In the past tutorials about composite data types in Python, we came across two popular data types, namely Python lists and Python tuples. Both store data in a single place with indexed access, which is quite convenient for a developer. But for those who have worked in C++ or have studied computer science as part of their education, there is a common phenomenon called hashing where we associate unique keys to specific values. Consequently, one can achieve this process in Python using these dictionaries with a few advantages, twists in properties, and add-ons for more convenience. Although it is not hashing, it works in a similar key-value fashion.
For those who are blank about what I just talked about, this post will serve as the beginner's guide for you into the world of associating one value to another. Subsequently, for highlights, you will learn the following after reaching the end of this post:
- What are Python dictionaries?
- When to use it?
- What are their Properties?
- How to Access Elements in Python Dictionary?
- How to Access Values using Get Method?
- Also, how to add an entry in Python Dictionaries?
- How to Update a value?
- How to delete a key in Python Dictionaries?
- Removing a key with the del keyword.
- Removing a key with the pop method.
- How to remove all items from a Python Dictionary?
- How to iterate over a Python Dictionary?
- Merging Dictionaries in Python.
- Merging with the update method.
- And, Merging using Kwargs.
- Built-In Python Dictionary Methods.
- Built-In Functions in Python Dictionaries.
- Nested Dictionary in Python.
- Dictionary Comprehension in Python
- Why lists can't be Python dictionary keys?
What are Python Dictionaries?
Python dictionary is one of the composite data types in Python that stores data in key-value pair. Moreover, we can see the same pattern in actual dictionaries with information in the form of a key (word) - value (meaning) pair. Python dictionaries are unordered if you are using a version prior to Python 3.6. The later versions have made the dictionaries an ordered data type. Additionally, we can retrieve the values by calling out the key associated with them. One can initialize them with the following syntax:
dictionary_name = {'key1': 'value1', 'key2' : 'value2'}
A colon ": " separates the key and the value, and the key-value pairs are separated by a comma (,) in a dictionary. In addition to this, an important point to note is that the keys in the dictionary need to be of immutable data type only, such as string, numbers, or tuples.
When to use it?
With three data types in our hands, it is easy to get confused about when to use which one during programming some logic. Dictionaries are straightforward, though. Additionally, whenever you have a use case where you have to associate two values out of which one is always unique, a python dictionary is the best way to go forward. Moreover, they are extremely fast and can perform quick operations.
Take an example of hotel reception software that we need to build for a hotel. For simplicity, the software has only one module that excels in storing the records of the hotel rooms and the guests checking into them. Which way do you think is more optimum? Lists or dictionaries? Let's see the Python lists first.
In Python lists, we can call out the values concerning their index numbers. So to associate a room number with the guest name becomes easy by referring to the index as room number and the value at that index as the guest name. But, there is a small problem here. Let's say we have a ten-floor hotel with only ten rooms on each floor. Room numbers are constructed as <floor_number>
<room_number>
starting with 1. So, the eighth room on the ninth floor will be 908. Consequently, to save this record, we have to access the 908th index of the list, which is massive wastage of memory because we do not have 908 rooms in our hotel. In addition, deletion is an expensive operation in lists which will cost us more.
In such scenarios, the python dictionary is a better choice for implementation. For example, for 100 rooms (10 on each floor), we have to store 100 keys instead of 1000 indexes on Python lists. Moreover, we can associate room number to the guest names as follows:
hotel_records = {'101' : "John", '304' : "Paul", '403' : "Mia"}
To print the complete dictionary, use the print function with the dictionary name as an argument:
hotel_records = {'101' : "John", '304' : "Paul", '403' : "Mia"}
print(hotel_records)
Output:
If only three guests are staying in the hotel, our dictionary is just three key-value pairs, whereas, in Python lists, we have to fix 1000 indexes every time, even without any guest staying in the hotel.
Properties of Python Dictionaries
Similar to other data types in Python, Python dictionaries come with their set of properties too.
- They are mutable.
- Moreover, we can nest them with multiple dictionaries.
- Additionally, they are dynamic, and the size can be expanded (or shrunk) according to the requirement.
- Moreover, the Python dictionary does not allow duplicate key values.
- The keys in the Python dictionary need to be of an immutable type.
- Also, Python dictionary keys are case-sensitive. Hence, "James" and "james" are two different keys.
- Python dictionary values have no restrictions on the data type as the keys.
Now that we know our Python dictionary quite well, let's perform a few operations on them.
How to access elements in Python Dictionary?
We can create a Python dictionary by defining the key-value pair as explained above. To recall, let's create a simple dictionary with the same example of hotel rooms associated with guest names as follows:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
Moreover, to access a value in the dictionary, we need to call out their respective key. This process is demonstrated in the below code:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
print(hotel_records[301])
Output:
This is the most simple and straightforward method to access a value in the dictionary. Let's see one more method to perform the same operation.
