Every HTTP Response received as a result of an HTTP request sent by the client to the server has a status code. This status code value tells us if HTTP Response was successful or not. This article is a continuation of our previous article in which we performed a sample REST API test call. In this article, we will discuss how to validate the HTTP response status using REST Assured. The content of the post is reflected by the following index:
- Validate HTTP Response Status using Rest Assured
- How to validate HTTP response status code
- Validating the HTTP error status code?
- How to validate HTTP Response Status Line?
Validate HTTP Response Status using Rest Assured
An HTTP response object typically represents the HTTP packet (response packet) sent back by Web Service Server in response to a client request. An HTTP Response contains:
- A status.
- Collection of Headers.
- A Body.
We have a detailed article on HTTP Response here.
So when we say we need to validate HTTP response status, we are looking forward to having a mechanism to read and validate the entire response object including the status, headers, and the body. Hence, we will validate each of the HTTP response components separately. So in this article, the validation of an HTTP response status will be dealt with in three parts as follows:
- Validating HTTP Response Status Code.
- How to validate the Error Status Code
- Validating Response Status Line.
As we already know the same REST API returns a response message in XML or JSON format. This format depends on the Media-Type attribute in the HTTP request.
But then how will the client know what type of response it will get from the API? Well, this is managed by the response headers. Response Header contains a Content-Type attribute that informs about the type of response body format.
Consider the Swagger UI example we discussed in earlier articles. Suppose we send a GET request to the Book Store through our browser as follows:
java curl -X GET "https://demoqa.com/BookStore/v1/Books" -H "accept: application/json"
When the above command executes we obtain the response shown in below screen:
As seen from the above screenshot, the response has a status, headers, and a body. If we check the "Response headers" section, in the above screen, it has a content-type attribute that has the value along with other attributes. On validating this header, the client knows what type of response (body) we can expect.
Let us now proceed with validating the status part of the response.
How to validate HTTP response status code
When the client requests a piece of particular information from the server, the server sends a response with a status code back to the client. The status code that the server returns tells us whether the request was successful or not. If the request was successful, the server sends the status code in the range of 200-299. If the request was not successful, then the status code other than the range is returned. We can get the list of HTTP status codes along with their description on the W3 page.
Rest Assured library provides a package named "io.restassured.response" which has a Response interface. The Response interface provides methods that can help to get parts of the received response. The following screenshot shows some of the important methods of the response interface.
The method *getStatusCode() is used to get the status code of the response. This method returns an integer and then we can verify its value. TestNG Assert* is used to verify the Status Code. Now consider the code given below:
java import static org.junit.Assert.*; import org.testng.Assert; //used to validate response status import org.testng.annotations.Test; import io.restassured.RestAssured; import io.restassured.response.Response; import io.restassured.specification.RequestSpecification;
public class RestAssuredTestResponse {
@Test
public void GetBookDetails()
{
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/BookStore/v1/Books";
// Get the RequestSpecification of the request to be sent to the server
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("");
// Get the status code of the request.
//If request is successful, status code will be 200
int statusCode = response.getStatusCode();
// Assert that correct status code is returned.
Assert.assertEquals(statusCode /*actual value*/, 200 /*expected value*/,
"Correct status code returned");
}
}
The below line of code extracts the status code from the message:
java int statusCode = response.getStatusCode();
The return value "statusCode" is compared with the expected value i.e. 200. If both values are equal, then an appropriate message is returned.
java // Assert that correct status code is returned. Assert.assertEquals(statusCode /actual value/, 200 /expected value/, "Correct status code returned");
If you run the above test, you will see that the test passes since the web service returns the status code as 200 as shown in the below image.
In this manner, we can verify the status code of the response using the "getStatusCode()" method of the response interface. Please note that since we already knew that the success code here is 200, we wrote the appropriate code. Your server may respond as success with a code anywhere between 200 and 299. It is best to check that out beforehand. Let us now move on to discuss how to validate the status code that returns values other than 200 i.e. error status code.
How to validate the HTTP error status code?
So far the request-response situations are all good and we have only received 200 status codes indicating successful requests. But this may not be always true in the real world. There can be reasons like the server is down or REST API not functioning properly or the requests themselves may be problematic. In conclusion, we may face the following scenarios:
- The server hosting REST API is down.
- Incorrect client request.
- The resource requested by the client does not exist.
- An error occurs on the server side during the processing of the request.
