We all should have been browsing various websites and would have noticed that web pages these days are very dynamic and intractable. When a user interacts with an HTML element, it raises an "Event". These interactions can be explicit such as the end-user clicking on a button or link, moving the mouse over certain HTML elements, clicking any of the keyboard keys or implicit such as the web page completing the page load and resizing the browser window. Now, as a programmer, we can execute certain functions when any of these events kick off on any HTML element, and one can achieve it by attaching the event handlers to these events. In this article, we will be covering the following topics to understand the concepts of Event Handlers in JavaScript:
- What are the Event Handlers in JavaScript?
- Additionally, what are the different types of event handlers provided by JavaScript?
What are the Event Handlers in JavaScript?
As discussed, when an event, such as clicking an element or pressing a keyboard key, occurs on an HTML or DOM element, we can invoke certain functions based on these events. So, how do the HTML element knows when to execute the mentioned JavaScript function or JavaScript code? The event handlers handle this. The event handlers are the properties of the HTML or DOM elements, which manages how the element should react to a specific event. The below figure briefs the concept and processing of the event handlers:
As we can see from the above figure, when the user performs a particular mouse or keyword action on the browser, it triggers the corresponding event handler associated with that HTML element. The event handler, in turn, executes a piece of JavaScript code, which performs a particular action on the webpage, and the browser displays the results of those actions to the end-users.
As event handlers are also the properties of the HTML/DOM elements, they can be assigned directly using the equal(=) operator. Its syntax looks like below:
Syntax:
name_of_EventHandler = "JavaScript function/code which need to be executed"
What are the different types of event handlers provided by JavaScript?
JavaScript provides various kinds of event handlers that get triggered based on specific actions on the HTML elements. Few of the event handlers are:
Event Handler | Description |
---|---|
onclick | This event handler invokes a JavaScript code when a click action happens on an HTML element. E.g., when we click a button, a link is pushed, a checkbox checks or an image map is selected, it can trigger the onClick event handler. |
onload | This event handler invokes a JavaScript code when a window or image finishes loading. |
onmouseover | This event handler invokes a JavaScript code when we place the mouse over a specific link or an object. |
onmouseout | This event handler invokes a JavaScript code when the mouse leaves a particular link or an object. |
onkeypress | This event handler invokes a JavaScript code when the user presses a key. |
onkeydown | This event handler invokes a JavaScript code when during the keyboard action, we press the key down. |
onkeyup | This event handler invokes a JavaScript code when during the keyboard action, the key is released. |
Let's understand the usage and details of the few of these event-handlers in the following sections:
What is the OnClick Event Handler in JavaScript?
The onclick event invokes the Javascript code on clicking a button, link, or tag. Its syntax looks like below:
Syntax:
<htmlTag onclick="JavaScript Code need to be executed"></htmlTag>
Let's understand the usage of onClick when we directly embed the javascript code inside the HTML tag itself.
<html>
<meta charset="utf-8"/>
<body> Demonstrating onclick based on DOM object</br>
<input type="button" id="btn" value="Submit Button" onclick="confirm('Are you sure?')" />
</body>
</html>
Save the file with name onClick.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above code snippet, when a user clicks on the submit button, then the onclick event will be triggered, which results in displaying the confirm box.
Let's implement one more example where the event handler invokes a JavaScript method:
<html>
<meta charset="utf-8"/>
<script>
function popop(){
confirm("Are you sure?");
}
</script>
<body> Demonstrating onclick based on DOM object</br>
<input type="button" id="btn" value="Submit Button"
onclick="popop()" />
</body>
</html>
Save the file with name onClick.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we see in the above code, when a user clicks on the submit button, the onclick event will be triggered where it is showing the confirm box. But the JavaScript code which will open the popup is embedded in the popup method and calls for that method when the onclick event is triggered.
What is the OnLoad Event Handler in JavaScript:
The onLoad event executes when a page or a frame or an image is loaded. It initializes some variables when a page is loaded or set some properties when an image is loaded. Its syntax looks like below:
Syntax when a page loads:
<body onload = "JavaScript code need to be executed">
When a frame loads:
<frameset onload="JavaScript code need to be executed">
Syntax when an image loads:
<img src="image need to be loaded" onload = "Javascript code that need to be executed">
Let's understand the usage of onload event handler with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
function logInfo(info){
console.log(info+" onload event is triggerred")
}
</script>
<body onload="logInfo('Page')"> Demonstrating onload based on DOM object</br>
<img src="../MyLogo.jpg" onload="logInfo('image')"/>
</body>
</html>
Save the file with name onLoad.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above example, when the page and the image are loaded, the onload event triggers for both the actions and prints the statement on the browser's console.
