What are Constructors in Java?
Constructors in Java is a method that is used to set initial values for field variables. In Java when the object is created, the compiler calls the constructor first. It means any code written in the constructor will then get executed. On top of it, there is no need to make any special calls to a constructor method - it happens automatically at the run time when the compiler creates a new object.
Constructor methods take the same name as the class.
public class Car {
//Class Member Variables & Fields
String sModel;
int iGear;
int iHighestSpeed;
String sColor;
int iMake;
boolean bLeftHandDrive;
String sTransmission;
int iTyres;
int iDoors;
//This is the syntax to create a Constructor
public Car(){
}
}
So, if you have noticed in the above example, the name of the constructor is 'Car'. Which is exactly the same name as the class itself. Unlike normal methods, class constructors don't need a return type like int or double, nor any return value.
Note: If we do not explicitly write a constructor for a class the Java compiler builds a default constructor for that class.
How to Pass Values to the Constructor in Java?
We can pass values to the constructor.
public class Car {
//Class Member Variables & Fields
String sModel;
int iGear;
int iHighestSpeed;
String sColor;
int iMake;
boolean bLeftHandDrive;
String sTransmission;
int iTyres;
int iDoors;
//Constructor with values passed
public Car(String Model, int Make,boolean LeftHandDrive ){
sModel = Model;
iMake = Make;
bLeftHandDrive = LeftHandDrive;
}
//Method
public void DisplayCharacterstics(){
System.out.println("Model of the Car: " + sModel);
System.out.println("Number of gears in the Car: " + iGear);
System.out.println("Max speed of the Car: " + iHighestSpeed);
System.out.println("Color of the Car: " + sColor);
System.out.println("Make of the Car: " + iMake);
System.out.println("Transmission of the Car: " + sTransmission);
}
}
Main Method
public class ConstructorTestExercise {
public static void main(String[] args) {
//Use the Car keyword to declare Car Class variable
//Passing values to the constructor
Car Toyota = new Car("Camry",2014,true);
Toyota.iDoors = 4;
Toyota.iGear = 5;
Toyota.iHighestSpeed = 200;
Toyota.iTyres = 4;
Toyota.sColor = "Black";
Toyota.sTransmission = "Manual";
//Using Car class method
Toyota.DisplayCharacterstics();
}
}
Above code will produce:
Model of the Car: Camry
Number of gears in the Car: 5
Max speed of the Car: 200
Color of the Car: Black
Make of the Car: 2014
Transmission of the Car: Manual
How to assign default values to Constructor in Java?
It's a good idea to just set some default values to the field variables. These values will then be assigned when the object is created. Add the following code in the constructor:
Car Class
public class Car {
//Class Member Variables & Fields
String sModel;
int iGear;
int iHighestSpeed;
String sColor;
int iMake;
boolean bLeftHandDrive;
String sTransmission;
int iTyres;
int iDoors;
//Default values set in Constructor
public Car(){
sModel = "Camry";
iMake = 2014;
bLeftHandDrive = true;
}
public void DisplayCharacterstics(){
System.out.println("Model of the Car: " + sModel);
System.out.println("Number of gears in the Car: " + iGear);
System.out.println("Max speed of the Car: " + iHighestSpeed);
System.out.println("Color of the Car: " + sColor);
System.out.println("Make of the Car: " + iMake);
System.out.println("Transmission of the Car: " + sTransmission);
}
}
Main Method
public class ConstructorTestExercise {
public static void main(String[] args) {
//Use the Car keyword to declare Car Class variable
Car Toyota = new Car();;
Toyota.iDoors = 4;
Toyota.iGear = 5;
Toyota.iHighestSpeed = 200;
Toyota.iTyres = 4;
Toyota.sColor = "Black";
Toyota.sTransmission = "Manual";
//Using Car class method
Toyota.DisplayCharacterstics();
}
}
The above code will produce again the same result:
Model of the Car: Camry
Number of gears in the Car: 5
Max speed of the Car: 200
Color of the Car: Black
Make of the Car: 2014
Transmission of the Car: Manual
Why do we need a Constructor?
There can be a situation where exposing the class variable to the main program is not secure. At that point of time, class variables can be turned to private because the private variables are not accessible from the other classes. In this situation, if the constructors are defined, then the main method would not need to access class variables directly.
Car Class
public class Car {
//Class Member Variables & Fields
//Private variables
private String sModel;
private int iMake;
private int iGear;
int iHighestSpeed;
String sColor;
boolean bLeftHandDrive;
String sTransmission;
int iTyres;
int iDoors;
//Default values set in Constructor
public Car(){
sModel = "Camry";
iMake = 2014;
iGear = 5;
}
public void DisplayCharacterstics(){
System.out.println("Model of the Car: " + sModel);
System.out.println("Number of gears in the Car: " + iGear);
System.out.println("Max speed of the Car: " + iHighestSpeed);
System.out.println("Color of the Car: " + sColor);
System.out.println("Make of the Car: " + iMake);
System.out.println("Transmission of the Car: " + sTransmission);
}
}
Main Method
public class ConstructorTestExercise {
public static void main(String[] args) {
//Use the Car keyword to declare Car Class variable
Car Toyota = new Car();;
Toyota.iDoors = 4;
Toyota.iHighestSpeed = 200;
Toyota.iTyres = 4;
Toyota.sColor = "Black";
Toyota.sTransmission = "Manual";
Toyota.bLeftHandDrive = true;
//Using Car class method
Toyota.DisplayCharacterstics();
}
}
Another situation can be where few class variables are very important for the class object and the programmer wants to make sure that who so ever uses the class and create the object of the class, does not forget to initialize these important variables.
