Data Types and Variables in Java
Having a good understanding of Data types and variables is the basic step towards understanding programming. This chapter is critical and please go through the details and practice the exercises given below related to Data types and Variables in Java.
What is Variable?
As its names suggest, variable is a value that can change. A simple computer program uses a set of instructions and data. Data can be constants or fixed values that never change and it can be variable that can change during the execution. Rather than entering data directly into a program, a programmer can use variables to represent the data. When compiler runs the program, variables used in the instructions are replaced with the real data entered by the programmer.
In technical terms, a variable is a reserved space or a memory location to store some sort of information. Information can be of any data type such as int, string, bool or float. Each variable in the program has its space allocated in the memory and based on the data type, the system allocates only required memory to the variables.
Every variable in the program has its name and data type.
How Variables work?
Declaring Variables: Ask the compiler to allocate some amount of memory to the variable for storing some value. As compiler would like to know the data type of the variable so that it can allocate only required memory to the variable. That can be done by 'declaring the data type' of the variable.
Naming Variables: After variable declaration, when the program needs the stored value, the compiler will go to the memory location to fetch the value and pass it back to the program. To effectively handle this transaction, the compiler would need two pieces of information from the program: name and data type of the variable. Give the name of your choice to the variable and let the compiler know about it. So that next time when you refer to that name, the compiler would know what piece of memory you are referring to. The name of the variable is called the Identifier.
This process is called as Variable Declaration.
DataTypeOfVariable VariableName;
Initialization of Variables: Once the variable declaration is done, then a value can be stored in the reserved memory location. Before that, you would not be able to use the variable. The initialization of a variable is very important before using the variable for any sort of operation. Putting an initial value is referred to as Initializing the Variable.
VariableName = Value;
What is Data Type?
A data type indicates what sort of value or the type of data the variable can represent, such as integer, floating-point numbers, character, boolean or an alphanumeric string.
There are other data types as well like short, long and float but in Selenium programming you may not face any situation where you have to use these data types. These data types are used when each byte of memory is important for better performance of the system.
Examples of different data types
Data Type - boolean
Boolean data type is used to store only 'boolean' values such as 'true' and 'false'. To declare a Boolean variable, you can use the 'boolean' keyword.
Here is an example:
package JavaTutorials;
public class BooleanTestExercise {
public static void main(String[] args) {
//Use the boolean keyword to declare boolean variable
boolean testResult;
//Initialize the boolean variable with value true or false, once boolean declaration is done
testResult = true;
//Print the value of boolean variable
System.out.println("Test Result is: " + testResult);
//Change the value of boolean variable
testResult = false;
//Print the value of boolean variable
System.out.println("Test Result is: " + testResult);
}
}
Above test will produce this:
Test Result is: true
Test Result is: false
Data Type - int
Integer data type is used to store numeric/numbers in the variable. In technical term, an integer store 32- bit of information and it stores the value between -2,147,483,648 and 2,147,484,647. But a decimal number can not be stored in the 'int' data type.
Here is an example:
package JavaTutorials;
public class IntegerTestExersice {
public static void main(String[] args) {
//Use the int keyword to declare integer variable
int carSpeed;
//Initialize the integer variable with value 20
carSpeed = 20;
//Print the value of integer variable
System.out.println("Car is running at the speed of: " + carSpeed);
//Change the value of integer variable
carSpeed = carSpeed + 20;
//Print the value of integer variable
System.out.println("Current speed of the car is: " + carSpeed);
}
}
Above test will produce this:
Car is running at the speed of : 20
Current speed of the car is: 40
Data Type - char
Character data type is used to store just one character in the variable. It is very rarely used in Selenium. To initialize such a variable, assign a character within the single-quoted.
Here is an example:
package JavaTutorials;
public class CharTestExercise {
public static void main(String[] args) {
//Use the char keyword to declare character variable
char a;
//Initialize the char variable with value 'P'
a = 'P';
//Print the value of char variable
System.out.println("Value of char is : " + a);
}
}
Above test will produce this:
Value of char is : P
Data Type - double
To declare a variable used to hold such a decimal number, you can use the double keyword.
Here is an example:
package JavaTutorials;
public class DoubleTestExercise {
public static void main(String[] args) {
//Use the double keyword to declare decimal variable
double PI;
//Initialize the double variable with value 'P'
PI = 3.14159;
//Print the value of double variable
System.out.print("PI: " + PI);
}
}
Above test will produce this:
PI: 3.14159
Notes: Rules for naming a variable
- A variable name can be of single letter of alphabets (A-Z).
- Variable name can start only with a letter, under score '_' and the dollar sign '$'.
- A variable name can not start with digit.
- A variable name can include digits.