How to access values in a Python Dictionary using the Get method?
Similar to accessing the value directly through keys, we can also use the "get" method for the same purposes. In the example below, the get method is used in the same code defined in the previous section:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
print(hotel_records.get(301))
The output will be the same as we got through direct access.
I know what you are wondering right now. If both methods are used to access the values, why do we even have two methods in the first place? That's a genuine question that I asked myself when I learned about the get method until I accessed something which did not even exist in my Python dictionary.
Let's see the following code that uses both of the methods to target a key that does not exist in the Python dictionary:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
print(hotel_records[109])
Output:
Edit the above code to use the get method for the same key:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
print(hotel_records.get(109))
Output:
The difference is now lucid with the above outputs. While using the get method, we do not get an error when the key is missing from the Python dictionary, but direct access does. Therefore, the program execution does not break, and we can even use the get method to apply some checks and other logic. Moreover, in the direct access method, we get an error that is of no use in a program where we are not sure if the keys would exist or not, such as our hotel example.
How to add an entry in Python Dictionaries?
Adding an entry in a Python dictionary is a straightforward process. First, we need to assign a key to a value, and the pair will automatically add to the dictionary.
Consequently, the following code adds the key 507 into the existing python dictionary "hotel_records":
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
print(hotel_records)
hotel_records[507] = "Russell"
print(hotel_records)
Output:
As seen in the above output, the key-value is added automatically.
How to update a value in Python Dictionary?
While adding a value to the python dictionary, Python looks for the key in the dictionary. Additionally, if it does not exists, Python adds it to the dictionary. But if the key already exists in the dictionary, then Python will update its value to the new value. So, the code remains the same, except this time, we assign an existing key a newer value.
In the following code, I have added an extra line to the code mentioned above, assigning the value to an existing key 507:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul"}
print(hotel_records)
hotel_records[507] = "Russell"
print(hotel_records)
hotel_records[507] = "Peter"
print(hotel_records)
Output:
The value of key 507 is updated accordingly.
How to delete a key in Python Dictionaries?
To delete a key in the dictionary, we have two methods in Python- with the del keyword and the pop method. Although both can remove the key from the dictionary, the pop method gives us an added advantage that we can use in certain situations.
Removing a key with the del keyword
The del keyword is used to delete the key-value pair from the python dictionary. To use the keyword the adhere to the following syntax:
del <space> dictionary_name[key]
Consequently, the usage is shown in the following code:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
print(hotel_records)
del hotel_records[301]
print(hotel_records)
Output:
The key-value (301) has been deleted successfully.
Removing a key with the pop method
An alternative method by which we can remove the keys in a python dictionary is using the "pop" method. Additionally, the programmer needs to pass the key inside the pop method as an argument, and the key-value pair will be deleted successfully (if the key exists).
In the following code, the pop method is demonstrated by deleting the key 301:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
print(hotel_records)
hotel_records.pop(301)
print(hotel_records)
Output:
The pop method deletes the key-value pair. So, what is the added advantage offered by the pop method over the del keyword?
The pop method can delete and return the key's value simultaneously if used inside the print method. This way, if the programmer needs to see or show the deleted key's value, it can be done with the pop method directly without a third variable. For example, the following code demonstrates the same:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
print(hotel_records)
print(hotel_records.pop(301))
Output:
Although we get the popped out value, it depends on the use cases and the requirement to choose one of these methods. For example, the del method can delete the entire dictionary for you, but the pop method cannot (del dictionary_name). It is convenient to have the options, though. Also, note that both of these methods work only if the key exists in the dictionary. Otherwise, an error is thrown.
How to remove all items from a Python Dictionary?
The del keyword will delete the dictionary completely and will make it non-existent. But what if we don't want to delete the dictionary but empty the contents of it? To do so, we make use of the clear method as follows:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
hotel_records.clear()
print(hotel_records)
Output:
The elements get deleted, but the dictionary still exists.
How to iterate over a Python Dictionary?
Now that we have added elements to our dictionary and deleted the unwanted elements, we need a method that can help us iterate the dictionary to view its elements. Additionally, to iterate over a python dictionary, we can use the "for" loop in Python. The following code demonstrates the process:
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
for i in hotel_records.items():
print(i)
Output:
Since we iterate over only one variable (i), both the key and its value is visible in the output. Moreover, taking two iterating variables would save keys in the first variable while values in the second variable.
hotel_records = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
for i,j in hotel_records.items():
print(i)
Output:
As a practice, try to print out the values of these keys with two variables.
