A prototype is an existing inbuilt functionality in JavaScript. Whenever we create a JavaScript function, JavaScript adds a prototype property to that function. A prototype is an object, where it can add new variables and methods to the existing object. i.e., Prototype is a base class for all the objects, and it helps us to achieve the inheritance. In this article, we will cover the following aspects of the JavaScript Prototype:
- What is the Prototype in JavaScript?
- When to use the prototype in JavaScript?
- How to add variables to an object using the prototype in JavaScript?
- How to add methods to the object using the prototype in JavaScript?
What is the Prototype in JavaScript?
As discussed, a prototype is an inbuilt object where it associated with the functions by default, which can be accessible, modifiable, and create new variables and methods to it and share across all the instances of its constructor function.
When to use Prototype in JavaScript?
As we all know, Javascript is a dynamic language where we can add new variables and methods to the object at any point in time, as shown below.
function Employee() {
this.name = 'Arun';
this.role = 'QA';
}
var empObj1 = new Employee();
empObj1.salary = 30000;
console.log(empObj1.salary); // 15
var empObj2 = new Employee();
console.log(empObj2.salary); // undefined
As we see in the above example, the salary variable adds to the empObj1. But when we try to access the salary variable using the empObj2 object, it doesn't have the salary variable because the salary variable is defined only in the empObj1 object.
Now comes the question, Can there be a way that the new variable can be added to the function itself so as it is accessible to all the objects created using the function?
The answer to this question is the use of a prototype. A prototype is an invisible inbuilt object which exists with all the functions by default. The variables and methods available in the prototype object can be accessible, modifiable, and even can create new variables and functions.
We can attach a new variable or method to the object using proto keyword, as shown below:
Now, let's see some practical implementations of how to add new variables and methods using the prototype functionality:
How to add variables to an object using the Prototype in JavaScript?
As discussed, there can be a time when we need to add the new variables to an existing object, which is almost impossible in other programming languages. But in javascript, we can achieve with the help of the prototype. It follows the following syntax for adding a new variable:
Syntax:
ClassName.prototype.variableName = value;
Let's understand the usage of "prototype" to add a new variable with the help of the following example:
Example:
<html>
<body>
Demonstrating prototype for variables in javascript
</br>
</br>
<script type = "text/javascript">
function details(){
this.website="Tools QA",
this.language="Java Script"
}
var tutorails = new details();
document.write(tutorails.website);
document.write("</br>");
document.write(tutorails.language);
details.prototype.Author = "Arunkumar Chandra";
document.write("</br>");
document.write(tutorails.Author);
</script>
</body>
</html>
Save the file with name prototypeVariable.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as
As we can see from the above example, we added a new variable "Author" to the existing function "details", and the same was accessible on the object "tutorials" later on.
How to add methods to the object using the Prototype in JavaScript?
Similar to the variables, sometimes, we need to add the methods for the existing object. The same can be achieved in JavaScript using the prototype functionality. Its syntax looks like below:
Syntax:
className.prototype.functionname = ()=>{
};
Let's understand the usage of "prototype" to add a new method with the help of the following example:
Example:
<html>
<body>
Demonstrating prototype for method in javascript
</br>
</br>
<script type = "text/javascript">
function details(){
this.website="Tools QA",
this.language="Java Script"
}
var tutorails = new details();
document.write(tutorails.website);
document.write("</br>");
document.write(tutorails.language);
details.prototype.Author = ()=>{
return "Arunkumar Chandra";
};
document.write("</br>");
document.write(tutorails.Author());
</script>
</body>
</html>
Save the file with name prototypeMethods.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
In the above example, We are adding the "Author" method after the object is created and invoked it on the existing object "tutorials".
So, this way, we can add new variables and methods to the existing objects dynamically, which will be accessible to all the objects created using the function.
Key Takeaways
- A prototype is an object which associates with all the functions. Additionally, it is invisible, but all the properties inside the prototype are accessible.
- When a programmer needs to add new properties like variables and methods at a later point of time, and these properties need sharing across all the instances, then the prototype will be very handy.
- The prototype can add both variables and methods to an existing object dynamically.
Let's now move to the next article to understand some of the advanced features provided by JavaScript, such as Destructuring.
