In earlier articles, we have seen how to read various components of an *HTTP Response (Headers,Body, and Status) and to Post a request* using Rest Assured. In this article, we will continue our exploration of HTTP request methods and move on to the next method - the PUT request method using REST Assured.
- What is an HTTP PUT Request Method?
- PUT Vs. POST Request.
- Status codes were obtained for PUT and POST requests.
- REST API example.
- How to implement a PUT Request using Rest Assured?
- Create JSON data using Simple JSON library.
- Send JSON content in the body of the Request.
- Validate the Response.
What is an HTTP PUT Request Method?
The *PUT method *(HTTP PUT request method) creates a new resource or updates (substitutes) a representation of the target resource with the request payload. This means a Put request updates a resource at a specified URI. It is also used to create a new resource at the given URI or replace the entire product entity.
The official HTTP RFC specifies:
- A PUT method puts or places a file or resource precisely at a specific URI.
- In case a file or a resource already exists at that URI, the PUT method replaces that file or resource.
- If there is no file or resource, PUT creates a new one.
- Responses to the PUT method are non-cacheable.
- PUT requests usually respond back with status code 200.
PUT Vs. POST Request
Let us now discuss the main differences between a PUT and a POST request.
PUT | POST |
---|---|
This method is idempotent. This means it will produce the same results if executed more than once. | This method is not idempotent. It produces different results every time it is executed. |
When we need to modify a single resource that is already part of resource collection, we call the PUT method. | POST method is called when a child resource is to be added under resources collection. |
PUT method syntax : PUT /questions/{question-id} | POST method syntax: POST /questions |
PUT works as specific. | POST works as abstract. |
Put method makes use of the "UPDATE" query. | POST method makes use of the "CREATE" query. |
In the PUT method, the client decides which URI resource should have. | In the POST method, the server decides which URI resource should have. |
*PUT /vi/cake/orders/1234 indicates updation of a resource identified by "1234". | POST /vi/cake/orders* indicate that we are creating a new resource and return an identifier to describe the resource. |
Status codes obtained for PUT and POST requests
The methods POST and PUT use the following status codes:
POST request
- 201 with a location header pointing to the new resource.
- 400 if the new item is not created.
PUT request
- 204 for OK/SUCCESS (but no content).
- 200 for OK with Content Body (Updated response).
- 400 if the data sent was invalid.
REST API example
Now we'll demonstrate the PUT request using Swagger UI. The URL for the PUT request is given here https://demoqa.com/BookStore/v1/Books/
Also, you can take a look at the Book Store Swagger
When we access this URL, we get the following screen.
In the above screen, we a trying to update a book record with ISBN="9781449325862". The two parameters that we will update are userId: "toolsqa_test" and ISBN: "9781449325865". When we execute this put request we get the following output.
We can see the status code 200 which we received from the server indicating the request was successful.
In the next section, we will implement the PUT request using Rest Assured.
How to implement a PUT Request using Rest Assured?
As explained in the tutorial on a *POST request, to create JSON objects, we will add a Simple JSON* library in the classpath in the code. Once this is done, we follow the below-given steps to put a request using REST Assured.
- Create JSON data using a simple JSON library.
- Send JSON content in the body of the request.
- Validate the response.
Let us discuss each of these steps below.
Create JSON data using Simple JSON library
We will use the same URL for implementing the PUT request using Rest Assured.
Endpoint: https://demoqa.com/BookStore/v1/Books/
The first step is to create a JSON data request that we need to send using the "put()" method. The following piece of code achieves this.
java import io.restassured.RestAssured; import io.restassured.specification.RequestSpecification; import org.junit.Test;
public class PUTMethod { @Test public void putMethod() {
String userId = "toolsqa_test";
String baseUrl = "https://demoqa.com";
String token = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyTmFtZSI6InRlc3RpbmcxMjMiLCJwYXNzd29yZCI6IlBhc3N3b3JkQDEiLCJpYXQiOjE2Mjg1NjQyMjF9.lW8JJvJF7jKebbqPiHOBGtCAus8D9Nv1BK6IoIIMJQ4";
String isbn = "9781449325865";
RequestSpecification httpRequest = RestAssured.given().header("Authorization", "Bearer " + token).header("Content-Type", "application/json");
}
}
In the above code, we have used an ISBN parameter. Note that we already have a record with this ISBN. We will update this record by sending the PUT request. So for this ISBN, we will update the record with a new ISBN and a userId. We set a new value for the ISBN and also updated userId. So when we send this request, the record having ISBN="9781449325862" will have its userId="toolsqa_test" and ISBN updated to the new value, "9781449325865".
