All the object-oriented programming languages such as C++, Java, etc. provides the "this" keyword to refer to an instance of an object from within class definition. But the "this" keyword in JavaScript has varying usage and creates a lot of confusion for someone new to JavaScript. This confusion is because the behavior of "this" in JavaScript is different if we compare it with another programming language, as it always has a different contextual meaning depending on where its put to use. In this article, we will try to overcome this confusion by explaining the details and usage of "this" keyword using the following topics:
- What is this keyword in JavaScript?
- What does "this" in JavaScript refers to when used in a method?
- Moreover, what does "this" refers to when used alone?
- What does "this" in JavaScript refers to when used in a function in default mode?
- Similarly, what does "this" refers to when used in a function in strict mode?
- What does "this" in JavaScript refers to when used in an event?
What is this keyword in JavaScript?
The "this" keyword references the object, which is executing the current function. In another way, every JavaScript function has its reference to its current execution context, called "this". By execution context, we mean "how the function invokes?". The below table shows a 360 view of what all references the "this" keyword can hold:
Usage (Context) | Refers to |
---|---|
In a Method | Owner Object(Which invoked method) |
Alone | Global Object |
In a function (Default Mode) | Global Object |
In a function (Strict Mode) | undefined |
In an event | The element that received the event |
Let's understand all these usage and values of "this" in various contexts:
What does "this" refers to when used in a method?
As we know, if we define functions as properties of objects, they are referred to as methods. If a method uses "this" keyword, it refers to that object and can be used to refer/access the properties of the object.
Let's understand its usage with the help of the following example:
Example:
<html>
<body>
<script> // Create an employee object:
var employee = {
id: 1,
firstName: "Sachin",
middleName: "Ramesh",
lastName: "Tendulkar",
name: function()
{
return this.firstName + " " + this.middleName + " " + this.lastName; ;
}
};
// Display data from the object:
document.write(employee.name());
</script>
</body>
</html>
Save the file with name thisKeywordInMethod.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see the above code and screenshot, we can access the properties of the object using the "this" keyword. So, in this case, the "this" keyword refers to the object, which invokes the method.
What does "this" refers to when used alone?
When we use "this" alone, it refers to the global object, which is an object window in the browser.
Let's understand the usage of "this" with the help of the following example:
Example:
<html>
<body>
<script>
var temp = this;
document.write(temp);
</script>
</body>
</html>
Save the file with name thisKeywordAlone.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
In the above example, we are demonstrating the use of this keyword alone. It refers to object windows, as shown in the output above.
What does "this" refers to when used in a function in default mode?
When we use this in a JavaScript function in default mode, it refers to the global object, which is an object window in the browser. Let's understand the concept with the help of the following example:
Example:
<html>
<body>
<script>
function myFunction() {
return this;
}
document.write("This keyword in function : " + myFunction());
</script>
</body>
</html>
Save the file with the name thisKeywordInFunctionDefaultMode.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see from the above screenshot, the "this" keyword returned by the function refers to the global object. Additionally, it is the "Object Window" in the case of the browser.
What does THIS Keyword in JavaScript refer to when used in a function in strict mode?
As we know that default binding is not allowed in JavaScript's strict mode, So, when we use ‘this’ in JavaScript function in strict mode, it is undefined. Let's understand the concept with the help of the following example:
Example:
<html>
<body>
<script>
"use strict";
function myFunction()
{
return this;
}
document.write("This keyword in function : " + myFunction());
</script>
</body>
</html>
Save the file with name thisKeywordInFunctionInStrictMode.htmland open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see from the above screenshot, the "this" keyword returned from the function prints the "undefined" value when used in a strict mode.
What does THIS Keyword in JavaScript refer to when used in an event?
In the case of event handlers, "this*" refers to the particular HTML element which has received the event. Let's understand the concept with the help of the following example:
Example:
<html>
<body>
<button onclick = “this.style.color=’Red’ ”>Click to Change color !!!</button>
</body>
</html>
Save the file with name thisKeywordInEventHandlers.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see from the above gif image, "this" is referring to the "button" Clicking on the button changes the color of the button to "Red" which we invoke using the "this" keyword for the style of color.
Key Takeaways
- The "this" keyword refers to the object which invoked the method when referenced inside a method
- Additionally, the "this" keyword refers to the global object when used inside a function, but is undefined when invoked in function in strict mode.
- The "this" keyword in Javascript refers to the DOM element when used inside an event fired on the DOM element.
Let's now move to the next article to understand the concept of "prototype" in JavaScript.
