In this course, we have come a long way now. From data types to operators to loops, we have covered the basics of building up a program in the python programming language. But still, we are missing a critical point. Sure we can declare a few variables and compare them, but that is a temporary solution for single points lying around in the program. Are we going to declare ten variables to save the names of ten persons? And then ten more to save their age? I know we can! But we need to find smarter ways to construct a program. It brings us to data collections in python and, specifically: python lists.
Python list is a type of compound data type used for data collection and storing the values in a single place. Lists in python are probably the most used data type and offer many functions for the programmer for quick operations. We will learn all these functions and methods step by step. You can navigate this post with the following index:
- What are lists in Python?
- How to implement a list in Python?
- How to access an element in a Python list?
- Can we use a Negative index in the Python list?
- How to add and delete elements in a list in Python?
- Lists inside List - Can we do that?
- List Membership test: How to find an element in Python Lists?
- How to traverse python lists using loops?
- How to slice a list in Python?
- List comprehension in Python?
- Conditional list comprehension in Python.
- Python List Methods.
What are lists in Python?
Lists in python are the data collectibles that help in storing data in a single place. Lists in Python closely resemble arrays in C++ and Java but offer a lot more in-built functionalities and methods. A simple structure of a list in python storing names is as follows:
names = ["Jack", "Jill", "Harvey", "Bill", "Joseph"]
In this list, "names" is the name of the list, while the names Jack, Jill, Harvey, Bill, and Joseph are the list elements or list items. We order these list items in nature, i.e., they have a defined order that does not change when adding more elements to the list.
So, adding another name, "Agatha," will not interfere with the position of the already existing names in the list. The list will hence become:
names = ["Jack", "Jill", "Harvey", "Bill", "Joseph", "Agatha"]
Secondly, Python lists are changeable. If there arises a need to replace an element with another element, you can do that by referencing the index. For example, if I want to change the name at index 0, i.e., Jack with Christopher, I can write the following code:
names[0] = "Christopher"
print(names)
Thirdly, since lists in python are in index form, they allow duplicate values, unlike sets. So, we can change the element at index 1 to "Christopher" again.
names[1] = "Christopher"
print(names)
Lastly, python lists a critical property list because one list can collect various data types that are not possible in C++ or Java.
mixed_list = ["Jack", "Jill", 23, 32, True]
The above list contains string data type, integer data type, and boolean data type.
How to implement a list in Python?
Creating a list in python is extremely simple. There are two use cases for initializing a list: an empty list or a list with elements in it.
An empty list can be created by simply providing two square brackets with no element inside.
names = []
We can write a list with elements inside the square brackets with the defined order you want them to be.
names = ["Jack", "Jill", "Christopher"]
You can also combine various data types, as we saw in the previous sections.
How to access an element in Python lists?
As the elements are indexed, you can access the elements like C++ vector or array providing the index with the list name.
With the following list:
names = ["Jack", "Jill", "Thomas"]
I can access "Jack" as print(names[0]). The index starts from 0 and is up to the length of the list - 1 (len(list_name)). Keep in mind to write the index number within that range only to prevent out-of-bound errors. for the list, we have declared -
names[0] will refer to Jack
names[1] will refer to Jill
names[2] will refer to Thomas
name[3] will give you an index out of bound error, which means that the index you refer to does not exist.
Can we use a negative index in python lists?
Yes! Python allows the programmer to use negative indexes while accessing the list elements. The negative indexes correspond to accessing the elements from the end of the list starting from -1.
So let's take the same "names " list that we defined above. In this case:
names[-1] will refer to Thomas
names[-2] will refer to Jill
names[-3] will refer to Jack
How to add and delete elements in a list in Python?
In writing a program, a lot of the time, you would want to add a few elements to the list and delete a few. It can be achieved with the pre-defined function in the list called append and remove.