Send JSON content in the body of the Request
The generated request is sent to the server.
java Response res = httpRequest.body("{ "isbn": "" + isbn + "", "userId": "" + userId + ""}")
.put("/BookStore/v1/Book/9781449325862");
So in the above code, we have created a request body as a JSON string and then we call the "put()" method with this request by sending the ISBN as an argument. This ensures the record with the given ISBN is updated.
Validate the Response
The next step is to validate the response to ensure record updation. This is done by reading the status code of the response as follows:
java //validate the response System.out.println("The response code - " +res.getStatusCode());
Assert.assertEquals(res.getStatusCode(),200);
We have used the getStatusCode () method that returns the status code of the response. The value 200 indicates the PUT request was successful. The execution of the above tests gives the following response:
We see that the test case has passed.
The complete code for the PUT request demonstration using Rest Assured is as follows:
java package org.example;
import io.restassured.RestAssured; import io.restassured.specification.RequestSpecification; import org.junit.Assert; import org.junit.Test; import io.restassured.response.Response; import io.restassured.response.ResponseBody; import io.restassured.specification.RequestSpecification; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest;
public class PUTMethod { String userId= "toolsqa_test"; String baseUrl="https://demoqa.com"; String token = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyTmFtZSI6InRlc3RpbmcxMjMiLCJwYXNzd29yZCI6IlBhc3N3b3JkQDEiLCJpYXQiOjE2Mjg1NjQyMjF9.lW8JJvJF7jKebbqPiHOBGtCAus8D9Nv1BK6IoIIMJQ4"; String isbn ="9781449325865";
@Test
public void updateBook() {
RestAssured.baseURI = baseUrl;
RequestSpecification httpRequest = RestAssured.given().header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json");
//Calling the Delete API with request body
Response res = httpRequest.body("{ \"isbn\": \"" + isbn + "\", \"userId\": \"" + userId + "\"}").put("/BookStore/v1/Book/9781449325862");
//Fetching the response code from the request and validating the same
System.out.println("The response code - " +res.getStatusCode());
Assert.assertEquals(res.getStatusCode(),200);
}
}
The steps discussed above demonstrate the basic working of a PUT request and how it differs from a POST request. We used the JSON library to create JSON data sent in the content of the body of the request. We then validate the response using the status code after obtaining the response.
Note: Video tutorial for Put request is available at Put Request in Rest Assured
Key TakeAways
In this article, we discussed the PUT request.
- Put request is idempotent i.e. it produces the same results irrespective of the number of times the request is executed.
- The HTTP Put request updates or creates a resource.
- Put request usually responds with status code 200 when it is successful. The responses to the PUT request are not cacheable.
- We can use the Rest Assured library to put a request (using the put method). For this, we send a JSON object with the request and obtain a response.
- We validate the response by checking the status code.
In the next article of the Rest Assured series, we will discuss the "DELETE" method.