- The name of a variable cannot be one of the words that the Java languages have reserved for its own use such as static, this, void, final, for, etc.
Everybody follows their own pattern to declare variables and I follow my own. I usually declare all the string variables with small letter 's' and int variable with small letter 'i'. So that in my program when I see any variable starts with the letter 's', I can understand that it is a String variable. For example:
String sName = "ToolsQA";
int iNum = 10;
boolean bResult = true;
Example of declaring multiple variables:
int a, b, c; // Declares multiple int, a, b, and c.
int a = 10, b = 20, c= 30; // Example of multiple initialization
Core Java - Data Types/Variables Practice Exercises, Programs & Questions
1. Write a Java program to print "Hello World" on the screen.
Ans:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
OUTPUT
Hello World
2. Write a Java program to print the following output:
++++++
@@@@@@
******
######
Ans:
public class PrintPattern {
public static void main(String[] args) {
System.out.println("++++++");
System.out.println("@@@@@@");
System.out.println("******");
System.out.println("######");
}
}
OUTPUT
++++++
@@@@@@
******
######
3. Write a Java program to declare three variable a, b, & c and store the values respectively 10, 20.3 & 3.14785 . Then display their values on the screen.
Ans:
public class Exercise_DataType_1 {
public static void main(String[] args) {
int a = 10;
double b = 20.3;
double c = 3.14785;
System.out.println("The value of a is : "+ a);
System.out.println("The value of b is : "+ b);
System.out.println("The value of c is : "+ c);
}
}
OUTPUT
The value of a is : 10
The value of b is : 20.3
The value of c is : 3.14785
4. Write a Java program to declare a Boolean variable with initial value of "true" and later change it to "false" before printing it.
Ans:
public class Exercise_DataType_2 {
public static void main(String[] args) {
boolean value = true;
value = false;
