In our previous post of Retry Failed tests in TestNG we discussed about the IRetryAnalyzer Interface and various ways in which we can specify Retry Analyzer for a test. In this post we will look at the How to Implement IRetryAnalyzer to Retry Failed Test in TestNG Framework in a most design conscious way, so that we can have maintainable and easy to manage tests. This post will require you to have a good understanding of Java Annotations. We will create a custom annotation which we will use on a @Test
Method so that we can specify the retry count directly in the Test
Assuming that you have gone through the linked post already. It is necessary to understand what's written here.
1) Steps to Create a Custom Java Annotation
The first thing that we have to do is create an Java annotation that we will to annotate our test method. Lets say the name of the annotation is RetryCountIfFailed. This annotation is a pure Java annotation. Here is code to do that
package CustomAnnotations;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
public @interface RetryCountIfFailed {
// Specify how many times you want to
// retry the test if failed.
// Default value of retry count is 0
int value() default 0;
}
You can see that there is only one variable "value" inside the RetryCountIfFailed annotation. This value specifies how many times a test needs to be re-executed in case of failures. Note that the default value is set to 0. Also, one key point to note is that its a runtime annotation. Hence we have specified
@Retention(RetentionPolicy.RUNTIME)
2) Step to Use the Custom Created Java Annotation in TestNG Automation Test
One you have the annotation its time to use the annotation in the test code. This is the update Test code that uses this annotation.
package Tests;
import org.testng.Assert;
import org.testng.annotations.Test;
import CustomAnnotations.RetryCountIfFailed;
public class Test001 {
@Test
@RetryCountIfFailed(10)
public void Test1()
{
Assert.assertEquals(false, true);
}
@Test
public void Test2()
{
Assert.assertEquals(false, true);
}
}
Pay attention to the the usage of the RetryCountIfFailed annotation. First, it is used just like any other annotation by using the @ in front of the name (@RetryCountIfFailed
). Second a numeric value is passed to the annotation which specifies the number of times a failed test needs to be rerun. So the actual usage of the annotation becomes @RetryCountIfFailed(5)
, where 5 is the number of times you want this test to be rerun in case of failure. This value can be Integer value.
3) Implement IRetryAnalyzer to Retry Failed Test in TestNG Framework
With this annotation being used in tests its time to update the implementation of IRetryAnalyzer interface. There are the two things that we will need to do once a call to IRetryAnalyzer::retry method comes
- Check if the Test method for which retry is called has RetryCountIfFailed annotation
- Then compare current retry attempt with value of this annotation.
Here is the new implementation of RetryAnalyzer Class
package Listeners;
import org.testng.IRetryAnalyzer;
import org.testng.ITestResult;
import CustomAnnotations.RetryCountIfFailed;
public class RetryAnalyzer implements IRetryAnalyzer {
int counter = 0;
/*
* (non-Javadoc)
*
* @see org.testng.IRetryAnalyzer#retry(org.testng.ITestResult)
*
* This method decides how many times a test needs to be rerun. TestNg will
* call this method every time a test fails. So we can put some code in here
* to decide when to rerun the test.
*
* Note: This method will return true if a tests needs to be retried and
* false it not.
*
*/
@Override
public boolean retry(ITestResult result) {
// check if the test method had RetryCountIfFailed annotation
RetryCountIfFailed annotation = result.getMethod().getConstructorOrMethod().getMethod()
.getAnnotation(RetryCountIfFailed.class);
// based on the value of annotation see if test needs to be rerun
if((annotation != null) && (counter < annotation.value()))
{
counter++;
return true;
}
return false;
}
}
This way retry decision is taken based on the count specified in the RetryCountIfFailed annotation. Rest of the code remains same as explained in previous post. Now lets see how many times Test1 and Test2 are run.
Here you can see Test1 is run 10 times and Test2 is run just once. I hope I was able to explain this topic in details. You can also see/clone this project on github.
