Vector
The Vector class implements a grow-able array of objects, whose size is designed to be changed at run time.
List<String> words = new Vector<String>();
Similar to elements of an ArrayList, those of a Vector are accessible via index, and the size of a Vector can grow or shrink as needed.
// Grow this instance, if necessary,
// to ensure that it can hold at least the number of elements specified
// by the minimum capacity argument.
void ensureCapacity(int minCapacity);
// Shrink the capacity of this Vector instance to be this instance's current size
void trimToSize();
Internally, a Vector optimizes storage by maintaining two private variables capacity and a capacityIncrement. Its capacity is always no less than the vector size, and the capacity is designed to increase in steps of capacityIncrement. However, an application may opt to set a large initial capacity of a vector to reduce the amount of incremental reallocation.
// set initial size of the underlying array to 1024 and incremental step to 64
List<String> words = new Vector<String>(1024, 64);
Example
Here is a test class for the CRUD operations on Java Vector:
// File: VectorTest.java
import java.util.List;
import java.util.Vector;
public class VectorTest {
public static void printList(List<String> words) {
for (int i = 0; i < words.size(); i++) {
System.out.println("Words[" + i + "]: " + words.get(i));
}
}
public static void main(String []args) {
List<String> words = new Vector<String>();
// append
words.add("school");
printList(words);
words.add(0, "at");
System.out.println("> Insert via index");
printList(words);
words.set(1, "work");
System.out.println("> Update via index");
printList(words);