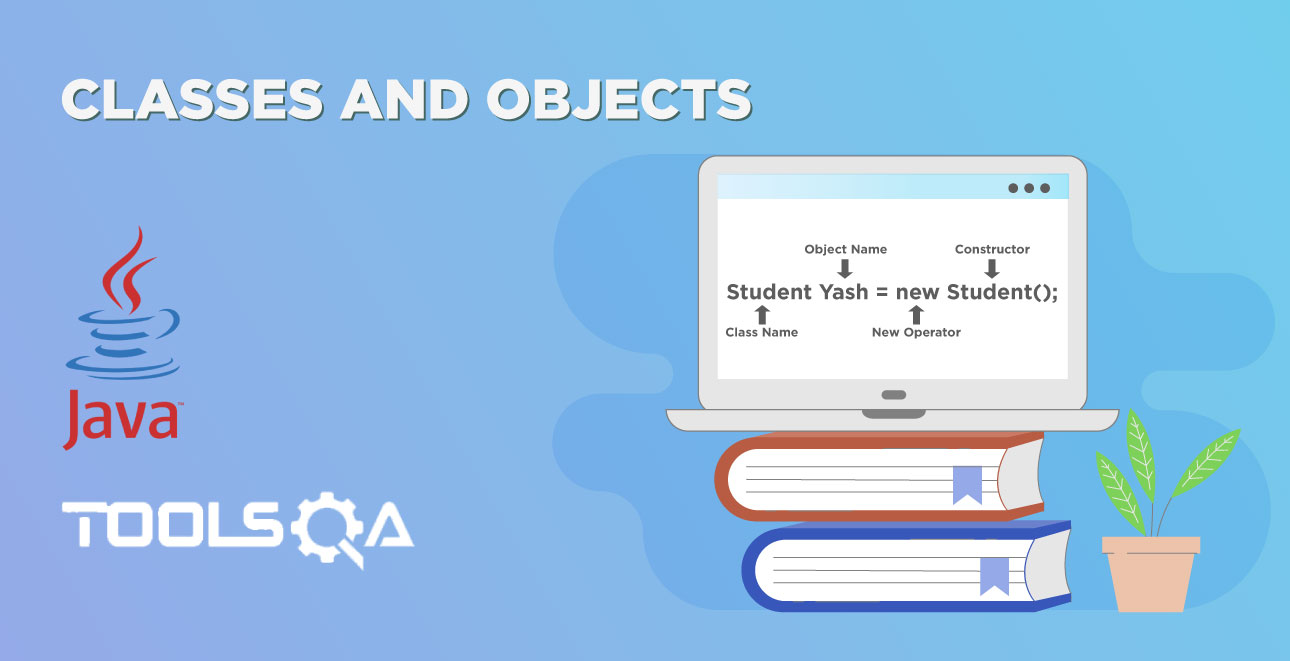
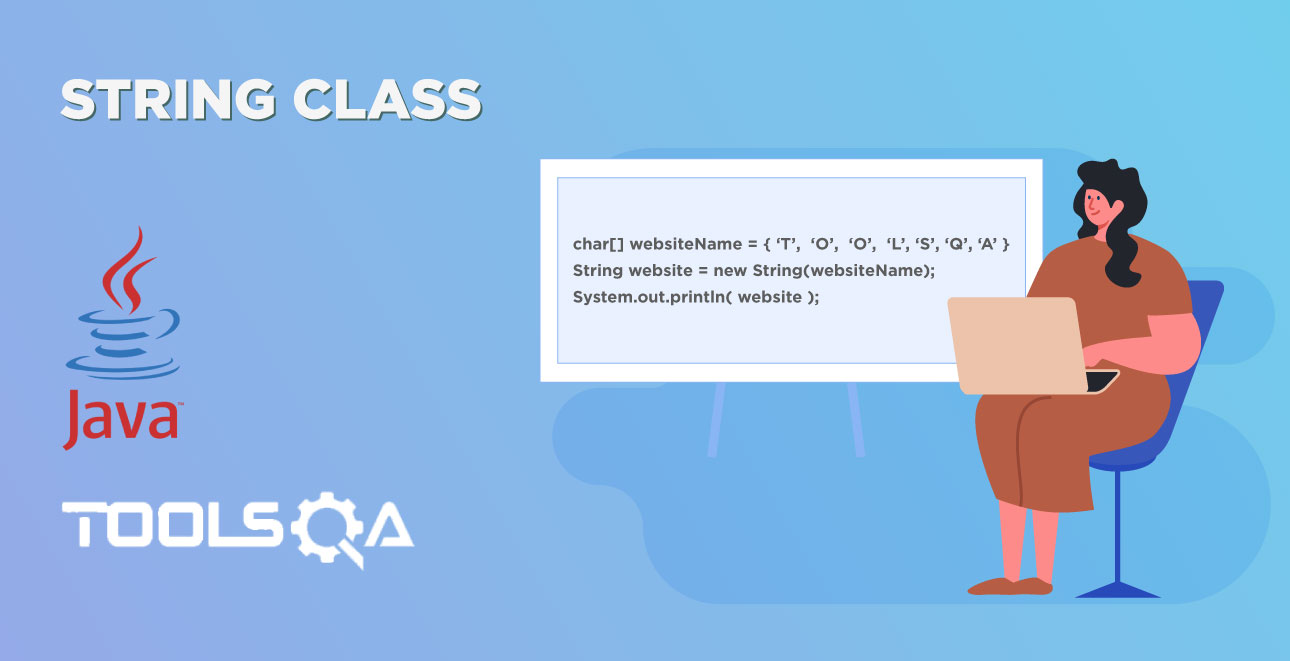