What is a String?
String is nothing but a sequence of characters. The String is a Class and not a data type like 'int & char'. So the String class represents the characters of String and all string objects in java are the instance of this class. The string is the most usable class in java programming and in Selenium WebDriver.
The most important fact about the String class that it is an immutable class, which means once it is created a String object can not be changed.
How to create a String in Java?
The way we declare any other data type, the same way we declare String as well. A string is always be described in the double-quotes.
String WebSite = "ToolsQA.com";
As with any other object, you can also create String objects by using the new keyword and a constructor.
char[] webSiteName = { 'T', 'O', 'O', 'L', 'S', 'Q', 'A'};
String webSite = new String(webSiteName);
System.out.println( webSite );
This would produce: TOOLSQA
String Manipulations
As we have already discussed above that string is the most important class in Java and it has got many methods. You will be using all these methods very frequently in Selenium Automation or Java Programming.
String Length:
This length method returns the number of characters in the string. It counts every alphanumeric char, special char or even spaces.
public class StringTestExercise {
public static void main(String[] args) {
//Declaring the String and Int Variable
String sPopularTopic = "Selenium Automation Framework";
int ilength = sPopularTopic.length();
//Print the value of String variable & int Variable
System.out.println("Popular Topic of ToolsQA: " + sPopularTopic);
System.out.println("Length of the Popular Topic: " + ilength);
}
The above will produce:
Popular Topic of ToolsQA: Selenium Automation Framework
Length of the Popular Topic: 29
Concatenating String
String class gives you the method to join two strings.
public class StringTestExercise {
public static void main(String[] args) {
//Declaring the String Variables
String sPopularTopic_1 = "Selenium Automation Framework";
String sPopularTopic_2 = "Basic Java Tutorial";
String sSpace = " ";
//Print the value of Concat String
//String1.concat(String2);
System.out.println("First Output");
System.out.println(sPopularTopic_1.concat(sPopularTopic_2));
//Another way of using String Concat
//"String1".concat(String2);
System.out.println("Second Output");
System.out.println("Selenium Automation Framework".concat(sPopularTopic_2));
//Another way of using String Concat
//"String1" + "String2";
System.out.println("Third Output");
System.out.println("Selenium Automation Framework" + "Basic Java Tutorial");
//Another way of using String Concat
//"String1" + String + "String2";
System.out.println("Fourth Output");
System.out.println("Selenium Automation Framework" +sSpace+ "Basic Java Tutorial");
}
}
The above code would produce:
Selenium Automation FrameworkBasic Java Tutorial
Second Output
Selenium Automation FrameworkBasic Java Tutorial
Third Output
Selenium Automation FrameworkBasic Java Tutorial
Fourth Output
Selenium Automation Framework Basic Java Tutorial
Various String Methods
Let's take an example and cover all the important String operations.
public class StringTestExercise {
public static void main(String[] args) {
//Declaring the String
String sPopularTopic_1 = "Selenium Automation Framework";
String sPopularTopic_2 = "Basic Java Tutorial";
//Compare two String: This would compare two strings
//If the twos strings are equal, it will return 'true' else it will return 'false'
boolean bCompareResult = sPopularTopic_1.equals(sPopularTopic_2);
System.out.println("The result of String Comparison is : " + bCompareResult);
//Character at: This would return the single character at index value from the String
char cIndex = sPopularTopic_1.charAt(5);
System.out.println("The fifth character of Popular Topic 1 is : " + cIndex);
//Contains: This would return 'true' if the passed string is contained in the another String
boolean bContainResult = sPopularTopic_1.contains("Framework");
System.out.println("The result of string Framework is contained in the String sPopularTopic_1 is : " + bContainResult);
//Index of: This would return the starting index of the passed string
int iIndex = sPopularTopic_1.indexOf("Framework");
System.out.println("The start index of the string Framework is : " + iIndex);
//Sub String First index: This would return the sub string of the string from the passed index number
String sSubString = sPopularTopic_1.substring(iIndex);
System.out.println("The sub string from the index number is : " + sSubString);
//Sub String First & Last index: This would return the sub string from first index to end index
sSubString = sPopularTopic_1.substring(8, 19);
System.out.println("The sub string of Popular Topic 1 from index 8 to 18 is : " + sSubString);
//To Lower Case: It would return the complete string in the lower case
String sLowerCase = sPopularTopic_1.toLowerCase();
System.out.println("The lower case of the Popular Topic 1 is : " + sLowerCase);
//Split: It breaks the string into two parts from the passed argument and stores it into an array
String [] aSplit = sPopularTopic_1.split("Automation");
System.out.println("The first part of the array is : " + aSplit[0]);
System.out.println("The last part of the array is : " + aSplit[1]);
}
}
The above code would produce:
The result of String Comparison is : false
The fifth character of Popular Topic 1 is : i
The result of string Framework is contained in the String sPopularTopic_1 is : true
The start index of the string Framework is : 20
The substring from the index number is : Framework
The substring of Popular Topic 1 from index 8 to 18 is : Automation
The lower case of the Popular Topic 1 is : selenium automation framework
The first part of the array is : Selenium
The last part of the array is : Framework
Still, there are many more methods are left with the String class, take a look over all the methods, so that you would be able to know what you are capable of doing with the String object. When you type any string variable and type dot, it populates all the available methods of the object.
