APIs form the base for any application and in every application, you perform a lot of different actions. These actions may range from adding, viewing, editing or deleting data. Since we have so many actions, we have to have a variety of tests to test the correctness of these actions. In our previous post, we learnt how to perform PUT requests using Rest Assured, and in this post, we will learn about the HTTP DELETE request method using the same method. If you have not gone through our previous articles I would urge you to go through our Rest Assured series step-by-step to understand the basics.
In this article, we will cover the below point-
- What is a Delete request?
- What are the different response codes for Delete Request?
- How to make a Delete Request using Rest Assured?
What is a delete request method?
An HTTP delete request is performed using the HTTP delete method (written as delete) which deletes a resource from the server identified by the URI we are sending through it. Also note that a delete method intends to change the state of the server. Although even a 200 code does not guarantee this. A few major points as highlighted in the official HTTP RFC with respect to the delete request method are listed below-
- The Delete method requests the server to delete the resource identified by the request URI.
- The resource deletion depends on the server and is deleted if prescribed for deletion. Additionally, the restoration implementation of the resource is also considerable.
- There is a deletion request for association between the resource and corresponding current functionality.
- It is similar to the rm UNIX command, where the current association of the resource is deleted instead of the previous ones(if any).
- Delete request method is placed under the idempotent category of the W3C documentation. It means that once a resource is requested for deletion, a request on the same resource again would give the same result as the resource has already been deleted.
- Delete method response is non-cacheable. The user cannot cache the server response for later use. Caching a delete request creates inconsistencies.
Let's see the response code of Delete request now.
What are the different response codes for Delete Request?
On sending the Delete request, the server responds with some response codes which signify the action performed by the Delete method. Consider the following code for the same-
- 202(Accepted): The server accepts the request but does not enact.
- 204(No Content)- A status code of 204 on the HTTP delete request method denotes successful enactment of the delete request without any content in the response.
- 200(OK)- The action was successful and the response message includes representation with the status.
- 404(Not Found) - When the server can't find the resource. The reason could either does not exist or previously deleted.
Now that we understand the basics of the Delete method we will see how we can send a Delete request using rest assured but before that let us see a simple DELETE request using Swagger UI. The URL for the request is-
The URL will show a screen like below-
Now you will have to select the first "Delete" in the BookStore section from this page.
Note: We have disabled the UserID to prevent an empty database. In practical application, you have to pass the required values to the server.
When you will click on the section, you will see a response depicting that the execution was a success. A specific response is received for cases like "bookid not found" or "user not found". See the snapshot below for more clarity-
In the introduction, we defined the delete method as something that can delete a resource. As a reminder, you can relate the "resource" with the pet record here. A generic term "resource" denotes varying resources in various requests. Now that we have seen how we may go about manually deleting a resource using swagger next we will try to automate the Delete request using Rest Assured.
How to make a Delete Request using Rest Assured?
We will now see how we can delete a resource from the server using rest assured. To do so, we will use BookStore APIs which we have been using in our previous examples. We will be automating the following use case-
- Get a list of available books for to a user using the Get User Data API.
- Delete a book corresponding to a specific ISBN and validate the response code using the Delete Book API.
- Verify that the execution of API from step 1 updates the book list and does not display the deleted book.
Let us now see the code for the above use case using Rest Assured.
