During the development cycle, many of us would have faced the situation when we need to search or replace a particular string in a larger string. Still, the search string is not a predefined set of fixed characters but is an expression that can match multiple strings in the larger string. Additionally, one can specify this kind of search criteria using "Regex", also known as Regular Expressions. Moreover, a regular expression can either be a single character or a series of characters. We will understand the details of Regular Expressions in JavaScript by covering the following topics:
- What are Regular Expressions in JavaScript?
- How to define Regular Expressions in Javascript?
- How to evaluate Regular Expressions in Javascript using various methods?
- What are the different ways of writing a Regular Expression in Javascript?
What are Regular Expressions in JavaScript?
A JavaScript Regular Expression is an object, which specifies a pattern of characters. Moreover, a typical example of a regular expression implementation is the find and replace functionality provided by all the text editors. Apart from it, one can use regex in the below scenarios:
- First, we can split the string into multiple strings.
- Second, we can use it to replace a particular word in the given string.
- Thirdly, we can use it to validate text fields based on the pattern.
JavaScript provides specific ways to create the Regular Expression object. Let's understand the details of the same in the following section:
How to define Regular Expressions in Javascript?
JavaScript* provides two approaches to define a regular expression object:
- Firstly, defining a regular expression object using a regular expression literal.
- Secondly, defining a regular expression using a regex object.
Let's understand the detailed implementation of both of these approaches in the below sections:
Defining a Regular Expression Object using a Regular Expression Literal:
In this approach of implementing a regular expression, the pattern comes between the forward slashes. Moreover, its syntax looks like below:
Syntax:
let variableName = /RegExp/
Subsequently, let's understand the implementation details of the regular expression using a regular expression literal with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
let reg=/toolqa/
console.log(reg.test("Welcome to toolqa"))
</script>
<body> Demonstrating regular expression with the help of expression literal</br>
</body>
</html>
Save the file with name regExpLit.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
As we see in the example, we created regex "/toolqa/", which will match whether the toolqa text is present in the given string.
Note: The test method validates a regex. Additionally, we will cover the details in the following sections.
Defining a Regular Expression using a Regex object:
Another way of creating a regex in JavaScript is with the help of the RegExp object. Moreover, its syntax looks like below:
Syntax:
let variableName = new RegExp('Regex Expression')
Let's understand the implementation details of the regular expression using a RegExp object with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
let reg=new RegExp('toolqa')
console.log(reg.test("Welcome to toolqa"))
</script>
<body> Demonstrating regular expression with the help of RegExp object</br>
</body>
</html>
Save the file with name regExpObj.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
As we see in the above example, we created a regex variable with the help of the RegExp object. Additionally, it will match based on whether the toolqa text is present in the given string.
What methods does JavaScript provide for evaluating a Regular Expression?
JavaScript regular expression object provides two methods: test() and exec() to evaluate a regular expression. Subsequently, let's understand the usage of both of these methods in the following sections:
How to use the test() method to evaluate a regular expression?
The "test" method evaluates the regular expression. In addition to that, it returns a boolean value based on whether the regular expression resulted in a successful result or not. Moreover, its syntax looks like below:
Syntax:
let variableName = /Pattern/;
variableName.test("String data")
or
let variableName = new RegExp("Pattern");
variableName.test("String data")
Subsequently, let's understand the usage of the "test()" method with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
let reg = /T..l/ // This represent a single character
console.log('T..l Metacharacter for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
</script>
<body> Demonstrating regular expression test method</br>
</body>
</html>
Save the file with name regExpTest.html. After that, open it in any browser (Chrome, Firefox, or IE). As a result, it should show the output as:
As we can see in the above example, the test method resulted in true. Because the regular expression resulted in a successful match.
How to use the exec() method to evaluate a regular expression?
Similar to the "test()" method, the "exec()" method is used to evaluate the regular expression. Moreover, it returns an array of Strings based on the successful match of the regular expression. Additionally, its syntax looks like below:
Syntax:
let regObj = /Pattern/
regObj.exec( string );
or
let regObj = new RegExp("Pattern")
regObj.exec( string );
Subsequently, let's understand the usage of the "exec()" method with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
let reg = /T..l/ // This represent a single character
console.log('T..l Metacharacter for String ToolsQAT')
console.log(reg.exec("ToolsQAT"))
</script>
<body> Demonstrating regular expression exec method</br>
</body>
</html>
Save the file with name regExpExec.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
As we see in the above example, the exec method will provide the array string which is matching with the given pattern.