The following code adds a new name, "Richard," to the existing list of names.
names = ["Jack", "Jill", "Christopher", "Harvey"]
names.append("Richard")
print(names)
Output:
To add multiple names together, you can use the "extend " method:
names = ["Jack", "Jill", "Christopher", "Harvey"]
names.extend(["Robert", "Paul", "Vincent"])
print(names)
Output:
The following code removes the name "Christopher " from the existing list of names.
names = ["Jack", "Jill", "Christopher", "Harvey"]
names.remove("Christopher")
print(names)
Output:
All these methods have also been stated at the end of this post for your reference.
Lists inside Lists - Can we do that?
A good question to think around, especially for C++ and Java programmers, is how to implement a 2-D matrix in Python? Or, is it possible to implement one list inside another, a nested list in simpler words? I don't know that either! Let's find out!
We can try to implement a 2-D matrix of integers (random numbers). A 3X3 matrix is probably sufficient.
The first step is to construct a skeleton by initializing a list of numbers that is simple to implement:
matrix = [1,2,3]
Looking at the above list, it does signify a 1X3 matrix. So, how can we make it 3X3?
Maybe we can try inserting three numbers at each index in the form of another list.
matrix = [[1,2,3],[4,5,6],[7,8,9]]
Print the above matrix to see the results.
We were successful in the implementation. Try accessing the element "5 " using two indices as follows:
print(matrix[1][1])
Output:
Can we implement a list inside a list of lists? Try around and find out!!
List Membership test: How to find an element in Python Lists?
A membership test is an assurance that the element we are searching for is inside the python list or not. Reading this concept, the first thing that comes to mind is tracing the entire list and comparing each element. If we find a match, we have the element! That's one of the approaches to yield the correct result. But programming is not always about working things out but working things in a concise, faster, and smarter way. Python developers have left no stone unturned in providing a language that is programmer-friendly in nature. Python solves a four-line code in a single line by providing in-built membership test implementation.
To test whether the element is inside the python lists, we use the word "in," Conversely, the word "not in" to test that word is not inside the list.
names = ["Jack", "Jill", "Harvey", "Mike"]
print("Harvey" in names)
The output, as expected, comes out to be True.
Similarly, try "not in" with various use cases by yourself. It is an important topic, and while reading other's code, you will find this a common practice in Python. Therefore, a good grip over it will benefit your python programming career and PCEP exam.
How to traverse python lists using loops?
A list is a sequence of elements. Rewinding to the "for" loops in python post, I mentioned the definition of the "for" loop as follows:
"The “for” loop in Python is a way to run a repetitive block of code traversing over a sequence of any kind."
One kind of sequence we have here is the python lists which we can traverse in two ways:
- Defining the length of the list in the loop:
for i in range(0, len(names)):
print(names[i])
you would have guessed by now that len (names) will give the length of the list - Try printing out len (names) and see the result?
- Using list membership:
for i in names:
print(i)
The second way is cooler and faster, and we can achieve it on any data collectable such as tuple, dictionary, etc. These will have their dedicated posts in this course.
How to slice a list in Python?
Slicing a list is exactly what it means in English. We cut the list from one index to another index, but the good thing is, the original list does not get altered with this operation.
A python list can be sliced with the ":" symbol as follows:
names = ["Jack", "Jill", "Harvey", "Bill", "Joseph", "Agatha"]
To print the names from Jill to Joseph, we can slice it out as follows:
print(names[1:5])
Output:
The end index is not covered. So we get the list from 1 to 4.
List Comprehension in Python
The term List comprehension in Python is a way to create a new list from an existing list. List comprehension uses for loop to iterate over the existing list and create a new list out of it. The syntax for list comprehension is as follows:
[expression for element in list]
Suppose I have a list with integers from 1 to 5. In that case, I can use list comprehension in Python to create another list after multiplying each index element with 2 (or any of your favorite numbers!)
list_1 = [1,2,3,4,5]
list_2 = [2 * i for i in list_1]
print(list_2)
The above code can be related to the syntax in the following way:
Output:
Is it clean, concise, and simple? You can also use conventional for loop code to implement the same but then again, why would you!
