In the last two chapters of Tables (Transform Tables into Dictionary & Transform Table into DataTable we observed that data can be passed to the Steps as argument in the form of Table. Data can also be used as Vertical & Horizontal according to the scenario need.
But Specflow Table also provides extensive methods to make the best use of it. CreateSet in SpecFlow Table is also one of the best ways of Parameterizing Data in Horizontal format.
CreateSet<T>
is an extension method off of Table that will convert the table data to a set of objects.
CreateSet in SpecFlow Table
We will be using the same example of LogIn Feature and modify it according to our usage. Before moving on to this chapter, please have a look at the base chapter of Data Driven Testing and see how the simple functionality works for LogIn Scenario.
1) Create a New Step
The first step is to create a new Step which will take Data in Table format. It is again an easy job to specify data for the step in Horizontal format. Just provide the Header and their values.
When User enter credentials
| Username | Password |
| testuser_1 | Test@123 |
Note: Single row of Data or Multiple rows of Data can used here. CreateSet<T>
is capable of handling multiple data sets.
2) Create a New Step Definition
The second step is to create a new Steps Definition for newly created step, which can be done if bring the cursor on the created step and press F12. The Specflow will display the pop up with Skeleton body of the step, which can be copied and used accordingly.
[When(@"User enter credentials")]
public void WhenUserEnterCredentials(Table table)
{
}
3) Create Plain C# class object
<T>
denotes Type, which means any type of object can be used. It can be a string, int or Class Object. In our case, it is required to create a plain C# class with having two string object (Username & Password).
Create a new folder in the solution and name it as Utils. Then create a new C# class with the name Credentials in the same folder and create.
public class Credentials
{
public string Username { get; set; }
public string Password { get; set; }
}
4) Complete Step Definition Method
The CreateSet<T>
method will return an IEnumerable based on the data that matches in the table. It will fill the values for each object, doing the appropriate conversions from string to the related property. This can be used like:
IEnumerable<Credentials> credentials = table.CreateSet<Credentials>
();
Or
var credentials = table.CreateSet<Credentials>
();
[When(@"User enter credentials")]
public void WhenUserEnterCredentials(Table table)
{
var credentials = table.CreateSet<Credentials>();
