In the previous article, we discussed Element Finder in Protractor. Additionally, ElementFinder is used to get a single element from the webpage. Subsequently, in this article, we will discuss ElementArrayFinder in Protractor. Moreover, we will use it to find and get multiple elements from the webpage as an array. Throughout the article, we will discuss:
- What is ElementArrayFinder in Protractor?
- How to Find sub-elements using an array of elements?
- How to Get the first element from ElementArrayFinder?
- Procedure to Get the last element from ElementArrayFinder?
- How to Get an element by index using ElementArrayFinder?
- How to Iterate through an array of elements returned by Protractor ElementArrayFinder?
- Procedure to use the Count function of ElementArrayFinder in Protractor?
What is ElementArrayFinder in Protractor?
ElementArrayFinder is an array of WebElements which sets up a chain of conditions that identify an array of elements. Additionally, it allows the performance of actions on them. The action will apply to each element identified by the ElementArrayFinder. Moreover, an ElementArrayFinder will wait for the angular app to load before performing finds or actions.
Syntax:element.all(locator):ElementArrayFinder
Parameter: This function takes one parameter called locator. Additionally, the locator can be protractor specific or webdriver inherited locator.
Returns: This function returns an array of elements of type ElementArrayFinder
Code example:
import { browser, by, element, ElementArrayFinder} from 'protractor'
describe('Protractor Element Array Finder Demo', function() {
it('Protractor Element Array Finder', function() {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
let sampleElements:ElementArrayFinder=element.all(by.repeater('section in menu.sections'))
});
});
Consequently, in the above example element.all(by.repeater('section in menu.sections')) returns array of elements which matches the locator by.repeater('section in menu.sections').
How to Find sub-elements using an array of elements?
ElementArrayFinder can be used to find all the sub-elements under an element by chaining the element(), and all() functions.
Syntax: element(locator).all(locator):ElementArrayFinder
Returns: Returns the array of elements of type ElementArrayFinder
Note: While chaining we can combine with first ElementFinder and ElementArrayFinder.
Example: element('FirstLocator').all('SecondLocator').all('ThirdLocator')
Code example:
//spec.ts
import { browser, by, element, ElementArrayFinder } from 'protractor'
describe('Protractor Element Array Finder Demo', function () {
it('ElementArray Finder demo', function () {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
let sampleElements: ElementArrayFinder = element(by.className('docs-menu')).all(by.repeater('section in menu.sections')) //chained locators sampleElement.then
sampleElements.then(() => {
sampleElements.getText().then((text) => {
console.log(text);
})
})
});
});
How to Get the first element from ElementArrayFinder?
Purpose: The first() function gets the first matching element for the ElementArrayFinder
Syntax: element.all(locator).first(): ElementFinder
Returns: This function returns an element representing the first matching element of type ElementFinder which is a single element.
Code Example:
//spec.ts
import { browser, by, element, ElementArrayFinder, ElementFinder} from 'protractor'
describe('Protractor Element Array Finder Demp', function() {
it('Finds First Element', function() {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
let sampleElement:ElementFinder=element.all(by.repeater('section in menu.sections')).first();
// Once we get the webelement we can perform any action on it like click, getText() etc.
});
});
How to Get the last element from ElementArrayFinder?
Purpose: The last() function gets last element. Additionally, it is available in the ElementFinder Array.
Syntax: element.all(locator).last(): ElementFinder;
Returns: This function returns an element representing the last matching element of type ElementFinder.
Code Example:
//spec.ts
import { browser, by, element, ElementArrayFinder, ElementFinder} from 'protractor'
describe('Protractor last element demo', function() {
it('Get last element', function() {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
let sampleElement:ElementFinder=element.all(by.repeater('section in menu.sections')).last();
// Once we get the webelement we can perform any action on it like click, getText() etc.
});
});
How to Get an element by index using ElementArrayFinder?
Purpose: The get() function gets an element within the ElementArrayFinder using the index. The index starts at 0. Additionally, Negative indices are available wrapped. That is to say; i mean ith element from last.
Syntax : element.all(locator).get(index: number | Promise<number>): ElementFinder;
Parameter: This function accepts Index, which is number, or Promise. Additionally, it will resolve to number.
Returns: This function returns the ElementFinder object available at the specified index
Code Example:
//spec.ts
import { browser, by, element, ElementArrayFinder, ElementFinder} from 'protractor'
describe('Protractor get element by index demo', function() {
it('Get element by index', function() {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
let sampleElement:ElementFinder=element.all(by.repeater('section in menu.sections')).get(0);
});
});
How to Iterate through an array of elements returned by Protractor ElementArrayFinder?
Purpose: The each() is used to iterate through an array of elements. Additionally, this function calls the input function on each ElementFinder represented by the ElementArrayFinder.
