The very first question which comes to my mind and has been asked in many interviews is What is Selenium IWebDriver? Is it an Automation Tool? Is it a Class? Is it an Interface or what actually it is? To answer this question we need to understand the Advance OOPs concepts first and then we would be able to visualize the IWebDriver Implementation. For the sake of simplicity, we will avoid this IWebDriver Implementation topic for now and will cover this in later chapters. As of now, we start with all the methods we get from IWebDriver.
Now the next question is, How to access the methods of IWebDriver? To check what all we have in IWebDriver, create a driver object from IWebDriver and press the dot key. This will list down all the methods of IWebDriver.
Let's just start discussing the Orange colored methods of IWebDriver but before that try to understand the syntax of the suggestions display by Visual Studio for IWebDriver.
Method: A method is a collection of statements that are grouped together to perform an operation.
- Method Name: To access any method of any class, we need to create an object of class and then all the public methods will appear for the object.
- Getter & Setter: To get and set the value.
- Return Type: Method can return a value or returning nothing (void). If the void is mentioned after the method, it means the method is returning no value. And if it is returning any value, then it must display the type of the value.
- Description: This is a short summary of the working of the method.
Now it would be very easy to understand the IWebDriver commands in the below chapter. The very first thing you like to do with Selenium is to Opening a new browser, Perform a few tasks and Closing the browser. Below are the numbers of commands you can apply on the Selenium opened browser.
URL Command
string IWebDriver.Url { get; set;} - This method Load a new web page in the current browser window. This method get and set the value and can be used both ways.
Command - driver.Url = "appUrl"; //To open the Url
Command - String Url = driver.Url; // To get the opened Url
Where appUrl is the website address to load. It is best to use a fully qualified URL.
Example:
driver.Url = ("https://www.demoqa.com");
Title Command
string IWebDriver.Title{ get;} - This method fetches the Title of the current page. Accepts nothing as a parameter and returns a String value.
Command - driver.Title;
As the return type is String value, the output must be stored in String object/variable.
Example:
String Title = driver.Title;
Page Source Command
string IWebDriver.PageSource{ get;} - This method returns the Source Code of the page. Accepts nothing as a parameter and returns a String value.
Command - driver.PageSource;
As the return type is String value, the output must be stored in String object/variable.
Example:
String PageSource = driver.PageSource;
Close Command
void IWebDriver.Close() - This method Close only the current window the IWebDriver is currently controlling. Accepts nothing as a parameter and returns nothing.
Command - driver.Close();
Quit the browser if it's the last window currently open.
Example:
driver.Close();
Quit Command
void IWebDriver.Quit() - This method Closes all windows opened by the IWebDriver. Accepts nothing as a parameter and returns nothing.
Command - driver.Quit();
Close every associated window.
Example:
driver.Quit();
Practice Exercise - 1
- Launch a new Firefox browser.
- Open Store.DemoQA.com
- Get Page Title name and Title length
- Print Page Title and Title length on the Console.
- Get Page URL and Page Url Length
- Print Page URL and Page Url Length on the Console.
- Get Page Source (HTML Source code) and Page Source length
- Print Page Length on Console.
- Close the Browser.
Solution
using NUnit.Framework;
using System;
using OpenQA.Selenium;
using OpenQA.Selenium.Firefox;
namespace ToolsQA.Selenium_Basics
{
class DriverCommands
{
[Test]
public void Test()
{
IWebDriver driver = new FirefoxDriver();
//Launch the ToolsQA Website
driver.Url = "https://www.demoqa.com";
// Storing Title name in String variable
String Title = driver.Title;
// Storing Title length in Int variable
int TitleLength = driver.Title.Length;
// Printing Title name on Console
Console.WriteLine("Title of the page is : "+Title);
// Printing Title length on console
Console.WriteLine("Length of the Title is : " + TitleLength);
// Storing URL in String variable
String PageURL = driver.Url;
// Storing URL length in Int variable
int URLLength = PageURL.Length;
// Printing URL on Console
Console.WriteLine("URL of the page is : " + PageURL);
// Printing URL length on console
Console.WriteLine("Length of the URL is : " + URLLength);
// Storing Page Source in String variable
String PageSource = driver.PageSource;
// Storing Page Source length in Int variable
int PageSourceLength = driver.PageSource.Length;
// Printing Page Source on console
Console.WriteLine("Page Source of the page is : " + PageSource);
// Printing Page SOurce length on console
Console.WriteLine("Length of the Page Source is : " + PageSourceLength);
//Closing browser
driver.Quit();
}
}
}
Output
Run started: D:\visual studio 2015\Projects\ToolsQA\ToolsQA\bin\Debug\ToolsQA.exe
Title of the page is : Demoqa | Just another WordPress site
Length of the Title is : 36
URL of the page is : http://demoqa.com/
Length of the URL is : 18
Length of the Page Source is : 36673
Practice Exercise - 2
- Launch a new Firefox browser.