System.out.println("The value for the Boolean variable is : "+ value);
}
}
OUTPUT
The value for the Boolean variable is : false
Q1. Which four options are correct in terms of Data Types and their syntax:
// 1
int value = "ToolsQA";
// 2
int value = 67;
// 3
boolean booleanValue = 67;
// 4
boolean booleanValue = "ToolsQA";
// 5
boolean booleanValue = "true";
// 6
boolean booleanValue = false;
Ans: 2, 6
Q2. What is the purpose of System.out.println() method?
Ans: Display output on the Console
Q3. Which of the following is not a numerical type?
Options
A – int
B – char
C – float
D – short
E – double
Ans: Option B - char
Q4. Which one of the below is not the integer value? Options
A - 56
B - 78.90
C - 08
D - '67'
E - 103.45
Ans: Option D - '67'
Q5. What are the data types of the following values?
Options
A - 56
B - 78.90
C - "08"
D - '6'
Ans:
A - Int
B - Double
C - String
D - Char
1. Find the syntax error in the below program:
public static void main(String[] args) {
System.out.println("ToolsQA")
}
Resolution: Missing semi-colon(;), correct statement is:
System.out.println("ToolsQA");
2. Find the syntax error in the below program:
public static void main(String[] args) {
System.out.println('ToolsQA');
}
Resolution: Missing single quote( ' ) instead of double quotes( " ) around the string, Correct statement is:
System.out.println("ToolsQA");
3. Find the error in the below program:
public static void main(String[] args) {
integer value = 18;
System.out.println("The value of the integer variable is : " + value);
}
Resolution: Integer is not a data type, it should be int;
int value = 18;
4. Find the errors in the below program:
public static void main(String[] args) {
valueInt int = 18;
valueDob double = 10.10;
valueBool boolean = true;
}
Resolution: Data types declarations are not correct, these should be:
int valueInt = 18;
double valueDob = 10.10;
boolean valueBool = true;
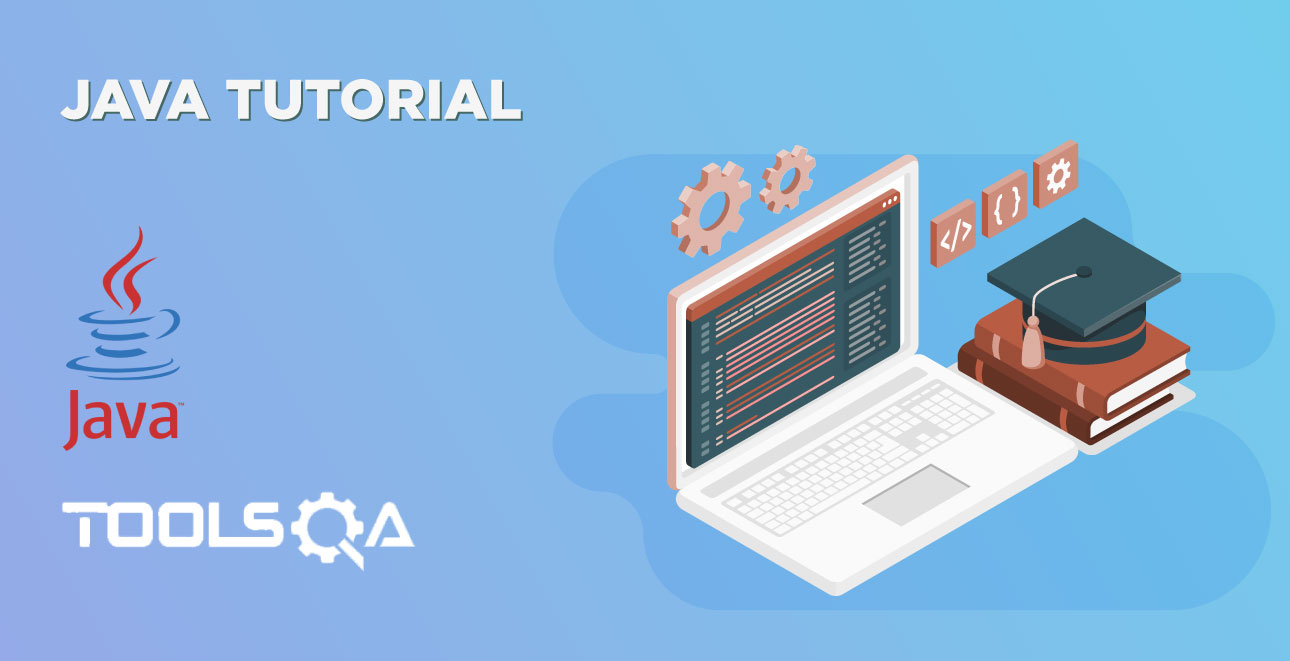
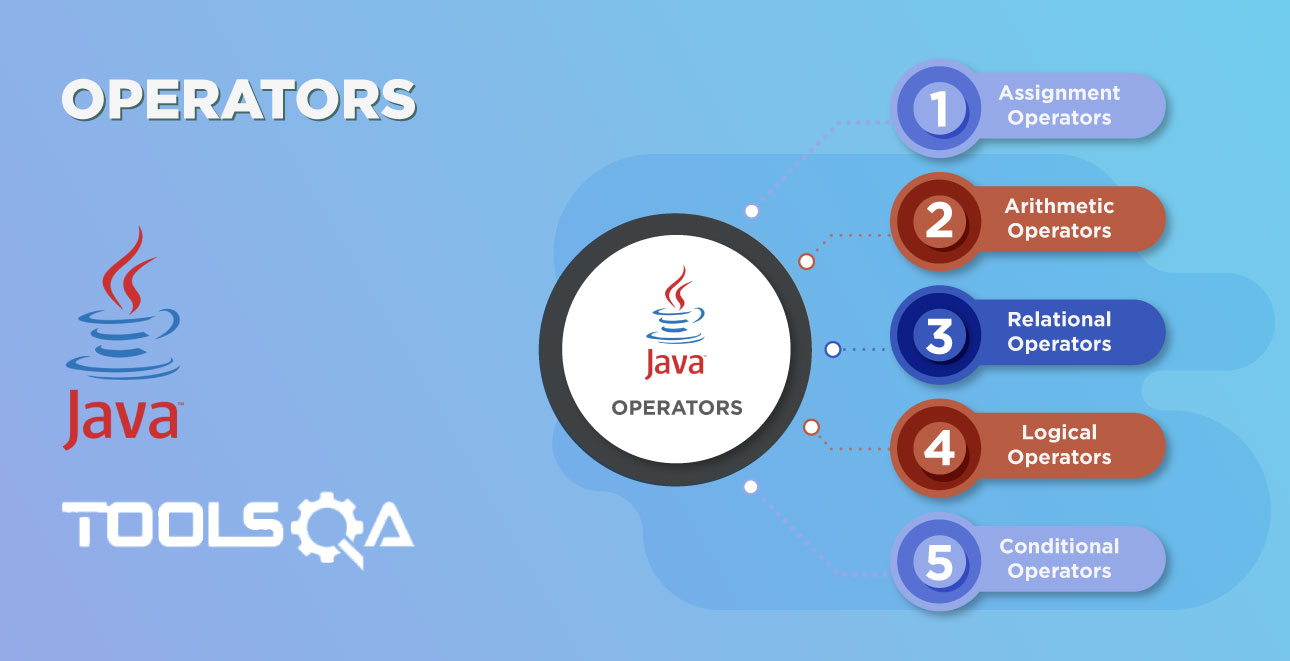