You must have noticed that we are using Action Keywords like 'click_MyAccount()', which is not at all a good practice. As there will be thousands of elements in any application and to click those elements we have to write thousands of click action keywords. So ideally there will be just one click action that should work on every other element on the test application. To achieve that, it is needed to separate the action from the object. For e.g. there will be an object called 'MyAccount' and an action called 'click()' and it would work like this: 'MyAccount.click()'
So our next task is to separate all the objects from the actions. To achieve that we need to create an Object Repository, which will hold all the objects properties in it and then those properties can be used in the main driver script. We can easily do this with the help of Property file. Normally, Java properties file is used to store project configuration data or settings. In this tutorial, we will show you how to set up and use a properties file. Time for action now.
Step 1: Set Up Object Repository properties file
- Create a new property file by right-clicking on 'config' package and select New > File and name it as 'OR'.
- Now take out all the object properties from Action Keywords class and put in 'OR' file.
- All the objects will be defined like this: Object Name = Object Property, where object property is element locator.
OR text File:
# Home Page Objects
btn_MyAccount=.//*[@id='account']/a
btn_LogOut=.//*[@id='account_logout']
# Login Page Object
txtbx_UserName=.//*[@id='log']
txtbx_Password=.//*[@id='pwd']
btn_LogIn=.//*[@id='login']
Step 2: Modify Data Engine sheet to separate Page Objects with Actions
- Insert an extra row in the 'dataEngine' excel sheet just before the 'Action Keywords' column.
- Name this new row as 'Page Object'.
- Add all the objects in the 'Page Object' column.
- Remove the objects names from the Action Keywords, only actions should be left in the Action Keywords column.
Excel file now look like this:
Step 3: Modify Action Keyword class to work with OR properties
- There were three click methods (click_MyAccount(), click_Login(), click_Logout()) in the previous chapter, replace all the click methods with just one method and name it as 'click()'.
- Now when we have just one click method, this method should know on which element to perform click action. For that it is required to pass the element as an argument to this method.
- This argument will be the Object name taken from the 'Page Object' column in the excel sheet.
- Modify the action methods, so that it can use the OR properties.
Keyword Action Class:
package config;
import java.util.concurrent.TimeUnit;
import static executionEngine.DriverScript.OR;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class ActionKeywords {
public static WebDriver driver;
//All the methods in this class now accept 'Object' name as an argument
public static void openBrowser(String object){
driver=new FirefoxDriver();
}
public static void navigate(String object){
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get(Constants.URL);
}
public static void click(String object){
//This is fetching the xpath of the element from the Object Repository property file
driver.findElement(By.xpath(OR.getProperty(object))).click();
}
public static void input_UserName(String object){
driver.findElement(By.xpath(OR.getProperty(object))).sendKeys(Constants.UserName);
}
public static void input_Password(String object){
driver.findElement(By.xpath(OR.getProperty(object))).sendKeys(Constants.Password);
}
public static void waitFor(String object) throws Exception{
Thread.sleep(5000);
}
public static void closeBrowser(String object){
driver.quit();
}
}
Note: We have still used 'input_Password()' and not detached this object from action. We will take care of this in next coming chapter of Data Driven.
Note: If you see carefully, object argument is passed in every method, even if it is not required in the method, such as 'closeBrowser()'. This is the mandatory condition of the reflection class that all the methods will have same arguments, even if the argument is not used in some methods.
Step 4: Changes in Constants class
- New entry in Constants class for the new column of Page Objects.
- Modify the value of the Action Keyword column, as we inserted a new column before the Action Keword column in dataEngine excel sheet.
Constants Variable Class:
package config;
public class Constants {
//List of System Variables
public static final String URL = "https://www.store.demoqa.com";
public static final String Path_TestData = "D://Tools QA Projects//trunk//Hybrid KeyWord Driven//src//dataEngine//DataEngine.xlsx";
public static final String Path_OR = "D://Tools QA Projects//trunk//Hybrid KeyWord Driven//src//config//OR.txt";
public static final String File_TestData = "DataEngine.xlsx";
//List of Data Sheet Column Numbers
public static final int Col_TestCaseID = 0;
public static final int Col_TestScenarioID =1 ;
//This is the new column for 'Page Object'
public static final int Col_PageObject =3 ;
//This column is shifted from 3 to 4
public static final int Col_ActionKeyword =4 ;
//List of Data Engine Excel sheets
public static final String Sheet_TestSteps = "Test Steps";
//List of Test Data
public static final String UserName = "testuser_3";
public static final String Password = "Test@123";
}
Step 5: Load OR properties in the Driver Script
Driver Script:
package executionEngine;
import java.io.FileInputStream;
import java.lang.reflect.Method;
import java.util.Properties;
import config.ActionKeywords;
import config.Constants;
import utility.ExcelUtils;
public class DriverScript {
public static Properties OR;
public static ActionKeywords actionKeywords;
public static String sActionKeyword;
public static String sPageObject;
public static Method method[];
public DriverScript() throws NoSuchMethodException, SecurityException{
actionKeywords = new ActionKeywords();
method = actionKeywords.getClass().getMethods();
}
public static void main(String[] args) throws Exception {
String Path_DataEngine = Constants.Path_TestData;
ExcelUtils.setExcelFile(Path_DataEngine, Constants.Sheet_TestSteps);
//Declaring String variable for storing Object Repository path
String Path_OR = Constants.Path_OR;
//Creating file system object for Object Repository text/property file
FileInputStream fs = new FileInputStream(Path_OR);
//Creating an Object of properties
OR= new Properties(System.getProperties());
//Loading all the properties from Object Repository property file in to OR object
OR.load(fs);
for (int iRow=1;iRow<=9;iRow++){
sActionKeyword = ExcelUtils.getCellData(iRow, Constants.Col_ActionKeyword);
sPageObject = ExcelUtils.getCellData(iRow, Constants.Col_PageObject);
execute_Actions();
}
}
private static void execute_Actions() throws Exception {
for(int i=0;i<method.length;i++){
if(method[i].getName().equals(sActionKeyword)){
//This is to execute the method or invoking the method
//Passing 'Page Object' name and 'Action Keyword' as Arguments to this method
method[i].invoke(actionKeywords,sPageObject);
break;
}
}
}
}
Note: Name of the object in the OR file is case sensitive, it has to matched exactly the same way it is mentioned in the DataEngine sheet.
