Classes and Objects in Java, or any other Object-Oriented Programming Language, form the basis of the language. Classes allow a user to create complex data structures by logically grouping simple data structures. The most important part here will be to visualize the concepts. Once you are able to visualize and relate the concepts to real-life you will pick up a programming language quite fast.
What is an Object in Java?
In Object-Oriented Programming (OOP), an object is an instance of a class. An object can be considered as a thing, which performs a set of activities. An object can be anything and in the real-world an object can be a dog, table, window, car, etc.
But objects always share two characteristics, they all have state and they all have behaviors.
For example, a car has state (make, brand, color, model) and has behavior (braking, accelerating, slowing down, changing gears).
The same way an object in any programming language too has state and behavior. A software object maintains its state in variables and implements its behavior with methods. So from the above example of car object, we can say that the object is a collection of methods ( behavior - braking, accelerating, slowing down, changing gears) and variables (state - make, brand, color, model).
What is Class in Java?
Classes are the templates for the creation of objects. When we say a template, it means it always has the same structure and implementation. They set out rules for how the objects that they contain may behave. So all the objects created from a single class share the same behavior and state. The difference between a class and an object is that classes are created when the program is created but the objects are created at the run time.
For example, if we create a class 'Car' and create an object 'Toyota' from that car class, then we can say that the Toyota car object is an instance of the class of objects known as Car. Toyota car have some states and behavior. However, each brand of car will have its own state and behaviors.
How to create a Class in Java?
A class can have just one variable or a group of variables. To create a class you start with the class keyword followed by a name and its body delimited by curly brackets.
class Car{
}
How to declare a Variable of Class?
In the previous chapter, we have learned how to create a variable. It works the same with creating a class but in place of any data type just use the class name. Like above we create a class Car, now we can easily create a variable of the above class.
public class ClassTestExercise {
public static void main(String[] args) {
//Use the Car keyword to declare Car Class variable
Car Toyota;
//Initialize the Toyota variable with a new Car object
Toyota = new Car();
}
}
You can also do the creating and initializing of the Class variable on the same line.
Car Toyota = new Car();
How to Declare Member Variables in Java?
The curly braces '{ and }', of a class is referred to as its body. So that variables declared in the body of the class are called member variables. In java, these member variables are called fields. The fields can be any type.
public class Car {
//Class Member Variables & Fields
String sModel;
int iGear;
int iHighestSpeed;
String sColor;
int iMake;
boolean bLeftHandDrive;
String sTransmission;
int iTyres;
int iDoors;
}
How to initialize a Class Object in Java?
After declaring an instance of a class, you can access each of its members and assign it the desired value.
public class ClassTestExercise {
public static void main(String[] args) {
//Use the Car keyword to declare Car Class variable
Car Toyota = new Car();;
Toyota.bLeftHandDrive = true;
Toyota.iDoors = 4;
Toyota.iGear = 5;
Toyota.iHighestSpeed = 200;
Toyota.iMake = 2014;
Toyota.iTyres = 4;
Toyota.sColor = "Black";
Toyota.sTransmission = "Manual";
Toyota.sModel = "Camry";
}
}
How to create Methods of a Class in Java?
So far we have seen that while creating a class, fields are meant to describe it. In our case the class Car is described with its gear, color, make, brand,, etc. Apart from these characteristics, A car object can also perform some actions. These actions are referred to as methods of a class.
A method can produce some value or return some value. If the method is returning some value then the type of value that a method can provide (or return) is written on the left side of the method name. But when it does not, then the method is to be declared as void method.
public class Car {
//Class Member Variables & Fields
String sModel;
int iGear;
int iHighestSpeed;
String sColor;
int iMake;
boolean bLeftHandDrive;
String sTransmission;
int iTyres;
int iDoors;
public void DisplayCharacterstics(){
System.out.println("Model of the Car: " + sModel);
System.out.println("Number of gears in the Car: " + iGear);
System.out.println("Max speed of the Car: " + iHighestSpeed);
System.out.println("Color of the Car: " + sColor);
System.out.println("Make of the Car: " + iMake);
System.out.println("Transmission of the Car: " + sTransmission);
}
}
After creating a method in its body delimited by its curly brackets, you can define the desired behaviors and once you are done with that, you can use that method by typing the class name followed by dot and the method name.
