A very common type of control used on the website is the HTML iframe. And this control needs to be handled in a particular manner when testing. This article explains to you how to handle iframes properly in Katalon Studio.
What is an iframe?
An iframe (Inline Frame) is an HTML document which is embedded in another HTML document. The iframe HTML element often inserts the content from another source, such as an advertisement, into a Web page.
Why is it important to know how to test iframes?
Verifying text and objects within iframes can be a difficult job. For example, even though you can see a text displayed in an iframe, automation tools may not detect the text. You have to tell your script how to traverse through a website's iframes structure and select the correct one where the text and its object are present.
Example #1
- Given that you want to capture the Comment text field of a particular question in Katalon Forum (this text field is an iframe), you can use the Web Object Spy of Katalon and see that it can detect the one in the red highlighted area.
- Once we capture the Comment iframe, Katalon shows all of its child elements, which you can see in the Object Spy dialog as below:
- As you save the captured object to Katalon Studio, its iframe is also included in it. It is as shown in the following screenshot:
- Then, you can set the text to the Comment field by specifying the child element for the Set Text keyword as described below:
Example #2
- Given that you want to take the JQueryUI's Drag and Drop example (this draggable control is an iframe), as shown in the screenshot below, you can drag the ‘Drag me around’ object to different areas of the iframe.
- Use the Object Spy to capture/ take the iframe as usual. The Object Spy can detect, capture/ take the iframe, and show all of the iframe’s elements accordingly.
- As you save the captured object to Katalon Studio, the iframe is also included in it as the object’s parent element. It is as shown in the following screenshot (Note that you can uncheck the option to use parent iframe if needed):
- Given the situation where you opt not to specify/ mention the iframe parent for an element, to interact with the element, you need to use the keyword Switch To Frame to have Katalon focus on the parent iframe before interacting with the element.
The sample code below shows how to switch to the parent frame before using the drag and drop action on the elements within iframe:
import com.kms.katalon.core.annotation.SetUp as SetUp
import com.kms.katalon.core.annotation.TearDown as TearDown
import com.kms.katalon.core.model.FailureHandling as FailureHandling
import com.kms.katalon.core.testobject.ObjectRepository as ObjectRepository
import com.kms.katalon.core.mobile.keyword.MobileBuiltInKeywords as Mobile
import com.kms.katalon.core.webservice.keyword.WSBuiltInKeywords as WS
import static com.kms.katalon.core.testobject.ObjectRepository.findTestObject
import static com.kms.katalon.core.testdata.TestDataFactory.findTestData
import static com.kms.katalon.core.testcase.TestCaseFactory.findTestCase
import com.kms.katalon.core.testcase.TestCase as TestCase
import com.kms.katalon.core.testdata.TestData as TestData
import com.kms.katalon.core.testobject.TestObject as TestObject
import com.kms.katalon.core.webui.keyword.WebUiBuiltInKeywords as WebUiBuiltInKeywords
import com.kms.katalon.core.webui.keyword.WebUiBuiltInKeywords as WebUI
import internal.GlobalVariable as GlobalVariable
'Open browser and navigate to jQuery UI page'
WebUI.openBrowser('https://jqueryui.com/')
'Maximize current browser window'
WebUI.maximizeWindow()
'Click on \'Draggle\' link'
WebUI.click(findTestObject('Page_jQuery_Homepage/lnk_Draggable'))
'Switch to iframe of Demo panel'
WebUI.switchToFrame(findTestObject('Page_jQuery_Drag and Drop Example/ifr_Demo Frame'),
GlobalVariable.G_Timeout_Small, FailureHandling.STOP_ON_FAILURE)
'Drag and drop iframe into other position'
WebUI.dragAndDropByOffset(findTestObject('Page_jQuery_Drag and Drop Example/div_Frame_Draggable'),
200, 38)
'Switch back to current window'
WebUI.switchToDefaultContent()
'Click on \'Droppable\' link'
WebUI.click(findTestObject('Page_jQuery_Homepage/lnk_Droppable'))
'Switch to iframe of Demo panel'
WebUI.switchToFrame(findTestObject('Page_jQuery_Drag and Drop Example/ifr_Demo Frame'),
GlobalVariable.G_Timeout_Small, FailureHandling.STOP_ON_FAILURE)
'Drag the left rectangle and Drop it the right-side one'
WebUI.dragAndDropToObject(findTestObject('Page_jQuery_Drag and Drop Example/div_Frame_Draggable'),
findTestObject('Page_jQuery_Drag and Drop Example/div_Frame_Droppable'), FailureHandling.STOP_ON_FAILURE)
WebUI.closeBrowser()
Example #3: Switch To Frame
We can switch to frames using the switchTo() method then act on that element.