What are the different ways of writing a Regular Expression in Javascript?
JavaScript provides various ways of writing regular expressions. Moreover, all these methods or techniques can be combined with each to define a unique regular expression. Subsequently, let's understand the usage of all of these methods in the following sections:
How to use brackets [] to define a regular expression?
Regular expressions can use square brackets [] to define a condition for regular expressions. Additionally, its syntax looks like below:
Syntax:
[RegExp Conditions]
The table below shows different ways in which we can use the square brackets to define a regular expression:
Expression | Description |
---|---|
[ ] | It will match any of the characters inside the brackets. |
[^ ] | It will match any of the characters which are not in the brackets. |
[A-Z] | Matches any character between Uppercase A to uppercase Z. |
[a-z] | It will match any character between lowercase a to lowercase z. |
[0-9] | It will match any character between 0 to 9. |
Subsequently, let's understand the usage of these bracket syntax with the help of following code snippet:
<html>
<meta charset="utf-8"/>
<script>
let reg=/[toolsqa]/; //Mathcing any one character
console.log('Matching any character available in given string')
console.log(reg.test("TOOLSqA"))
reg=/[^toolsqa]/; //checking any characters is available apart from regex exp
console.log('Checking there is character in a string which is not available in regexp')
console.log(reg.test("toolsqa"))
reg=/[a-z]/; //Matches any character from lowercase a to lowercase z
console.log('Checking given string is in lowercase letter')
console.log(reg.test("toolsqa"))
reg=/[A-Z]/ //Matches any character from uppercase A to uppercase Z
console.log('Checking given string is in uppercase letter')
console.log(reg.test("TOOLSqa"))
reg=/[0-9]/ //Matches any character from 0 to 9
console.log('Checking given string is in any number')
console.log(reg.test("TOOLSqa123"))
</script>
<body> Demonstrating regular expression Brackets</br>
</body>
</html>
Save the file with name regExpBrackets.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
Let's understand the above example with details:
- At line number 4 (let reg = /[toolsqa]/) means we are creating the regex expression as match any character in the given string(TOOLSqA) contain anyone the of character in the expression. That is to say, in given string q contains as per the expression.
- Similarly, at line number 7 (reg = /[^toolsqa]/) means given string (toolsqa) should not contain any characters given in the expression (toolqa). Since it contains characters available in the expression output is false.
- At line number 10 (reg=/[a-z]/) means given string (toolsqa) should contains at least one lowercase character between a-z.
- Similarly, at line number 13 (reg = /[A-Z]/) means given string (TOOLSqa) should contains at least one uppercase character between a-z.
- At line number 16 (reg=/[0-9]/) means given string (TOOLSqa123) should contains at least one number between 0-9.
How to use Quantifiers to define a regular expression?
Regular expressions can use quantifiers to define a condition for regular expressions. Quantifiers provide a frequency or position of the bracketed characters. The below table shows various usage of ** to define a regular expression:
Quantifiers | Description |
---|---|
T+ | It will match any string containing T character one or more times. |
T* | It will match any string containing T character zero or more times. |
T? | It will match any string containing T zero or one time. |
T{N} | It will match any string containing T character consecutively N times |
T{4,7} | It will match any string containing T character consecutively 4 or 7 times. |
T{4,} | It will match any string containing T character consecutively at least four times. |
T$ | It will match any string containing T character at the end of a string |
^T | It will match any string containing T character at the starting of the string |
Let's understand the usage of Quantifiers with the help of the following example:
<html>
<meta charset="utf-8"/>
<script>
let reg = /T+/ // this quantifier is used to match string contains one or more T
console.log('T+ Quantifier for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
console.log('T+ Quantifier for String oolsQA')
console.log(reg.test("oolsQA"))
reg = /T*/ // this quantifier is used to match string contains zero or more T
console.log('T* Quantifier for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
console.log('T* Quantifier for String oolsQA')
console.log(reg.test("oolsQA"))
reg = /T$/ // this quantifier is used to match string contains T at end of the string
console.log('T$ Quantifier for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
console.log('T$ Quantifier for String ToolsQA')
console.log(reg.test("ToolsQA"))
reg = /^T/ // this quantifier is used to match string contains T at Starting of the string
console.log('^T Quantifier for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
console.log('^T Quantifier for String oolsQAT')
console.log(reg.test("oolsQAT"))
reg = /T?/ // this quantifier is used to match string contains T zero or one
console.log('T? Quantifier for String ToolsQA')
console.log(reg.test("ToolsQA"))
console.log('T? Quantifier for String oolsQA')
console.log(reg.test("oolsQA"))
reg = /T{3}/ // this quantifier is used to match string contains T consecutively 3 times
console.log('T{3} Quantifier for String TTToolsQA')
console.log(reg.test("TTToolsQA"))
console.log('T{3} Quantifier for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
reg = /T{1,3}/ // this quantifier is used to match string contains T consecutively 1 or 3 times
console.log('T{1,3} Quantifier for String TTToolsQA')
console.log(reg.test("TTToolsQA"))
console.log('T{1,3} Quantifier for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
reg = /T{2,}/ // this quantifier is used to match string contains T consecutively atleast 2 times
console.log('T{1,3} Quantifier for String TTToolsQA')
console.log(reg.test("TTToolsQA"))
console.log('T{1,3} Quantifier for String ToolsQA')
console.log(reg.test("ToolsQA"))
</script>
<body> Demonstrating regular expression Quantifiers</br>
</body>
</html>
Save the file with name regExpQuantifiers.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
As we can see in the above screenshot, the test() method returns true/false based on the condition specified by the Quantifiers.
