Non Access Modifiers:
Java provides a number of non-access modifiers to achieve many other functionality.
The static modifier for creating class methods and variables.
The final modifier for finalizing the implementations of classes, methods, and variables.
The abstract modifier for creating abstract classes and methods.
The synchronized and volatile modifiers, which are used for threads.
Static Modifier
Static keyword can be used for variables, methods, blocks and nested classes. It cannot be used for classes. The advantage of the static keyword in Java is efficient memory management. The static keyword belongs to the class rather than the instance of the class, which means it is not required to instantiate the class to access its fields. For e.g. to use a static variable & method of any class, all you need to do is to write class name followed by the static variable like this:
ClassName.StaticVariabe;
ClassName.Method();
Car.Speed;
Car.DisplayCharacterstics();
Why it is memory efficient is because the memory allocation that happened to the static variable is just one time in the whole program. And all the classes accessing the static variable are referring to the same memory location. Memory allocation happened for all the static variables at the start of the program. Unlike class members where memory allocation happened at the time of instantiating the class objects.
The static keyword can be used for the following:
- variable (also known as class variable)
- method (also known as class method)
- block
- nested class
Example for Static Variable: Static means fixed, so if you have any variable whose value is fixed throughout the program you can use the static keyword in its declaration. For e.g. as we are using car class in our previous chapters. Think of the Car properties which remain the same for every car object. Car steering and the Car wheels are the properties that you can use as static because every car object you will create will always have only one steering and four wheels.
Car Class with Static Variables
package vehicle;
public class Car {
public String sModel;
public int iHighestSpeed;
public static int iSteering;
public static int iWheels;
public static int iDoors;
public Car(){
iSteering = 1;
iWheels = 4;
}
}
Explanation: Above is a Car class in which two class members/fields (sModel & iHighestSpeed) and three static variables (iSteering, iWheels & iDoors) are declared. Notice that we have initialized the values of the class variables (iSteering & iWheels) inside the Car class only. And it makes sense too because these values will remain same for the whole program. Although we have one more class variable left, which is iDoors. We will assign a value to it in the other class for illustration purposes. As the doors of the Car can be two or four. Maybe we are interested in creating two doors cars only. In the below code we will create an object of the Car class and will assign the values of its members (sModel & iHighestSpeed).
Main Program
package javaPracticeProgram;
import vehicle.Car;
public class TestStaticModifiers {
public static void main(String[] args) {
Car Toyota = new Car();
Toyota.sModel="Camry";
Toyota.iHighestSpeed = 230;
Car.iDoors = 2;
System.out.println("Model of the Car: " + Toyota.sModel);
System.out.println("Max speed of the Car: " + Toyota.iHighestSpeed);
System.out.println("Number of Wheels in the Car: " + Toyota.iWheel); //Compile Time Error
System.out.println("Number of Wheels in the Car: " + Car.iWheel);
System.out.println("Number of Steering in the Car: " + Car.iSteering);
System.out.println("Number of Doors in the Car: " + Car.iDoors);
}
}
Explanation: In the above example, a new Car class object is created as Toyota. Then assigned values to the members of Toyota Car. You can see that static variable iDoors is initialized in this class. This proofs that static member value can also be initialized and change in other classes.
In the print statements, we tried to access the iWheel static variable with Toyota object but a compile-time error is thrown. As the variable is declared as static we would not be able to use it with instanced class. It is only accessible through its original class and which is the Car class. In the last three print statements, we printed the values for wheel, steering, and doors. You will see their output as 1, 4 & 2 in the console for the last three statements.
As I said above, all the static variables initialized during the start of the program. So when you run the TestStaticModifiers class, the first thing it did is to initialize all the class variables and allocate the right amount of memory.
Example of a Static Method: If the method has a static modifier in the declaration, it is called as Static Method. The basic functionality of the static keywords remains same for variables & methods means a static method belongs to the class rather than the object of the class. It means that a static method can be used without instantiating the class. Simply refer the original class name followed by static method. the most important rule for static method is that you cannot use the non-static members inside the static method. Only local variables & static/class variables can be used in the static method.
