Consider a scenario when you need to assign the array values or properties of an object to individual variables. Wasn't that a tedious task to access and assign each value of the array or object and then assign to the individual variable. Destructuring has made it very easy in JavaScript. It was introduced in ES6 and has proved helpful in extracting values from objects and arrays. In this tutorial, we will be focusing on below topics related to "JavaScript Destructuring":
- What is Destructuring in JavaScript?
- What are the different ways of Destructuring in JavaScript?
- How to assign variables from an object using Destructuring?
- How to assign variables from the arrays using Destructuring?
- What are the different ways of Destructuring in JavaScript?
What is Destructuring in JavaScript?
Destructuring is a new feature in JavaScript, which introduced in ES6 2015. It breaks apart the structured stuff like objects or arrays into the variables.
Sometimes your cup is overflowing, and all you want is a sip. It is where destructuring comes into play. Say you have the following data structure:
In the above data structure, if you only needed the Hobbit's name and family, you can achieve the same in two ways. Firstly, either you could drill into the data structure to obtain them and access them using the dot(.) operator. Or you could extract the needed values (in this case name and family) in local variables using destructuring.
Let's understand the details of destructuring with the help of the following code snippet:
var vehicle = {
type:'car',
wheels:4,
engine:'4 cylinder'
}
In the above example, we have a vehicle object with three properties. Let's assign any two properties to local variables in a traditional way:
const type = vehicle.type;
const engine = vehicle.engine;
Now, don't you feel that creating multiple variables in multiple lines and calling all objects variables to assign is as good as using objects itself instead of doing this? Let's try to achieve the same more straightforwardly with the help of destructuring, as shown below:
const {type, engine} = vehicle;
The above line will do the same work as assigning the variables in a normal way but an efficient manner. We are destructuring the vehicle object into two local variables that are type and engine. When we do this, the properties type and engine are assigned to local variables type and engine, giving us the ability to access them.
What are the different ways of Destructuring in JavaScript?
There are multiple ways in which destructuring can unpack the values from an object or an array. Let's understand a few of them in the following sections:
How to assign variables from an object using Destructuring?
Destructuring on objects allows you to bind variables to different properties of an object. JavaScript provides two ways to destructure an object:
1.Destructuring an object with the same property names and variable names: One way to destructure an object is when the object property names and the local variable names are the same. Let's understand the details with the help of the following example:
<html>
<body> Demonstrating destructuring object with same property and variable name</br>
<script type="text/javascript">
var student = {
name:'Arun',
room:'4',
course:'BE',
fees:50000
}
var {name,room, course,fees} = student;
console.log(name);
console.log(room);
console.log(course);
console.log(fees);
</script>
</body>
</html>
Save the file with name destructuringObject.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see, in the above program, we see the properties of a student are destructured and assigned to a local variable of the same name as were there in the Object properties.
2. Destructuring objects with the same property names but different variable names. Another way to destructure an object is when the object property names and the local variable names are different. Let's understand the details with the help of the following example:
<html>
<body> Demonstrating destructuring object</br>
<script type="text/javascript">
var student = {
name:'Arun',
room:'4',
course:'BE',
fees:50000
}
var {name: w,room: x, course:y,fees:z} = student;
console.log(w);
console.log(x);
console.log(y);
console.log(z);
</script>
</body>
</html>
Save the file with name destructuringObject.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As seen in the above program, the properties of a student are destructured and assigned to a local variable with a different name. Where the name property is assigned to the local variable "w" and so on.
3. Destructuring a nested object: There can be scenarios when one object nests inside another object. JavaScript provided the functionality to destructure a nested object also. Let's look at the below example to understand how to destructure the nested objects:
<html>
<body> Demonstrating destructuring nested object</br>
<script type="text/javascript">
var student = {
name:'Arun',
room:'4',
course:'BE',
fees:50000,
address:{
city:'Bangalore',
state:'karnataka',
country:'india'
}
}
var {address:{city,state,country}} = student;
console.log(city);
console.log(state);
console.log(country);
</script>
</body>
</html>
Save the file with name destructuringNestedObject.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above code snippet and screenshot, we are destructuring the address object, which nests inside the student object.
How to assign variables from the arrays using Destructuring?
Similar to the objects, we can destructure the arrays also. JavaScript provides following ways to destructure arrays:
1.Destructure arrays using basic ordered restructuring: Until now, we have seen how to destructure the objects. In the same manner, we can destructure the arrays also. One of the easy ways to destructure the arrays is the basic ordered assignment, where the array elements will assign to the declared variables from the start. Let's understand the usage with the help of following code snippet:
<html>
<body> Demonstrating destructuring Array</br>
<script type="text/javascript">
var[name1,name2] = ['Arun','Kumar','Tools','QA'];
console.log(name1);
console.log(name2);
</script>
</body>
</html>
Save the file with name destructuringArrays.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above example, the array elements directly assign to the local variables. As we have created only two variables, so only the first two values of the array were assigned to the variables.
2. Destructure arrays by skipping array elements: If we want to assign specific values of the array to the variables, then we can destructure the array by skipping the indexes which are not needed to assign to the variables. Let's understand with the help of the following example, how to achieve the same:
<html>
<body> Demonstrating destructuring Array</br>
<script type="text/javascript">
var[name1,,,name2] = ['Arun','Kumar','Tools','QA'];
console.log(name1);
console.log(name2);
</script>
</body>
</html>
Save the file with name destructuringArrays.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
In the above example, we can see that by using commas, we can skip as many array indexes we want to move further and then assign the needed values to the variables.
Key Takeaways
- Destructuring is one of the essential and unique JavaScript features which help to breakdown the arrays and objects into a local variable in a more efficient way.
- Destructuring can happen on objects, nested objects, and Arrays.