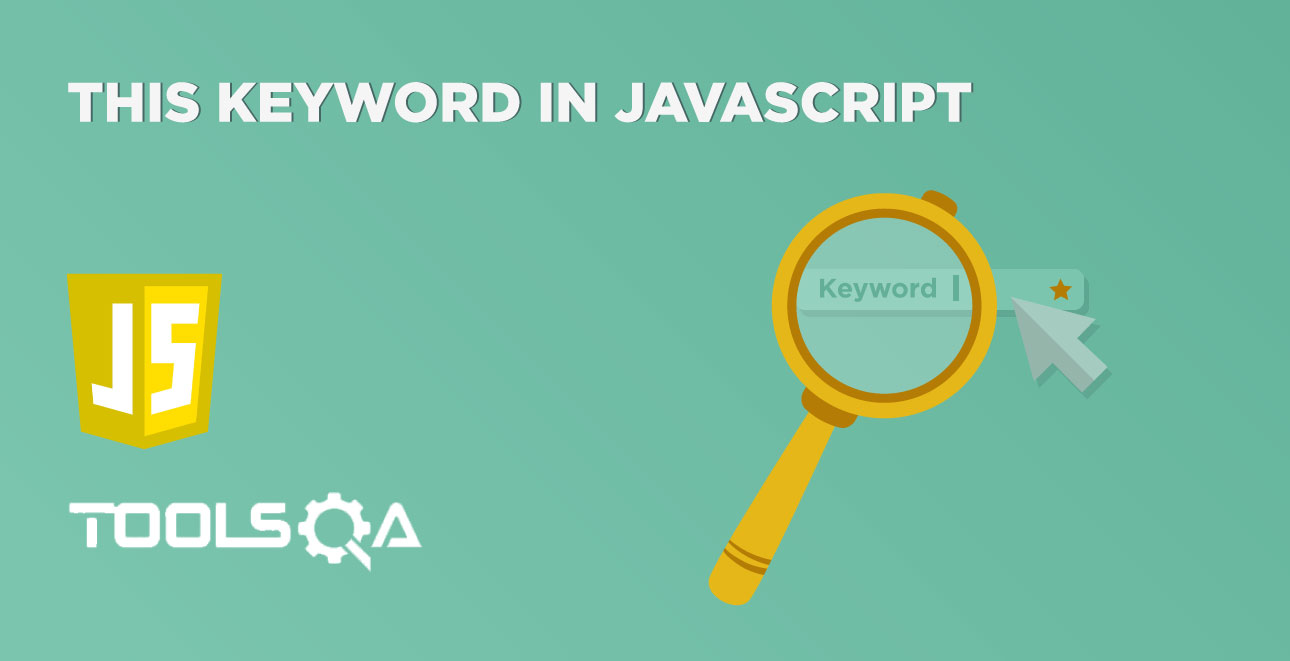
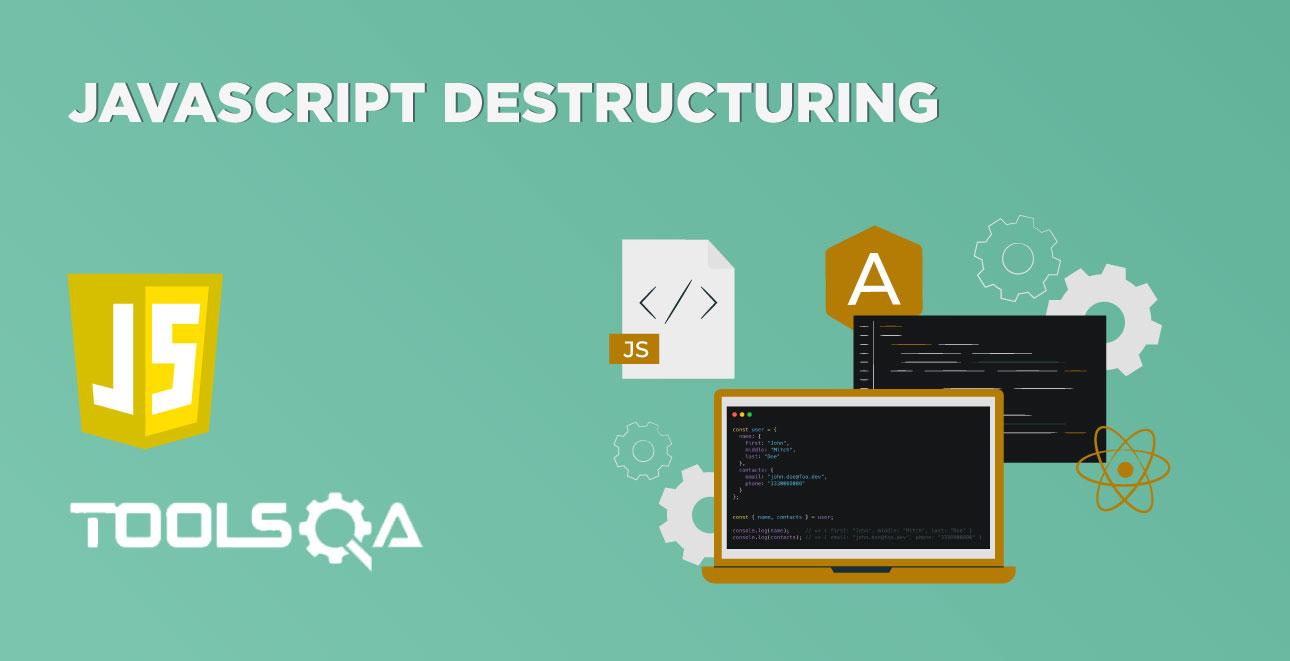