Merging Dictionaries in Python
The final operation we shall see before concluding this post is how to merge two dictionaries into a single one. To merge two dictionaries, Python ships with two built-in methods, among which one is only applicable in Python 3.5+. Consequently, let's explore the conventional method first.
Merging Python dictionaries with the update method
The first method to merge two dictionaries in Python is the update method. The update method can be used with any Python version and is the conventional method of merging the dictionaries. We can use the update method in the following form:
hotel_records_1 = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
hotel_records_2 = {304 : "Michael", 506 : "Nelson"}
hotel_records_1.update(hotel_records_2)
print(hotel_records_1)
Output:
The second dictionary is merged at the end of the first.
Merging Python Dictionaries using Kwargs
The second method of merging two dictionaries in Python is by using kwargs. "kwargs" passes the argument list in Python. Here in this reference, the argument list will be the dictionary that we intend to merge. The following code demonstrates the usage of kwargs in Python to merge two dictionaries:
hotel_records_1 = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
hotel_records_2 = {304 : "Michael", 506 : "Nelson"}
merged_dict = {**hotel_records_1, **hotel_records_2}
print(merged_dict)
Output:
The updated dictionary is visible in the output. A small question arises here. What would happen when the second dictionary contains a key that already exists in the first dictionary? In the above code, change the second dictionary key 304 to 301 and observe the changes:
hotel_records_1 = {301 : "James", 104 : "Jack", 803 : "John", 305 :"Paul", 507: "Russell"}
hotel_records_2 = {301 : "Michael", 506 : "Nelson"}
merged_dict = {**hotel_records_1, **hotel_records_2}
print(merged_dict)
Output:
With the above output, we can conclude that if there is a duplicate key in the second dictionary, its value is replaced in the updated dictionary. The updated value is from the second dictionary, and hence the property of the unique key is maintained.
Built-In Python Dictionary Methods
The following list exhibits various dictionary methods available in Python:
Method | Description | Example |
---|---|---|
items() | Returns an object in the dictionary’s list of items in the form of key-value pair. | dictionary_name.items() |
clear() | Removes all the dictionary items. | dictionary_name.clear() |
copy() | Returns a shallow copy of the dictionary. | dictionary2 = dictionary1.copy() |
update(dict) | Updates the values if the key already exists in the dictionary 1. Else, adds that key to the dictionary. | dictionary_name.update({key: value}) |
pop() | Returns the key’s value and deletes the key from the dictionary. | dictionary_name.pop(key) |
get(key) | Returns the value of the key. | variable = dictionary.get(key) |
setdefault(key) | Return the value of the key if it exists. Else, inserts the key into the dictionary and returns its value. | dictionary_name.setdefault(“key”, “value”) |
values() | Returns a new object of the dictionary’s values. | dictionary_name.values() |
In the list given above about the methods used in Python dictionaries, we use two of the methods keys() and values() frequently. So let's understand them with examples.
The keys() and values() methods print out the keys and the values respectively on the console. These are separate entities and don't pair up like key-value pairs. However, since they work quite similarly, we can confine both of them into a single code snippet as follows:
hotel_records = {'101' : "John", '304' : "Paul", '403' : "Mia"}
print("The Keys Are")
print(hotel_records.keys())
print("The Values Are")
print(hotel_records.values())
Output:
We can see the keys and the values in the output without being in pairs. You can also use this function to take all the keys in a variable and then iterate over it.
Built-In Functions in Python Dictionaries
The following list exhibits various functions that we use, along with their descriptions:
Function | Description | Example |
---|---|---|
len() | Returns the length of the dictionary (key count). | len |
(dictionary_name) | ||
sorted() | Returns the dictionary with keys sorted in ascending order. | sorted (dictionary_name) |
all() | Returns True if all the keys in the dictionary are True (not 0 and False). | all (dictionary_name) |
any() | Returns True is any of the keys in the dictionary is True. | any |
(dictionary_name) | ||
str() | Returns a string representation of the dictionary passed as the argument. | str (dictionary_name) |
Nested Dictionary in Python
Nested dictionaries in Python are similar to the nested lists where we construct a dictionary inside another dictionary. We have demonstrated a simple use case in accordance with our running example of the hotel software below:
hotel_records = {304 : {"Name" : "James", "ID" : "DL"}, 104 : {"Name": "Jack", "ID" : "Birth Certificate"}, 803 : {"Name": "John", "ID" : "Employee Card"}}
print(hotel_records)
Output:
In the above example, I have used a nested dictionary to store guest records in a hotel. Now I can retrieve the name of the guests staying in the hotel rooms and the ID they presented at the time of check-in. In addition, nested dictionaries are easier to traverse and contain much readable info, as shown above.
