All of us have seen various programming errors during the development process. Broadly these errors are categorized as - Syntax errors, Logical errors, and Runtime errors. The syntax errors generally happen during the compile or interpretation level. They can be caught easily by the compiler or interpreter. Still, the logical fallacies are the bugs in the program/system and come under the responsibility of the developer or QA to get them fixed before the delivery. The most tricky ones are the Runtime error, which happens while the program/system is running and leads to an error or crash situation due to an unhandled exception. So, it is the responsibility of the programmer to handle such runtime conditions, so as they don't lead to a disaster while the system is running. In this article, we will learn the concept Error Handling in JavaScript with the help of the following topics:
- What is Error Handling in Javascript?
- How to use the "try...catch" block to handle runtime errors?
- What is the finally block?
- How to create a user-defined exception with the help of throw keyword?
What is Error Handling in JavaScript?
Error handling, as the name states, is a strategy that handles the errors or exceptions which occur at runtime. Javascript is a loosely coupled language, so it doesn't raise many errors at the compile/interpretation level, and you will see many mistakes at runtime only. Few of the common examples which can lead to a runtime error are:
- When you try to access a variable or property, which is undefined.
- When you try to access an undefined method
In all the above cases, an error occurs, which results in terminating the program abruptly or stops the execution without completing. It might cause a problem in many cases like someone opens a database connection and the program fails/crashes without gracefully closing the database connection, which may lead to a situation of exhausting the number of allowed connection to the database instance and may lead to a situation where the database itself becomes inaccessible.
Consider the following code snippet, which generates a runtime error when, by mistake, the method name is not correct:
<html>
<meta charset="utf-8"/>
<body> Demonstrating Runtime error in JavaScript</br>
<script type="text/javascript">
function divide(num1, num2) {
console.log("Result of dividing " + num1 + " by " + num2 + " is:" + num1/num2);
}
divide(200,100);
//the folllowing line will cause the run time exception, as by mistake method name is wrong
divid(200,50);
console.log("The code from here does not execute")
console.log("This program is expected to be executed")
</script>
</body>
</html>
Save the file with name runTimeError.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident by the above code snippet and screenshot, by mistake, we put the function name as "divid" instead of "divide", and it leads to a runtime exception. The side impact is, instead of completing the program gracefully, the program execution halts at the same place where it raised the exception.
So, by handling such errors, it will be possible to handle such situations gracefully and avoiding an unintended state of the system. Like the maximum of the programming languages, JavaScript also provides the try...catch block, which can handle the runtime errors. Let's understand the details of how to use these in the following section:
How to use the "try...catch" block to handle runtime errors?
JavaScript supports the use of try...catch block to handle the runtime errors gracefully. The Javascript code, which may cause the runtime errors, should be wrapped under the try block. When a specific line under the try block causes an exception/error, the control immediately transfers to the associated catch block skipping the rest of the lines in the try block. Its syntax looks like below:
Syntax:
try{
//lines of code in javascript which might cause an error/exception
}
catch(e){
//lines of code need to be executed when there is an exception
}
Let’s understand these details using the following example, where we are wrapping the possible errors in a try-catch block:
<html>
<meta charset="utf-8"/>
<body> Demonstrating try catch block</br>
<script type="text/javascript">
function divide(num1, num2) {
console.log("Result of dividing " + num1 + " by " + num2 + " is:" + num1/num2);
}
try{
divide(200,100);
//the folllowing line will cause the run time exception, as by mistake method name is wrong
divid(200,50);
console.log("The code from here does not execute")
}
catch(e){
console.log(e.message)
console.log(e.description)
console.log(e.stack)
}
console.log("This program is expected to be executed")
</script>
</body>
</html>
Save the file with name tryCatchDemo.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see from the above code snippet, this time we put the method calls in the "try" block, and when the program raised the exception at the time, it was caught gracefully, and the control was passed to the "catch" block, where we logged all the details of the exception. The last statement after the "catch" block executed successfully, as the program didn't lead to any unintended situation.
if you notice, the "catch" block accepts a parameter "e" which is an object of exception in JavaScript and provides the following methods:
- e.message provides information about the exception.
- e.description describes the exception.
- e.stack provides the stack trace information like in above it is showing which line number and column number in that line caused an exception
What is the finally block?