So when any of the above scenarios occur, the REST API will return an appropriate status code other than 200. The client in turn has to validate this status code and process it accordingly.
For the ToolsQA Book Store service, let’s create another test with an erroneous scenario. Here we will validate the HTTP status code returned by Book store Web Service when an invalid parameter is entered.
So here we provide the parameter to get user details. Here we provide nonexistent userId as the parameter. The code looks as below:
java import static org.junit.Assert.*; import org.testng.Assert; //used to validate response status import org.testng.annotations.Test; import io.restassured.RestAssured; import io.restassured.response.Response; import io.restassured.specification.RequestSpecification;
public class RestAssuredTestResponse {
@Test
public void GetPetDetails()
{
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/Account/v1/User/";
// Get the RequestSpecification of the request to be sent to the server
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("test");
// Get the status code of the request.
//If request is successful, status code will be 200
int statusCode = response.getStatusCode();
// Assert that correct status code is returned.
Assert.assertEquals(statusCode /*actual value*/, 200 /*expected value*/,
"Correct status code returned");
}
}
When we run this test it returns the error code of 401. We can see the result of the test execution below.
Note: We can make a quick change to the code above to make sure the test passes. This change is shown below:
java Assert.assertEquals(statusCode /actual value/, 401 /expected value/, "Correct status code returned");
So here we expect the value returned to be 401 instead of 200, hence the test is passed. Next, we will validate the "Status line".
How to validate the response status line?
A "Status-Line" is the first line returned in the HTTP response. The status line consists of three substrings:
- HTTP protocol version.
- Status Code.
- Status Code’s string value.
For example, when the request is successful the status line will have the value "HTTP/1.1 200 OK". Here, the first part is the HTTP protocol (HTTP/1.1). Next is the HTTP status code (200). The third is the status message (OK).
We can read the entire status line using the method getStatusLine () of the response interface. The following code shows the demonstration.
java
@Test
public void GetBookDetails()
{
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/BookStore/v1/Books";
// Get the RequestSpecification of the request to be sent to the server
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("");
// Get the status line from the Response in a variable called statusLine
String statusLine = response.getStatusLine();
Assert.assertEquals(statusLine /*actual value*/, "HTTP/1.1 200 OK"
/*expected value*/, "Correct status code returned");
}
Here we perform a similar test as we have done for the status code. We read the status line using the getStatusLine () method into a string value. Then we compare this returned value with "HTTP/1.1 200 OK" to check if the status is successful.
Note: The postman article on the same topic (Response in Postman) can be found at Response in Postman.
A video tutorial for Verifying the Status code is available at Verify Status Code in Rest Assured.
Key TakeAways
In this article, we have discussed the HTTP status validation in REST Response.
- The response obtained from the server consists of status, header, and body.
- The status of the response in turn contains a status code and status string.
- Rest Assured library provides a "Response" interface that provides numerous methods to extract the response fields.
- We can read the status code using the getStatusCode() method. Similarly, we can read the status line using the getStatusLine () method of the response interface.
- Once the status is read, then we can verify if the code is a success (200) or any other code.