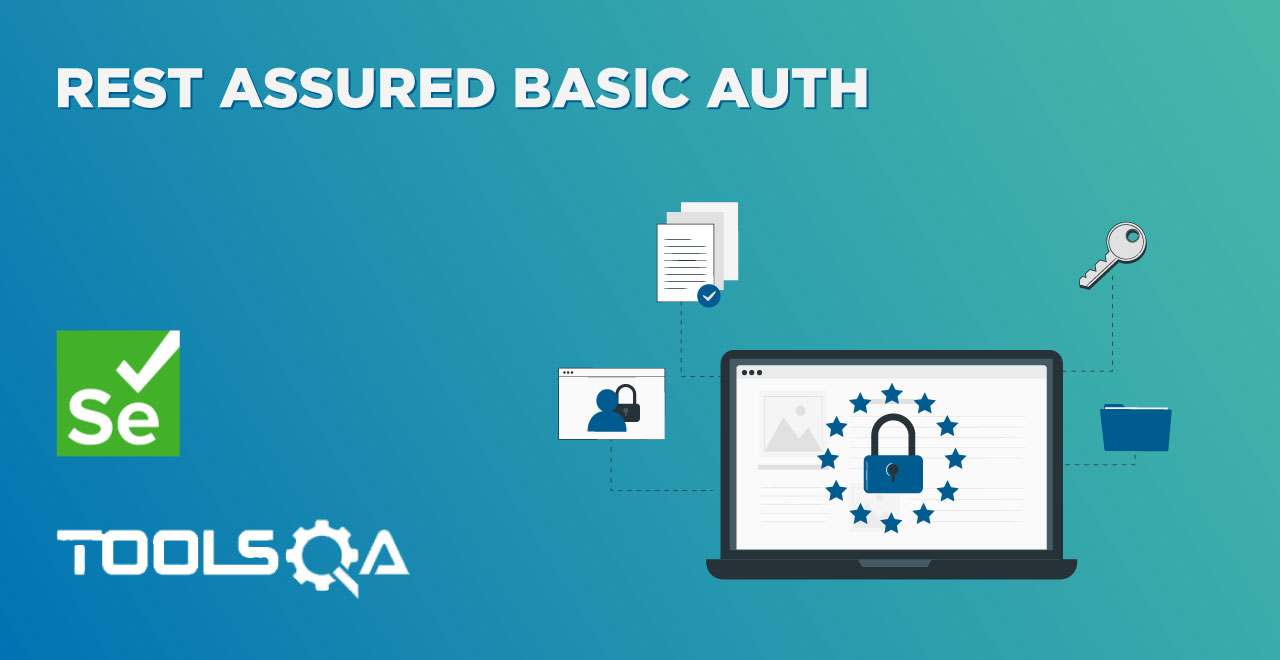
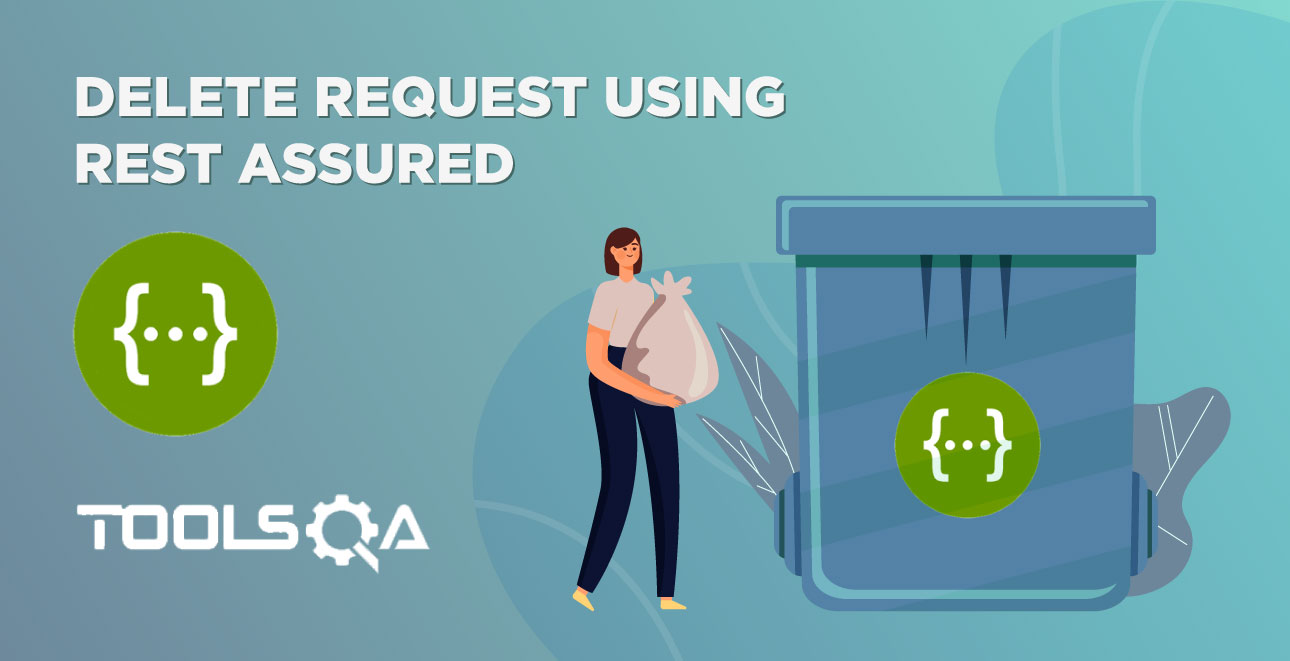