- Open http://demoqa.com/frames-and-windows/
- Use this statement to click on a New Window button "driver.FindElement(By.XPath(".//[@id='tabs-1']/div/p/a")).Click();"*
- Close the browser using Close() command
You will notice that only one window will close. Next time use Quit() command instead of Close(). At that time selenium will close both the windows.
using NUnit.Framework;
using OpenQA.Selenium;
using OpenQA.Selenium.Firefox;
namespace ToolsQA.Selenium_Basics
{
class DriverCommands_2
{
[Test]
public void Test()
{
IWebDriver driver = new FirefoxDriver();
//Launch the ToolsQA Website
driver.Url = "https://demoqa.com/frames-and-windows/";
driver.FindElement(By.XPath(".//*[@id='tabs-1']/div/p/a")).Click();
driver.Close();
}
}
}
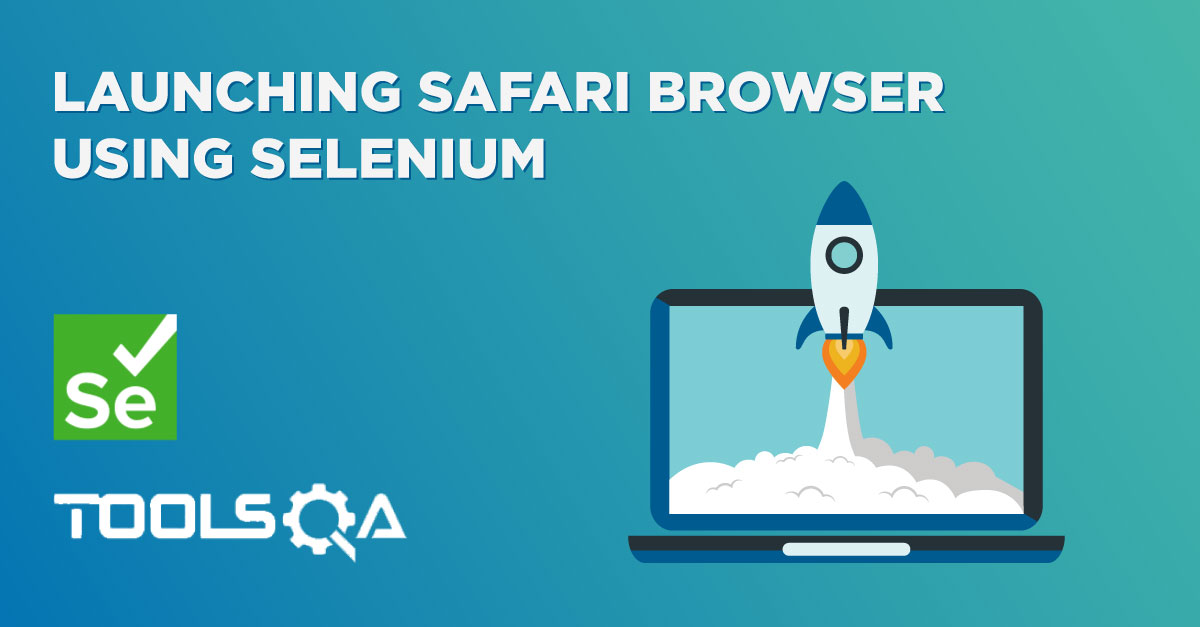