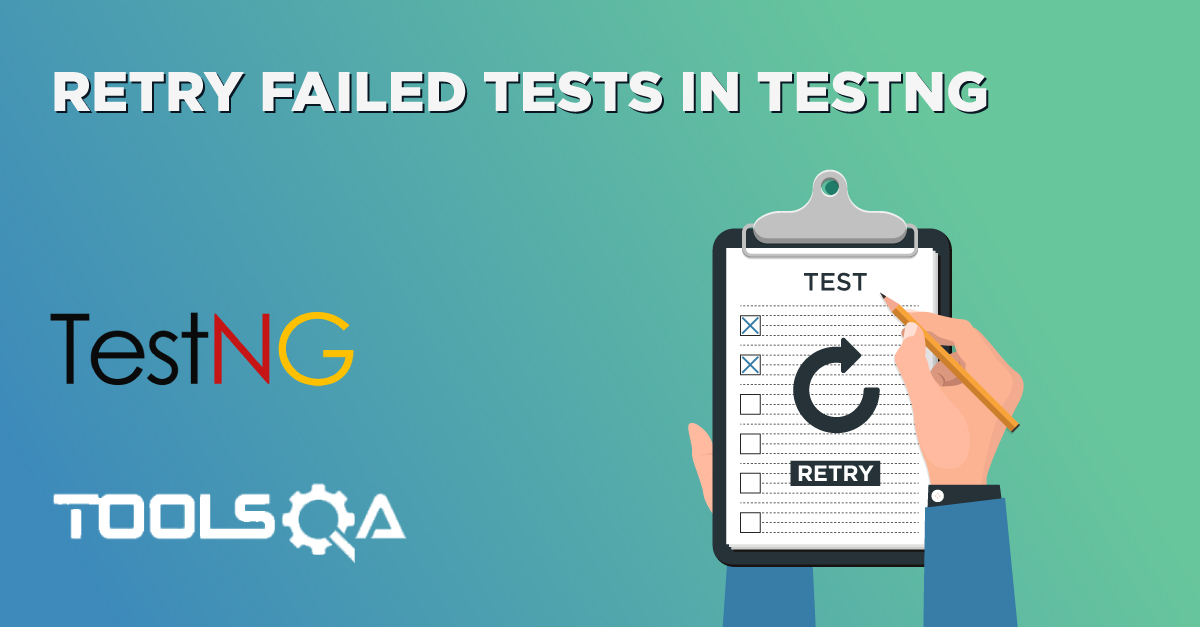
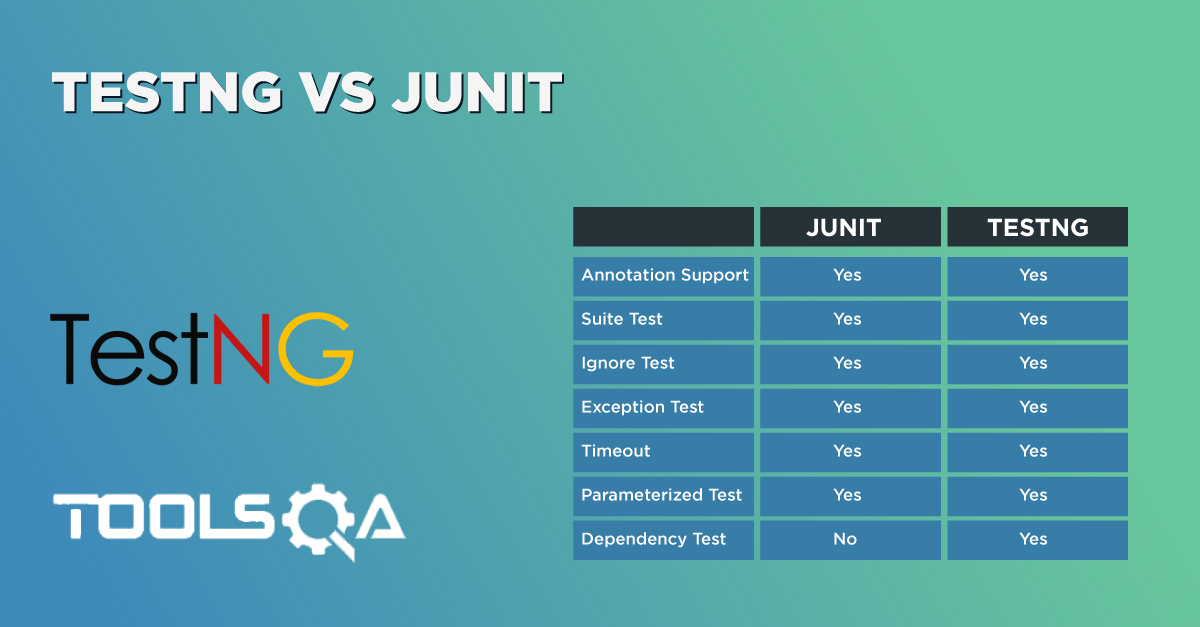