If we want to get the text in the Text Area, then we cannot get it directly by taking the Xpath of the element. As it is available in the iframe, we need to switch to the frame first, and then we can get the text of the element.
Manual Mode:**
Script Mode:**
import static com.kms.katalon.core.testobject.ObjectRepository.findTestObject
import com.kms.katalon.core.webui.keyword.WebUiBuiltInKeywords as WebUI
'Launching and Navigating to URL\r\n'
WebUI.openBrowser('https://the-internet.herokuapp.com/iframe')
'Maximize the window\r\n'
WebUI.maximizeWindow()
'Switching to text area which is situated in Iframe'
WebUI.switchToFrame(findTestObject('Frames/textArea_Iframe'), 60)
WebUI.scrollToElement(findTestObject('Frames/TextArea'), 60)
'Fecthing the text from Text area and storing it in a variable'
Text = WebUI.getText(findTestObject('Frames/TextArea'))
'Verifying the Actual text with Expected text from Text Area.'
WebUI.verifyEqual(Text, 'Your content goes here.')
Note: Please download the source code here and get the HTML here.
Example #4: Switch to Default Content
Whenever we switch to frames to handle specific features, we must switch back to the parent node to access other features of the application. If we do not switch back to the parent node, your code looks for the next locators within that same frame itself.
The switch switches back to the main window or parent window frame.
Manual Mode:
Script Mode:
import static com.kms.katalon.core.testobject.ObjectRepository.findTestObject
import com.kms.katalon.core.webui.keyword.WebUiBuiltInKeywords as WebUI
'Launching and Navigating to URL\r\n'
WebUI.openBrowser('https://the-internet.herokuapp.com/iframe')
'Maximize the window\r\n'
WebUI.maximizeWindow()
'Switching to text area which is situated in Iframe'
WebUI.switchToFrame(findTestObject('Frames/textArea_Iframe'), 60)
'Fecthing the text from Text area and storing it in a variable'
Text = WebUI.getText(findTestObject('Frames/TextArea'))
'Switching back to the main window or parent window frame'
WebUI.switchToDefaultContent()
'Verifying the Actual text with Expected text from Text Area.'
WebUI.verifyEqual(Text, 'Your content goes here.')
'Getting the text of the web element and storing it in a variable'
Heading = WebUI.getText(findTestObject('Frames/Frame Heading'))
'Verifying the Actual text with Expected text from Text Area.'
WebUI.verifyEqual(Heading, 'An iframe containing the TinyMCE WYSIWYG Editor')
Note: Please download the source code here and get the HTML here.
Common exceptions
Noted that NoSuchFrameException or InvalidSwitchToTargetException exceptions throw when the target frame that needs switching doesn’t exist.
