So far in this series, we have covered reading the response body. But as you proceed ahead, a simple GET or a POST request won't suffice to the complexity your test scenario might have. You may need to test the API for different query parameters. In this article, we will cover how query parameters are passed in the HTTP request using rest assured with the following main sections-
- What is the composition of the URL?
- What are Query Parameters?
- How to send a request using Query Parameters in Rest Assured?
What is the composition of the URL?
Similar to the examples used in the previous articles we will be using the Bookstore API in this article as well. Consider the below sample URL to fetch details corresponding to a book-
https://demoqa.com/BookStore/v1/Book?ISBN=9781449325862 Though the above URL looks very basic, it still let us understand its different components.
- https:// - It is a protocol that ensures secure connection between the web server and the web browser.
- https://demoqa.com/swagger/#/BookStore - It is the domain name that hosts the website. It generally ends with .com, .in, .net, etc.
- BookStore/v1/Book - This is the path or the URI that identifies the resource applied by the request.
- ?ISBN=9781449325862 - This is a string query parameter. The question mark denotes the start of a query string parameter. There can be one or more query parameters in the URL.
Let us now understand more about query parameters in the next section.
What are Query String Parameters?
You might not always want to fetch all the results corresponding to a request. There may be scenarios where you need to fetch only a few or a single record. In such cases, query string parameters play an important role. These are appended at the end of the URL after using a '?'. Try entering the example URL in the browser address bar and observe the results available under Network -> Payload-
The query parameter, i.e., ISBN=9781449325862, is displayed under the Query String Parameters field. In a similar way, if there are multiple query parameters passed in the URL, you will see all of them in this field. As an exercise, you may search some keywords on Google search and see the query parameters that get appended to the URL. Observe the search keyword is displayed after 'q=your search keyword'. Additionally, you will observe other query parameters which are separated from each other through an '&' sign.
These signs are termed URL parameters and are beyond the scope of our discussion here.
Now that we have understood what query parameters are and how they are used in requests, we can proceed to see how we can send requests with Query Parameters in Rest Assured.
How to send a request using Query Parameters in Rest Assured?
In this section, we will see how we can automate our test case by passing query parameters in Rest assured. We will simply use the URL containing the query parameter as discussed in sections above to show how we can send a request using query parameters in rest assured. Let us see how the code would look like and then we will walkthrough it step by step.
package bookstore;
import org.junit.Test;
import io.restassured.RestAssured;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class QueryParam {
@Test
public void queryParameter() {
//Defining the base URI
RestAssured.baseURI= "https://bookstore.toolsqa.com/BookStore/v1";
RequestSpecification httpRequest = RestAssured.given();
//Passing the resource details
Response res = httpRequest.queryParam("ISBN","9781449325862").get("/Book");
//Retrieving the response body using getBody() method
ResponseBody body = res.body();
//Converting the response body to string object
String rbdy = body.asString();
//Creating object of JsonPath and passing the string response body as parameter
JsonPath jpath = new JsonPath(rbdy);
//Storing publisher name in a string variable
String title = jpath.getString("title");
System.out.println("The book title is - "+title);
}
}
RestAssured.baseURI= "https://bookstore.toolsqa.com/BookStore/v1";
RequestSpecification httpRequest = RestAssured.given();
We first define the base URI to create a request to the service endpoint.
Response res = httpRequest.queryParam("ISBN","9781449325862").get("/Book");
Next we send the resource details like the book ISBN as a query parameter to search in books using the GET request.
Note: If you need to send multiple query parameters you simply need to append queryParam() method with the parameter name and value to the RequestSpecification object, i.e. httpRequest in the above case.
ResponseBody body = res.body();
String rbdy = body.asString();
JsonPath jpath = new JsonPath(rbdy);
String title = jpath.getString("title");
System.out.println("The book title is - "+title);
Finally, we are storing the response body as a String object and parsing its different values using the JSONPath object. We then fetch the title from the response body.
Note: You may read more about JSONPath in our elaborate article on the same.
And that's it! See how easily we could send the query parameters to our request by simply using a queryParam() method. The above code execution displays the title of the book with respect to the ISBN.
You can now go ahead with automating your test scripts with request having query parameter(s) and enhance your rest assured code.
Key Takeaways
- We have seen the different components of a URL and the purpose of each component.
- We also understood what are query parameters and how they work when we access a URL in the browser.
- Query parameters passed to the rest assured tests using the queryParam() method accepts the parameter name and value.
- You may use the queryParam() method not just once, but as many times as the number of query parameters in your GET request.