What is the OnMouseOver Event Handler in JavaScript:
Just like the onclick event, the onMouseOver event triggers when the user moves a mouse over the particular web element. Its syntax looks like below:
Syntax:
<tag onmouseover=" JavaScript needs to be executed">
Let's understand the usage of onmouseover event handler with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
function over(){
var ele = document.getElementById('btn');
ele.style.background='red';
ele.style.color='white';
}
</script>
<body> Demonstrating onmouseover based on DOM object</br>
<input type="button" id="btn" value="Submit Button" onmouseover="over()" />
</body>
</html>
Save the file with name onMouseOver.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
In the above example, when the user moves the mouse over the submit button, the mouseover event is triggered, and the button's background, the color of the text gets changed.
What is the OnMouseOut event handler?
Just like the onclick event, the onMouseOver event triggers when a user moves a mouse out of the particular web element. Its syntax looks like below:
Syntax:
<tag onmouseout=" JavaScript needs to be executed">
Let's understand the usage of onmouseout event handler with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
function over(){
var ele = document.getElementById('btn');
ele.style.background='red';
ele.style.color='white';
}
function out(){
var ele = document.getElementById('btn');
ele.style.background='Green';
ele.style.color='black';
}
</script>
<body> Demonstrating onmouseout based on DOM object</br>
<input type="button" id="btn" value="Submit Button" onmouseover="over()"
onmouseout="out()" />
</body>
</html>
Save the file with name onMouseOut.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As an example, when a user moves the mouse over the button, then the mouse over event is triggered, and when a user moves the mouse out of that button, then the mouse out event is triggered, i.e., out function is triggered.
What is the OnKeyPress Event Handler?
This event triggers when a user presses any keyboard key. Its syntax looks like below:
Syntax:
<tag onkeypress = "Javascript that needs to be executed">
Let's understand the usage of onkeypress event handler with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
function press(){
var ele = document.getElementById('input');
ele.style.background='red';
ele.style.color='white';
}
</script>
<body> Demonstrating onkeypress based on DOM object</br>
<input type="text" id="input" onkeypress="press()"/>
</body>
</html>
Save the file with name onKeyPress.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above example when a user presses a keyboard key in the text field, the onkeypress event is triggered, and the press() function executes.
What is the OnKeyDown Event Handler?
This event triggers when a user presses any keyboard key down. Its syntax looks like below:
Syntax:
<tag onkeydown = "Javascript that needs to be executed">
Let's understand the usage of onkeydown event handler with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
function press(){
var ele = document.getElementById('input');
ele.style.background='red';
ele.style.color='white';
}
</script>
<body> Demonstrating onkeydown based on DOM object</br>
<input type="text" id="input" onkeydown="press()"/>
</body>
</html>
Save the file with name onKeyDown.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above example, when a user presses any key down in the textbox, it triggers the keydown event, which in turn invokes the press function.
What is the OnKeyUp Event Handler?
This event is similar to the onkeydown event but triggered when the user releases the pressed key. Its syntax looks like below:
Syntax:
<tag onkeyup = "Javascript that needs to be executed">
Let's understand the usage of onkeyup event handler with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
function press(){
var ele = document.getElementById('input');
ele.style.background='red';
ele.style.color='white';
}
function up(){
var ele = document.getElementById('input');
ele.style.background='green';
ele.style.color='black';
}
</script>
<body> Demonstrating onkeyup based on DOM object</br>
<input type="text" id="input" onkeydown="press()" onkeyup="up()"/>
</body>
</html>
Save the file with name onKeyUp.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above example when the user presses a key in the text box, then it will invoke press function, and when a user releases a key, then the up function will be invoked.
Apart from the event as mentioned above handler's, the list is very exhaustive. As a developer, we can use any of those based on what action needs to perform on a particular action on an HTML element.
Key Takeaways
- Event handlers are the JavaScript code that invokes a specific piece of code when a particular action happens on an HTML element.
- The event handler can either invoke the direct JavaScript code or a function.
- One HTML tag can contain multiple event handlers and will invoke the mentioned code depending on the event/action that happens on the HTML element.
Let's move to the next article to understand one more important concept of JavaScript: "Regex Expressions in JavaScript".