Now, suppose, there is a particular piece of code that you want to execute, no matter whether the exception raised or not. In that scenario, you can use the "finally" block. The "finally" block executes for sure, irrespective of whether there is an exception or not. It generally cleans or frees resources that the script was holding onto during the program execution. Example: if the program opened the DB connection and tries to process some action in that time, an exception occurs, then finally block can be used to close the DB connection. It s syntax looks like below:
Syntax:
try{
//Code which might get an exception
}catch(){
//Code what needs to happen when there is an exception
}finally{
//Code that will be executed whether there is an exception or not
}
Let’s understand these details using the following example, where we are using the finally block when there is an exception:
<html>
<meta charset="utf-8"/>
<body> Demonstrating try catch finally block</br>
<script type="text/javascript">
function divide(num1, num2) {
console.log("Result of dividing " + num1 + " by " + num2 + " is:" + num1/num2);
}
try{
divide(200,100);
//the folllowing line will cause the run time exception, as by mistake method name is wrong
divid(200,50);
console.log("The code from here does not execute")
}
catch(e){
console.log(e.message)
console.log(e.description)
console.log(e.stack)
}
finally
{
console.log("Finally Block: This will be executed always")
}
console.log("This program is expected to be executed")
</script>
</body>
</html>
Save the file with name finallyDemo.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we see in the above program, even though there is an exception, the finally block is executes.
How to create a user-defined exception with the help of throw keyword?
If you want to give some customized messages or details when an exception raises, JavaScript provides the "throw" keyword to handle such a situation. You can create your personalized exceptions, which will be thrown based on a condition. Its syntax looks like below:
Syntax:
throw{
error://error message
message://description of the message
}
Let’s understand these details using the following example. Whereby we are creating the custom error when a user tries to divide a number by zero.
<html>
<meta charset="utf-8"/>
<body> Demonstrating user created exception with help of throw block</br>
<script type="text/javascript">
function divide(a,b){
if(b==0){
throw{
error:"Divide by zero",
message:"Denominator cannot be zero",
description:"User sent the denominator as zero"
}
}
}
try{
divide(10,0)
}
catch(e){
console.log(e.error)
console.log(e.message)
console.log(e.description)
}
</script>
</body>
</html>
Save the file with name userDefinedExceptionDemo.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As seen above, when variable b is zero, the user is creating a custom exception that he catches in the catch block. Additionally, it will have the same details as specified by the programmer. Therefore, you can create your customized exceptions on certain conditions that the programming language does not handle by default.
Key Takeaways
- Error handling handles the runtime errors in the JavaScript
- try is the keyword creates the try block. Here the code that can cause exception is under the wrap under this block.
- The catch is the keyword creates the catch block. Additionally, we use it to catch the exception thrown from the try block and execute the code.
- Finally, the block runs a piece of code always whether there is an exception or not.
- The throw keyword can create customized exceptions, which can be thrown based on certain conditions.
To conclude, let’s now move to the next article to understand how to use the "Event handlers in JavaScript".
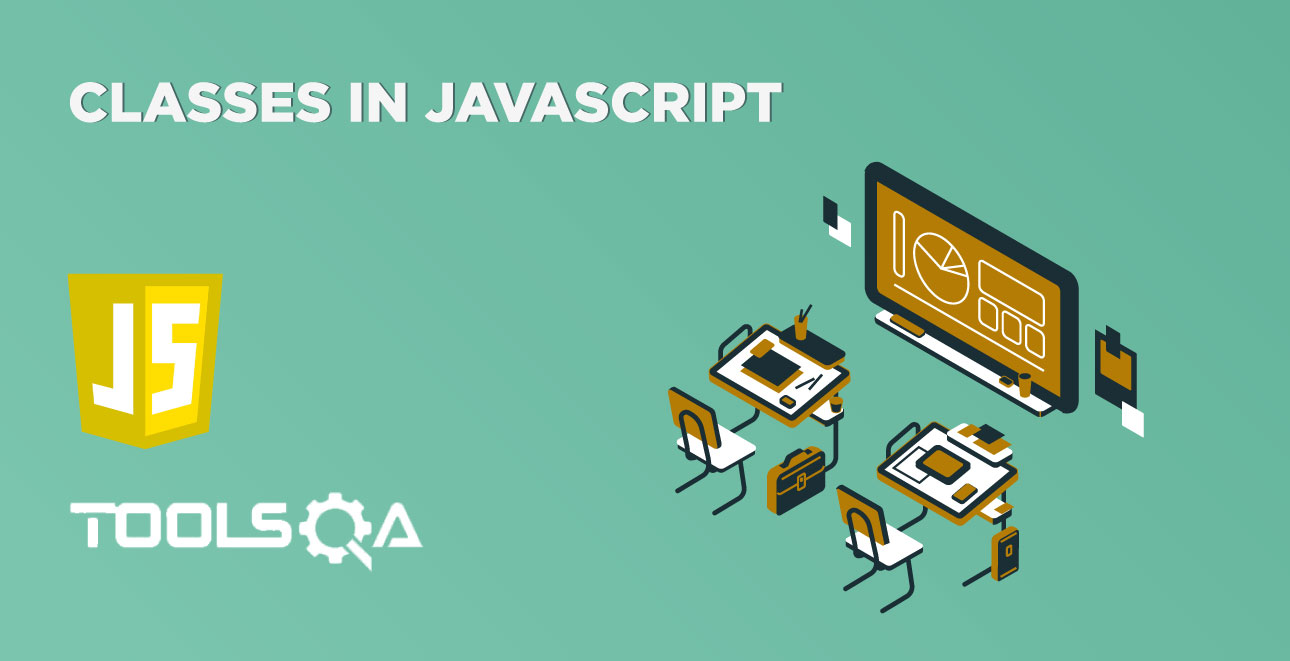
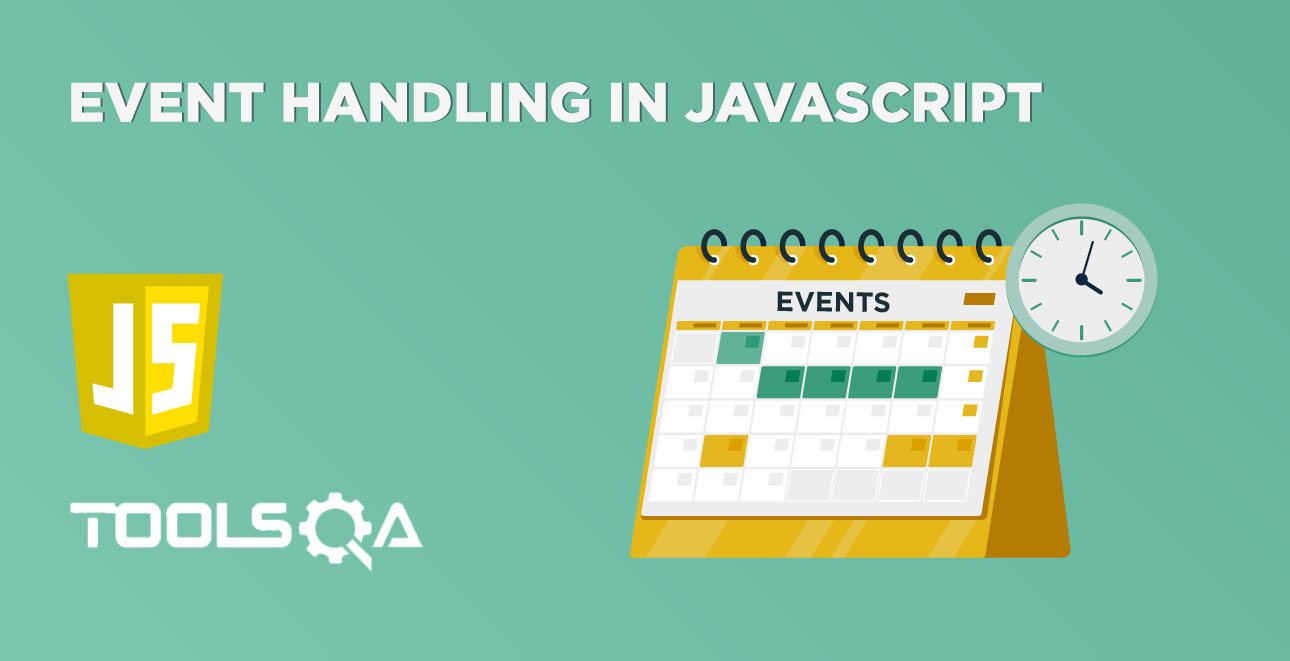