In the previous article of Protractor Test Script, we learned about executing protractor tests. We used the javascript code as a sample snipped. As the complexity increases javascript code is quite difficult to maintain. Especially if you are switching from OOPS based language like C# or Java to Javascript. In this article let's discuss typescript:
What is TypeScript?
TypeScript is an open-source programming language developed and maintained by Microsoft. It is a strict syntactical superset of JavaScript and adds optional static typing to the language. If you are aware of OOPS principles,typescript support all OOPS concepts just like C# and Java. It is a typed superset, which means it provides an additional layer to javascript. So that it will be easy for developers coming from another programming background and knows the OOPS concept.
For example: Let's create a simple class using Javascript:
var Greeter = (function () {
function Greeter(message) {
this.greeting = message;
}
return Greeter;
}());
above code looks quite complicated right?
Let's see how to do the same in Typescript:
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
}
In typescript, it's almost similar how we create a class in C# or Java.
What is pre-requisite to learning TypeScript?
1. It is built based on OOPS concepts so you should be familiar with OOP concepts.
2. Since this language is a typed superset of JavaScript you need to know JavaScript.
Note: Microsoft has very well documented typescript so we are not going to cover this in-depth. Read thedocumentation from MS which helps you understand it better.
How to install TypeScript?
It is easy to install, you just need to add it as a node-module. Open your command prompt simply type command
npm install -g typescript