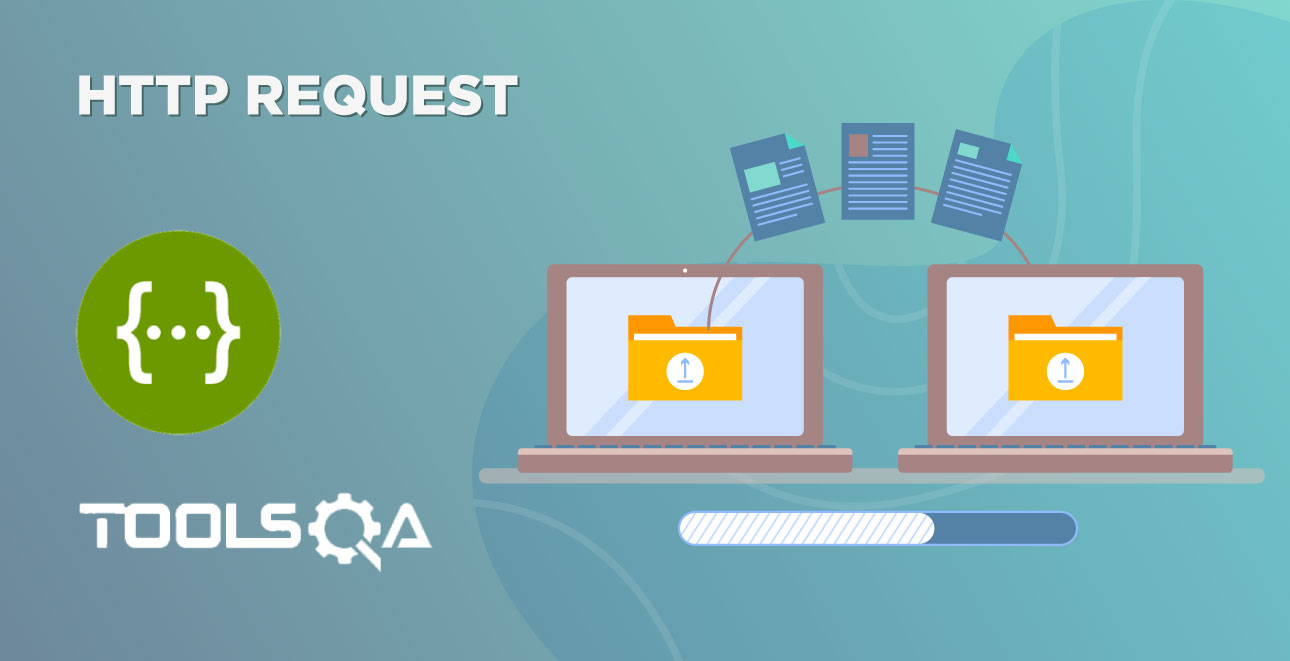
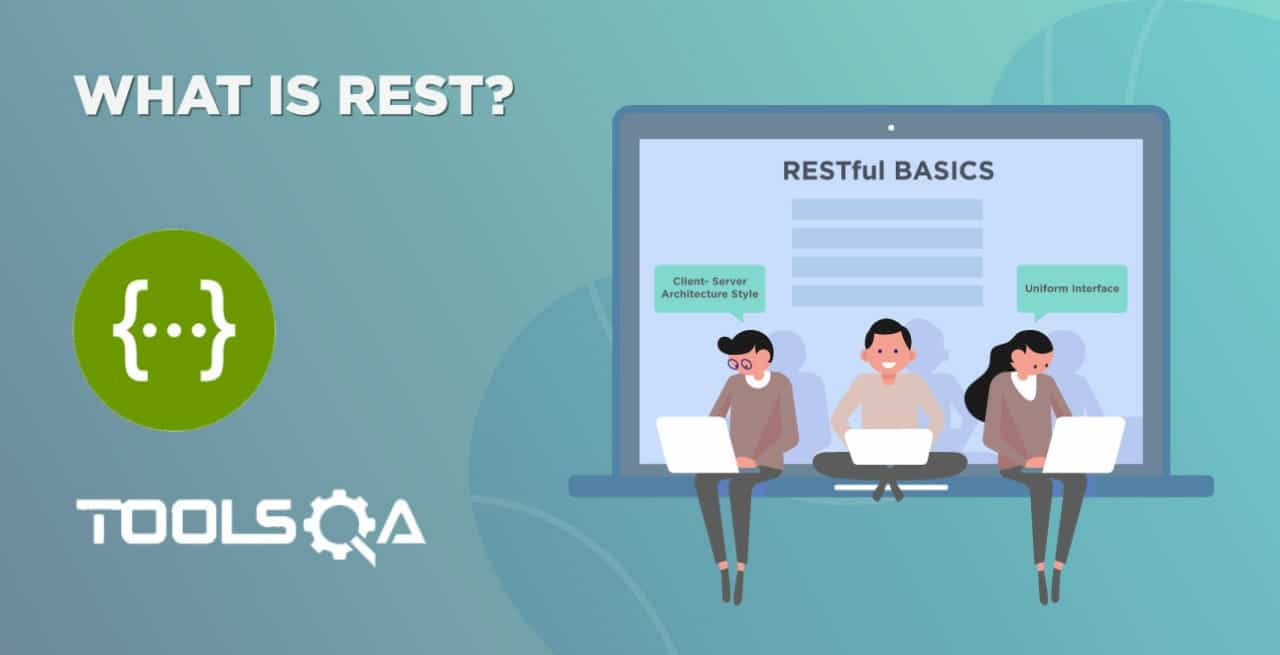