***Syntax: element.all(locator).each(eachFunction) :Promise<any>
; ***
or
Syntax:element.all(locator).each(fn: (elementFinder?:ElementFinder, index?:number) =>any):Promise<any>
;
Parameter: This function accepts another function as an input function. Moreover, optionally the input function can have arguments as elementFinder and index Returns: This function returns a promise that will resolve when the function has been called on all the ElementFinders.
Code Example:
//spec.ts
import { browser, by, element, ElementArrayFinder, ElementFinder } from 'protractor'
describe('Protractor each function demo', function () {
it('Each function demo', function () {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
element.all(by.className('docs-menu'))
.each((ele, index) => {
if (ele != undefined){
ele.getText().then((text) => {
console.log(index, text);
});
}
});
});
});
In the above example
- element.all(by.className('docs-menu')): We get all the elements which have class name docs-menu
- .each((ele, index): Subsequently, in this line of code follows the previous one. In other words, each() function helps to iterate all the items found in the previous line.
- ele.getText().then((text): Lastly, in this line of code, we are trying to get the text for the element.
Conclusively, in the above example, we are trying to get all the elements having a class name as docs-menu. After that, display the text of each of the elements.
How to use the Count function of ElementArrayFinder in Protractor?
Purpose: The count() function is used to count the number of elements represented by the ElementArrayFinder
Syntax: element.all(locator).count(): Promise<number>
;
Returns: This function returns promise that will be resolved to number.
Code example:
//spec.ts
import { browser, by, element, ElementArrayFinder, ElementFinder } from 'protractor'
describe('Protractor count function demo', function () {
it('Counts number of elements', function () {
browser.get("https://material.angularjs.org/latest/demo/autocomplete")
let elementArray:ElementArrayFinder= element.all(by.repeater('section in menu.sections'));
expect(elementArray.count()).toBeGreaterThan(5);
});
});
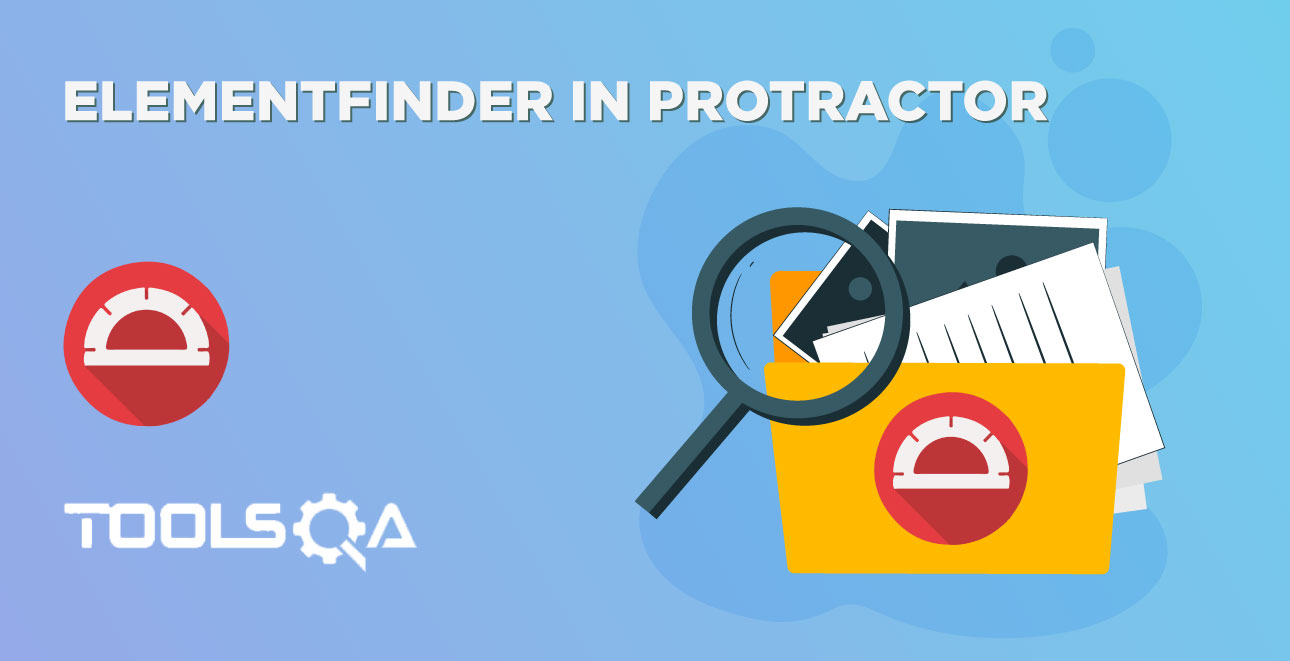