Python runs on two main loops discussed in the previous posts; the "for" loop and the "while" loop. These loops check the conditions and run multiple iterations until our sequence consumes or the condition satisfies. With the knowledge acquired in the previous posts, we can control the loop from its beginning: the condition and the sequence. This post will help us enforce control in the Python loops from within the loop code body. By the end of this post, we will write a code that can break a loop between or skip some code-based. The concepts used to achieve these actions are the loop control statements: the python break statement, control statement and pass statement. This article will revolve around the same loop control statements with the following major sections:
- What is Python break statement?
- How to implement a break statement in Python?
- Flowchart for Python break statement
- What is Python continue statement?
- How to implement a python continue statement?
- Flowchart for Python continues the statement.
- What is Python pass statement?
- How to implement a python pass statement?
- Flowchart for Python pass statement
What is Python break statement?
The first one in our list of loop control statements in Python is the break statement. Guessing the job of a break statement in Python by its name is relatively easy, and it does what it means. A break statement breaks the python loop flow regardless of the loop's natural completion. In simple words, a break statement can terminate the current iteration and exit the loop. Once a break statement is met, the loop cannot continue.
The break statement can be written by the word "break" anywhere inside the Python program. Usually, a break statement meets specific conditions, but it is a programmer's choice to use this loop control statement.
How to implement a break statement in Python?
Python break statement example: A simple example of a python break statement is to iterate the word "ToolsQA" and break the loop when we encounter the letter "Q."
for c in "ToolsQA":
if c == "Q":
break
else:
print(c)
The above loop prints the characters starting from "T" until a "Q" encounters. The above code uses for loop in Python. Please refer to What are Python "for" loops and how to implement them.
Output:
The output shows that the statement breaks the loop when we encounter a "Q ." Therefore, "A" is also skipped along with it (as it will execute in the next iteration). Hence, our loop terminated on meeting the break statement.
So, what happens when we apply a break statement in a nested loop? Will, both of the loops, get terminated or only the one where the break statement executes?
The following code demonstrates the working of a break statement inside nested loops.
for i in range(3):
print("i == " + str(i))
for j in range(3):
if j == 2:
break
print("j == " + str(j))
Output:
Notice that for every iteration of the variable "i, "the variable "j" is executing only two times (0 and 1) instead of 4 times (0,1,2,3) as intended. Still, the variable "i " complete all of its iterations. Hence we can conclude that a python break statement inside a nested loop terminates only the immediate loop and not all of them.
Flowchart for Python break statement
The flowchart of the Python break statement is as follows:
What is Python Continue Statement?
The Python continue statement skips the current iteration and lets the loop execute or "continue" naturally. If the "continue" conditions meet, the loop is executed only before the continue statement. All the statements after "continue" get skipped, and the next iteration follows.
The continue statement can be written by the word "continue" inside the Python loop. A "continue" statement can be put anywhere inside a loop but only with caution as it affects a loop's flow.
How to implement Python continue statement?
The Python continue statement example: The following example iterates over the word "ToolsQA" and implements a continue statement when the character "Q " is iterating.
for c in "ToolsQA":
if c == "Q":
continue
else:
print(c)
Output:
In the above program, the iteration skips as soon as we find the character "Q." But, unlike the break statement, the loop continues naturally until all the characters of "ToolsQA" don't iterate.
Can you guess what would happen if the print statement is moved to the top section of the loop as follows:
for c in "ToolsQA":
print(c)
if c == "Q":
continue
Output:
It is the same as not having a "continue" statement at all!! Why would "Q " get printed even after having a continue statement?
The answer to this question is inside the definition defined above. A continue statement only skips the code after itself, but all the code before a continue statement gets executed normally. So, when you implement a continue statement in Python, pay extra attention to the code arrangement.
Flowchart for Python Continue Statement
The flowchart of the Python continue statement is as follows:
What is Python Pass Statement?
With the python continue statement, we can skip the iteration, and with the break statement, we can break the iteration, so what else remains in this segment? Something that will do nothing!!
Python pass statement is an empty check which does not interrupt the normal flow of the loop. When a pass statement executes, nothing happens. So, why do we have such a thing that does nothing?
Python pass statements are constructive when a developer wants to keep the code as it is and write the logic afterwords. Instead, they can write "pass" in place of that logic to check the program's execution without the code. It is also helpful for a stub code. A Python pass statement resembles a null operation.
How to implement a Python pass statement?
Python pass statement example: In the below example, we have a pass statement that denotes a piece of code that will be implemented later.
for c in "ToolsQA":
if c == "Q":
pass
else:
print(c)
Output:
The above Python code uses the "pass" statement whenever we encounter a "***Q ***." The result: Python compiler does nothing and lets the loop continue. However, the pass statement in Python is different from the continue statement as in the pass statement; the loop will execute all the code after "pass," whereas, in the continue statement, we skip the code.
Flowchart for Python pass statement
The flowchart for the Python pass statement is as follows:
It brings us to the end of loop control statements, which are an essential part of the PCEP course structure and a regular programming routine. These statements can be a bit confusing in their work at the start. Therefore, we will recommend mixing and playing with these statements and learn all the use cases you are thinking about.
Key Takeaways
- The Python programming language comprises three control statements for loops that break the natural flow of the loop.
- The break statement in Python breaks the current iterations of the loop and exits the loop once executed.