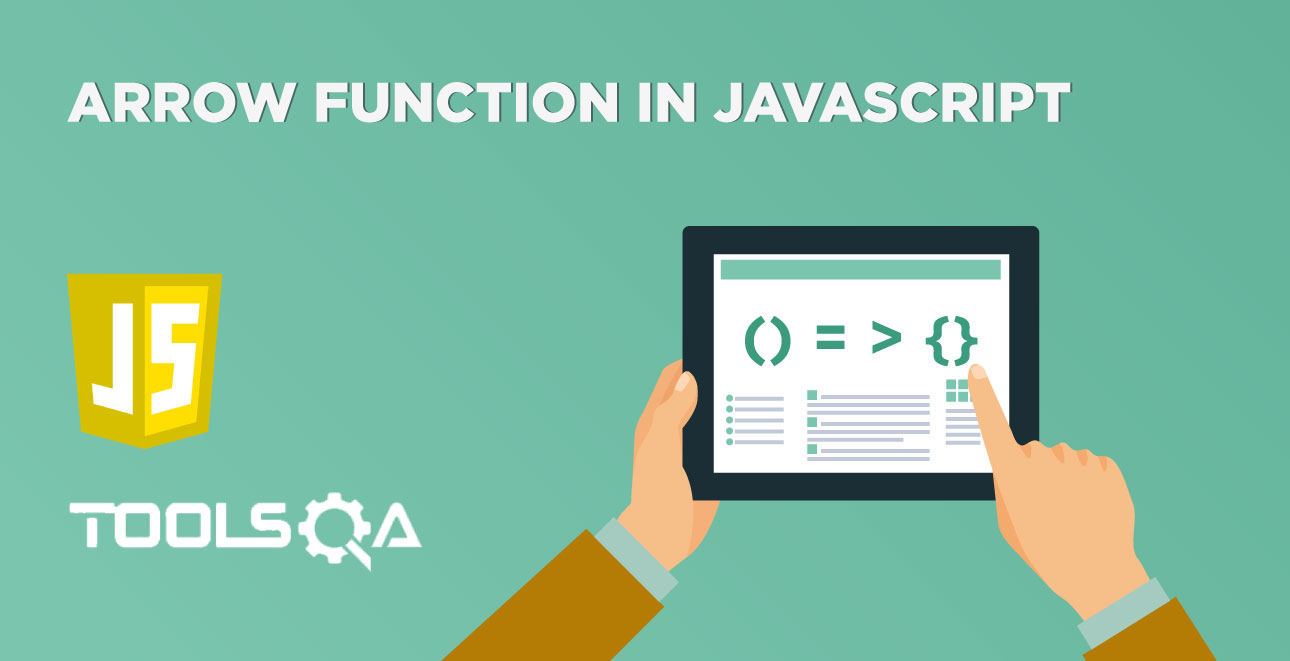
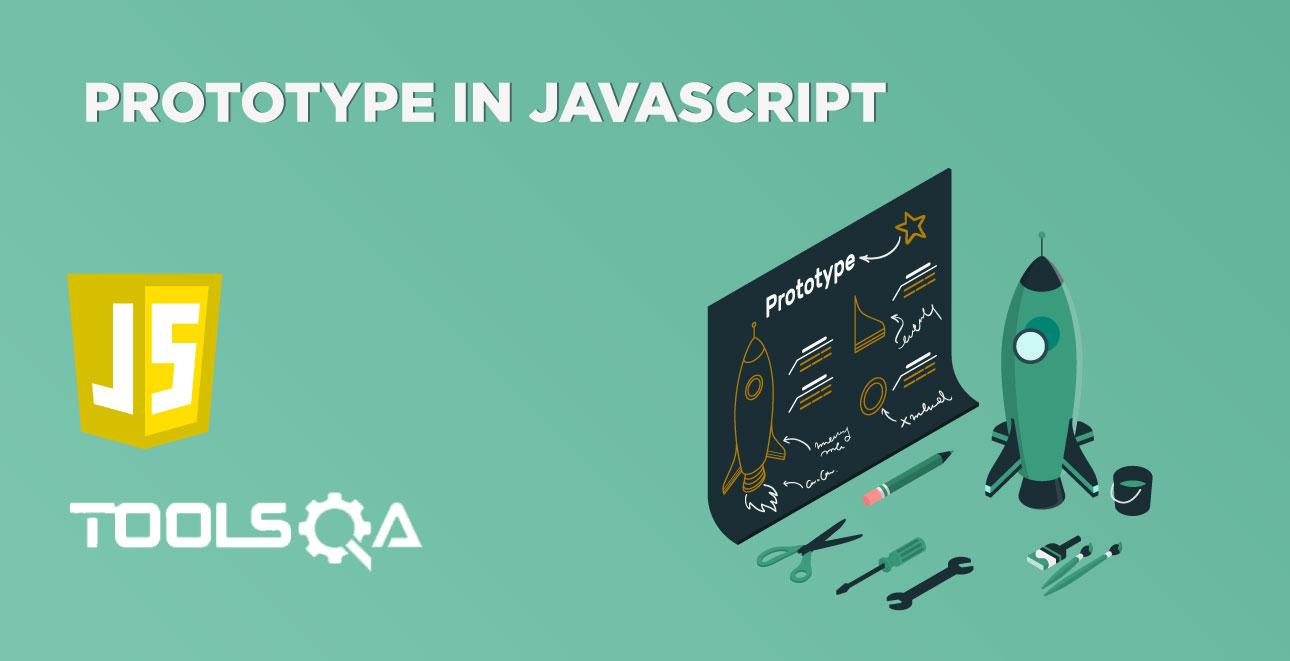