How to use Metacharacters to define a regular expression?
Regular expressions can use metacharacters to define a condition for regular expressions. The below table shows various usage of Metacharacters to define a regular expression:
Metacharacter | Description |
---|---|
. | It will match a single character |
\s | It will match spaces like Tab, space or newline |
\S | It will match a non-space character. |
\d | It will match a numerical value. |
\D | It will match a non-numerical value. |
\w | It will match a word character. In other words, an alphanumeric value. |
\W | It will match a non-word character or special characters |
Subsequently, let's understand the usage of Metacharacters with the help of the following example:
<html>
<meta charset="utf-8"/>
<script>
let reg = /T..l/ // This represent a single character
console.log('T..l Metacharacter for String ToolsQAT')
console.log(reg.test("ToolsQAT"))
reg = /\s/ //This is for white space or tab or new line
console.log('/\s/ Metacharacter for String ToolsQA')
console.log(reg.test("ToolsQA"))
console.log('/\s/ Metacharacter for String Tools QA')
console.log(reg.test("Tools QA"))
reg = /\S/ //This is for non white space or tab or new line
console.log('/\S/ Metacharacter for String ToolsQA')
console.log(reg.test("ToolsQA"))
console.log('/\S/ Metacharacter for String <space> ')
console.log(reg.test(" "))
reg = /\d/ //This is for only digits
console.log('/\d/ Metacharacter for String 123test123')
console.log(reg.test("123test123"))
console.log('/\d/ Metacharacter for String ToolsQA')
console.log(reg.test("ToolsQA"))
reg = /\D/ //This is for only non digits
console.log('/\D/ Metacharacter for String 12345')
console.log(reg.test("12345"))
console.log('/\D/ Metacharacter for String ToolsQA')
console.log(reg.test("ToolsQA"))
reg = /\w/ //This is for only alpha numeric
console.log('/\w/ Metacharacter for String ToolQA123')
console.log(reg.test("ToolsQA123"))
console.log('/\w/ Metacharacter for String ++/;[]')
console.log(reg.test("++/;[]"))
reg = /\W/ //This is for only non alpha numeric
console.log('/\w/ Metacharacter for String ToolQA123')
console.log(reg.test("ToolsQA123"))
console.log('/\w/ Metacharacter for String ++/;[]')
console.log(reg.test("++/;[]"))
</script>
<body> Demonstrating regular expression Metacharacters</br>
</body>
</html>
Save the file with name regExpMeta.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
As we can see in the above screenshot, the test() method returns true/false based on the condition specified by the Metacharacters.
How to use Modifiers to define a regular expression?
