Cypress provides two essential methods get() and find() to search for the web elements based on the locators. The results for both of these methods are almost identical. But each has its importance and place of implementation. Subsequently, in this article, we will be covering aspects detailing where get() and find() methods which can be used during the web test automation using Cypress:
- What is the get() command in Cypress?.
- How to access multiple values returned by "get()" command?
- How to chain other Cypress commands with "get()" command?
- What is ".within()" block?
- What is the find() command in Cypress?
What is the "get()" command in Cypress?
The get() method gets one or more elements based on the selector passed as a parameter. Additionally, it can return a web element or a list of web elements. After that, appropriate action can be performed on that.
Syntax:
cy.get(selector)
cy.get(selector, options)
The below image shows the syntax and various parameters which can pass to the "get" method:
As we can see that, the first parameter that the "get" method accepts is the "CSS selector" of the web element and the second parameter, which is an optional parameter, can take the following values:
Option | Default | Description |
---|---|---|
log | true | Toggle which enables whether the command should log on the console or not. |
timeout | defaultCommandTimeout | Time for which get() should wait before raising timeout error. |
withinSubject | null | Specifies from where element search should start. Additionally, null specifies that Cypress will start searching element from the root element. |
Examples:
Get an element of type input
cy.get('input')
Specify logging as false. Moreover, it will skip the command output printing on the Cypress Test runners console.
cy.get('input',{ log: false })
Wait for an explicit timeout
cy.get('input',{ timeout: 1000 }) // It will wait for 1000ms before timing out
Note: cy.get() will automatically retry (default Cypress Timeout) until the element you are searching exists in DOM or until assertions you've chained all pass. Moreover, if it is taking more time than the default timeout, then Cypress can timeout waiting. For handling such situations, we can pass explicit timeout as an option in cy.get() command.
Search element within an element:
cy.get('input',{ withinSubject : document.querySelector('#menu-top')}) // uses jQuery Selector
or
cy.get('input',{ withinSubject : document.getElementById('#menu-top')}) // uses HTML Selector
How to access multiple values returned by "get()" command?
The cy.get( ) method might not return just one element. It can return a list or array of web elements, and then we have to pick certain elements and do our operations or assertions. Therefore, for handling such situations, we can use the "eq" method.
Additionally, the Eq method helps us in getting a DOM element at a specific index in an array of elements.
For example, if we have five input tags in our DOM having different values in every tag. Below is the DOM :
<ul>
<input>Cypress</input>
<input>Cucumber</input>
<input>Gherkin</input>
<input>JBehave</input>
<input>BDD</input>
</ul>
Our situation is that we want to validate that if the second input tag has Cucumber in it, which it has. So we can achieve that using the eq method. Moreover, below will be our cypress command.
cy.get('input').eq(1).should('contain', 'Cucumber')
Note: The index value in eq starts from 0, the same as arrays in other programming languages. That is to say, the first element will be accessed using eq(0), the second element using eq(1), and so on.
How to chain other Cypress commands with "get()" command:
Another critical aspect of the "get" command is that we can chain commands to do respective actions on a particular web element. As shown in the below screenshot, the get command returns an object of the "Chainable" interface, which enables other Cypress commands to invoke on that object.
Cypress manages a Promise chain on your behalf, with each command returning a ‘subject’ to the next command until the chain ends or an error encounters. So, all the commands returning a chainable interface allows invoking any other Cypress command without explicitly using the "cy" object. Let's understand this behavior with the help of the following examples:
Example:
Find the link with an "href" attribute containing the word “demoqa” and click it.
cy.get('a[href*="demoqa"]').click()
Find the web element with class name as 'mobile-nav' and pass the timeout as options (we learned above) and then chain commands having different assertions for visibility and containing 'Home' keyword.
cy.get('.mobile-nav', { timeout: 10000 })
.should('be.visible')
.and('contain', 'Home')
What is ".within()" block?
As we see in the above topics, we can chain Cypress commands along with other commands and assertions. In the automation world, we do come across such a situation where we want to search a web element inside a parent web element. So for doing that, we use "within" block chained with "cy.get" command to search for a particular parent element.
cy.get() always looks for the element within the whole document, with an exception that when its used inside the .within() code block. Its syntax will look like below:
Syntax:
.within(callbackFn)
.within(options, callbackFn)
Where callbackFn will be any function that will invoke after the parent function.