Car Class with STatic Method
package vehicle;
public class Car {
public String sModel;
public int iHighestSpeed;
public static int iSteering;
public static int iWheel;
public static int iDoors;
public Car(){
iSteering = 1;
iWheel = 4;
}
public void DisplayCharacterstics() {
System.out.println("Model of the Car: " + sModel);
System.out.println("Max speed of the Car: " + iHighestSpeed);
System.out.println("Number of Steering in the Car: " + iDoors);
}
public static void DisplayStaticCharacterstics() {
DisplayCharacterstics();//Compile time error
System.out.println("Max speed of the Car: " + iHighestSpeed);//Compile time error
System.out.println("Number of Wheels in the Car: " + iWheel);
System.out.println("Number of Steering in the Car: " + iSteering);
}
}
Explanation: In the above Car class, there are two methods (DisplayCharacterstics & DisplayStaticCharacterstics). DisplayStaticCharacterstics method is a static method. You will see that in the DisplayStaticCharacterstics method we tried to access a class member (iHighestSpeed) but a run time error is thrown because as discussed above only static & local variables can be accessed in static methods. But a static variable (e.g. iDoor) can be accessed outside the static method. The same way only static methods are accessible within the static method.
Main Program
package javaPracticeProgram;
import vehicle.Car;
public class TestStaticModifiers {
public static void main(String[] args) {
Car Toyota = new Car();
Toyota.sModel="Camry";
Toyota.iHighestSpeed = 230;
Car.iDoors = 2;
Car.DisplayStaticCharacterstics();
Toyota.DisplayCharacterstics();
}
}
Explanation: Notice that static method DisplayStaticCharacterstics is accessible with just the class name. The way we use the class variable we use class methods. No need to instantiated a Car class. Whereas for DisplayCharacterstics method we had to instantiate a new car class as Toyota to use its method.
Example for Static block: Block is nothing but a piece of code written between parenthesis {}. If the static modifiers is used in the declaration of the block, it means that the piece of code will run first when the program is started, it will execute before the main method. If a block is static, it almost works like the class constructors.
Car Class with Static Block
package vehicle;
public class Car {
public String sModel;
public int iHighestSpeed;
public static int iSteering;
public static int iWheel;
public static int iDoors;
static {
iSteering = 1;
iWheel = 4;
System.out.println("This block executed first");
}
public static void DisplayCharacterstics() {
System.out.println("Number of Wheels in the Car: " + iWheel);
System.out.println("Number of Steering in the Car: " + iSteering);
}
}
Main Program
package javaPracticeProgram;
import vehicle.Car;
public class TestStaticModifiers {
public static void main(String[] args) {
Car.DisplayCharacterstics();
}
}
Output: The output of the code will be like :
This block executed first
4
1
Explanation: A static block is defined in the above Car class. This static block is initializing/assigning the value to its class variables (iSteering & iWheel). This piece of code will run, as soon as the run command is fired on the main method. DisplayCharacterstics method is called first on TestStaticModifiers class, still, the print log mentioned on the static block displayed first on the output console.
Final Keyword
Final in java is a very important keyword and can be applied to classes, methods, and variables in Java. Final is often used along with static keyword in Java to make static final constant and you will see how final in Java can increase the performance of Java application.
Example of a Final Class: If the Final modifier is used in the declaration of the class, it means the class is final and cannot be sub-classed or inherited. When we say it cannot be inherited means no other class would be able to extend this class.
Car Class with Final Keyword
package vehicle;
public final class Car {
// Code goes here
}
Explanation: Above Car class is declared as final, it means what so ever class tries to extend this class would get an error. In the below SmartCar class, we will try to extend Car class.