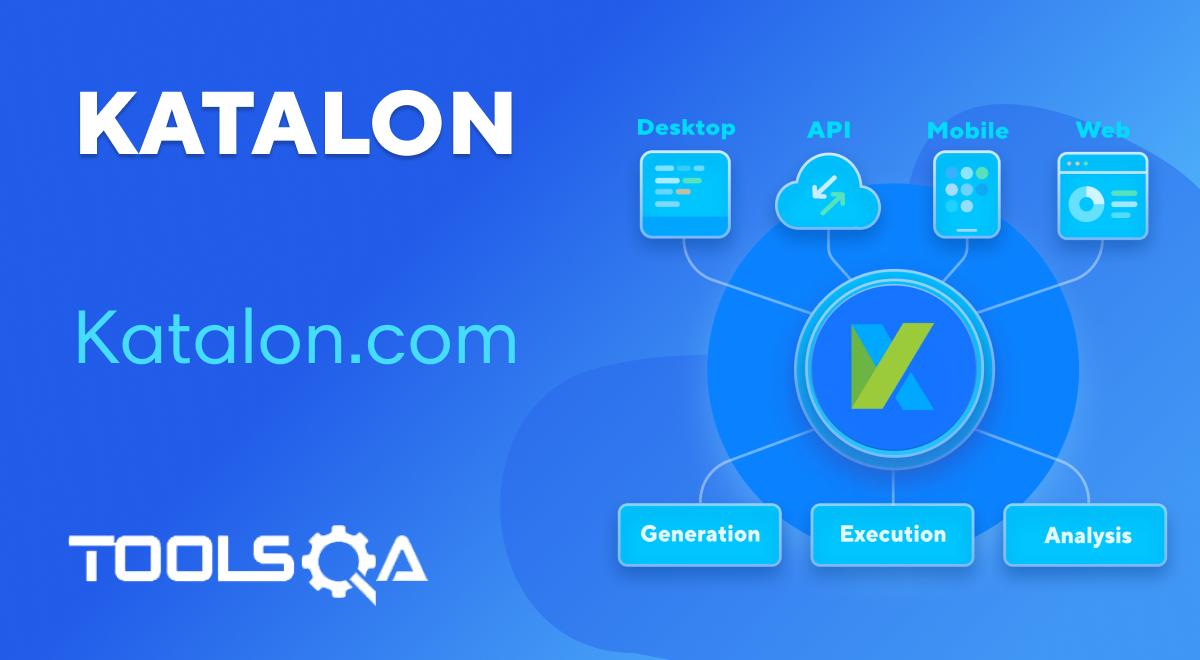
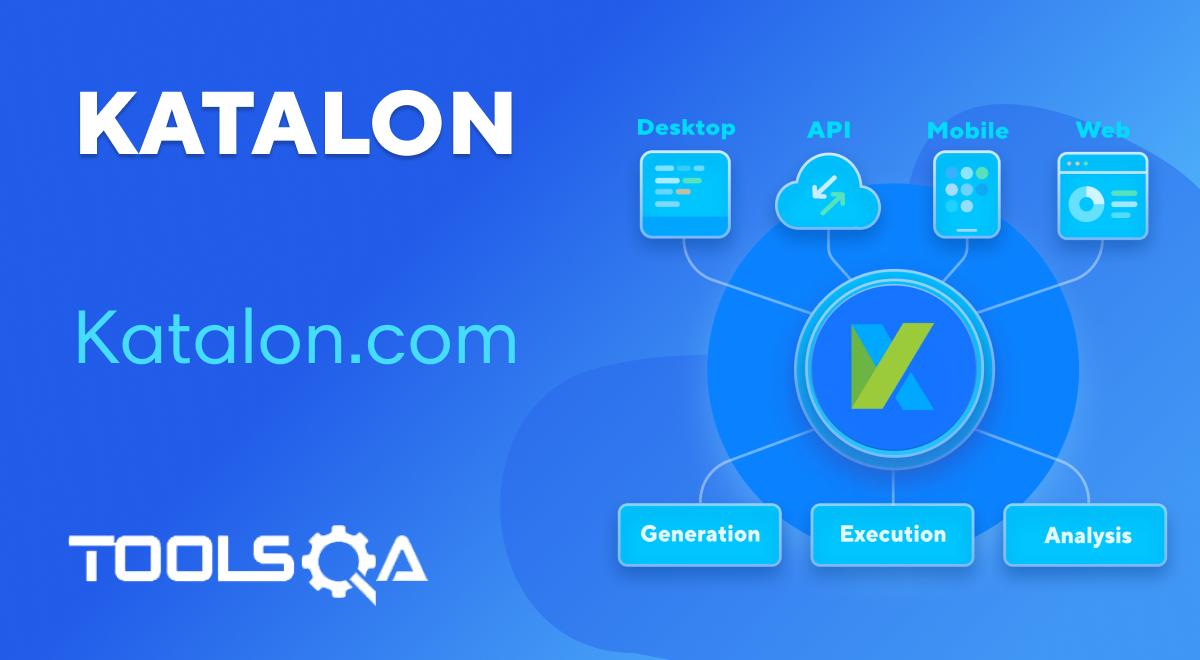