Conditional List Comprehension in Python
In the above section, we saw a simple demonstration of list comprehension where you traverse a sequence, implement an expression, and create a new list. Along with it, you can also use some conditional statements (used popularly) to create a list comprehension which is a little more helpful but complex.
The syntax for conditional list comprehension is as follows:
[expressions for element in list condition]
The following code saves even numbers from a list of natural numbers.
natural_numbers = [1,2,3,4,5,6,7,8,9,10]
even = [i for i in natural_numbers if i % 2 == 0]
print(even)
Output:
List comprehension in python can do a lot of work in a single statement. But, it would be best if you were careful while using these in the code. Too complicated comprehensions are also difficult to read for others which is not a good programming practice.
Python List Methods
The basics of python lists and their implementation have been discussed until this point. But python is incomplete without providing a rich in-built method list for which it is famously known. Python lists also provide many useful functions that will help you construct a program with python lists more efficiently. They are described in the list below:
append() - It helps to add an element to the list.
extend() - To add multiple elements to the list / add all the elements inside a list to another list.
insert() - To insert the elements at a defined index.
remove() - To remove an element from the list.
sort() - To sort the list elements into ascending order.
sort(reverse = True) - To sort the list elements into descending order.
reverse() - Reverse the order of the python list elements.
count() - Returns the count of the number of times the passed argument is present in the list.
index() - Returns the first matched index of the passed argument.
clear() - Removes all the items from the python list.
pop() - Removes and returns an element at the given index.
len() - Returns the number of elements inside the python list.
The use of these methods will make your program a lot more programmer-friendly and efficient in nature.
We will cover these methods with examples later in the course. With these methods, we successfully conclude this post with a quote said by the designer of Python. Guido van Rossum said once, "Python is an experiment in how much freedom programmers need. If there is too much freedom, then, nobody can read another's code; if it's too little, expressiveness is endangered".
Key Takeaways:
- Lists in python are a type of data collectibles that help in storing data in a single place.
- Python lists can contain elements with different data types.
- Python lists are ordered, changeable and allow duplicate values.
- Negative indexes in python lists access the elements from the end.
- Implementing a list inside another list creates a 2-D list of elements.
- Python provides list membership tests to test whether an element is inside the list or not.
- To implement a new list from an existing list, python uses list comprehensions.
- List comprehensions also support conditional statements.
- Python lists come equipped with many methods for quick results such as append, extend and sort.
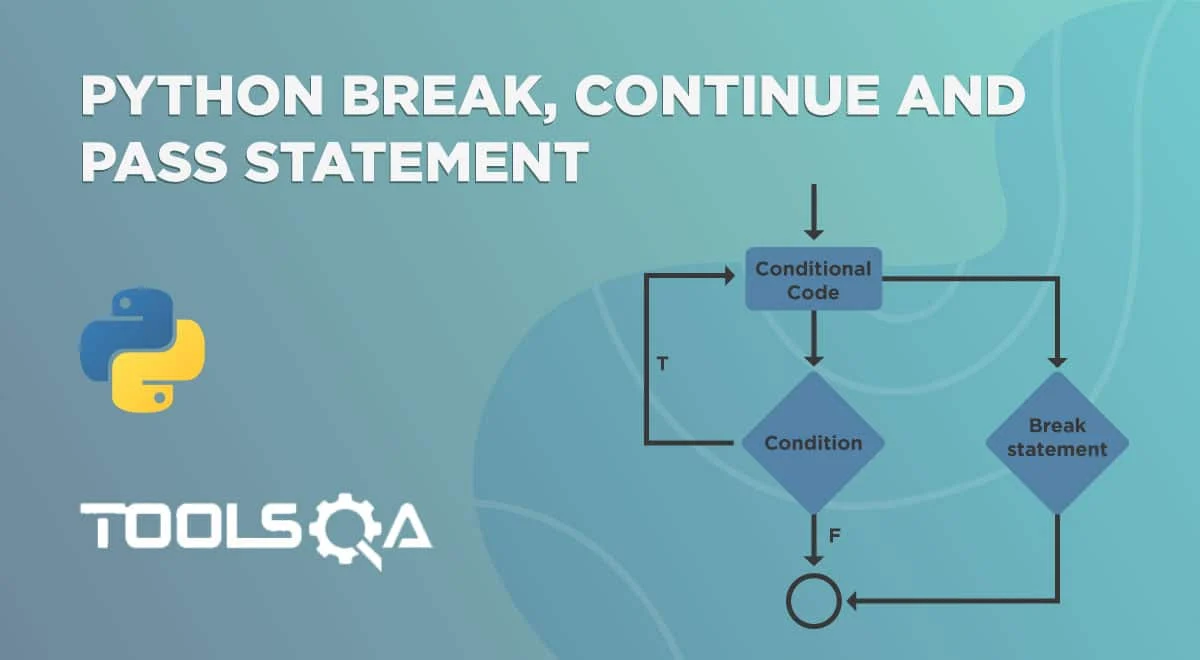
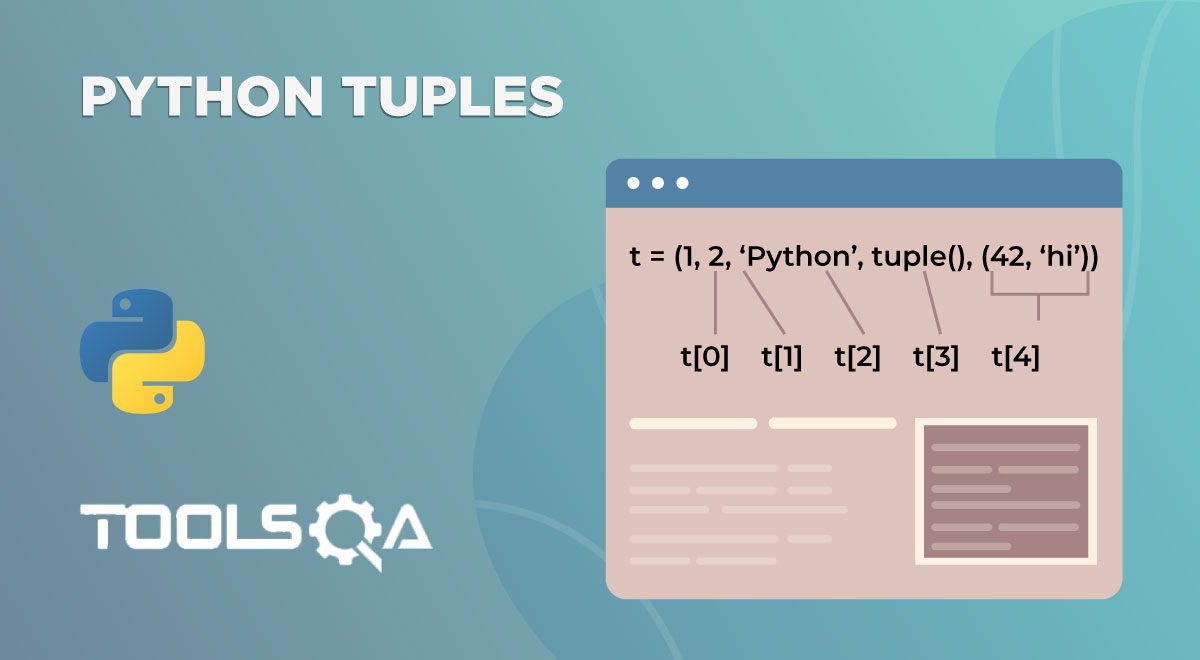