SmartCar Class
package vehicle;
public class SmartClass extends Car { // Remove final modifier of Car
// Code goes here
}
Explanation: We tried to extend the Car class but a compile-time error is thrown. But an object can be created of a class which is declared as final just like a regular class. Example of a Final Method: If a Final modifier is used in the declaration of a method, it means the method cannot be overridden. If you think that the method is complete and would not need to modify in any of the sub-class then the method can be declared as final method.
package vehicle;
public class Car {
public void DisplayCharacterstics_1(){
//Code goes here
}
public final void DisplayCharacterstics_2(){
//Code goes here
}
}
Explanation: Notice we have two methods in the above Car class (DisplayCharacterstics_1 & DisplayCharacterstics_2). One is declared as normal and the other is declared as final. In the below code we will extend the Car class and try to Override its methods. For the sake of this example, please ignore keyword override, we will discuss this in detail in the later chapters. Changing the logic or the implementation is called override.
SmartCar Extending Car Class
package vehicle;
public class SmartClass extends Car{
@Override
public void DisplayCharacterstics_1(){
//Code goes here
}
@Override
public void DisplayCharacterstics_2(){ //Compile Time Error
//Code goes here
}
}
Explanation: In the above code, we successfully override the method DisplayCharacterstics_1. But a compile-time error is thrown, when we tried to override the method DisplayCharacterstics_2. As the second method is declared as final, we cannot change its implementation and override annotation will not work on it.