Let's understand the details with the help of the following example:
cy.get('#searchBox').within(() => {
cy.get('input').type('Cucumber') // Only searches inputs within searchBox
})
In this example, it will first get the "searchBox" element, and inside that searchBox element, it will search for input tags and will ignore other input tags that are not inside this. There can be a situation where we have five input tags, but we want to work on one input tag, which is under the "searchBox" element so that the situation can resolve using the ".within" block.
So, in actual, the callback function within the ".within" block is invoked after the cy.get ('#searchBox') function and restricts the scope of the commands inside the callback function to the scope returned by the parent get command.
What is the "find()" command in Cypress?
The find() method returns one or more DOM elements based on the selector that's passed as a parameter. However, the only difference being is that the find() method always chains with other methods that return DOM elements, such as the get() method. Moreover, you can never chain/invoke the find() method on the "cy" object, as we did with the "get()" method in the previous section.
Syntax:
.find(selector)
.find(selector, options)
Below options can be passed as a parameter:
Option | Default | Description |
---|---|---|
log | true | Toggle which enables whether the command should log on the console or not. |
timeout | defaultCommandTimeout | Time for which get() should wait before raising timeout error. |
Note: As we know that find() command will always be chained on other cypress commands, we will majorly use it with the "get()" command and its syntactical representation is as follows:
cy.get(parentSelector).find(childSelector)
The below screenshot shows a sample invocation of find command and its various parameters and return values:
Let's try to understand the usage of "find()" command with the help of the following examples:
Examples:
Consider, we do have the following HTML snapshot:
<ul id="parent">
<li class="child"></li>
<li class="child"></li>
</ul>
We have to find all the <li> elements which have the #id as "parent", then the same can be achieved using the following command:
cy.get('#parent').find('li')
So, this makes it clear that, if users need to search for a web-element which is nested inside the other web-element or have a parent-child relationship, then find() will be the best fit and will provide the results quickly.
In the above code, the cy.get('#parent') will do a search for the parent element only, and inside the parent web element, it will search for tags starting with "li" and will return two web elements.
Note: the log and timeout parameters have the same syntax as we used them in the "get()" command.
How to use get() and find() commands for automating a user scenario?
Let's automate one user journey using get and find commands. We will continue the example from the previous article of clicking on Widget Menu Items on the "demoqa.com" to demonstrate the use of the "get()" and "find()" methods. In continuation of the clicking, let's search all the hyperlinks or items found and assert the length of all the items found. So, let's validate the following elements:
- The total number of articles returned after clicking on the Widgets menu item.
- The total number of articles that have a hyperlink value of "keyboard".
Let's extend the code of "cypressTest1.js" under the examples directory:
// type definitions for Cypress object "cy"
/// <reference types="cypress" />
describe('My First Cypress Test', function() {
it('Visits the ToolsQA Demo Page and check the menu items', function() {
//Visit the Demo QA Website
cy.visit("https://demoqa.com/");
// Clicking on Widget Menu Item
cy.get('#menu-top > li:nth-child(3) > a').click();
//Verify number of items present on Widget Tab
cy.get('.demo-frame > ul > li').should('have.length',19);
//Verify number of items having keyboard as text on Widgets Tab
//Get and Find Command (Parent - Child Relationship)
cy.get('.demo-frame > ul > li').find('[href*=keyboard]').should('have.length',2);
})
})
The following figure details the usage of "get()" (highlighted with marker 1) and "find()" (highlighted with marker 2) in the below code snippet and also the sample output of the test where the same assertions execute are highlighted with marker 1 and 2.
So as we have seen both in code and in the screenshot that our assertions worked fine. Additionally, we have also seen the practical implementation of "get" and "find" commands.
Key Takeaways
- get() command helps in searching web elements on a web page and returns one or more elements depending on the elements matching the locator/selector passed as parameter.
- Additionally, eq() command can access elements by index when multiple elements are returned by the get() command.
- within() clause can be used to search for elements within the scope of other elements.
- Moreover, find() command is also used to search for web elements on the webpage. But it can't be directly invoked on the "cy" object. Additionally, it will always change with other cypress commands.
To conclude, we have a clear understanding of get and find commands and their practical usage. Let's proceed to the next tutorial, where we will learn What is Asynchronous Nature of Cypress Framework?.