Project folder would look like this:
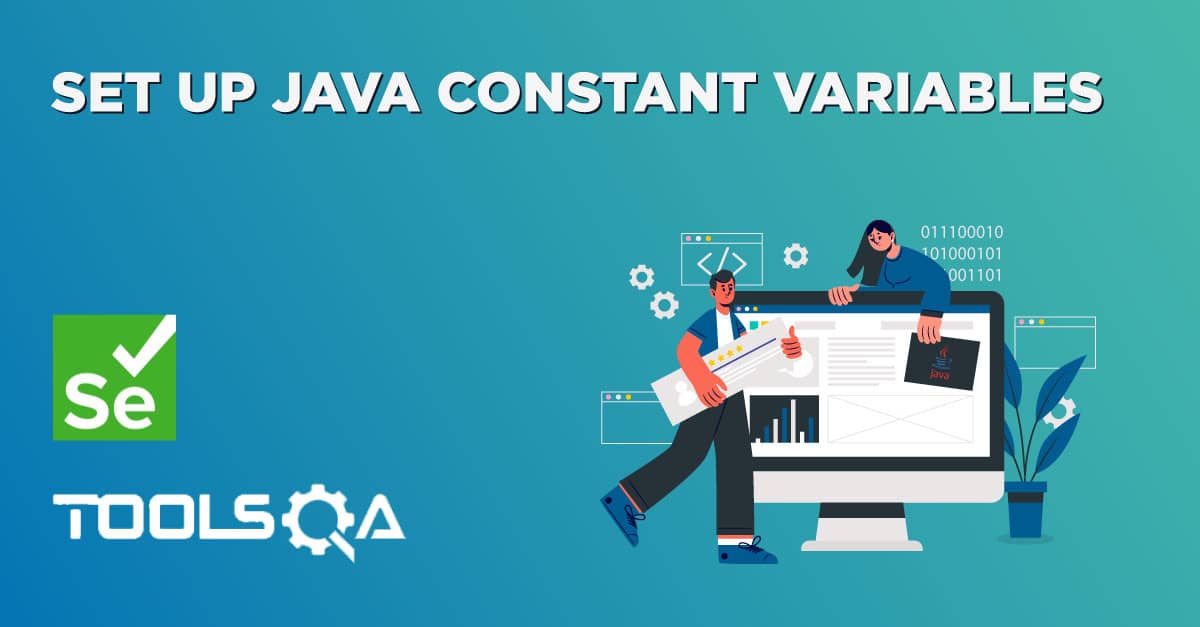
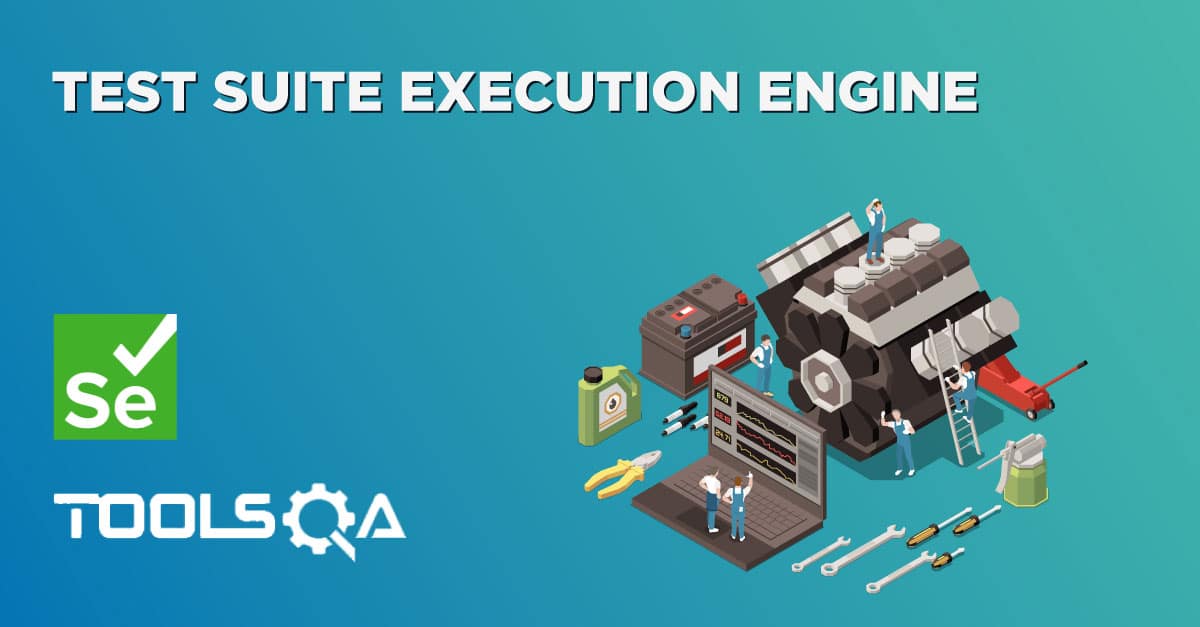