Example of Final Variable: If the final modifier is used in the declaration of the variable, it means the variable value is fixed and cannot be changed. A final variable can be initialized only once. The final keyword can be used for local variables as well which initialized within the block. A blank final field cannot be initialized, whenever you declare a final variable, you must assign it a value.
package vehicle;
public class Car {
public String sModel;
public int iHighestSpeed;
public final int iSteering=1;
public static final int iWheels=4;
}
Exmaplantion : In the above Car class we have declared two of the fields (iSteering & iWheels) are final. In the below code we will try to change the value of these final variables.
Main Program
package javaPracticeProgram;
import vehicle.Car;
public class TestStaticModifiers {
public static void main(String[] args) {
Car Toyota = new Car();
Toyota.sModel="Camry";
Toyota.iHighestSpeed = 230;
Toyota.iSteering = 2; //Compile time error
Car.iWheels = 6; //Compile time erro
}
}
Explanation: You can see that when we tried to modify the values of final variables (iSteering & iWheels), run time error is thrown for both the variables. This also concludes that when the variable is final, then it makes sense to declare the variable as static. As it will always refer to the same memory location.
Final Static Variable
Final variables are often declared with static keyword in java and treated as constant. As we discussed the static keyword above in this chapter that static variables have the same memory ref throughout the program and most of the time when we use static variable because we want to allocate a fixed value to it. For ex we used the static keyword for iWheels, as we always have four wheels in the car. For that, we can also use final, as the value for wheel will never expect to change.
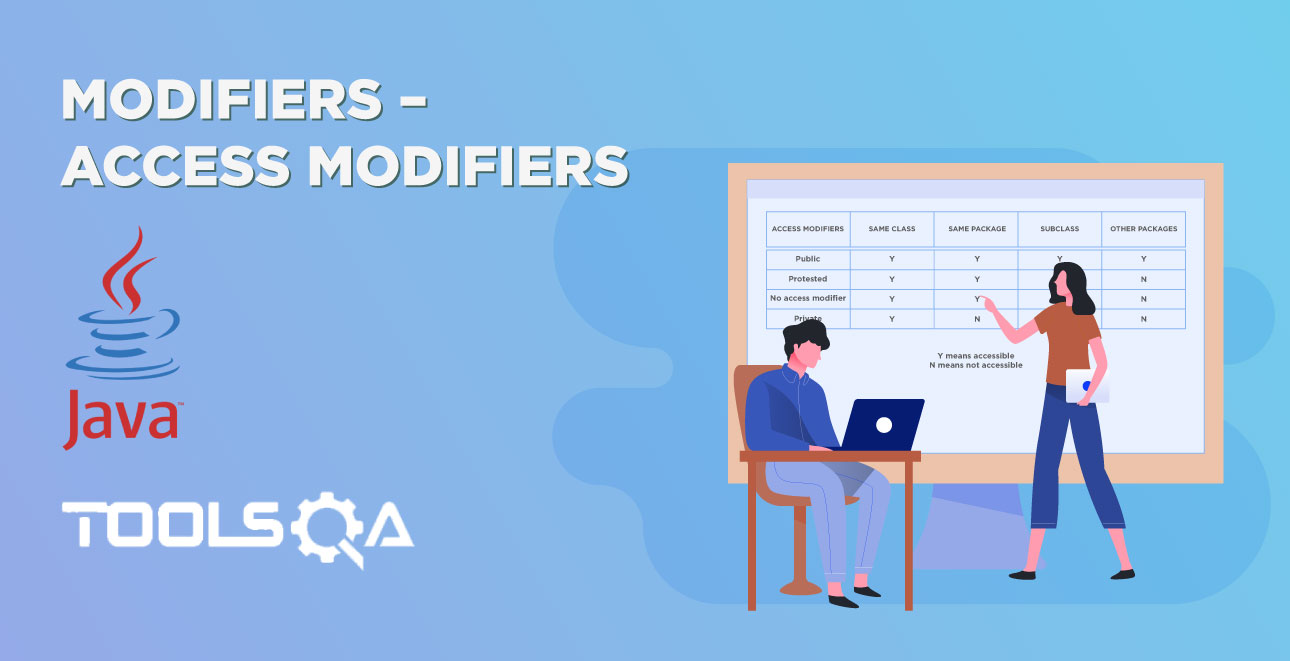
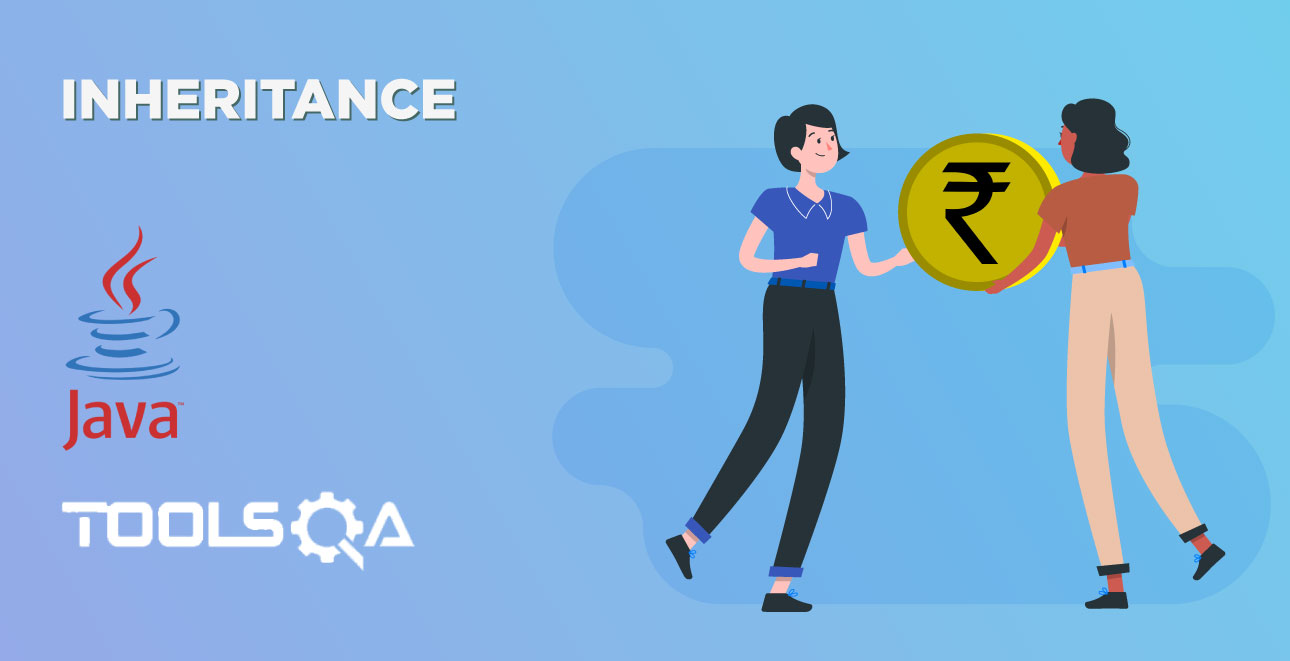