words.remove(0);
System.out.println("> Remove via index");
printList(words);
}
}
The above code produces:
Words[0]: school
> Insert via index Words[0]: at Words[1]: school
> Update via index Words[0]: at Words[1]: work
> Remove via index Words[0]: work
For more examples on Iterator and for:loop, please read ArrayList.
Vector vs. ArrayList
ArrayList and Vector store data in an internal array, yet their behaviors are quite different. Let us examine some of the differences in behavior between ArrayList and Vector.
Resize: Both ArrayList and Vector are designed to grow and shrink for optimal memory usage, however the ways they are re-sized are different. While ArrayList grows by half of its size, Vector doubles its own size by default or increment its size by capacityIncrement` variable if this variable is set to a positive integer.
Synchronization: For each element of a Vector, there is a lock to synchronize operations on it. That is because Vector was designed to be used by many threads concurrently. ArrayList has no lock at all.
Performance: Since ArrayList is designed to be non-synchronized, its performance is better than Vector.
For many programmers, including me, ArrayList is a better choice when writing single-threaded applications. Later in this tutorial, you will find more tips on how to write safe code for multi-thread applications in Java.
Summary
- Vector and ArrayList are both array-based implementations of the List interface
- Like ArrayList, Vector offers CRUD operations on index and Iterator.
- For single threaded applications, ArrayList is preferable to Vector.
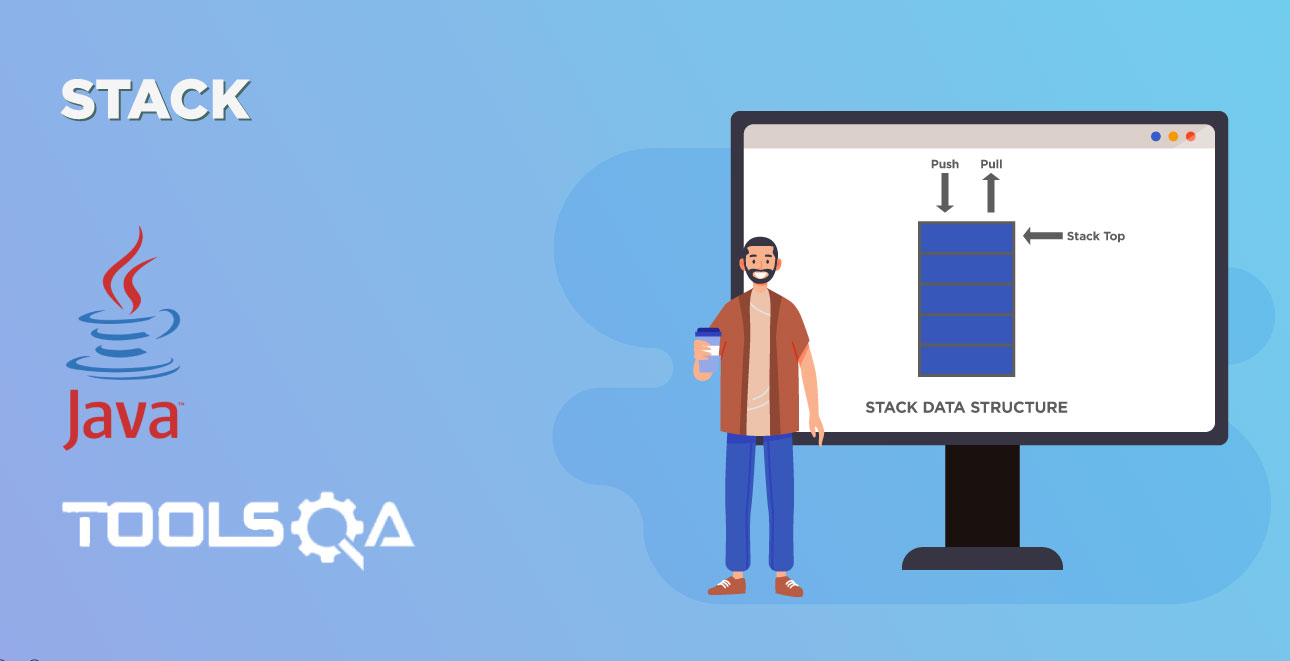
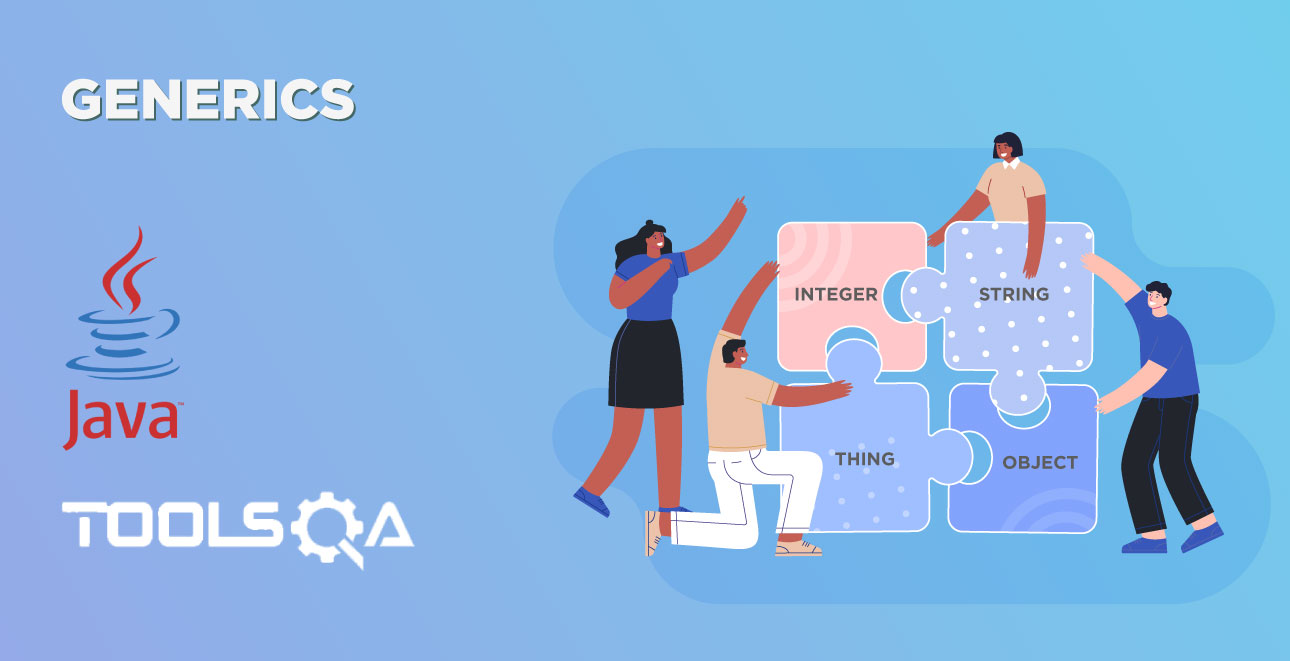