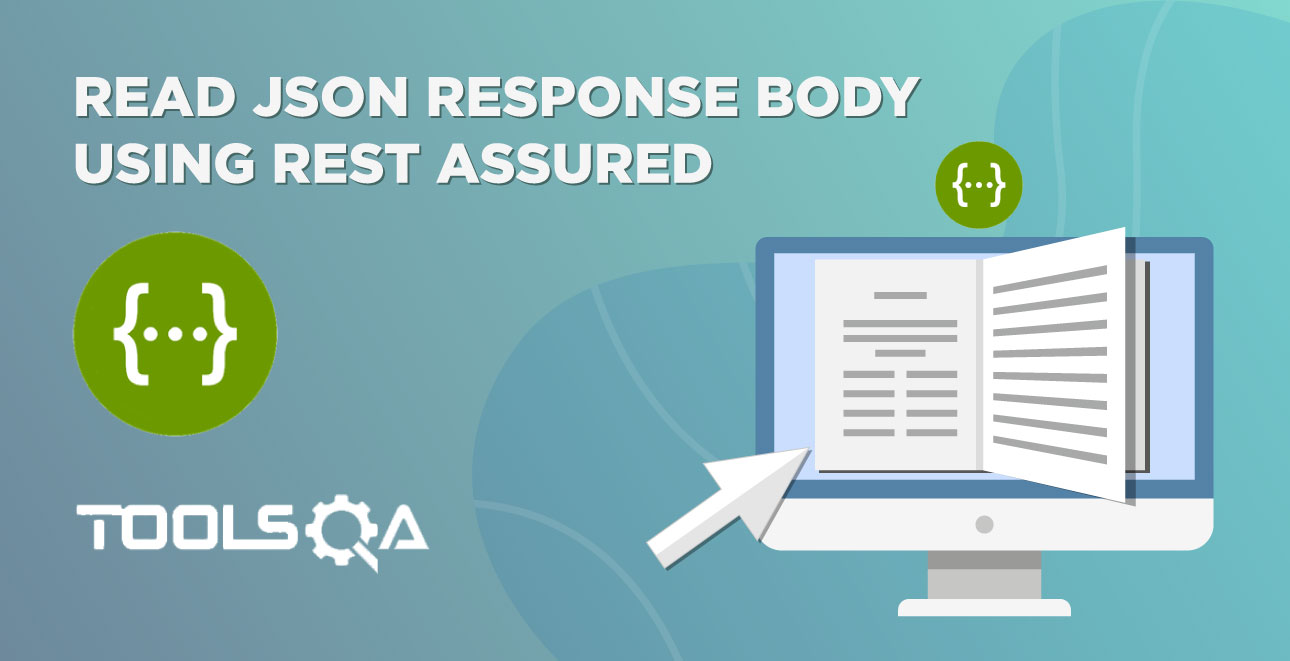
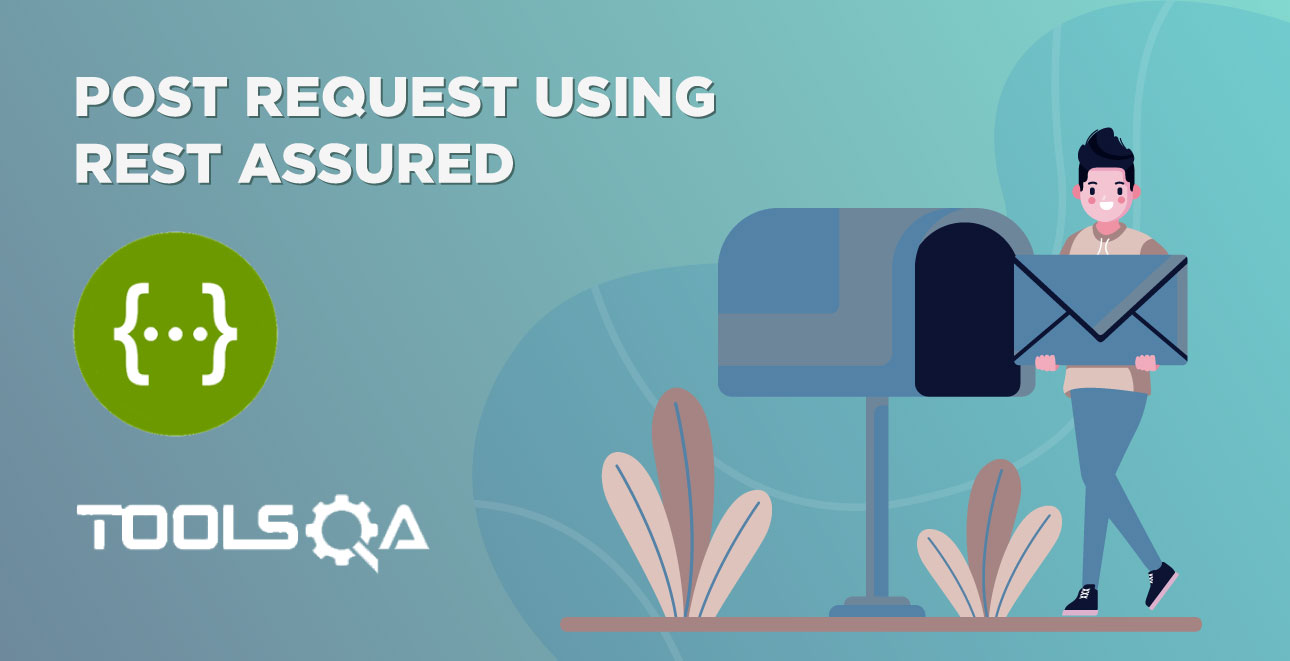