Note: In order to install it, you need to have node installed on your machine. Node installation is covered in the chapter of Set Up Protractor.
Verify the version once you installed, use command tsc -v to verify.
If you don't see the version output like above, means it is not installed.
How does typescript work?
Look at the above image,
- A file called hello.ts is a ts file
- When compiling the ts file with compiler using tsc command
- The compiler automatically generates a javascript file with the same name as hello.js.
Let's do it practically, Create a ts file(example hello.ts) add some sample code like below:
//hello.ts
class Greeter {
greeting: string;
constructor() {
this.greeting = "World";
}
greet() {
return "Hello, " + this.greeting;
}
}
let greeter = new Greeter();
console.log(greeter.greet());
How to compile typescript?
TypeScript's commands can be run in the command prompt. Command to compile into java is: tsc <ts_file>
After successful compilation, the equivalent javascript .js file will be generated (i.e hello.js in our example) in the same folder.
hello.js will look like this:
//hello.js
//Auto Generated code by tsc hello.ts
var Greeter = /** @class */ (function () {
function Greeter() {
this.greeting = "World";
}
Greeter.prototype.greet = function () {
return "Hello, " + this.greeting;
};
return Greeter;
}());
var greeter = new Greeter();
console.log(greeter.greet());
If you want to verify the generated javascript, open your command prompt and type node hello.js. Your console shows the output "Hello World"
Understanding the basics helps you build a good automation framework. Now you know the basics of typescript, you can explore yourself try it out various things. I even recommend you to use the typescript playground using link https://www.typescriptlang.org/play/index.html.
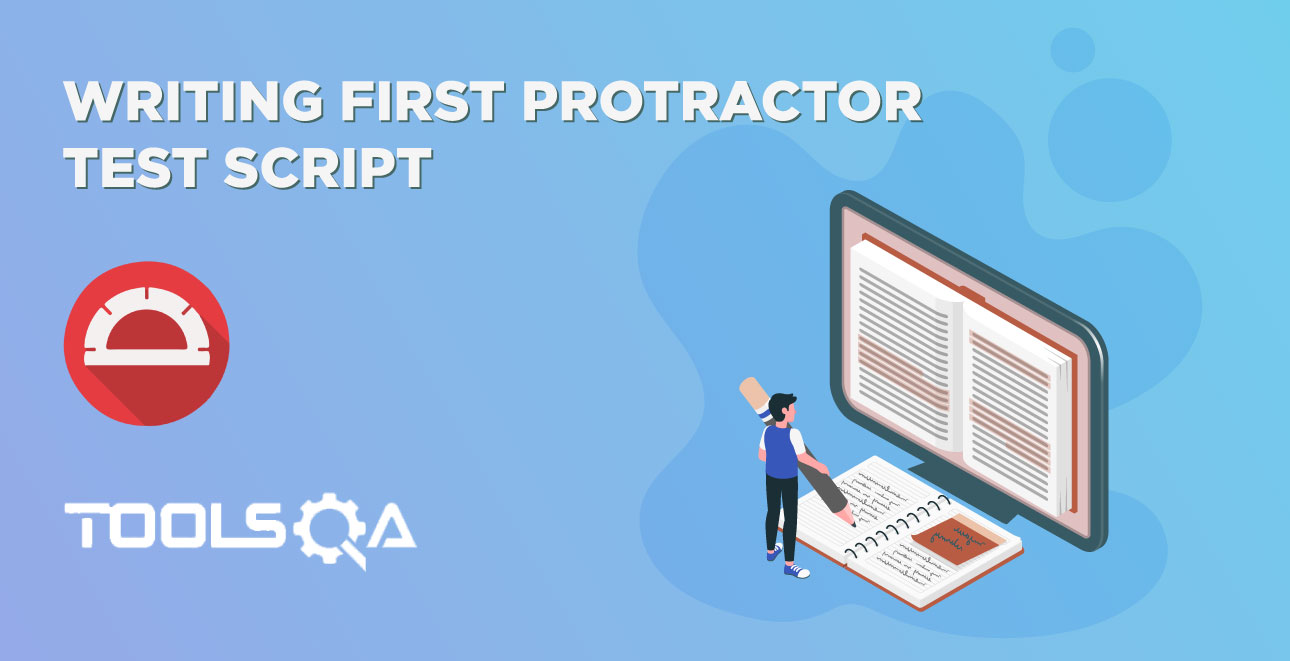
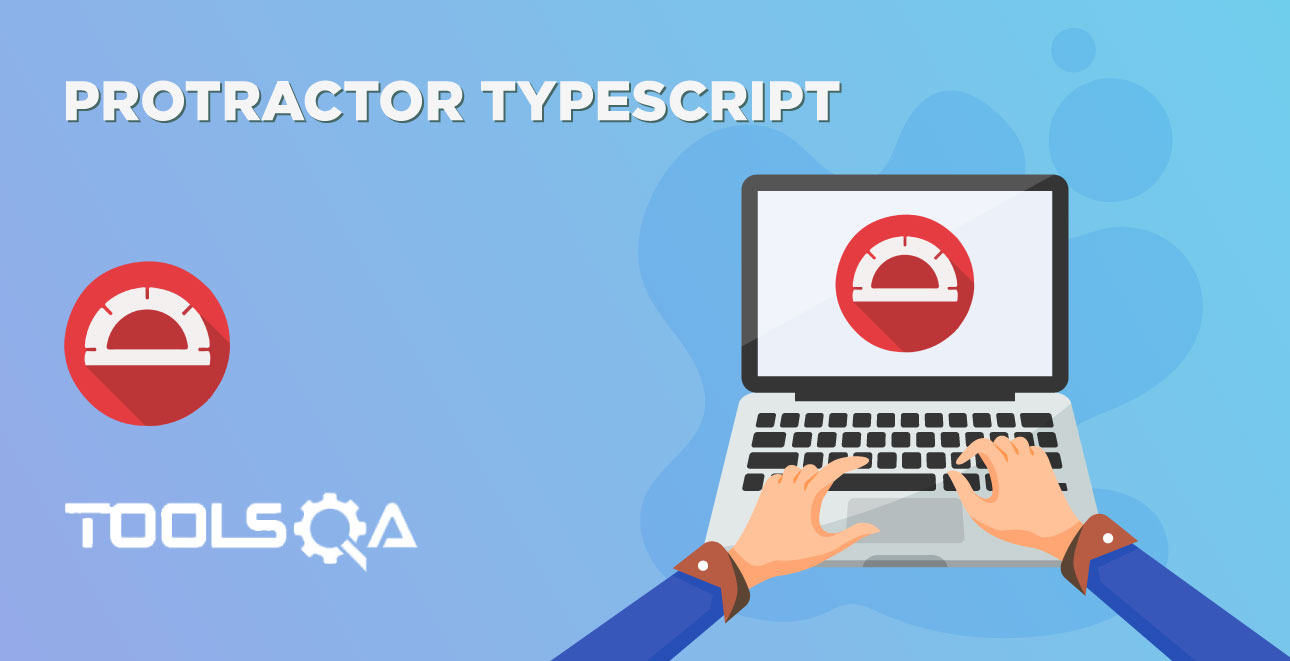