ClassName.MethodName();
public class ClassTestExecise {
public static void main(String[] args) {
//Use the Car keyword to declare Car Class variable
Car Toyota = new Car();;
Toyota.bLeftHandDrive = true;
Toyota.iDoors = 4;
Toyota.iGear = 5;
Toyota.iHighestSpeed = 200;
Toyota.iMake = 2014;
Toyota.iTyres = 4;
Toyota.sColor = "Black";
Toyota.sTransmission = "Manual";
Toyota.sModel = "Camry";
//Using Car class method
Toyota.DisplayCharacterstics();
}
}
The above code will produce:
Model of the Car: Camry
Number of gears in the Car: 5
Max speed of the Car: 200
Color of the Car: Black
Make of the Car: 2014
Transmission of the Car: Manual
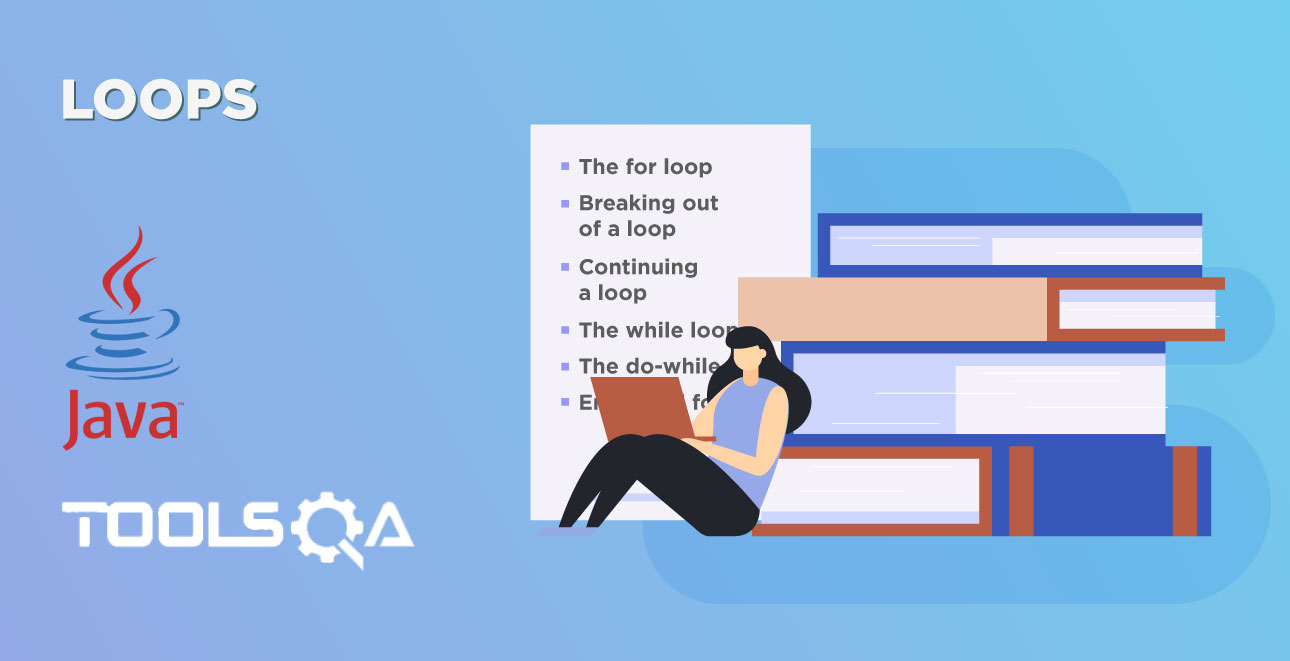
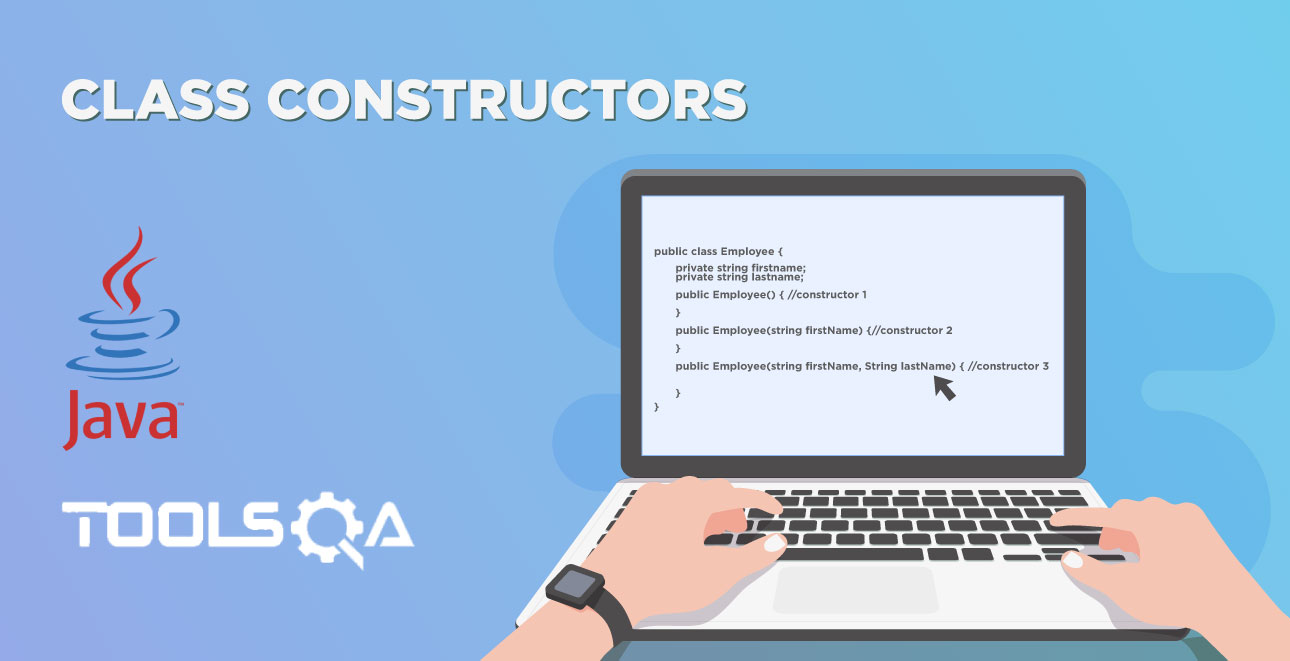