Dictionary Comprehension in Python
Dictionary comprehension in Python is an easy way to construct a new dictionary with the help of iterables used inside a "for" loop. The same phenomenon is also offered in Python lists which we recommend you to go through the post before going ahead in this section. A dictionary comprehension consists of a key-value pair logic followed by a "for" loop.
The following code constructs a Python dictionary using dictionary comprehension.
new_dict = {x : x + 2 for x in range(2, 9)}
print(new_dict)
Output:
The creation of a new dictionary happens with the logic provided in dictionary comprehension.
Why lists can't be python dictionary keys?
A lot of beginners raise a popular question who have just started to learn Python, which is "can we use Python lists as Python dictionary keys?" Unfortunately, the answer here is no; we cannot. This is because python dictionary keys have to be immutable in nature, as mentioned in the introduction of dictionaries in this post. These immutable elements can be strings, integers, or even tuples, but Python lists being mutable to their core cannot be used as dictionary keys. However, you have permission to use Python lists as the values inside the dictionary.
new_dict = {"name" : ["ABC", "XYZ"]}
print(new_dict)
Feel free to try and experiment with a Python dictionary that will help you learn and strengthen the foundation.
Conclusion
Python dictionaries are an important part of Python programming and PCEP exams as well. Often, you would find yourself in situations that could have made your work easier by using them. For example, working with forms in web development will signify that you will have a key (form input field name) and a value (user's input) associated with it. Using a list will also work here, but we might have to remember all the input elements. Also, if we need to insert one more input in between the form in the future, that will mess up our calculations of indexing.
In this post, we explained every part of the Python dictionary that we would require in the future for PCEP or programming or any development work. From a simple task of adding a key-value pair in the dictionary to dictionary comprehension, they are versatile and bring significant features to the table. I hope this post will help you in your Python career ahead.
Key Takeaways:
- Python dictionaries are one of the composite data types available in Python. Moreover, they store the data in the form of key-value pair.
- They do not allow duplicate key values.
- Additionally, Python dictionary keys should be immutable in nature. So, for example, we can use strings and integers as dictionary keys while a list cannot.
- Also, they allow dictionary comprehension.
- Moreover, a python dictionary can also nest with another python dictionary.
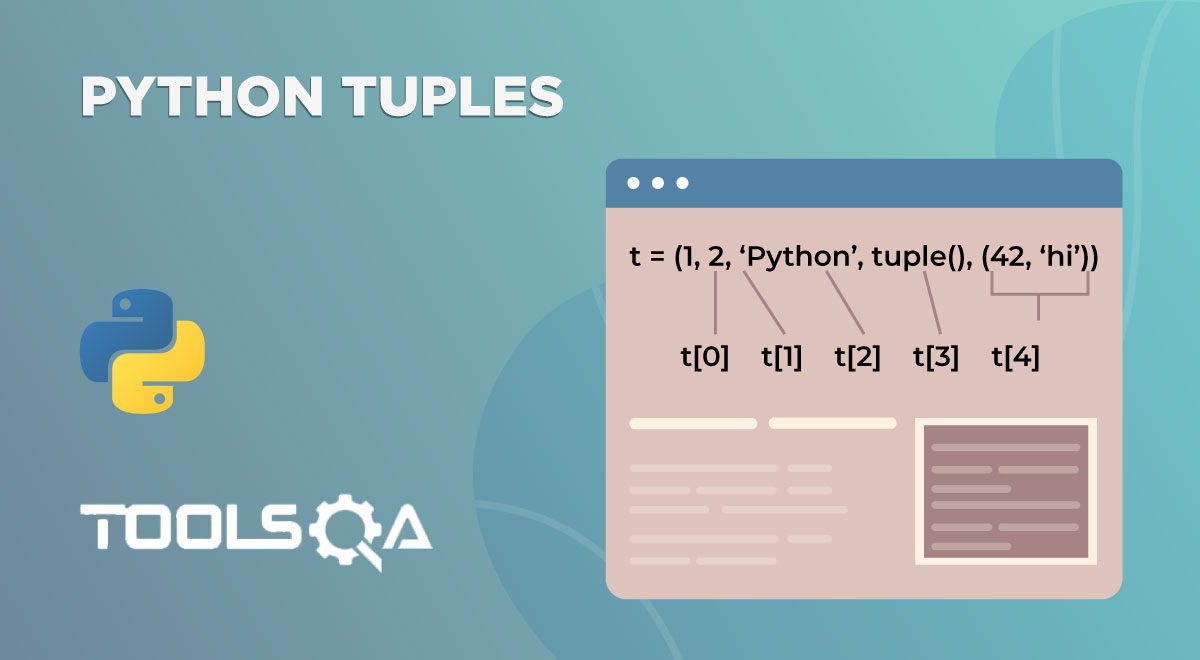