foreach (var userData in credentials)
{
driver.FindElement(By.Id("log")).SendKeys(userData.Username);
driver.FindElement(By.Id("pwd")).SendKeys(userData.Password);
driver.FindElement(By.Id("login")).Click();
}
}
Note: With this, one can use the multiple Data sets for the same step.
The complete code will look like this:
LogIn_Feature File
Feature: LogIn_Feature
In order to access my account
As a user of the website
I want to log into the website
@mytag
Scenario: Successful Login with Valid Credentials
Given User is at the Home Page
And Navigate to LogIn Page
When User enter credentials
| Username | Password |
| testuser_1 | Test@123 |
And Click on the LogIn button
Then Successful LogIN message should display
Scenario: Successful LogOut
When User LogOut from the Application
Then Successful LogOut message should display
LogIn_Steps File
using OpenQA.Selenium;
using OpenQA.Selenium.Firefox;
using TechTalk.SpecFlow;
using TechTalk.SpecFlow.Assist;
using SpecFlowDemo.Utils;
namespace SpecFlowDemo.Steps
{
[Binding]
public class LogIn_Steps
{
public IWebDriver driver;
[Given(@"User is at the Home Page")]
public void GivenUserIsAtTheHomePage()
{
driver = new FirefoxDriver();
driver.Url = "https://www.store.demoqa.com";
}
[Given(@"Navigate to LogIn Page")]
public void GivenNavigateToLogInPage()
{
driver.FindElement(By.XPath(".//*[@id='account']/a")).Click();
}
[When(@"User enter credentials")]
public void WhenUserEnterCredentials(Table table)
{
var credentials = table.CreateSet<Credentials>();
foreach (var userData in credentials)
{
driver.FindElement(By.Id("log")).SendKeys(userData.Username);
driver.FindElement(By.Id("pwd")).SendKeys(userData.Password);
driver.FindElement(By.Id("login")).Click();
}
}
[When(@"Click on the LogIn button")]
public void WhenClickOnTheLogInButton()
{
driver.FindElement(By.Id("login")).Click();
}
[When(@"User LogOut from the Application")]
public void WhenUserLogOutFromTheApplication()
{
ScenarioContext.Current.Pending();
}
[Then(@"Successful LogIN message should display")]
public void ThenSuccessfulLogINMessageShouldDisplay()
{
//This Checks that if the LogOut button is displayed
true.Equals(driver.FindElement(By.XPath(".//*[@id='account_logout']/a")).Displayed);
}
[Then(@"Successful LogOut message should display")]
public void ThenSuccessfulLogOutMessageShouldDisplay()
{
ScenarioContext.Current.Pending();
}
}
}
Credentials Class File
namespace SpecFlowDemo.Utils
{
public class Credentials
{
public string Username { get; set; }
public string Password { get; set; }
}
}
Project Solution Explorer
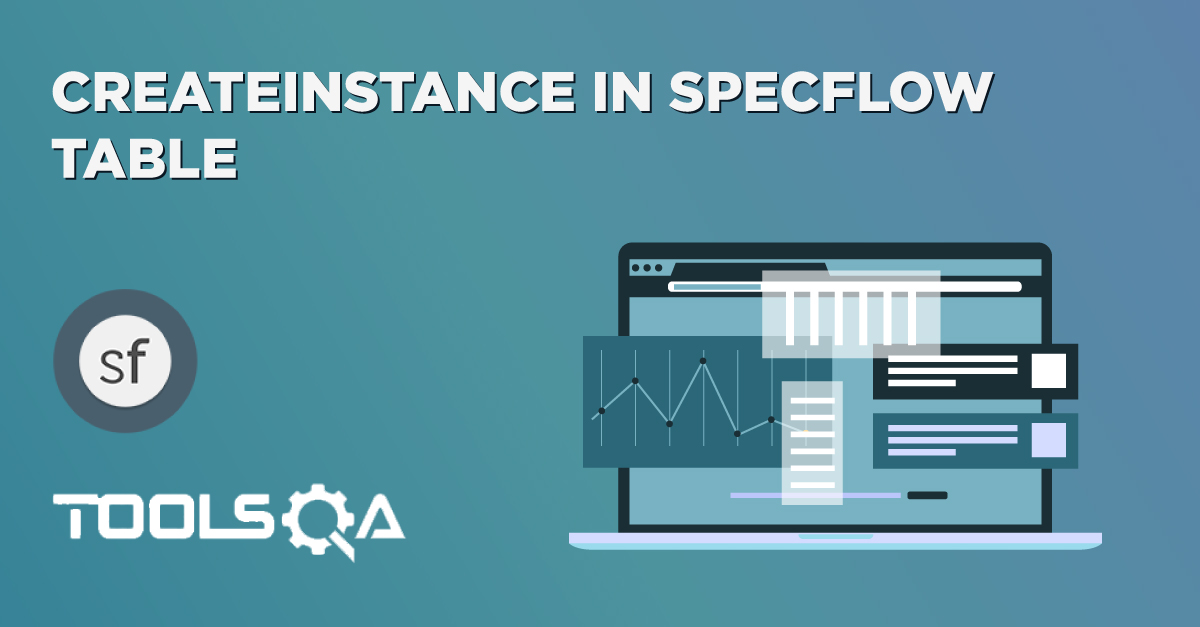
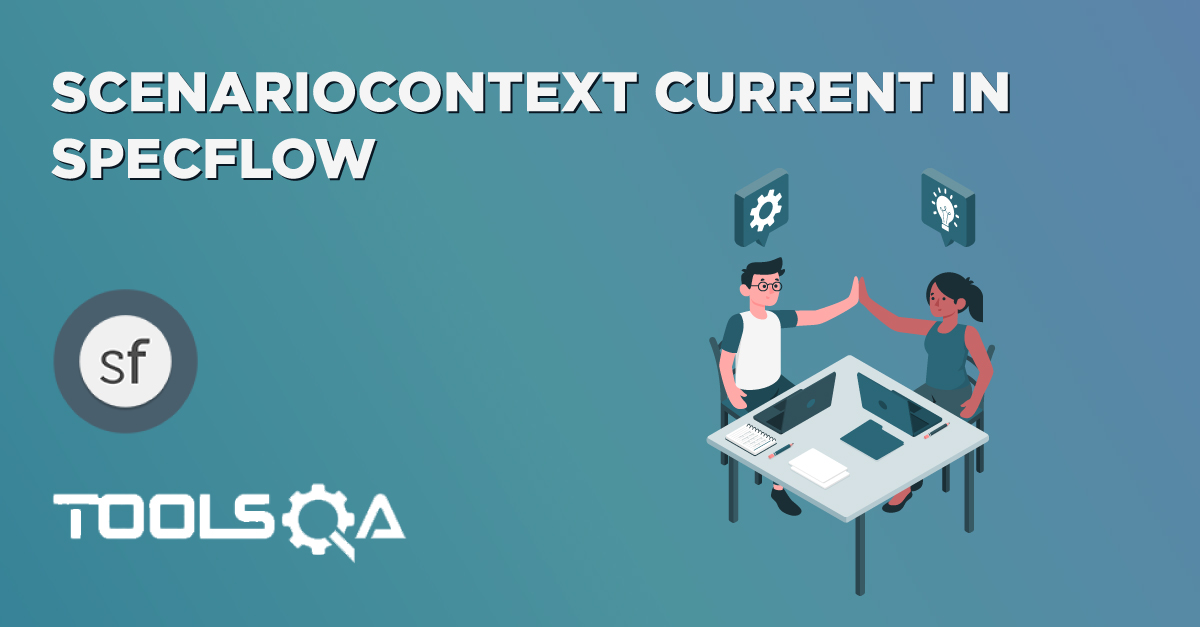