package bookstore;
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class DeleteBook {
String userId= "de5d75d1-59b4-487e-b632-f18bc0665c0d";
String baseUrl="https://demoqa.com/swagger/";
String token = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyTmFtZSI6InRlc3RpbmcxMjMiLCJwYXNzd29yZCI6IlBhc3N3b3JkQDEiLCJpYXQiOjE2Mjg1NjQyMjF9.lW8JJvJF7jKebbqPiHOBGtCAus8D9Nv1BK6IoIIMJQ4";
String isbn ="9781449337711";
@BeforeTest
@AfterTest
public void getUserData() {
RestAssured.baseURI = baseUrl;
RequestSpecification httpRequest =
RestAssured.given().header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json");
Response res = httpRequest.get("/Account/v1/User/"+userId);
ResponseBody body = res.body();
//Converting the response body to string
String rbdy = body.asString();
System.out.println("Data from the GET API- "+rbdy);
}
@Test
public void deleteBook() {
RestAssured.baseURI = baseUrl;
RequestSpecification httpRequest = RestAssured.given().header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json");
//Calling the Delete API with request body
Response res = httpRequest.body("{ \"isbn\": \"" + isbn + "\", \"userId\": \"" + userId + "\"}").delete("/BookStore/v1/Book");
//Fetching the response code from the request and validating the same
System.out.println("The response code is - " +res.getStatusCode());
Assert.assertEquals(res.getStatusCode(),204);
}
}
Code walkthrough
Let's walk through the above code and see what we tried to achieve here. Note that the first method, i.e getUserData() is a simple GET method where we are trying to fetch the user data corresponding to a userId. You may refer to our article on Rest API using Rest Assured to understand the code if you have not gone through it yet. Additionally, you will notice the authorization field. For this particular tutorial on the delete request method, you need not worry about it. However, if you want to learn about authorization and OAuth 2.0, you can refer to our other tutorials. In this tutorial about the delete method, we will go through the code written under deleteBook() method here.
RestAssured.baseURI = baseUrl;