If you want to learn more you can look at this video series: ++Cypress video series++
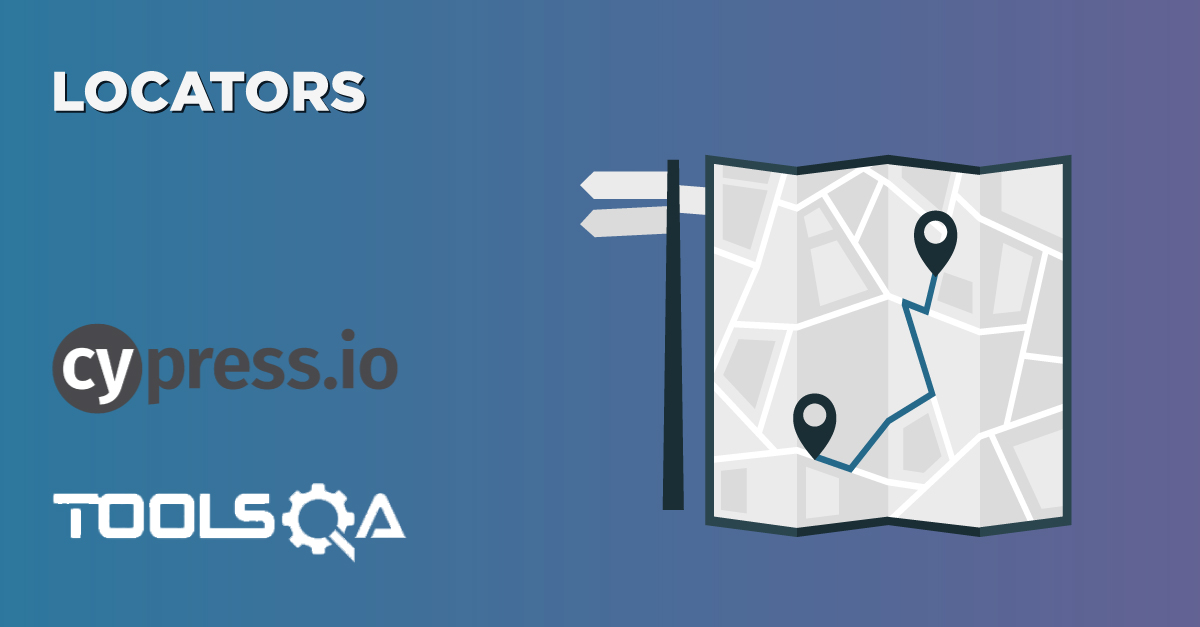
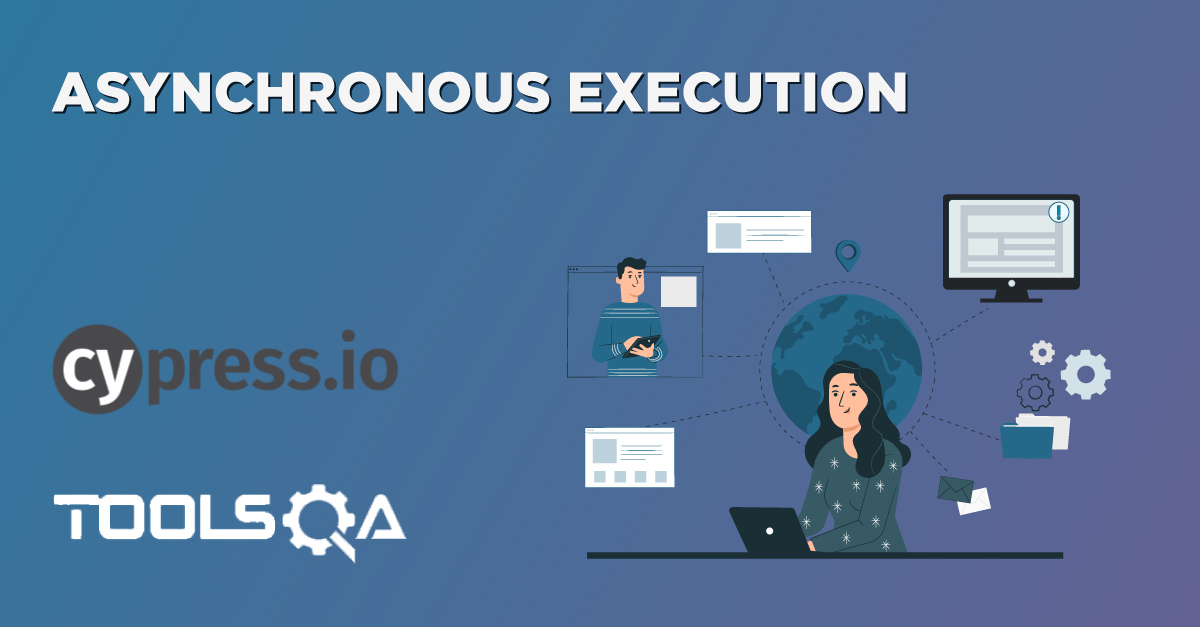