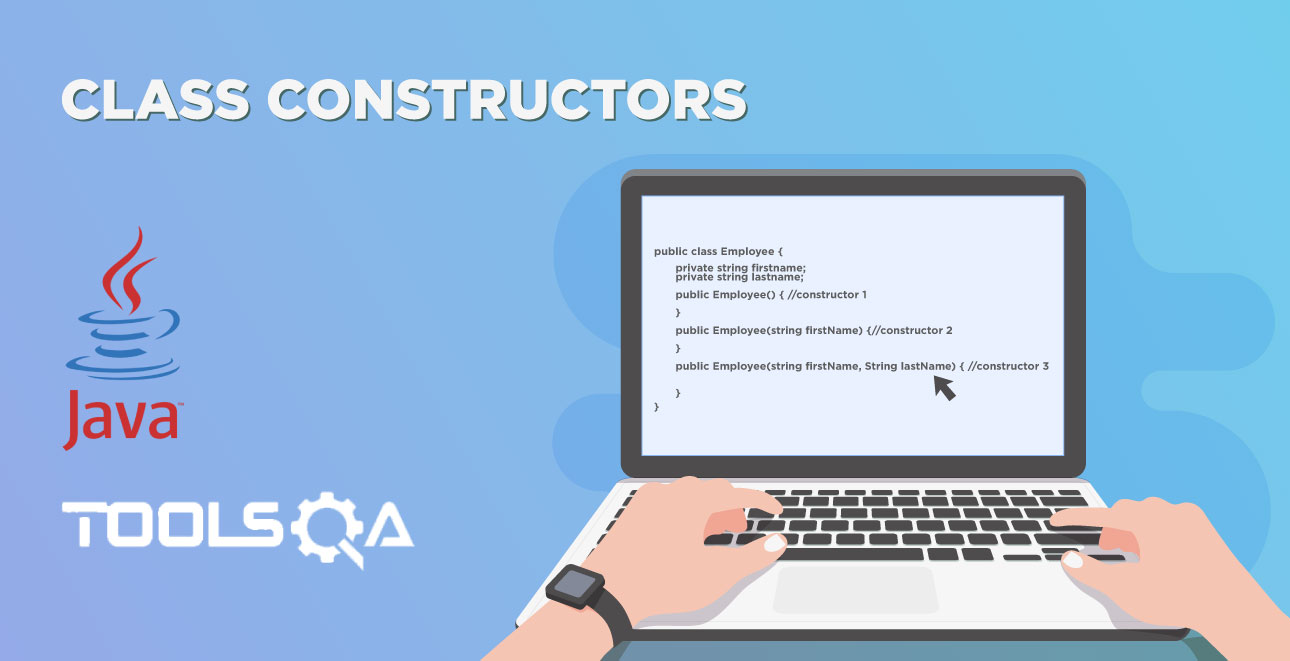
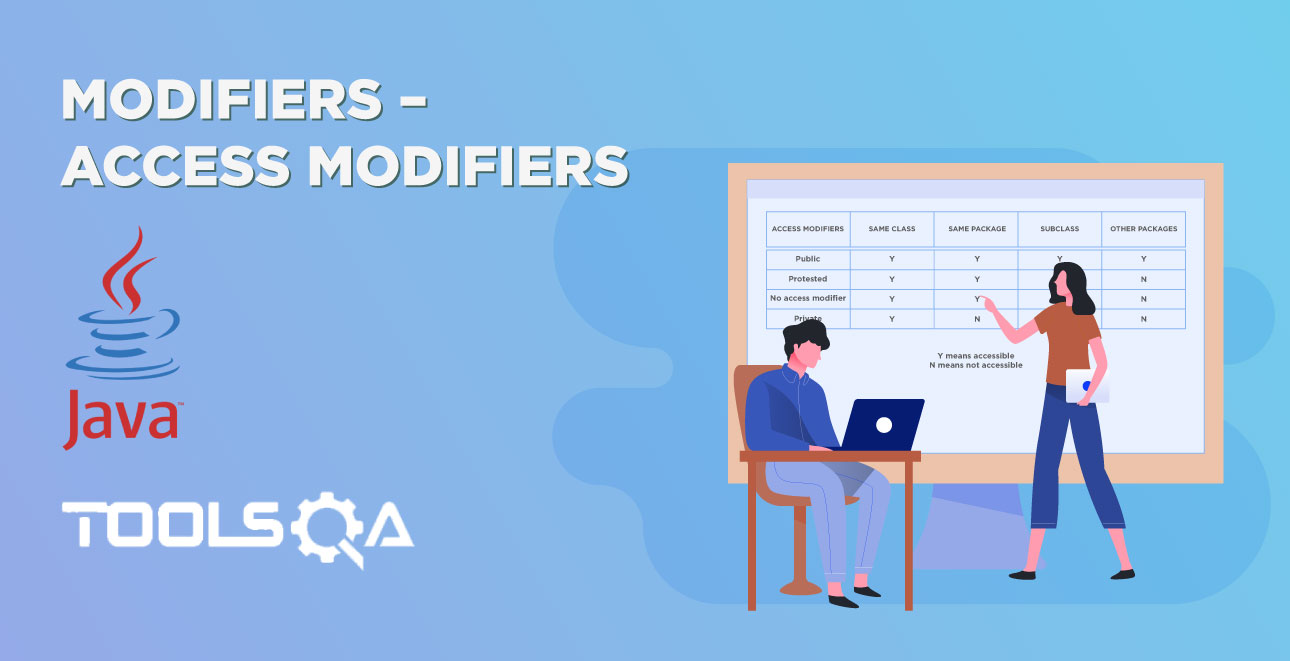