RequestSpecification httpRequest = RestAssured.given().header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json");
We first set up the request with the base URI. Next, we add the header(s) which also include the Authorization token using the RequestSpecification interface.
Response res = httpRequest.body("{ \"isbn\": \"" + isbn + "\", \"userId\": \"" + userId + "\"}").delete("/BookStore/v1/Book");
Next, store the Response object using the RequestSpecification object. Here we are passing the JSON body of our request along with the endpoint URL for the Delete method. The response gets store in a variable.
System.out.println("The response code is - " +res.getStatusCode());
Assert.assertEquals(res.getStatusCode(),204);
Lastly, we are simply printing the response status code and using the TestNG Assert to validate the same. Since in our case the expected response code is 204, our test would have it as the expected parameter.
Once you execute the code you will see that the getUserData method executes first, followed by the deleteBook method. And then finally the getUserData method runs again. This is because we used the @BeforeTest and @AfterTest for the getUserData method. The console would show you the result as below-
And there you go! You have successfully deleted a record from the user data using the Delete API. Now you may go ahead and try out the different API methods using Rest Assured and strengthen your concepts. In our next post, we will see how Basic Auth handles Rest Assured.
Note: Video tutorial for Delete request is available at Delete Request in Rest Assured
Key Takeaways
- The "delete" method deletes a resource from the server. It is quite similar to the rm UNIX command.
- The deletion of a resource is based on the server implementation and the response received is non-cacheable.
- The delete request returns any of the three types of response codes, i.e., 202, 204, and 200.
- The delete() method used with "path" or "pathParams" deletes the request.
- To verify the deletion, you may use assertion or any other relevant code as required.
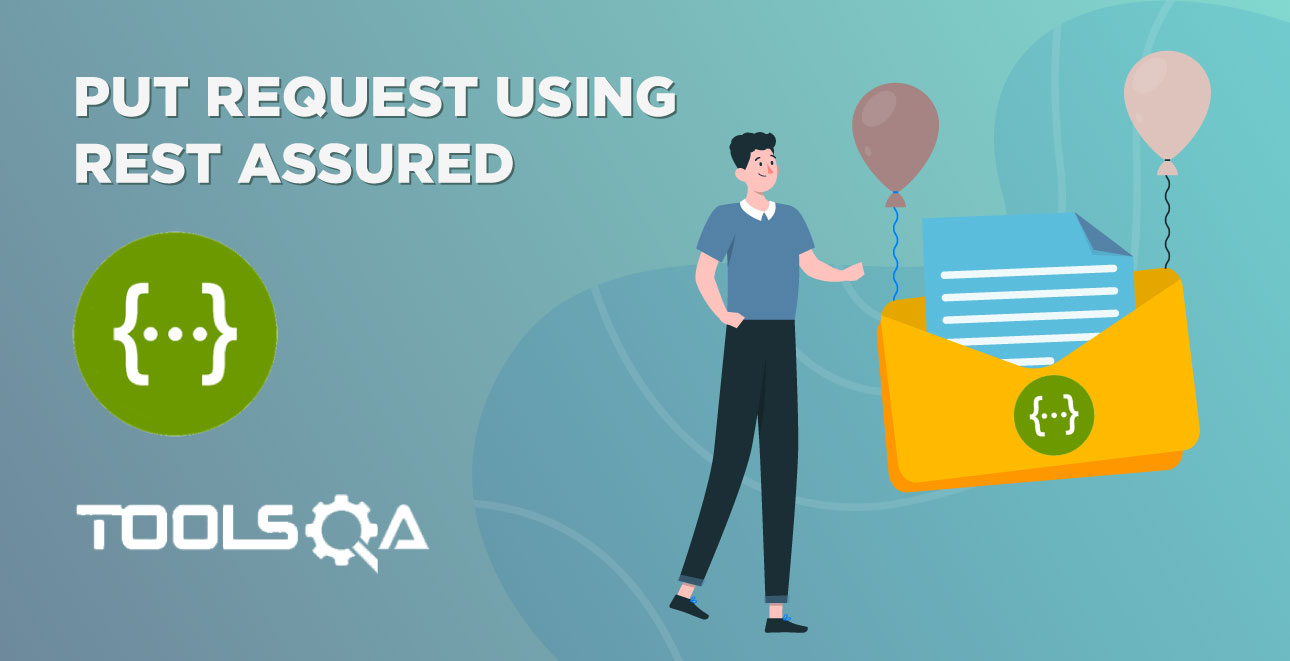
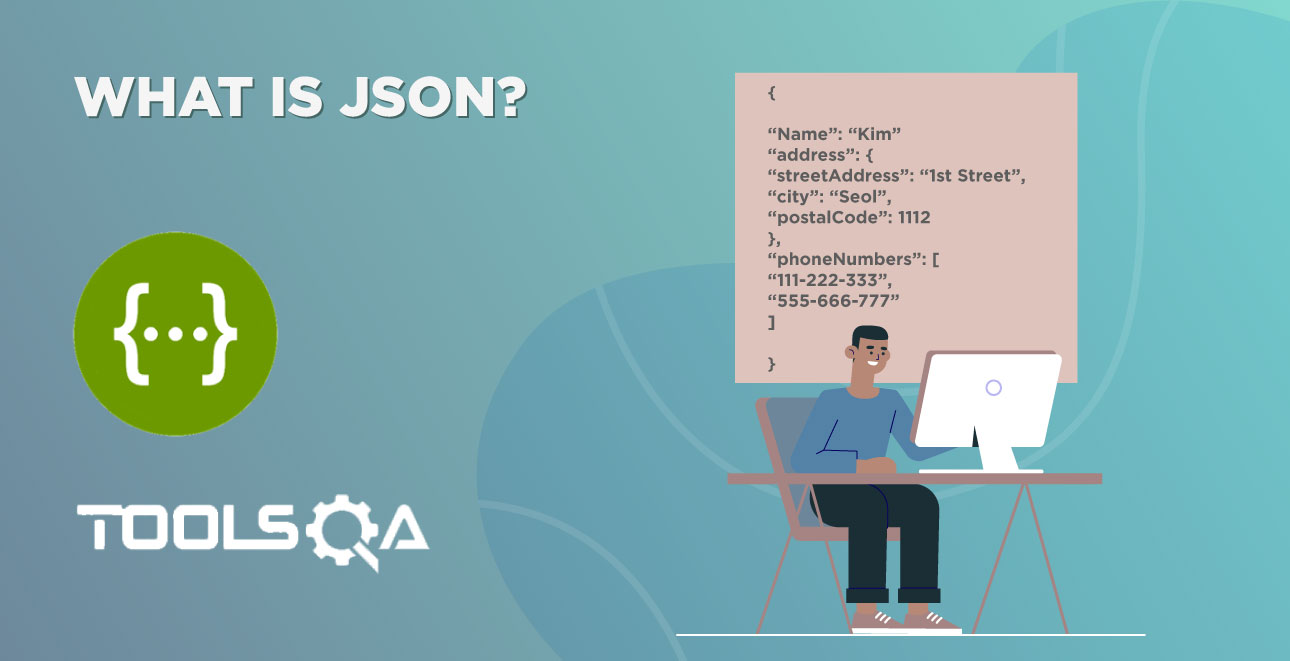