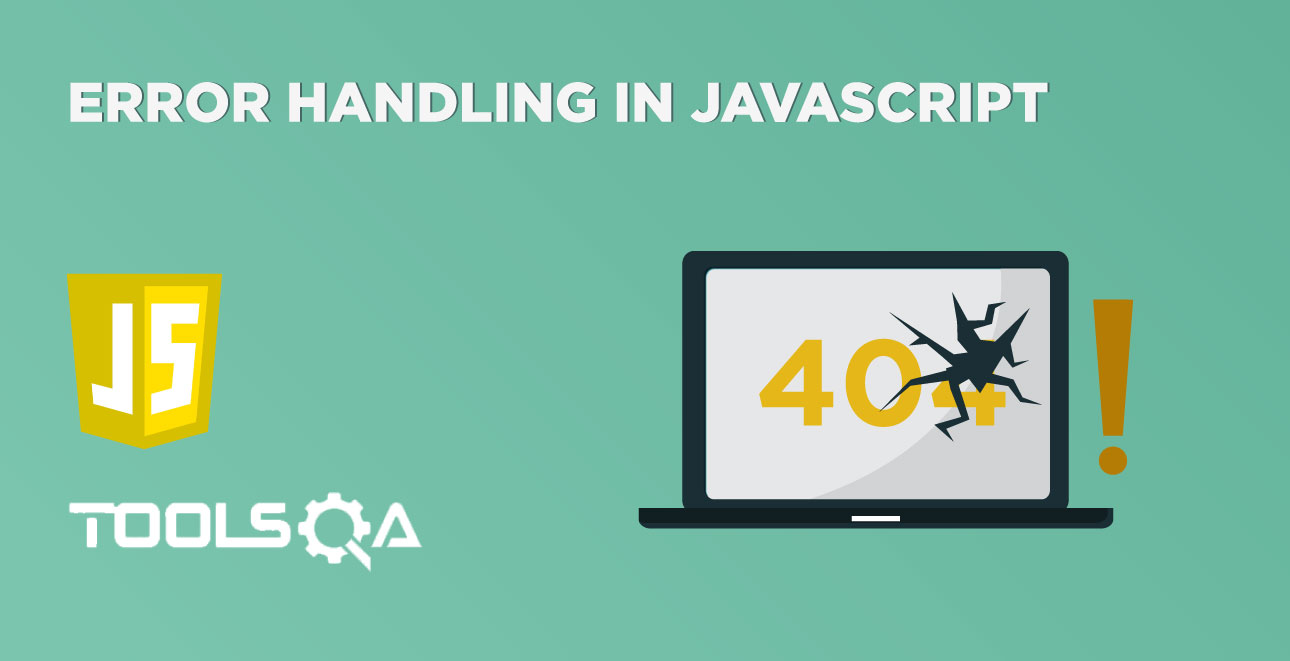
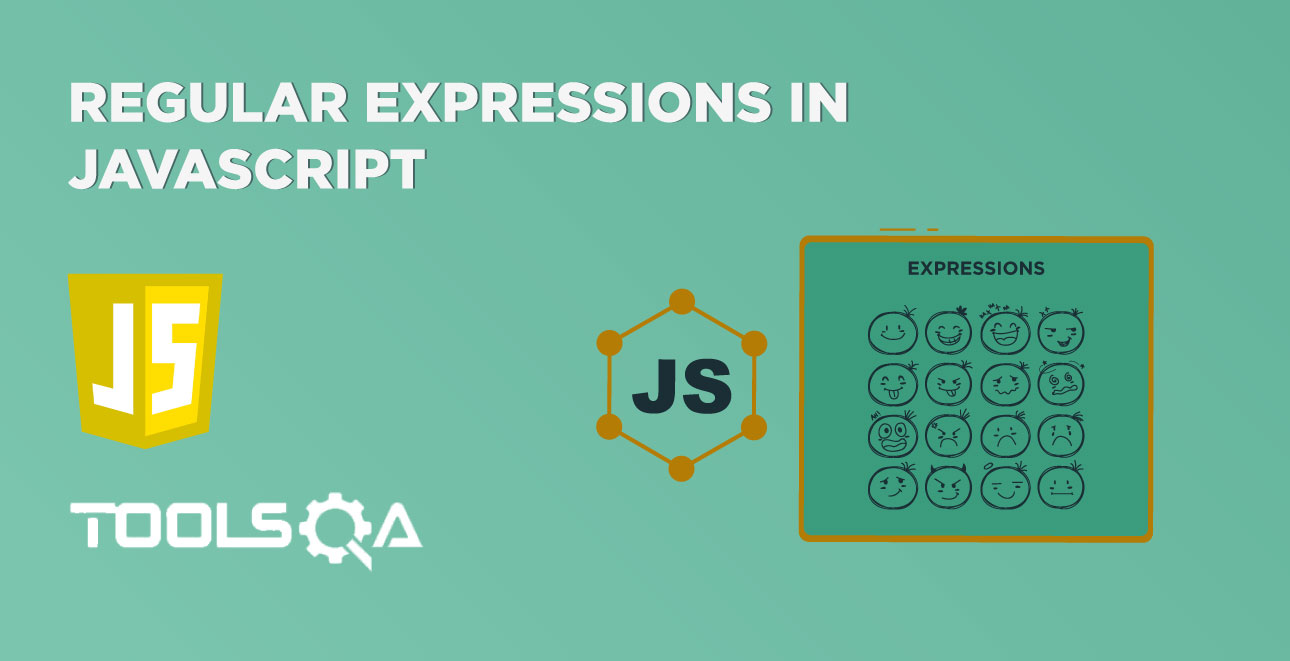