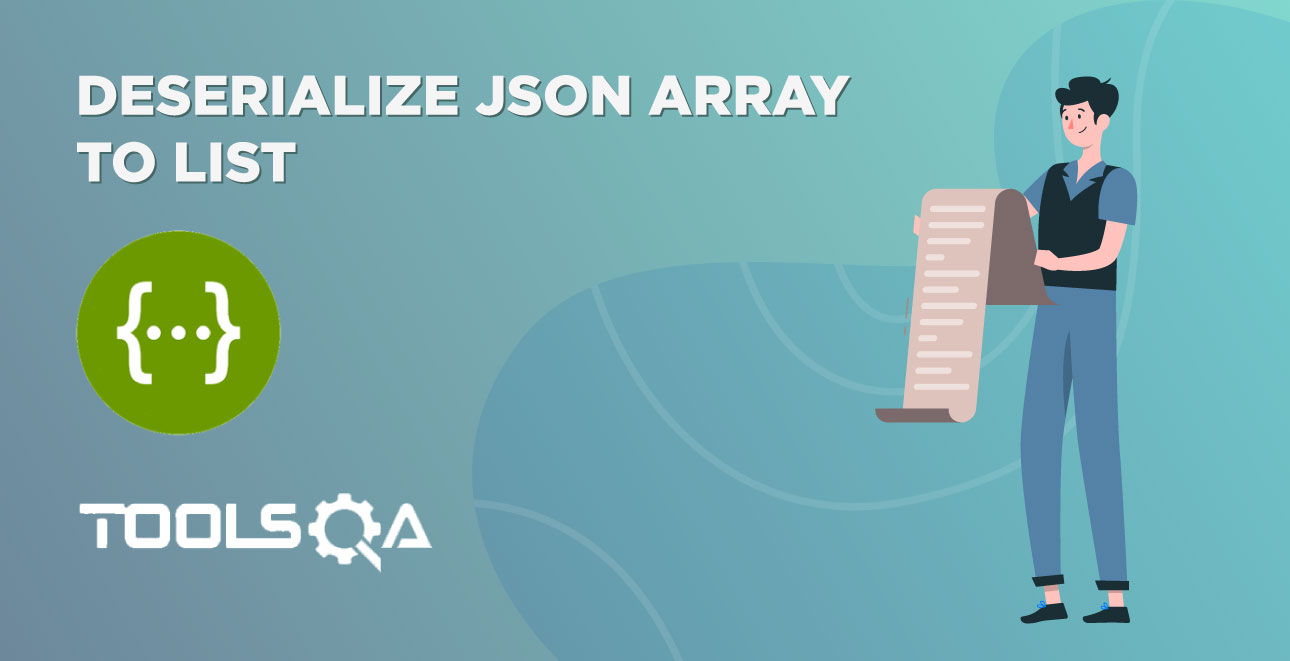
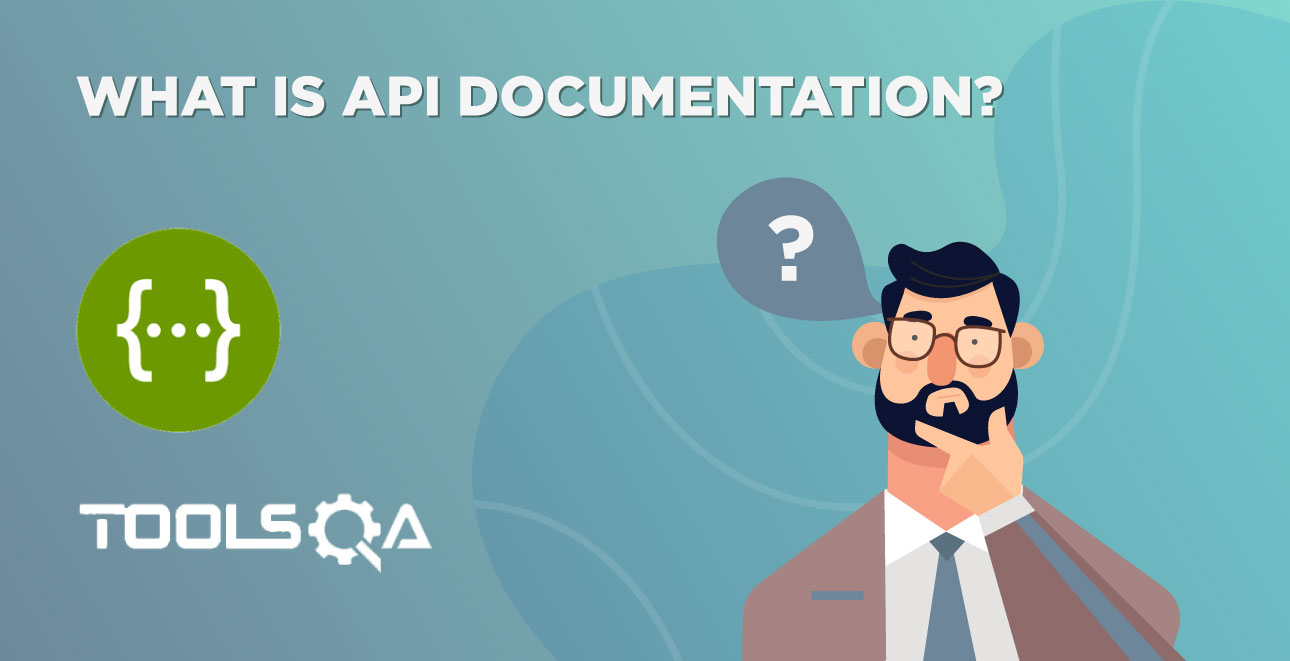