- Python's continue statement skips the loop's current iteration while the loop continues naturally till the end.
- The pass statement in Python is equivalent to the null operation. The pass statement does nothing and is commonly used to check the loop and bookmark a point for adding the code later.
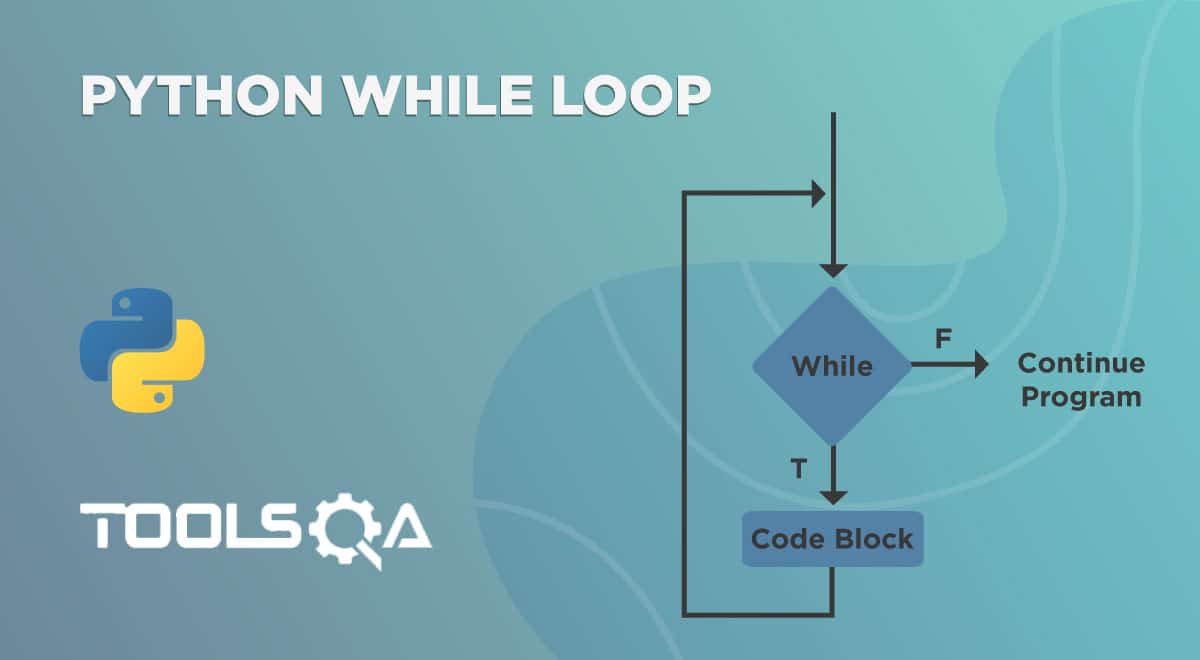
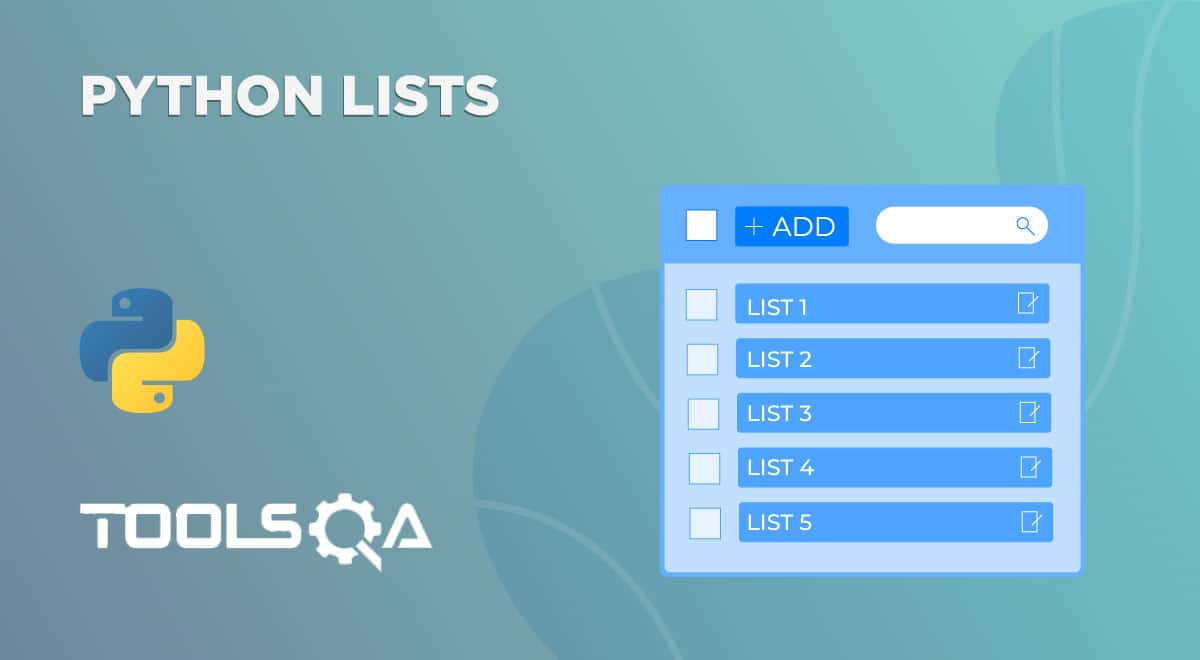