Let's move to the next article where we will be covering the details of Rest and Spread operators in JavaScript.
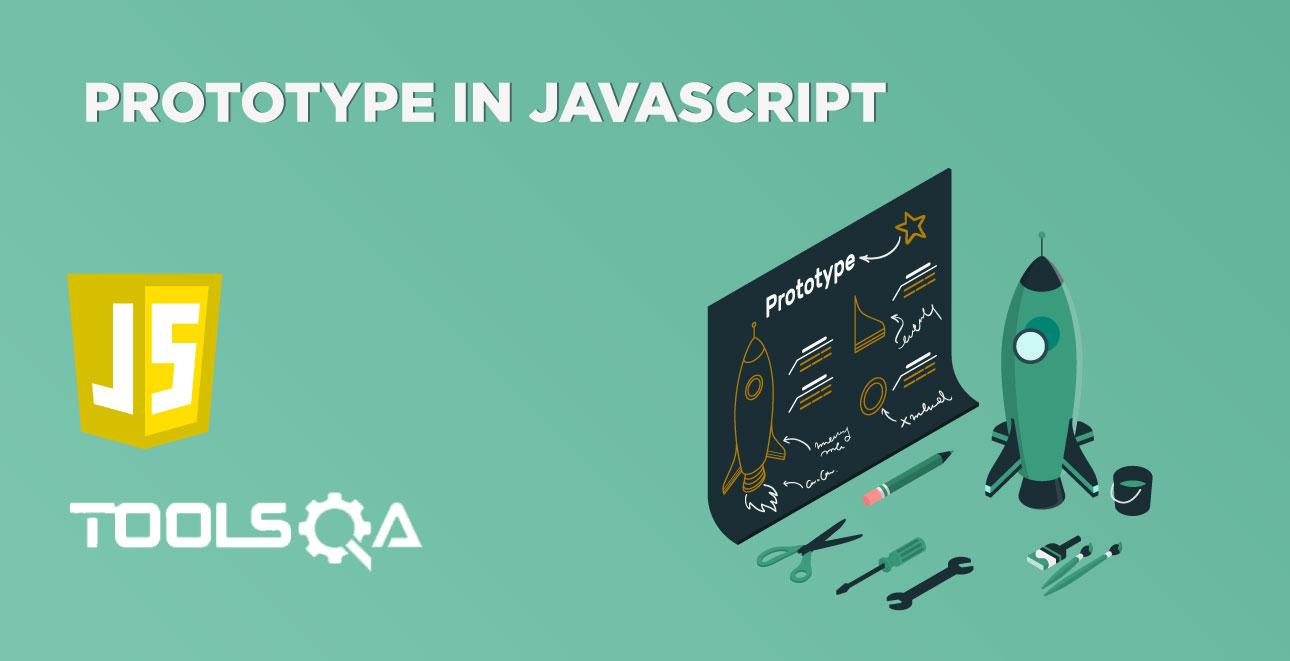
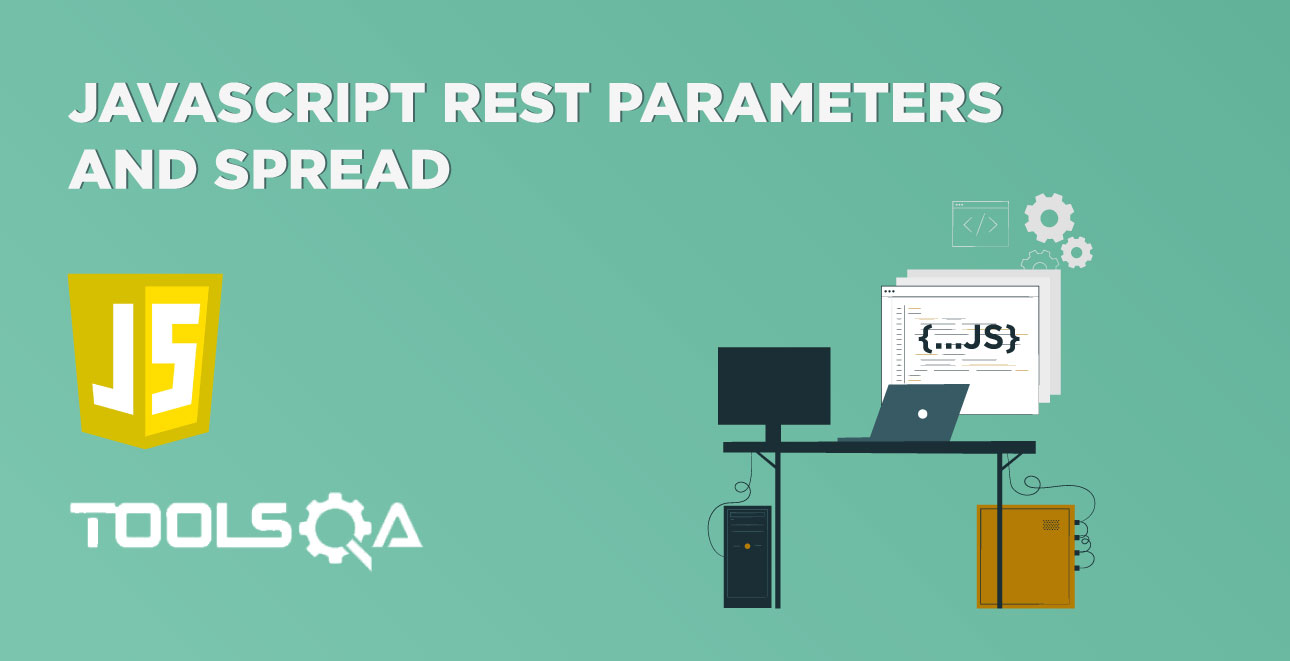