Regular expressions can use modifiers to define a condition for regular expressions. Additionally, modifiers simplify the use of regexp by some functionalities like case sensitivity, global search, and searching in multiple lines. The below table shows various usage of Modifiers to define a regular expression:
Modifier | Description |
---|---|
i | It will evaluate the regular expression by ignoring case sensitivity. |
m | It will evaluate the regular expression by considering new string present in new lines also. |
g | It will evaluate the regular expression by performing a global match instead of stopping at the first match. |
Let's understand the usage of Modifiers with the help of the following example:
<html>
<meta charset="utf-8"/>
<script>
let reg= /toolsqa/i
console.log(" i modifiers is used to ignore case")
console.log(reg.test("TOOLSQA"));
reg = /qa/m
console.log(" m modifer is used to match even in new line")
console.log(reg.exec("ToolsQA \n i am in new line qa"))
reg = new RegExp("qa","g");
console.log(" g modifer is used to match all the string compared to expression")
console.log(reg.exec("Tools qa is a qa site"))
console.log(reg.exec("Tools qa is a qa site"))
console.log(reg.exec("Tools qa is a qa site"))
</script>
<body> Demonstrating regular expression modifiers</br>
</body>
</html>
Save the file with name regExpModifiers.html. After that, open it in any browser (Chrome, Firefox, or IE). Consequently, it should show the output as:
As we can see from the above screenshot:
- For the first case, the test method returned true, even if the case of the string was different than the regular expression, as we used the modifier "i" in the regular expression.
- In the second case, where we used the modifier "m", it returned the result even by searching the string in the next line.
- Similarly, for the third case, where we used the modifier "g", the exec() method returned the next index of "qa" each time invoked. Therefore, the first time it returned index 6, next time it returned index 14 and next time it returned "null", as no further occurrences of the search criteria exist in the string.
Key Takeaways
- Firstly, a regular expression is a sequence of characters that define a search pattern.
- Secondly, Regex will help to match the texts based on the pattern.
- Thirdly, a regular expression can be defined either using literal(/) or the RegExp object.
- Also, the Quantifier, Metacharacters, and Modifiers modify the way regular expressions are defined. Additionally, we can also use them to customize the search strings.
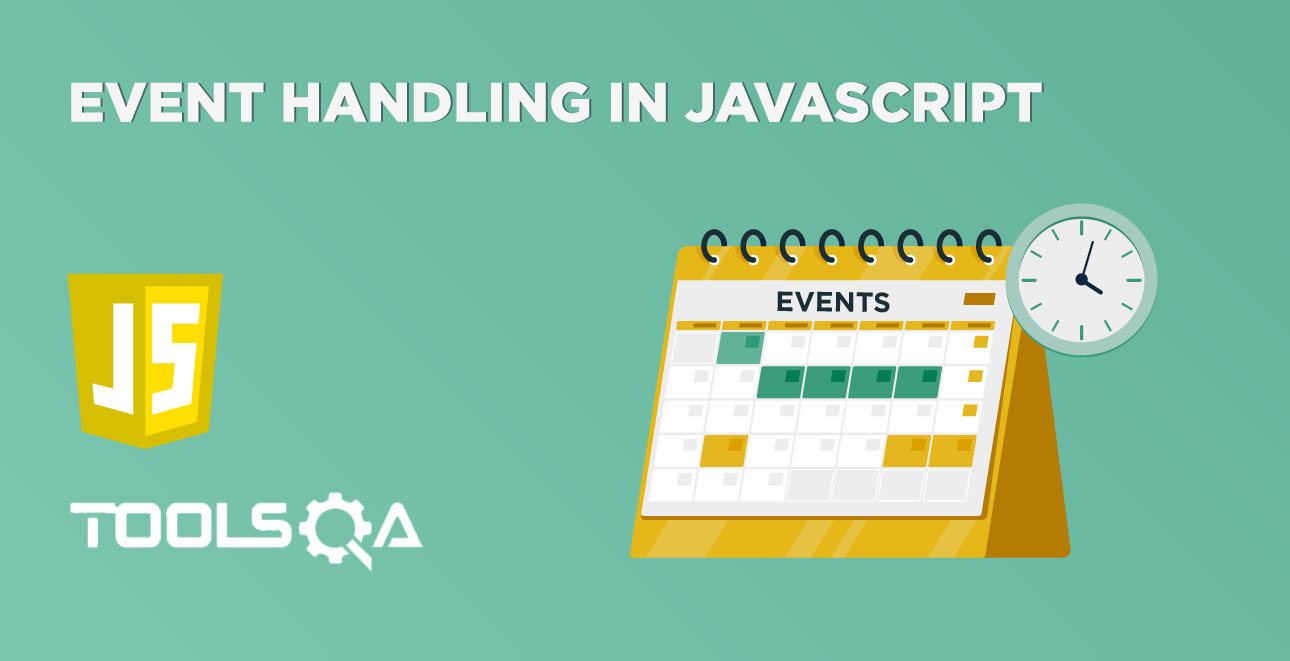